Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / GroupBoxDesigner.cs / 1 / GroupBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ //#define TESTVALUEUI [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.GroupBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using System.Diagnostics; using System; using System.Design; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Design; using System.ComponentModel.Design; using System.Collections; ////// /// This class handles all design time behavior for the group box class. Group /// boxes may contain sub-components and therefore use the frame designer. /// internal class GroupBoxDesigner : ParentControlDesigner { private InheritanceUI inheritanceUI; ////// /// Determines the default location for a control added to this designer. /// it is usualy (0,0), but may be modified if the container has special borders, etc. /// protected override Point DefaultControlLocation { get { GroupBox gb = (GroupBox)this.Control; return new Point(gb.DisplayRectangle.X,gb.DisplayRectangle.Y); } } #if TESTVALUEUI ////// /// Initializes the designer with the given component. The designer can /// get the component's site and request services from it in this call. /// public override void Initialize(IComponent component) { base.Initialize(component); AutoResizeHandles = true; IPropertyValueUIService pvUISvc = (IPropertyValueUIService)component.Site.GetService(typeof(IPropertyValueUIService)); if (pvUISvc != null) { pvUISvc.AddPropertyValueUIHandler(new PropertyValueUIHandler(this.OnGetUIValueItem)); } } private void OnGetUIValueItem(object component, PropertyDescriptor propDesc, ArrayList valueUIItemList){ if (propDesc.PropertyType == typeof(string)) { Bitmap bmp = new Bitmap(typeof(GroupBoxDesigner), "BoundProperty.bmp"); bmp.MakeTransparent(); valueUIItemList.Add(new LocalUIItem(bmp, new PropertyValueUIItemInvokeHandler(this.OnPropertyValueUIItemInvoke), "Data Can")); //bmp = new Bitmap("BoundProperty.bmp"); valueUIItemList.Add(new LocalUIItem(bmp, new PropertyValueUIItemInvokeHandler(this.OnPropertyValueUIItemInvoke), "Little Button")); } } private void OnPropertyValueUIItemInvoke(ITypeDescriptorContext context, PropertyDescriptor descriptor, PropertyValueUIItem invokedItem) { Debug.Fail("propertyuivalue '" + invokedItem.ToolTip + "' invoked"); } #endif ////// /// We override this because even though we still want to /// offset our grid for our display rectangle, we still want /// to align to our parent's grid - so we don't look funny /// protected override void OnPaintAdornments(PaintEventArgs pe) { if (DrawGrid) { Control control = (Control)Control; Rectangle rectangle = Control.DisplayRectangle; rectangle.Width++; // gpr: FillRectangle with a TextureBrush comes up one pixel short rectangle.Height++; ControlPaint.DrawGrid(pe.Graphics, rectangle, GridSize, control.BackColor); } // If this control is being inherited, paint it // if (Inherited) { if (inheritanceUI == null) { inheritanceUI = (InheritanceUI)GetService(typeof(InheritanceUI)); } if (inheritanceUI != null) { pe.Graphics.DrawImage(inheritanceUI.InheritanceGlyph, 0, 0); } } } ////// /// We override our base class's WndProc here because /// the group box always returns HTTRANSPARENT. This /// causes the mouse to go "through" the group box, but /// that's not what we want at design time. /// protected override void WndProc(ref Message m) { switch (m.Msg) { case NativeMethods.WM_NCHITTEST: // The group box always fires HTTRANSPARENT, which // causes the message to go to our parent. We want // the group box's designer to get these messages, however, // so change this. // base.WndProc(ref m); if ((int)m.Result == NativeMethods.HTTRANSPARENT) { m.Result = (IntPtr)NativeMethods.HTCLIENT; } break; default: base.WndProc(ref m); break; } } #if TESTVALUEUI internal class LocalUIItem : PropertyValueUIItem { private string itemName; public LocalUIItem(Image img, PropertyValueUIItemInvokeHandler handler, string itemName) : base(img, handler, itemName) { this.itemName = itemName; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
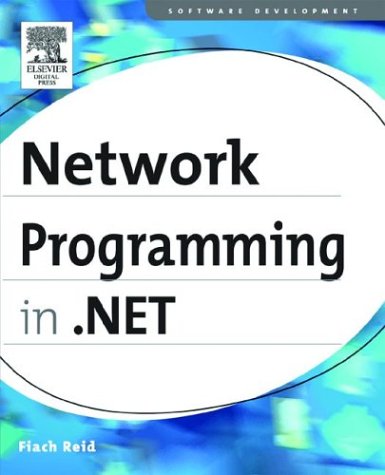
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Int32.cs
- StrokeFIndices.cs
- XmlElementCollection.cs
- RegexCapture.cs
- MarkupProperty.cs
- TextProperties.cs
- PathData.cs
- EditingCoordinator.cs
- XPathPatternBuilder.cs
- ConfigurationSectionGroup.cs
- ImageIndexConverter.cs
- StringInfo.cs
- x509store.cs
- ZipIOExtraFieldElement.cs
- BaseUriHelper.cs
- BaseResourcesBuildProvider.cs
- WizardPanel.cs
- HttpCookie.cs
- FormatConvertedBitmap.cs
- CancellationTokenSource.cs
- GradientPanel.cs
- SQLMembershipProvider.cs
- _NestedSingleAsyncResult.cs
- PrefixHandle.cs
- ClosableStream.cs
- UniqueIdentifierService.cs
- ServiceChannelFactory.cs
- EncodingNLS.cs
- Crypto.cs
- FontFamilyIdentifier.cs
- TypeSource.cs
- Random.cs
- Win32PrintDialog.cs
- XmlAutoDetectWriter.cs
- ReflectionTypeLoadException.cs
- HotCommands.cs
- CharEntityEncoderFallback.cs
- Baml2006ReaderContext.cs
- WriteTimeStream.cs
- SelectionItemPattern.cs
- HttpProcessUtility.cs
- XPathMultyIterator.cs
- TagMapInfo.cs
- MenuTracker.cs
- ExecutionContext.cs
- DocumentSequence.cs
- RecognizerStateChangedEventArgs.cs
- ParameterBuilder.cs
- Utilities.cs
- HttpFileCollection.cs
- HTMLTagNameToTypeMapper.cs
- X509Logo.cs
- ConsoleKeyInfo.cs
- Soap.cs
- CodeAccessPermission.cs
- XmlTypeAttribute.cs
- EasingQuaternionKeyFrame.cs
- OdbcConnectionFactory.cs
- RoutedEventHandlerInfo.cs
- InvokeGenerator.cs
- HtmlTableRowCollection.cs
- TableSectionStyle.cs
- COM2ComponentEditor.cs
- InvokeHandlers.cs
- ComponentEditorPage.cs
- AttachedPropertiesService.cs
- WorkBatch.cs
- InputScopeAttribute.cs
- XmlObjectSerializerReadContextComplexJson.cs
- XmlHierarchyData.cs
- DescendantBaseQuery.cs
- TerminatorSinks.cs
- Facet.cs
- AssociationEndMember.cs
- XmlNullResolver.cs
- HMACSHA512.cs
- IconBitmapDecoder.cs
- HandlerFactoryWrapper.cs
- SoapAttributes.cs
- ListItemCollection.cs
- BinaryReader.cs
- InputLanguageSource.cs
- MtomMessageEncodingElement.cs
- SaveFileDialog.cs
- ScaleTransform.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- TextTreeRootTextBlock.cs
- ErrorStyle.cs
- KnownTypeAttribute.cs
- oledbmetadatacollectionnames.cs
- SizeChangedEventArgs.cs
- BlurEffect.cs
- WebRequestModuleElementCollection.cs
- ObjectItemCollection.cs
- AssemblyLoader.cs
- TypeForwardedToAttribute.cs
- IntPtr.cs
- RepeaterItemCollection.cs
- SQLInt64.cs
- UnlockInstanceCommand.cs