Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Automation / InteropAutomationProvider.cs / 1 / InteropAutomationProvider.cs
using System; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; namespace MS.Internal.Automation { [FriendAccessAllowed] // Built into Core, also used by Framework. internal class InteropAutomationProvider: IRawElementProviderFragmentRoot { #region Constructors internal InteropAutomationProvider(HostedWindowWrapper wrapper, AutomationPeer parent) { if (wrapper == null) { throw new ArgumentNullException("wrapper"); } if (parent == null) { throw new ArgumentNullException("parent"); } _wrapper = wrapper; _parent = parent; } #endregion Constructors #region IRawElementProviderSimple /// ProviderOptions IRawElementProviderSimple.ProviderOptions { get { return ProviderOptions.ServerSideProvider | ProviderOptions.OverrideProvider; } } /// object IRawElementProviderSimple.GetPatternProvider(int patternId) { return null; } /// object IRawElementProviderSimple.GetPropertyValue(int propertyId) { return null; } ////// Critical - Calls critical HostedWindowWrapper.Handle. /// TreatAsSafe - The reason is described in the following comment by BrendanM /// HostProviderFromHandle is a public method the APTCA assembly UIAutomationProvider.dll; /// ...\windows\AccessibleTech\longhorn\Automation\UIAutomationProvider\System\Windows\Automation\Provider\AutomationInteropProvider.cs /// This calls through to an internal P/Invoke layer... /// ...\windows\AccessibleTech\longhorn\Automation\UIAutomationProvider\MS\Internal\Automation\UiaCoreProviderApi.cs /// Which P/Invokes to unmanaged UIAutomationCore.dll's UiaHostProviderFromHwnd API, /// ...\windows\AccessibleTech\longhorn\Automation\UnmanagedCore\UIAutomationCoreAPI.cpp /// Which checks the HWND with IsWindow, and returns a new MiniHwndProxy instance: /// ...\windows\AccessibleTech\longhorn\Automation\UnmanagedCore\MiniHwndProxy.cpp /// /// MiniHwndProxy does implement the IRawElementProviderSimple interface, but all methods /// return NULL or empty values; it does not expose any values or functionality through this. /// This object is designed to be an opaque cookie to contain the HWND so that only UIACore /// itself can access it. UIACore accesses the HWND by QI'ing for a private GUID, and then /// casting the returnd value to MiniHwndProxy, and calling a nonvirtual method to access a /// _hwnd field. While managed PT code maybe able to do a QI, the only way it could extract /// the _hwnd field would be by using unmanaged code. /// IRawElementProviderSimple IRawElementProviderSimple.HostRawElementProvider { [SecurityCritical, SecurityTreatAsSafe] get { return AutomationInteropProvider.HostProviderFromHandle(_wrapper.Handle); } } #endregion IRawElementProviderSimple #region IRawElementProviderFragment ////// TreatAsSafe - The reason this method can be treated as safe is because it yeilds information /// about the parent provider which can even otherwise be obtained by using public APIs such /// as UIElement.OnCreateAutomationPeer and AutomationProvider.ProviderFromPeer. /// IRawElementProviderFragment IRawElementProviderFragment.Navigate(NavigateDirection direction) { if (direction == NavigateDirection.Parent) { return (IRawElementProviderFragment)_parent.ProviderFromPeer(_parent); } return null; } /// int [] IRawElementProviderFragment.GetRuntimeId() { return null; } /// Rect IRawElementProviderFragment.BoundingRectangle { get { return Rect.Empty; } } /// IRawElementProviderSimple [] IRawElementProviderFragment.GetEmbeddedFragmentRoots() { return null; } /// void