Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / SharedMemory.cs / 1 / SharedMemory.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security.Principal; using System.ServiceModel.Channels; using System.Threading; using System.ServiceModel; using System.ComponentModel; unsafe class SharedMemory : IDisposable { SafeFileMappingHandle fileMapping; SharedMemory(SafeFileMappingHandle fileMapping) { this.fileMapping = fileMapping; } public static unsafe SharedMemory Create(string name, Guid content, ListallowedSids) { int errorCode = UnsafeNativeMethods.ERROR_SUCCESS; byte[] binarySecurityDescriptor = SecurityDescriptorHelper.FromSecurityIdentifiers(allowedSids, UnsafeNativeMethods.GENERIC_READ); SafeFileMappingHandle fileMapping; UnsafeNativeMethods.SECURITY_ATTRIBUTES securityAttributes = new UnsafeNativeMethods.SECURITY_ATTRIBUTES(); fixed (byte* pinnedSecurityDescriptor = binarySecurityDescriptor) { securityAttributes.lpSecurityDescriptor = (IntPtr)pinnedSecurityDescriptor; fileMapping = UnsafeNativeMethods.CreateFileMapping((IntPtr)(-1), securityAttributes, UnsafeNativeMethods.PAGE_READWRITE, 0, sizeof(SharedMemoryContents), name); errorCode = Marshal.GetLastWin32Error(); } if (fileMapping.IsInvalid) { fileMapping.SetHandleAsInvalid(); fileMapping.Close(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } SharedMemory sharedMemory = new SharedMemory(fileMapping); SafeViewOfFileHandle view; // Ignore return value. GetView(fileMapping, true, out view); try { SharedMemoryContents* contents = (SharedMemoryContents*)view.DangerousGetHandle(); contents->pipeGuid = content; Thread.MemoryBarrier(); contents->isInitialized = true; return sharedMemory; } finally { view.Close(); } } public void Dispose() { if (fileMapping != null) { fileMapping.Close(); fileMapping = null; } } static bool GetView(SafeFileMappingHandle fileMapping, bool writable, out SafeViewOfFileHandle handle) { handle = UnsafeNativeMethods.MapViewOfFile(fileMapping, writable ? UnsafeNativeMethods.FILE_MAP_WRITE : UnsafeNativeMethods.FILE_MAP_READ, 0, 0, (IntPtr)sizeof(SharedMemoryContents)); int errorCode = Marshal.GetLastWin32Error(); if (!handle.IsInvalid) { return true; } else { handle.SetHandleAsInvalid(); fileMapping.Close(); // Only return false when it's reading time. if (!writable && errorCode == UnsafeNativeMethods.ERROR_FILE_NOT_FOUND) { return false; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } } public static string Read(string name) { string content; if (Read(name, out content)) { return content; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(UnsafeNativeMethods.ERROR_FILE_NOT_FOUND)); } public static bool Read(string name, out string content) { content = null; SafeFileMappingHandle fileMapping = UnsafeNativeMethods.OpenFileMapping(UnsafeNativeMethods.FILE_MAP_READ, false, ListenerConstants.GlobalPrefix + name); int errorCode = Marshal.GetLastWin32Error(); if (fileMapping.IsInvalid) { fileMapping.SetHandleAsInvalid(); fileMapping.Close(); if (errorCode == UnsafeNativeMethods.ERROR_FILE_NOT_FOUND) { return false; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(errorCode)); } try { SafeViewOfFileHandle view; if (!GetView(fileMapping, false, out view)) { return false; } try { SharedMemoryContents* contents = (SharedMemoryContents*)view.DangerousGetHandle(); content = contents->isInitialized ? contents->pipeGuid.ToString() : null; return true; } finally { view.Close(); } } finally { fileMapping.Close(); } } [StructLayout(LayoutKind.Sequential)] struct SharedMemoryContents { public bool isInitialized; public Guid pipeGuid; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
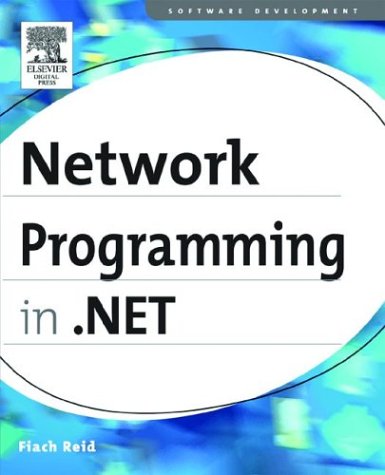
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SendReply.cs
- _FtpControlStream.cs
- FixedStringLookup.cs
- CodeDirectoryCompiler.cs
- WsdlHelpGeneratorElement.cs
- PointLight.cs
- SafeHandles.cs
- SQLStringStorage.cs
- QilTernary.cs
- ReflectionTypeLoadException.cs
- XmlUnspecifiedAttribute.cs
- CapacityStreamGeometryContext.cs
- KnownTypesProvider.cs
- ThemeDirectoryCompiler.cs
- DataSourceCollectionBase.cs
- WebControlsSection.cs
- AdornerLayer.cs
- RegexWriter.cs
- ExpressionStringBuilder.cs
- NetTcpSecurityElement.cs
- BuilderPropertyEntry.cs
- BindingNavigatorDesigner.cs
- _Connection.cs
- SqlTransaction.cs
- TreeNodeCollection.cs
- AttributeCollection.cs
- MemberRelationshipService.cs
- WebPartVerbsEventArgs.cs
- Authorization.cs
- WebPartEditorCancelVerb.cs
- Expander.cs
- UnsafeNativeMethods.cs
- TriggerBase.cs
- MetaModel.cs
- EdmTypeAttribute.cs
- ObjectTypeMapping.cs
- PrintPreviewDialog.cs
- VirtualizingPanel.cs
- StatusBarAutomationPeer.cs
- EFDataModelProvider.cs
- ClosableStream.cs
- ConfigXmlComment.cs
- CrossSiteScriptingValidation.cs
- MultiBinding.cs
- ImportContext.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- ReadOnlyDataSource.cs
- InvalidCastException.cs
- X509Certificate.cs
- AuthorizationRuleCollection.cs
- ComContractElement.cs
- ActivityDesignerAccessibleObject.cs
- KeyTimeConverter.cs
- RawStylusInput.cs
- SingleKeyFrameCollection.cs
- SocketInformation.cs
- State.cs
- HtmlTableCell.cs
- SchemaElementDecl.cs
- EntityDataSourceQueryBuilder.cs
- QilReference.cs
- SharedUtils.cs
- HierarchicalDataTemplate.cs
- CallTemplateAction.cs
- FormatConvertedBitmap.cs
- _BaseOverlappedAsyncResult.cs
- TemplateBuilder.cs
- XmlSyndicationContent.cs
- InputScopeConverter.cs
- XPathParser.cs
- SecuritySessionSecurityTokenProvider.cs
- RecognizedAudio.cs
- DrawToolTipEventArgs.cs
- DispatchChannelSink.cs
- Walker.cs
- DigitShape.cs
- StateDesignerConnector.cs
- GeneralTransformGroup.cs
- InvokeMethodActivity.cs
- MediaScriptCommandRoutedEventArgs.cs
- LoadRetryStrategyFactory.cs
- FontSourceCollection.cs
- BCLDebug.cs
- OdbcStatementHandle.cs
- XPathAncestorQuery.cs
- MetaForeignKeyColumn.cs
- TransformerInfo.cs
- NativeMethods.cs
- SqlCacheDependency.cs
- ConstraintEnumerator.cs
- SizeConverter.cs
- XsltException.cs
- XmlILOptimizerVisitor.cs
- LightweightCodeGenerator.cs
- X509CertificateValidator.cs
- DocumentSequenceHighlightLayer.cs
- AppliedDeviceFiltersEditor.cs
- IdnMapping.cs
- IOThreadScheduler.cs
- ReadOnlyTernaryTree.cs