Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / OleDb / SafeHandles.cs / 1 / SafeHandles.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Threading; using System.Runtime.ConstrainedExecution; internal sealed class DualCoTaskMem : SafeHandle { private IntPtr handle2; // this must be protected so derived classes can use out params. private DualCoTaskMem() : base(IntPtr.Zero, true) { this.handle2 = IntPtr.Zero; } // IDBInfo.GetLiteralInfo internal DualCoTaskMem(UnsafeNativeMethods.IDBInfo dbInfo, int[] literals, out int literalCount, out IntPtr literalInfo, out OleDbHResult hr) : this() { int count = (null != literals) ? literals.Length : 0; Bid.Trace("\n"); hr = dbInfo.GetLiteralInfo(count, literals, out literalCount, out base.handle, out this.handle2); literalInfo = base.handle; Bid.Trace(" %08X{HRESULT}\n", hr); } // IColumnsInfo.GetColumnInfo internal DualCoTaskMem(UnsafeNativeMethods.IColumnsInfo columnsInfo, out IntPtr columnCount, out IntPtr columnInfos, out OleDbHResult hr) : this() { Bid.Trace(" \n"); hr = columnsInfo.GetColumnInfo(out columnCount, out base.handle, out this.handle2); columnInfos = base.handle; Bid.Trace(" %08X{HRESULT}\n", hr); } // IDBSchemaRowset.GetSchemas internal DualCoTaskMem(UnsafeNativeMethods.IDBSchemaRowset dbSchemaRowset, out int schemaCount, out IntPtr schemaGuids, out IntPtr schemaRestrictions, out OleDbHResult hr) : this() { Bid.Trace(" \n"); hr = dbSchemaRowset.GetSchemas(out schemaCount, out base.handle, out this.handle2); schemaGuids = base.handle; schemaRestrictions = this.handle2; Bid.Trace(" %08X{HRESULT}\n", hr); } internal DualCoTaskMem(UnsafeNativeMethods.IColumnsRowset icolumnsRowset, out IntPtr cOptColumns, out OleDbHResult hr) : base(IntPtr.Zero, true) { Bid.Trace(" \n"); hr = icolumnsRowset.GetAvailableColumns(out cOptColumns, out base.handle); Bid.Trace(" %08X{HRESULT}\n", hr); } public override bool IsInvalid { get { return (((IntPtr.Zero == base.handle)) && (IntPtr.Zero == this.handle2)); } } protected override bool ReleaseHandle() { // NOTE: The SafeHandle class guarantees this will be called exactly once. IntPtr ptr = base.handle; base.handle = IntPtr.Zero; if (IntPtr.Zero != ptr) { SafeNativeMethods.CoTaskMemFree(ptr); } ptr = this.handle2; this.handle2 = IntPtr.Zero; if (IntPtr.Zero != ptr) { SafeNativeMethods.CoTaskMemFree(ptr); } return true; } } internal sealed class RowHandleBuffer : DbBuffer { internal RowHandleBuffer(IntPtr rowHandleFetchCount) : base((int)rowHandleFetchCount*ADP.PtrSize) { } internal IntPtr GetRowHandle(int index) { IntPtr value = ReadIntPtr( index * ADP.PtrSize); Debug.Assert(ODB.DB_NULL_HROW != value, "bad rowHandle"); return value; } } internal sealed class StringMemHandle : DbBuffer { internal StringMemHandle(string value) : base((null != value) ? checked(2+2*value.Length) : 0) { if (null != value) { // null-termination exists because of the extra 2+ which is zero'd during on allocation WriteCharArray(0, value.ToCharArray(), 0, value.Length); } } } internal sealed class ChapterHandle : WrappedIUnknown { internal static readonly ChapterHandle DB_NULL_HCHAPTER = new ChapterHandle(IntPtr.Zero); private IntPtr _chapterHandle; internal static ChapterHandle CreateChapterHandle(object chapteredRowset, RowBinding binding, int valueOffset) { if ((null == chapteredRowset) || (IntPtr.Zero == binding.ReadIntPtr(valueOffset))) { return ChapterHandle.DB_NULL_HCHAPTER; } return new ChapterHandle(chapteredRowset, binding, valueOffset); } // from ADODBRecordSetConstruction we do not want to release the initial chapter handle internal static ChapterHandle CreateChapterHandle(IntPtr