IRawElementProviderFragment.SetFocus() { throw new NotSupportedException(); } /// IRawElementProviderFragmentRoot IRawElementProviderFragment.FragmentRoot { get { return null; } } #endregion IRawElementProviderFragment #region IRawElementProviderFragmentRoot /// IRawElementProviderFragment IRawElementProviderFragmentRoot.ElementProviderFromPoint( double x, double y ) { return null; } /// IRawElementProviderFragment IRawElementProviderFragmentRoot.GetFocus() { return null; } #endregion IRawElementProviderFragmentRoot #region Data private HostedWindowWrapper _wrapper; private AutomationPeer _parent; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; namespace MS.Internal.Automation { [FriendAccessAllowed] // Built into Core, also used by Framework. internal class InteropAutomationProvider: IRawElementProviderFragmentRoot { #region Constructors internal InteropAutomationProvider(HostedWindowWrapper wrapper, AutomationPeer parent) { if (wrapper == null) { throw new ArgumentNullException("wrapper"); } if (parent == null) { throw new ArgumentNullException("parent"); } _wrapper = wrapper; _parent = parent; } #endregion Constructors #region IRawElementProviderSimple /// ProviderOptions IRawElementProviderSimple.ProviderOptions { get { return ProviderOptions.ServerSideProvider | ProviderOptions.OverrideProvider; } } /// object IRawElementProviderSimple.GetPatternProvider(int patternId) { return null; } /// object IRawElementProviderSimple.GetPropertyValue(int propertyId) { return null; } ////// Critical - Calls critical HostedWindowWrapper.Handle. /// TreatAsSafe - The reason is described in the following comment by BrendanM /// HostProviderFromHandle is a public method the APTCA assembly UIAutomationProvider.dll; /// ...\windows\AccessibleTech\longhorn\Automation\UIAutomationProvider\System\Windows\Automation\Provider\AutomationInteropProvider.cs /// This calls through to an internal P/Invoke layer... /// ...\windows\AccessibleTech\longhorn\Automation\UIAutomationProvider\MS\Internal\Automation\UiaCoreProviderApi.cs /// Which P/Invokes to unmanaged UIAutomationCore.dll's UiaHostProviderFromHwnd API, /// ...\windows\AccessibleTech\longhorn\Automation\UnmanagedCore\UIAutomationCoreAPI.cpp /// Which checks the HWND with IsWindow, and returns a new MiniHwndProxy instance: /// ...\windows\AccessibleTech\longhorn\Automation\UnmanagedCore\MiniHwndProxy.cpp /// /// MiniHwndProxy does implement the IRawElementProviderSimple interface, but all methods /// return NULL or empty values; it does not expose any values or functionality through this. /// This object is designed to be an opaque cookie to contain the HWND so that only UIACore /// itself can access it. UIACore accesses the HWND by QI'ing for a private GUID, and then /// casting the returnd value to MiniHwndProxy, and calling a nonvirtual method to access a /// _hwnd field. While managed PT code maybe able to do a QI, the only way it could extract /// the _hwnd field would be by using unmanaged code. /// IRawElementProviderSimple IRawElementProviderSimple.HostRawElementProvider { [SecurityCritical, SecurityTreatAsSafe] get { return AutomationInteropProvider.HostProviderFromHandle(_wrapper.Handle); } } #endregion IRawElementProviderSimple #region IRawElementProviderFragment ////// TreatAsSafe - The reason this method can be treated as safe is because it yeilds information /// about the parent provider which can even otherwise be obtained by using public APIs such /// as UIElement.OnCreateAutomationPeer and AutomationProvider.ProviderFromPeer. /// IRawElementProviderFragment