Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / EntitySql / QueryExpr.cs / 1 / QueryExpr.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; using System.Collections; using System.Collections.Generic; ////// represents select kind (value,row) /// internal enum SelectKind { SelectValue, SelectRow } ////// represents join kind (cross,inner,leftouter,rightouter) /// internal enum JoinKind { Cross, Inner, LeftOuter, FullOuter, RightOuter } ////// represents order kind (none=asc,asc,desc) /// internal enum OrderKind { None, Asc, Desc } ////// represents distinct kind (none=all,all,distinct) /// internal enum DistinctKind { None, All, Distinct } ////// represents apply kind (cross,outer) /// internal enum ApplyKind { Cross, Outer } ////// represents query ast node expression /// internal sealed class QueryExpr : Expr { private SelectClause _selectClause; private FromClause _fromClause; private Expr _whereClause; private GroupByClause _groupByClause; private HavingClause _havingClause; private OrderByClause _orderByClause; ////// initializes query ast node expression /// /// select clause /// from clasuse /// optional where clause /// optional group by clause /// optional having clause /// optional order by clause internal QueryExpr( SelectClause selectClause, FromClause fromClause, Expr whereClause, GroupByClause groupByClause, HavingClause havingClause, OrderByClause orderByClause ) { _selectClause = selectClause; _fromClause = fromClause; _whereClause = whereClause; _groupByClause = groupByClause; _havingClause = havingClause; _orderByClause = orderByClause; } ////// returns select clause /// internal SelectClause SelectClause { get { return _selectClause; } } ////// returns from clause /// internal FromClause FromClause { get { return _fromClause; } } ////// returns optional where clause (expr) /// internal Expr WhereClause { get { return _whereClause; } } ////// returns optional group by clause /// internal GroupByClause GroupByClause { get { return _groupByClause; } } ////// returns optional having clause (expr) /// internal HavingClause HavingClause { get { return _havingClause; } } ////// returns optional order by clause /// internal OrderByClause OrderByClause { get { return _orderByClause; } } ////// returns top level expression kind /// internal override AstExprKind ExprKind { get { return AstExprKind.Query; } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return _selectClause.HasMethodCall || (null != _havingClause && _havingClause.HasMethodCall ) || (null != _orderByClause && _orderByClause.HasMethodCall); } } } ////// Represents select clause /// internal sealed class SelectClause : Expr { private ExprList_selectClauseItems; private SelectKind _selectKind; private DistinctKind _distinctKind; private Expr _topExpr; private uint _methodCallCount; /// /// initialize Select Clause (row) /// /// /// /// /// internal SelectClause( ExprListitems, DistinctKind distinctKind, Expr topExpr, uint methodCallCount ) { _selectKind = SelectKind.SelectRow; _selectClauseItems = items; _distinctKind = distinctKind; _topExpr = topExpr; _methodCallCount = methodCallCount; } /// /// initializes single select clause /// /// /// /// /// internal SelectClause( Expr item, DistinctKind distinctKind, Expr topExpr, uint methodCallCount ) { _selectKind = SelectKind.SelectValue; _selectClauseItems = new ExprList(new AliasExpr(item)); _distinctKind = distinctKind; _topExpr = topExpr; _methodCallCount = methodCallCount; } /// /// projection list /// internal ExprListItems { get { return _selectClauseItems; } } /// /// select kind (row or item) /// internal SelectKind SelectKind { get { return _selectKind; } } ////// distinct kind (none,all,distinct) /// internal DistinctKind DistinctKind { get { return _distinctKind; } } ////// optional top expression /// internal Expr TopExpr { get { return _topExpr; } } ////// returns true if top expression is present /// internal