Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / Behavior / ToolStripPanelSelectionGlyph.cs / 1 / ToolStripPanelSelectionGlyph.cs
namespace System.Windows.Forms.Design.Behavior { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Windows.Forms.Design; using System.Windows.Forms; ////// /// internal sealed class ToolStripPanelSelectionGlyph : ControlBodyGlyph { private ToolStripPanel relatedPanel; private Rectangle glyphBounds; private IServiceProvider provider; private ToolStripPanelSelectionBehavior relatedBehavior; private Image image = null; private Control baseParent = null; private BehaviorService behaviorService; private bool isExpanded = false; private const int imageWidth = 50; private const int imageHeight = 6; ////// /// internal ToolStripPanelSelectionGlyph(Rectangle bounds, Cursor cursor, IComponent relatedComponent, IServiceProvider provider, ToolStripPanelSelectionBehavior behavior) : base(bounds, cursor, relatedComponent, behavior) { relatedBehavior = behavior; this.provider = provider; this.relatedPanel = relatedComponent as ToolStripPanel; this.behaviorService = (BehaviorService)provider.GetService(typeof(BehaviorService)); if (behaviorService == null) { Debug.Fail("Could not get the BehaviorService"); return; } IDesignerHost host = (IDesignerHost)provider.GetService(typeof(IDesignerHost)); if (host == null) { Debug.Fail("Could not get the DesignerHost"); return; } UpdateGlyph(); } public bool IsExpanded { get { return isExpanded; } set { if (value != isExpanded) { isExpanded = value; UpdateGlyph(); } } } public void UpdateGlyph() { if (behaviorService != null) { Rectangle translatedBounds = behaviorService.ControlRectInAdornerWindow(relatedPanel); //Reset the glyph. this.glyphBounds = Rectangle.Empty; // Refresh the parent ToolStripContainer parent = relatedPanel.Parent as ToolStripContainer; if (parent != null) { this.baseParent = parent.Parent; // get the control to which ToolStripContainer is added... } if (!isExpanded) { CollapseGlyph(translatedBounds); } else { ExpandGlyph(translatedBounds); } } } private void CollapseGlyph(Rectangle bounds) { DockStyle dock = relatedPanel.Dock; int x = 0; int y = 0; switch(dock) { case DockStyle.Top : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "topopen.bmp"); x = (bounds.Width - imageWidth) /2; if (x > 0) { this.glyphBounds = new Rectangle(bounds.X + x, bounds.Y + bounds.Height, imageWidth, imageHeight); } break; case DockStyle.Bottom : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "bottomopen.bmp"); x = (bounds.Width - imageWidth) /2; if (x > 0) { this.glyphBounds = new Rectangle(bounds.X + x, bounds.Y - imageHeight, imageWidth, imageHeight); } break; case DockStyle.Left : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "leftopen.bmp"); y = (bounds.Height - imageWidth) /2; if (y > 0) { this.glyphBounds = new Rectangle(bounds.X + bounds.Width, bounds.Y + y, imageHeight, imageWidth); } break; case DockStyle.Right : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "rightopen.bmp"); y = (bounds.Height - imageWidth) /2; if (y > 0) { this.glyphBounds = new Rectangle(bounds.X - imageHeight , bounds.Y + y, imageHeight, imageWidth); } break; default: throw new Exception(SR.GetString(SR.ToolStripPanelGlyphUnsupportedDock)); } } private void ExpandGlyph(Rectangle bounds) { DockStyle dock = relatedPanel.Dock; int x = 0; int y = 0; switch(dock) { case DockStyle.Top : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "topclose.bmp"); x = (bounds.Width - imageWidth) /2; if (x > 0) { this.glyphBounds = new Rectangle(bounds.X + x, bounds.Y + bounds.Height, imageWidth, imageHeight); } break; case DockStyle.Bottom : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "bottomclose.bmp"); x = (bounds.Width - imageWidth) /2; if (x > 0) { this.glyphBounds = new Rectangle(bounds.X + x, bounds.Y -imageHeight, imageWidth, imageHeight); } break; case DockStyle.Left : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "leftclose.bmp"); y = (bounds.Height - imageWidth) /2; if (y > 0) { this.glyphBounds = new Rectangle(bounds.X + bounds.Width, bounds.Y + y, imageHeight, imageWidth); } break; case DockStyle.Right : this.image = new Bitmap(typeof(ToolStripPanelSelectionGlyph), "rightclose.bmp"); y = (bounds.Height - imageWidth) /2; if (y > 0) { this.glyphBounds = new Rectangle(bounds.X - imageHeight, bounds.Y + y, imageHeight, imageWidth); } break; default: throw new Exception(SR.GetString(SR.ToolStripPanelGlyphUnsupportedDock)); } } ////// /// The bounds of this Glyph. /// public override Rectangle Bounds { get { return glyphBounds; } } ////// /// Simple hit test rule: if the point is contained within the bounds /// - then it is a positive hit test. /// public override Cursor GetHitTest(Point p) { if (behaviorService != null && baseParent != null) { Rectangle baseParentBounds = behaviorService.ControlRectInAdornerWindow(baseParent); if (glyphBounds != Rectangle.Empty && baseParentBounds.Contains(glyphBounds) && glyphBounds.Contains(p)) { return Cursors.Hand; } } return null; } ////// /// Very simple paint logic. /// public override void Paint(PaintEventArgs pe) { if (behaviorService != null && baseParent != null) { Rectangle baseParentBounds = behaviorService.ControlRectInAdornerWindow(baseParent); if (relatedPanel.Visible && image != null && glyphBounds != Rectangle.Empty && baseParentBounds.Contains(glyphBounds)) { pe.Graphics.DrawImage(image, glyphBounds.Left, glyphBounds.Top); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
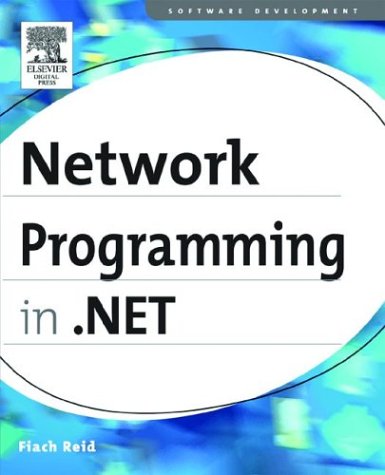
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- cryptoapiTransform.cs
- RecordsAffectedEventArgs.cs
- HttpProtocolImporter.cs
- DesignerProperties.cs
- CompilerCollection.cs
- CollectionContainer.cs
- DataColumnPropertyDescriptor.cs
- MouseActionConverter.cs
- Pts.cs
- SqlMethodAttribute.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- mediapermission.cs
- WindowsSecurityToken.cs
- InteropAutomationProvider.cs
- NegotiateStream.cs
- SelectionEditingBehavior.cs
- odbcmetadatafactory.cs
- VirtualStackFrame.cs
- ProjectionCamera.cs
- xmlformatgeneratorstatics.cs
- ChangePassword.cs
- DbProviderManifest.cs
- DescendantBaseQuery.cs
- ToolstripProfessionalRenderer.cs
- NodeInfo.cs
- ExpressionNode.cs
- SqlErrorCollection.cs
- InlineUIContainer.cs
- ToolStripArrowRenderEventArgs.cs
- recordstatefactory.cs
- ListViewGroupConverter.cs
- FormatConvertedBitmap.cs
- Rotation3DAnimationUsingKeyFrames.cs
- SQlBooleanStorage.cs
- FieldToken.cs
- SessionStateSection.cs
- TableDesigner.cs
- TransformationRules.cs
- Column.cs
- Mappings.cs
- CompiledELinqQueryState.cs
- ListMarkerSourceInfo.cs
- AsymmetricSignatureDeformatter.cs
- DecimalConstantAttribute.cs
- VersionedStream.cs
- MessageUtil.cs
- DESCryptoServiceProvider.cs
- WebPartConnectionsEventArgs.cs
- DrawingCollection.cs
- TypedRowGenerator.cs
- PathFigureCollection.cs
- XXXInfos.cs
- OrderingInfo.cs
- SessionEndingEventArgs.cs
- OrCondition.cs
- BinaryObjectWriter.cs
- Keyboard.cs
- ScriptManagerProxy.cs
- LateBoundBitmapDecoder.cs
- CustomErrorsSection.cs
- ColorAnimationUsingKeyFrames.cs
- StreamGeometry.cs
- Variable.cs
- BinaryKeyIdentifierClause.cs
- StickyNote.cs
- Config.cs
- WindowsSpinner.cs
- COSERVERINFO.cs
- HandleCollector.cs
- Stream.cs
- PrivateFontCollection.cs
- DisplayNameAttribute.cs
- VisemeEventArgs.cs
- SecurityUtils.cs
- CodeMemberMethod.cs
- MenuItemStyle.cs
- IndexOutOfRangeException.cs
- XslTransformFileEditor.cs
- TemplateColumn.cs
- ButtonColumn.cs
- ControlType.cs
- OdbcRowUpdatingEvent.cs
- WebServiceParameterData.cs
- PathGradientBrush.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WizardStepBase.cs
- WebPartTransformer.cs
- ContractMethodParameterInfo.cs
- CreateUserWizardStep.cs
- Unit.cs
- DbgUtil.cs
- SafeRightsManagementQueryHandle.cs
- UICuesEvent.cs
- OracleDataAdapter.cs
- PixelFormatConverter.cs
- BatchParser.cs
- TextTreeTextElementNode.cs
- EventHandlersStore.cs
- BooleanAnimationBase.cs
- DataGridViewRowPrePaintEventArgs.cs