chapter) { if (IntPtr.Zero == chapter) { return ChapterHandle.DB_NULL_HCHAPTER; } return new ChapterHandle(chapter); } // from ADODBRecordSetConstruction we do not want to release the initial chapter handle private ChapterHandle(IntPtr chapter) : base((object)null) { _chapterHandle = chapter; } private ChapterHandle(object chapteredRowset, RowBinding binding, int valueOffset) : base(chapteredRowset) { RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { _chapterHandle = binding.InterlockedExchangePointer(valueOffset); } } internal IntPtr HChapter { get { return _chapterHandle; } } protected override bool ReleaseHandle() { // NOTE: The SafeHandle class guarantees this will be called exactly once and is non-interrutible. IntPtr chapter = _chapterHandle; _chapterHandle = IntPtr.Zero; if ((IntPtr.Zero != base.handle) && (IntPtr.Zero != chapter)) { Bid.Trace(" Chapter=%Id\n", chapter); OleDbHResult hr = (OleDbHResult)NativeOledbWrapper.IChapteredRowsetReleaseChapter(base.handle, chapter); Bid.Trace(" %08X{HRESULT}\n", hr); } return base.ReleaseHandle(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
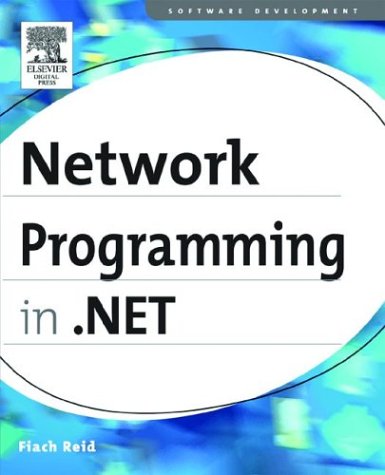
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TabControlCancelEvent.cs
- TypeUnloadedException.cs
- ListViewContainer.cs
- StrokeFIndices.cs
- SrgsGrammarCompiler.cs
- XmlSchemaParticle.cs
- CheckBoxAutomationPeer.cs
- LineUtil.cs
- DbConnectionPoolGroup.cs
- RoleManagerEventArgs.cs
- FixedStringLookup.cs
- SerializationSectionGroup.cs
- TranslateTransform.cs
- PersonalizationStateInfo.cs
- FontFamily.cs
- ListViewItem.cs
- WebUtil.cs
- UTF32Encoding.cs
- ModifiableIteratorCollection.cs
- WebReferencesBuildProvider.cs
- MetadataPropertyvalue.cs
- KeyNotFoundException.cs
- TreeViewItemAutomationPeer.cs
- SqlException.cs
- MessageBox.cs
- CounterSample.cs
- DerivedKeySecurityTokenStub.cs
- InheritablePropertyChangeInfo.cs
- ZipIOModeEnforcingStream.cs
- XhtmlStyleClass.cs
- SafeViewOfFileHandle.cs
- GridSplitter.cs
- DrawListViewColumnHeaderEventArgs.cs
- MimeFormReflector.cs
- Soap11ServerProtocol.cs
- ProcessModule.cs
- RunClient.cs
- ExpressionEditorAttribute.cs
- HandleCollector.cs
- ManualWorkflowSchedulerService.cs
- RenderData.cs
- AuthenticationModulesSection.cs
- WindowsFormsLinkLabel.cs
- EUCJPEncoding.cs
- SelectionItemPattern.cs
- SplitterPanelDesigner.cs
- ReadOnlyDataSourceView.cs
- WebPartConnectionsCancelEventArgs.cs
- Compiler.cs
- CodeBlockBuilder.cs
- WebInvokeAttribute.cs
- HostVisual.cs
- CompilerResults.cs
- GlyphInfoList.cs
- IChannel.cs
- StrokeNode.cs
- StorageTypeMapping.cs
- SubstitutionList.cs
- ShutDownListener.cs
- WebPartZoneCollection.cs
- Int64Converter.cs
- HMACRIPEMD160.cs
- DataGridViewCheckBoxColumn.cs
- XmlSchemaImporter.cs
- WindowPattern.cs
- ValueChangedEventManager.cs
- InvalidAsynchronousStateException.cs
- ScopelessEnumAttribute.cs
- ObjectDataSourceEventArgs.cs
- AmbientLight.cs
- TypedDatasetGenerator.cs
- XmlSchemaObjectTable.cs
- PathFigure.cs
- SignalGate.cs
- MutableAssemblyCacheEntry.cs
- MetafileHeader.cs
- WebMessageFormatHelper.cs
- UriScheme.cs
- EnumMember.cs
- ChangeConflicts.cs
- DialogResultConverter.cs
- Funcletizer.cs
- WinEventTracker.cs
- ComponentDispatcher.cs
- DataTableCollection.cs
- NamespaceInfo.cs
- QuaternionRotation3D.cs
- sqlnorm.cs
- BlurBitmapEffect.cs
- SchemaImporter.cs
- HtmlInputRadioButton.cs
- InvalidAsynchronousStateException.cs
- SiblingIterators.cs
- XmlAutoDetectWriter.cs
- HandleExceptionArgs.cs
- SimpleBitVector32.cs
- XPathNode.cs
- ConfigurationProperty.cs
- XmlCountingReader.cs
- CodeParameterDeclarationExpressionCollection.cs