bool HasTopClause { get { return (null != _topExpr); } } ////// Returns true if select list has ast method nodes /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// Represents from clause /// internal sealed class FromClause : Expr { ExprList_fromClauseItems; /// /// initializes from clause /// /// internal FromClause( ExprListfromClauseItems ) { _fromClauseItems = fromClauseItems; } /// /// list of from clause items /// internal ExprListFromClauseItems { get { return _fromClauseItems; } } } /// /// from clause item kind /// internal enum FromClauseItemKind { AliasedFromClause, JoinFromClause, ApplyFromClause } ////// represents single from clause item /// internal sealed class FromClauseItem : Expr { private Expr _fromClauseItemExpr; private FromClauseItemKind _fromClauseItemKind; ////// initializes as 'simple' aliased expression /// /// internal FromClauseItem( AliasExpr aliasExpr ) { _fromClauseItemExpr = aliasExpr; _fromClauseItemKind = FromClauseItemKind.AliasedFromClause; } ////// initializes as join clause /// /// internal FromClauseItem( JoinClauseItem joinClauseItem ) { _fromClauseItemExpr = joinClauseItem; _fromClauseItemKind = FromClauseItemKind.JoinFromClause; } ////// initializes as apply clause /// /// internal FromClauseItem( ApplyClauseItem applyClauseItem ) { _fromClauseItemExpr = applyClauseItem; _fromClauseItemKind = FromClauseItemKind.ApplyFromClause; } ////// From clause expression /// internal Expr FromExpr { get { return _fromClauseItemExpr; } } ////// from clause item kind (alias,join,apply) /// internal FromClauseItemKind FromClauseItemKind { get { return _fromClauseItemKind; } } } ////// Represents group by clause /// internal sealed class GroupByClause : Expr { private ExprList_groupItems; private Identifier _groupIdentifier; /// /// initializes as named group /// /// /// internal GroupByClause( ExprListgroupItems, Identifier groupIdentifier ) { _groupIdentifier = groupIdentifier; _groupItems = groupItems; } /// /// return group items /// internal ExprListGroupItems { get { return _groupItems; } } } /// /// Represents group by clause /// internal sealed class HavingClause : Expr { private Expr _havingExpr; private uint _methodCallCount; ////// Initializes Having clause /// /// /// internal HavingClause(Expr havingExpr, uint methodCallCounter) { _havingExpr = havingExpr; _methodCallCount = methodCallCounter; } ////// returns having inner expression /// internal Expr HavingPredicate { get { return _havingExpr; } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// represents order by clause /// internal sealed class OrderByClause : Expr { private ExprList_orderByClauseItem; private Expr _skipExpr; private Expr _limitExpr; private uint _methodCallCount; /// /// initializes Order by clause /// /// /// /// /// internal OrderByClause( ExprListorderByClauseItem, Expr skipExpr, Expr limitExpr, uint methodCallCount ) { _orderByClauseItem = orderByClauseItem; _skipExpr = skipExpr; _limitExpr = limitExpr; _methodCallCount = methodCallCount; } /// /// returns order by clause items /// internal ExprListOrderByClauseItem { get { return _orderByClauseItem; } } /// /// returns skip sub clause ast node /// internal Expr SkipSubClause { get { return _skipExpr; } } ////// returns true if Sort Expression has skip sub-clause /// internal bool HasSkipSubClause { get { return (null != _skipExpr); } } ////// returns limit sub-clause ast node /// internal Expr LimitSubClause { get { return _limitExpr; } } ////// returns true if Sort Expression has limit sub-clause /// internal bool HasLimitSubClause { get { return (null != _limitExpr); } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// represents a order by clause item /// internal sealed class OrderByClauseItem : Expr { private Expr _orderExpr; private OrderKind _orderKind; private Identifier _optCollationIdentifier; ////// initializes non-collated order by clause item /// /// /// internal