Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Utils / GrowingArray.cs / 1305376 / GrowingArray.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // GrowingArray.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A growing array. Unlike List{T}, it makes the internal array available to its user. /// ///internal class GrowingArray { T[] m_array; int m_count; const int DEFAULT_ARRAY_SIZE = 1024; internal GrowingArray() { m_array = new T[DEFAULT_ARRAY_SIZE]; m_count = 0; } //---------------------------------------------------------------------------------------- // Returns the internal array representing the list. Note that the array may be larger // than necessary to hold all elements in the list. // internal T[] InternalArray { get { return m_array; } } internal int Count { get { return m_count; } } internal void Add(T element) { if (m_count >= m_array.Length) { GrowArray(2 * m_array.Length); } m_array[m_count++] = element; } private void GrowArray(int newSize) { Contract.Assert(newSize > m_array.Length); T[] array2 = new T[newSize]; m_array.CopyTo(array2, 0); m_array = array2; } internal void CopyFrom(T[] otherArray, int otherCount) { // Ensure there is just enough room for both. if (m_count + otherCount > m_array.Length) { GrowArray(m_count + otherCount); } // And now just blit the keys directly. Array.Copy(otherArray, 0, m_array, m_count, otherCount); m_count += otherCount; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // GrowingArray.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A growing array. Unlike List{T}, it makes the internal array available to its user. /// ///internal class GrowingArray { T[] m_array; int m_count; const int DEFAULT_ARRAY_SIZE = 1024; internal GrowingArray() { m_array = new T[DEFAULT_ARRAY_SIZE]; m_count = 0; } //---------------------------------------------------------------------------------------- // Returns the internal array representing the list. Note that the array may be larger // than necessary to hold all elements in the list. // internal T[] InternalArray { get { return m_array; } } internal int Count { get { return m_count; } } internal void Add(T element) { if (m_count >= m_array.Length) { GrowArray(2 * m_array.Length); } m_array[m_count++] = element; } private void GrowArray(int newSize) { Contract.Assert(newSize > m_array.Length); T[] array2 = new T[newSize]; m_array.CopyTo(array2, 0); m_array = array2; } internal void CopyFrom(T[] otherArray, int otherCount) { // Ensure there is just enough room for both. if (m_count + otherCount > m_array.Length) { GrowArray(m_count + otherCount); } // And now just blit the keys directly. Array.Copy(otherArray, 0, m_array, m_count, otherCount); m_count += otherCount; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
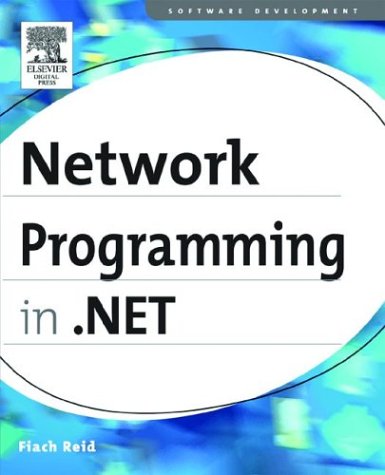
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransactionManager.cs
- WsatExtendedInformation.cs
- CollectionChange.cs
- PermissionRequestEvidence.cs
- AspNetHostingPermission.cs
- AutomationPatternInfo.cs
- TypeHelper.cs
- FrameworkContentElement.cs
- RoutedEventHandlerInfo.cs
- DefaultPrintController.cs
- TextView.cs
- Color.cs
- MapPathBasedVirtualPathProvider.cs
- EdmScalarPropertyAttribute.cs
- AnonymousIdentificationSection.cs
- PerformanceCounterPermissionAttribute.cs
- oledbconnectionstring.cs
- FormatSelectingMessageInspector.cs
- StateMachineDesignerPaint.cs
- PrivilegeNotHeldException.cs
- TemplateEditingService.cs
- ProviderCommandInfoUtils.cs
- Control.cs
- PersonalizationProviderCollection.cs
- DesignerLoader.cs
- ReadOnlyCollectionBuilder.cs
- ClientUtils.cs
- Operators.cs
- IPPacketInformation.cs
- ClientUriBehavior.cs
- DefinitionProperties.cs
- PartialArray.cs
- _HTTPDateParse.cs
- DiscardableAttribute.cs
- SchemaTableColumn.cs
- Tuple.cs
- LocalBuilder.cs
- DocumentSchemaValidator.cs
- TimelineClockCollection.cs
- SqlClientPermission.cs
- SqlDataSourceTableQuery.cs
- IdentityValidationException.cs
- XmlEnumAttribute.cs
- WebBrowserSiteBase.cs
- UpdateExpressionVisitor.cs
- AssemblyFilter.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- HandlerMappingMemo.cs
- ListBox.cs
- Animatable.cs
- CodeArgumentReferenceExpression.cs
- ManagedIStream.cs
- WebBrowserSiteBase.cs
- CodeTypeReference.cs
- CompilerGeneratedAttribute.cs
- CacheEntry.cs
- Timer.cs
- ElementMarkupObject.cs
- SqlDependencyListener.cs
- HttpCacheParams.cs
- SQLResource.cs
- NamespaceCollection.cs
- ThemeConfigurationDialog.cs
- ListViewInsertedEventArgs.cs
- FormParameter.cs
- DataStreamFromComStream.cs
- ChangeConflicts.cs
- InstanceCreationEditor.cs
- String.cs
- StylusDownEventArgs.cs
- DataGridRow.cs
- ReadContentAsBinaryHelper.cs
- Ref.cs
- ExtensionFile.cs
- EntitySet.cs
- DbMetaDataFactory.cs
- KeyGestureConverter.cs
- QueryStringParameter.cs
- GridViewCancelEditEventArgs.cs
- HttpEncoder.cs
- RowUpdatingEventArgs.cs
- InvokeGenerator.cs
- TypePropertyEditor.cs
- DirectoryObjectSecurity.cs
- PropertyChangingEventArgs.cs
- WebPartDescriptionCollection.cs
- ConstraintStruct.cs
- ResXResourceSet.cs
- WorkflowControlClient.cs
- relpropertyhelper.cs
- ToolZoneDesigner.cs
- RuleSettingsCollection.cs
- SessionKeyExpiredException.cs
- PointCollectionValueSerializer.cs
- AstTree.cs
- ListViewItemSelectionChangedEvent.cs
- ConstraintCollection.cs
- RegexCode.cs
- XmlLangPropertyAttribute.cs
- ButtonRenderer.cs