Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / UniqueEventHelper.cs / 1305600 / UniqueEventHelper.cs
using System; using System.Collections; using System.Diagnostics; namespace System.Windows.Media { ////// Aids in making events unique. i.e. you register the same function for /// the same event twice, but it will only be called once for each time /// the event is invoked. /// /// UniqueEventHelper should only be accessed from the UI thread so that /// handlers that throw exceptions will crash the app, making the developer /// aware of the problem. /// internal class UniqueEventHelperwhere TEventArgs : EventArgs { /// /// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } /// /// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } /// /// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, TEventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandlerhandler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } /// /// Clones the event helper /// internal UniqueEventHelperClone() { UniqueEventHelper ueh = new UniqueEventHelper (); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } /// /// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } // internal class UniqueEventHelper { ////// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } ////// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } ////// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, EventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandler handler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } ////// Clones the event helper /// internal UniqueEventHelper Clone() { UniqueEventHelper ueh = new UniqueEventHelper(); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } ////// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Diagnostics; namespace System.Windows.Media { ////// Aids in making events unique. i.e. you register the same function for /// the same event twice, but it will only be called once for each time /// the event is invoked. /// /// UniqueEventHelper should only be accessed from the UI thread so that /// handlers that throw exceptions will crash the app, making the developer /// aware of the problem. /// internal class UniqueEventHelperwhere TEventArgs : EventArgs { /// /// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } /// /// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } /// /// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, TEventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandlerhandler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } /// /// Clones the event helper /// internal UniqueEventHelperClone() { UniqueEventHelper ueh = new UniqueEventHelper (); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } /// /// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } // internal class UniqueEventHelper { ////// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } ////// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } ////// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, EventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandler handler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } ////// Clones the event helper /// internal UniqueEventHelper Clone() { UniqueEventHelper ueh = new UniqueEventHelper(); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } ////// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
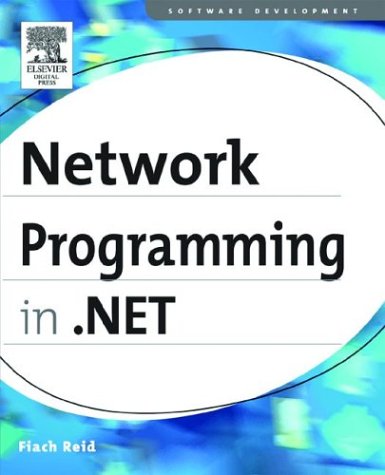
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonDialog.cs
- WriterOutput.cs
- WorkflowRequestContext.cs
- MemberProjectedSlot.cs
- MatrixAnimationBase.cs
- AnnotationStore.cs
- ContainerFilterService.cs
- ReachFixedDocumentSerializerAsync.cs
- SqlNode.cs
- DataContract.cs
- StructuralType.cs
- InfoCardProofToken.cs
- SemanticResolver.cs
- VerificationAttribute.cs
- CultureInfoConverter.cs
- DispatcherProcessingDisabled.cs
- TrackBarRenderer.cs
- DataGridViewColumnCollectionEditor.cs
- BevelBitmapEffect.cs
- SystemWebSectionGroup.cs
- DataSourceView.cs
- InheritablePropertyChangeInfo.cs
- HostingPreferredMapPath.cs
- HttpCachePolicyElement.cs
- EntityTransaction.cs
- GeneralTransform3DCollection.cs
- DateTimeConstantAttribute.cs
- PropertyNames.cs
- LayoutTableCell.cs
- TcpDuplicateContext.cs
- ImageConverter.cs
- FixedSOMContainer.cs
- autovalidator.cs
- MenuEventArgs.cs
- FileSystemEventArgs.cs
- XmlStreamNodeWriter.cs
- ItemCheckEvent.cs
- Content.cs
- JoinGraph.cs
- ArithmeticException.cs
- CodeChecksumPragma.cs
- SqlReferenceCollection.cs
- ContentDisposition.cs
- BooleanToVisibilityConverter.cs
- SqlXmlStorage.cs
- ExtendedTransformFactory.cs
- LowerCaseStringConverter.cs
- EntityDataSourceChangedEventArgs.cs
- LeafCellTreeNode.cs
- CommandConverter.cs
- commandenforcer.cs
- ProviderMetadataCachedInformation.cs
- TableProvider.cs
- CatalogZoneAutoFormat.cs
- WebDescriptionAttribute.cs
- WebBaseEventKeyComparer.cs
- ObjectStateFormatter.cs
- VSDExceptions.cs
- Asn1IntegerConverter.cs
- _FixedSizeReader.cs
- CodeDefaultValueExpression.cs
- ResourceDescriptionAttribute.cs
- PropertyDescriptorComparer.cs
- ProjectedSlot.cs
- UICuesEvent.cs
- EventLogHandle.cs
- GridViewDesigner.cs
- OpenTypeLayout.cs
- CellIdBoolean.cs
- XsltLibrary.cs
- CompModHelpers.cs
- ObjectQueryProvider.cs
- ClientRolePrincipal.cs
- RoleManagerEventArgs.cs
- EndpointConfigContainer.cs
- XPathParser.cs
- DataListItemEventArgs.cs
- HtmlTableRowCollection.cs
- WebPartAddingEventArgs.cs
- RadioButton.cs
- MasterPage.cs
- PathTooLongException.cs
- HandlerBase.cs
- RenderOptions.cs
- ObjectNotFoundException.cs
- XComponentModel.cs
- TrackingProfileCache.cs
- CallbackTimeoutsBehavior.cs
- OracleInternalConnection.cs
- XmlSchemaImport.cs
- RecordsAffectedEventArgs.cs
- MembershipPasswordException.cs
- HtmlHead.cs
- RichTextBox.cs
- WindowsMenu.cs
- MatrixCamera.cs
- DataBinder.cs
- TriggerBase.cs
- SafeFileMappingHandle.cs
- ProxyHelper.cs