OrderByClauseItem( Expr orderExpr, OrderKind orderKind ) : this(orderExpr, orderKind, null) { } ////// initializes collated order by clause item /// /// /// /// optional Collation identifier internal OrderByClauseItem( Expr orderExpr, OrderKind orderKind, Identifier optCollationIdentifier ) { _orderExpr = orderExpr; _orderKind = orderKind; _optCollationIdentifier = optCollationIdentifier; } ////// returns order expression /// internal Expr OrderExpr { get { return _orderExpr; } } ////// returns order kind (none,asc,desc) /// internal OrderKind OrderKind { get { return _orderKind; } } ////// returns collate identifier if one exists /// internal Identifier CollateIdentifier { get { return _optCollationIdentifier; } } ////// returns if order clause is collated /// internal bool IsCollated { get { return (null != _optCollationIdentifier); } } } ////// represents join clause item /// internal sealed class JoinClauseItem : Expr { private FromClauseItem _joinLeft; private FromClauseItem _joinRight; private JoinKind _joinKind; private Expr _onExpr; ////// initializes Join clause item without ON expression /// /// /// /// internal JoinClauseItem( FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind ) : this(joinLeft, joinRight, joinKind, null) { } ////// initializes Join clause item with ON expression /// /// /// /// /// internal JoinClauseItem( FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind, Expr onExpr ) { _joinLeft = joinLeft; _joinRight = joinRight; _joinKind = joinKind; _onExpr = onExpr; } ////// returns join left expression /// internal FromClauseItem LeftExpr { get { return _joinLeft; } } ////// returns join right expression /// internal FromClauseItem RightExpr { get { return _joinRight; } } ////// get/set join kind (cross, inner, full, left outer,right outer) /// internal JoinKind JoinKind { get { return _joinKind; } set { _joinKind = value; } } ////// returns on expression /// internal Expr OnExpr { get { return _onExpr; } } } ////// represents apply expression /// internal sealed class ApplyClauseItem : Expr { private FromClauseItem _applyLeft; private FromClauseItem _applyRight; private ApplyKind _applyKind; ////// initializes apply clause item /// /// /// /// internal ApplyClauseItem( FromClauseItem applyLeft, FromClauseItem applyRight, ApplyKind applyKind ) { _applyLeft = applyLeft; _applyRight = applyRight; _applyKind = applyKind; } ////// returns apply left expression /// internal FromClauseItem LeftExpr { get { return _applyLeft; } } ////// returns apply right expression /// internal FromClauseItem RightExpr { get { return _applyRight; } } ////// returns apply kind (cross,outer) /// internal ApplyKind ApplyKind { get { return _applyKind; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; using System.Collections; using System.Collections.Generic; ////// represents select kind (value,row) /// internal enum SelectKind { SelectValue, SelectRow } ////// represents join kind (cross,inner,leftouter,rightouter) /// internal enum JoinKind { Cross, Inner, LeftOuter, FullOuter, RightOuter } ////// represents order kind (none=asc,asc,desc) /// internal enum OrderKind { None, Asc, Desc } ////// represents distinct kind (none=all,all,distinct) /// internal enum DistinctKind { None, All, Distinct } ////// represents apply kind (cross,outer) /// internal enum ApplyKind { Cross, Outer } ////// represents query ast node expression /// internal sealed class QueryExpr : Expr { private SelectClause _selectClause; private FromClause _fromClause; private Expr _whereClause; private GroupByClause _groupByClause; private HavingClause _havingClause; private OrderByClause _orderByClause; ////// initializes query ast node expression /// /// select clause /// from clasuse /// optional where clause /// optional group by clause /// optional having clause /// optional order by clause internal QueryExpr( SelectClause selectClause, FromClause fromClause, Expr