IRawElementProviderFragment.Navigate(NavigateDirection direction) { if (direction == NavigateDirection.Parent) { return (IRawElementProviderFragment)_parent.ProviderFromPeer(_parent); } return null; } /// int [] IRawElementProviderFragment.GetRuntimeId() { return null; } /// Rect IRawElementProviderFragment.BoundingRectangle { get { return Rect.Empty; } } /// IRawElementProviderSimple [] IRawElementProviderFragment.GetEmbeddedFragmentRoots() { return null; } /// void IRawElementProviderFragment.SetFocus() { throw new NotSupportedException(); } /// IRawElementProviderFragmentRoot IRawElementProviderFragment.FragmentRoot { get { return null; } } #endregion IRawElementProviderFragment #region IRawElementProviderFragmentRoot /// IRawElementProviderFragment IRawElementProviderFragmentRoot.ElementProviderFromPoint( double x, double y ) { return null; } /// IRawElementProviderFragment IRawElementProviderFragmentRoot.GetFocus() { return null; } #endregion IRawElementProviderFragmentRoot #region Data private HostedWindowWrapper _wrapper; private AutomationPeer _parent; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
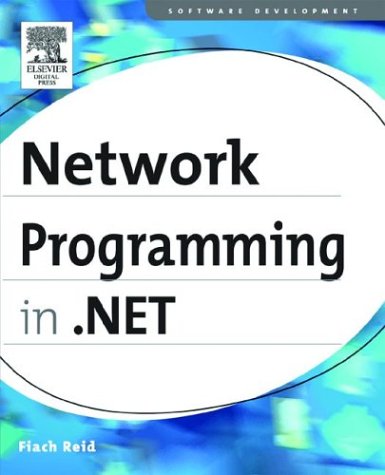
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlProcessingInstruction.cs
- SQLMembershipProvider.cs
- PagedDataSource.cs
- AxHost.cs
- DelegateHelpers.Generated.cs
- ButtonPopupAdapter.cs
- XamlSerializerUtil.cs
- ExtensionWindowHeader.cs
- ExpressionEditorSheet.cs
- ArrayList.cs
- InlinedAggregationOperatorEnumerator.cs
- LabelDesigner.cs
- columnmapfactory.cs
- ObjectQueryProvider.cs
- TextInfo.cs
- PersonalizationStateInfoCollection.cs
- XmlAttribute.cs
- EndEvent.cs
- DataGridViewCheckBoxCell.cs
- AnonymousIdentificationModule.cs
- LayoutEngine.cs
- GridViewColumnCollectionChangedEventArgs.cs
- CaseKeyBox.xaml.cs
- XmlKeywords.cs
- ValidationVisibilityAttribute.cs
- LinkedList.cs
- XmlLanguageConverter.cs
- BaseValidator.cs
- RelatedImageListAttribute.cs
- AspCompat.cs
- RemoteWebConfigurationHostStream.cs
- StructuralObject.cs
- SqlMethodAttribute.cs
- MexNamedPipeBindingElement.cs
- Soap12ProtocolReflector.cs
- MembershipValidatePasswordEventArgs.cs
- RijndaelManaged.cs
- Soap11ServerProtocol.cs
- EntityClassGenerator.cs
- SecurityDocument.cs
- SamlAssertionKeyIdentifierClause.cs
- Material.cs
- BindValidationContext.cs
- PartialList.cs
- MonitoringDescriptionAttribute.cs
- GridViewColumnHeader.cs
- Point3DCollection.cs
- AddInPipelineAttributes.cs
- ToolStripSplitStackLayout.cs
- input.cs
- ObjRef.cs
- ListBoxChrome.cs
- MailBnfHelper.cs
- ClientOptions.cs
- _NTAuthentication.cs
- TypeToken.cs
- IsolatedStorageFile.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- ScriptControl.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- SourceSwitch.cs
- CharEnumerator.cs
- PixelFormats.cs
- WizardStepBase.cs
- TransformDescriptor.cs
- SafeSystemMetrics.cs
- RouteParser.cs
- TextEditorParagraphs.cs
- WebSysDescriptionAttribute.cs
- SchemaInfo.cs
- PropertyPathWorker.cs
- TextTreeObjectNode.cs
- FlowLayout.cs
- DynamicRendererThreadManager.cs
- PersonalizableAttribute.cs
- TextDpi.cs
- PagedDataSource.cs
- TransportDefaults.cs
- WebRequestModuleElementCollection.cs
- CommonRemoteMemoryBlock.cs
- storepermission.cs
- QueryComponents.cs
- NativeMethods.cs
- SystemFonts.cs
- TypedTableBaseExtensions.cs
- Light.cs
- DNS.cs
- XmlEnumAttribute.cs
- OutputBuffer.cs
- Marshal.cs
- SchemaNames.cs
- DBDataPermission.cs
- ConstrainedGroup.cs
- CaseStatementSlot.cs
- Rotation3DAnimation.cs
- PointAnimationUsingPath.cs
- Literal.cs
- RegexBoyerMoore.cs
- SinglePageViewer.cs
- TemplatedEditableDesignerRegion.cs