whereClause, GroupByClause groupByClause, HavingClause havingClause, OrderByClause orderByClause ) { _selectClause = selectClause; _fromClause = fromClause; _whereClause = whereClause; _groupByClause = groupByClause; _havingClause = havingClause; _orderByClause = orderByClause; } ////// returns select clause /// internal SelectClause SelectClause { get { return _selectClause; } } ////// returns from clause /// internal FromClause FromClause { get { return _fromClause; } } ////// returns optional where clause (expr) /// internal Expr WhereClause { get { return _whereClause; } } ////// returns optional group by clause /// internal GroupByClause GroupByClause { get { return _groupByClause; } } ////// returns optional having clause (expr) /// internal HavingClause HavingClause { get { return _havingClause; } } ////// returns optional order by clause /// internal OrderByClause OrderByClause { get { return _orderByClause; } } ////// returns top level expression kind /// internal override AstExprKind ExprKind { get { return AstExprKind.Query; } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return _selectClause.HasMethodCall || (null != _havingClause && _havingClause.HasMethodCall ) || (null != _orderByClause && _orderByClause.HasMethodCall); } } } ////// Represents select clause /// internal sealed class SelectClause : Expr { private ExprList_selectClauseItems; private SelectKind _selectKind; private DistinctKind _distinctKind; private Expr _topExpr; private uint _methodCallCount; /// /// initialize Select Clause (row) /// /// /// /// /// internal SelectClause( ExprListitems, DistinctKind distinctKind, Expr topExpr, uint methodCallCount ) { _selectKind = SelectKind.SelectRow; _selectClauseItems = items; _distinctKind = distinctKind; _topExpr = topExpr; _methodCallCount = methodCallCount; } /// /// initializes single select clause /// /// /// /// /// internal SelectClause( Expr item, DistinctKind distinctKind, Expr topExpr, uint methodCallCount ) { _selectKind = SelectKind.SelectValue; _selectClauseItems = new ExprList(new AliasExpr(item)); _distinctKind = distinctKind; _topExpr = topExpr; _methodCallCount = methodCallCount; } /// /// projection list /// internal ExprListItems { get { return _selectClauseItems; } } /// /// select kind (row or item) /// internal SelectKind SelectKind { get { return _selectKind; } } ////// distinct kind (none,all,distinct) /// internal DistinctKind DistinctKind { get { return _distinctKind; } } ////// optional top expression /// internal Expr TopExpr { get { return _topExpr; } } ////// returns true if top expression is present /// internal bool HasTopClause { get { return (null != _topExpr); } } ////// Returns true if select list has ast method nodes /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// Represents from clause /// internal sealed class FromClause : Expr { ExprList_fromClauseItems; /// /// initializes from clause /// /// internal FromClause( ExprListfromClauseItems ) { _fromClauseItems = fromClauseItems; } /// /// list of from clause items /// internal ExprListFromClauseItems { get { return _fromClauseItems; } } } /// /// from clause item kind /// internal enum FromClauseItemKind { AliasedFromClause, JoinFromClause, ApplyFromClause } ////// represents single from clause item /// internal sealed class FromClauseItem : Expr { private Expr _fromClauseItemExpr; private FromClauseItemKind _fromClauseItemKind; ////// initializes as 'simple' aliased expression /// /// internal FromClauseItem( AliasExpr aliasExpr ) { _fromClauseItemExpr = aliasExpr; _fromClauseItemKind = FromClauseItemKind.AliasedFromClause; } ////// initializes as join clause /// /// internal FromClauseItem( JoinClauseItem joinClauseItem ) { _fromClauseItemExpr = joinClauseItem; _fromClauseItemKind = FromClauseItemKind.JoinFromClause; } ////// initializes as apply clause /// /// internal FromClauseItem( ApplyClauseItem applyClauseItem ) { _fromClauseItemExpr = applyClauseItem; _fromClauseItemKind = FromClauseItemKind.ApplyFromClause; } ////// From clause expression /// internal Expr FromExpr { get { return _fromClauseItemExpr; } } ////// from clause item kind (alias,join,apply) /// internal FromClauseItemKind FromClauseItemKind { get { return _fromClauseItemKind; } } } ////// Represents group by clause /// internal sealed class GroupByClause : Expr { private ExprList_groupItems; private Identifier _groupIdentifier; /// /// initializes as named group /// /// /// internal GroupByClause( ExprListgroupItems, Identifier groupIdentifier ) { _groupIdentifier = groupIdentifier; _groupItems = groupItems; } /// /// return group items /// internal ExprListGroupItems { get { return _groupItems; } } } /// /// Represents group by clause /// internal sealed class HavingClause : Expr { private Expr _havingExpr; private uint _methodCallCount; ////// Initializes Having clause /// /// /// internal HavingClause(Expr havingExpr, uint methodCallCounter) { _havingExpr = havingExpr; _methodCallCount = methodCallCounter; } ////// returns having inner expression /// internal Expr HavingPredicate { get { return _havingExpr; } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// represents order by clause /// internal sealed class OrderByClause : Expr { private ExprList_orderByClauseItem; private Expr _skipExpr; private Expr _limitExpr; private uint _methodCallCount; /// /// initializes Order by clause /// /// /// /// /// internal OrderByClause( ExprListorderByClauseItem, Expr skipExpr, Expr limitExpr, uint methodCallCount ) { _orderByClauseItem = orderByClauseItem; _skipExpr = skipExpr; _limitExpr = limitExpr; _methodCallCount = methodCallCount; } /// /// returns order by clause items /// internal ExprListOrderByClauseItem { get { return _orderByClauseItem; } } /// /// returns skip sub clause ast node /// internal Expr SkipSubClause { get { return _skipExpr; } } ////// returns true if Sort Expression has skip sub-clause /// internal bool HasSkipSubClause { get { return (null != _skipExpr); } } ////// returns limit sub-clause ast node /// internal Expr LimitSubClause { get { return _limitExpr; } } ////// returns true if Sort Expression has limit sub-clause /// internal bool HasLimitSubClause { get { return (null != _limitExpr); } } ////// returns true if method calls are present /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// represents a order by clause item /// internal sealed class OrderByClauseItem : Expr { private Expr _orderExpr; private OrderKind _orderKind; private Identifier _optCollationIdentifier; ////// initializes non-collated order by clause item /// /// /// internal OrderByClauseItem( Expr orderExpr, OrderKind orderKind ) : this(orderExpr, orderKind, null) { } ////// initializes collated order by clause item /// /// /// /// optional Collation identifier internal OrderByClauseItem( Expr orderExpr, OrderKind orderKind, Identifier optCollationIdentifier ) { _orderExpr = orderExpr; _orderKind = orderKind; _optCollationIdentifier = optCollationIdentifier; } ////// returns order expression /// internal Expr OrderExpr { get { return _orderExpr; } } ////// returns order kind (none,asc,desc) /// internal OrderKind OrderKind { get { return _orderKind; } } ////// returns collate identifier if one exists /// internal Identifier CollateIdentifier { get { return _optCollationIdentifier; } } ////// returns if order clause is collated /// internal bool IsCollated { get { return (null != _optCollationIdentifier); } } } ////// represents join clause item /// internal sealed class JoinClauseItem : Expr { private FromClauseItem _joinLeft; private FromClauseItem _joinRight; private JoinKind _joinKind; private Expr _onExpr; ////// initializes Join clause item without ON expression /// /// /// /// internal JoinClauseItem( FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind ) : this(joinLeft, joinRight, joinKind, null) { } ////// initializes Join clause item with ON expression /// /// /// /// /// internal JoinClauseItem( FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind, Expr onExpr ) { _joinLeft = joinLeft; _joinRight = joinRight; _joinKind = joinKind; _onExpr = onExpr; } ////// returns join left expression /// internal FromClauseItem LeftExpr { get { return _joinLeft; } } ////// returns join right expression /// internal FromClauseItem RightExpr { get { return _joinRight; } } ////// get/set join kind (cross, inner, full, left outer,right outer) /// internal JoinKind JoinKind { get { return _joinKind; } set { _joinKind = value; } } ////// returns on expression /// internal Expr OnExpr { get { return _onExpr; } } } ////// represents apply expression /// internal sealed class ApplyClauseItem : Expr { private FromClauseItem _applyLeft; private FromClauseItem _applyRight; private ApplyKind _applyKind; ////// initializes apply clause item /// /// /// /// internal ApplyClauseItem( FromClauseItem applyLeft, FromClauseItem applyRight, ApplyKind applyKind ) { _applyLeft = applyLeft; _applyRight = applyRight; _applyKind = applyKind; } ////// returns apply left expression /// internal FromClauseItem LeftExpr { get { return _applyLeft; } } ////// returns apply right expression /// internal FromClauseItem RightExpr { get { return _applyRight; } } ////// returns apply kind (cross,outer) /// internal ApplyKind ApplyKind { get { return _applyKind; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
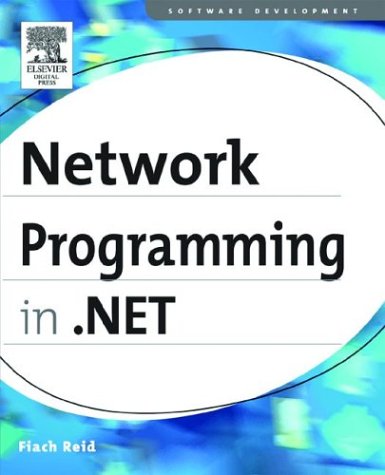
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CapiSymmetricAlgorithm.cs
- BaseCAMarshaler.cs
- AnnotationResource.cs
- ServiceParser.cs
- ActiveXHost.cs
- PlanCompiler.cs
- BrowserInteropHelper.cs
- WeakReferenceEnumerator.cs
- HostSecurityManager.cs
- UncommonField.cs
- InputBindingCollection.cs
- WebReferenceOptions.cs
- DataKey.cs
- StickyNoteHelper.cs
- ObjectSet.cs
- OleStrCAMarshaler.cs
- RepeatBehaviorConverter.cs
- DialogResultConverter.cs
- ObjRef.cs
- GrammarBuilderRuleRef.cs
- BezierSegment.cs
- TextElementEnumerator.cs
- TriggerCollection.cs
- ProfileSettingsCollection.cs
- EdmProviderManifest.cs
- PrintDocument.cs
- OletxTransactionFormatter.cs
- RSACryptoServiceProvider.cs
- PointLight.cs
- EventDescriptor.cs
- SafeLibraryHandle.cs
- TrustManager.cs
- DBConnection.cs
- StylusShape.cs
- fixedPageContentExtractor.cs
- SamlAuthorizationDecisionClaimResource.cs
- ReadContentAsBinaryHelper.cs
- WebHttpElement.cs
- EventHandlers.cs
- StrokeCollection.cs
- SoapObjectWriter.cs
- DataGridViewRowEventArgs.cs
- RawTextInputReport.cs
- SecurityElement.cs
- Splitter.cs
- XmlCompatibilityReader.cs
- DocumentReference.cs
- RecordsAffectedEventArgs.cs
- IgnoreSectionHandler.cs
- GlyphTypeface.cs
- ListControl.cs
- MouseCaptureWithinProperty.cs
- JsonGlobals.cs
- SqlOuterApplyReducer.cs
- ProgressChangedEventArgs.cs
- BoolLiteral.cs
- DeobfuscatingStream.cs
- ReadOnlyKeyedCollection.cs
- BamlReader.cs
- HwndPanningFeedback.cs
- ReaderWriterLock.cs
- DataGridViewRowCollection.cs
- Misc.cs
- DataSourceSelectArguments.cs
- DataGridState.cs
- EdmRelationshipRoleAttribute.cs
- FreeFormPanel.cs
- ComplexBindingPropertiesAttribute.cs
- WebPartZoneCollection.cs
- SqlDataSourceQueryEditor.cs
- AuthorizationRuleCollection.cs
- QuaternionAnimation.cs
- ProfileBuildProvider.cs
- ChangeConflicts.cs
- SerializationSectionGroup.cs
- DrawingBrush.cs
- ResourceAssociationSetEnd.cs
- BitmapImage.cs
- QuinticEase.cs
- PageCodeDomTreeGenerator.cs
- TransformDescriptor.cs
- HyperLinkStyle.cs
- Helper.cs
- MimeObjectFactory.cs
- StrongNameUtility.cs
- AssertFilter.cs
- ProviderSettingsCollection.cs
- XmlSchemaType.cs
- ProfilePropertyMetadata.cs
- SqlGenericUtil.cs
- Transactions.cs
- EventBuilder.cs
- ToolZone.cs
- ZoneMembershipCondition.cs
- LayoutSettings.cs
- Error.cs
- DataServiceHost.cs
- GeneralTransform3D.cs
- ParserExtension.cs
- CaseCqlBlock.cs