Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TableRow.cs / 1305600 / TableRow.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table row implementation // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Markup; using System.Collections.Generic; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Table row /// [ContentProperty("Cells")] public class TableRow : TextElement, IAddChild, IIndexedChild, IAcceptInsertion { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates an instance of a Row /// public TableRow() : base() { PrivateInitialize(); } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// void IAddChild.AddChild(object value) { if (value == null) { throw new ArgumentNullException("value"); } TableCell cell = value as TableCell; if (cell != null) { Cells.Add(cell); return; } throw (new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableCell)), "value")); } ////// /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } ////// /// Called when tablerow gets new parent /// /// /// New parent of cell /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; if (newParent != null && !(newParent is TableRowGroup)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if (oldParent != null) { ((TableRowGroup)oldParent).Rows.InternalRemove(this); } base.OnNewParent(newParent); if (newParent != null) { ((TableRowGroup)newParent).Rows.InternalAdd(this); } } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #region IIndexedChild implementation ////// Callback used to notify about entering model tree. /// void IIndexedChild.OnEnterParentTree() { this.OnEnterParentTree(); } /// /// Callback used to notify about exitting model tree. /// void IIndexedChild.OnExitParentTree() { this.OnExitParentTree(); } void IIndexedChild .OnAfterExitParentTree(TableRowGroup parent) { this.OnAfterExitParentTree(parent); } int IIndexedChild .Index { get { return this.Index; } set { this.Index = value; } } #endregion IIndexedChild implementation /// /// Callback used to notify the Row about entering model tree. /// internal void OnEnterParentTree() { if (Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the Row about exitting model tree. /// internal void OnAfterExitParentTree(TableRowGroup rowGroup) { if (rowGroup.Table != null) { Table.OnStructureChanged(); } } ////// ValidateStructure /// internal void ValidateStructure(RowSpanVector rowSpanVector) { Debug.Assert(rowSpanVector != null); SetFlags(!rowSpanVector.Empty(), Flags.HasForeignCells); SetFlags(false, Flags.HasRealCells); _formatCellCount = 0; _columnCount = 0; int firstAvailableIndex; int firstOccupiedIndex; rowSpanVector.GetFirstAvailableRange(out firstAvailableIndex, out firstOccupiedIndex); for (int i = 0; i < _cells.Count; ++i) { TableCell cell = _cells[i]; // Get cloumn span and row span. Row span is limited to the number of rows in the row group. // Since we do not know the number of columns in the table at this point, column span is limited only // by internal constants int columnSpan = cell.ColumnSpan; int rowSpan = cell.RowSpan; while (firstAvailableIndex + columnSpan > firstOccupiedIndex) { rowSpanVector.GetNextAvailableRange(out firstAvailableIndex, out firstOccupiedIndex); } Debug.Assert(i <= firstAvailableIndex); cell.ValidateStructure(firstAvailableIndex); if (rowSpan > 1) { rowSpanVector.Register(cell); } else { _formatCellCount++; } firstAvailableIndex += columnSpan; } _columnCount = firstAvailableIndex; bool isLastRowOfAnySpan = false; rowSpanVector.GetSpanCells(out _spannedCells, out isLastRowOfAnySpan); Debug.Assert(_spannedCells != null); if ((_formatCellCount > 0) || isLastRowOfAnySpan == true) { SetFlags(true, Flags.HasRealCells); } _formatCellCount += _spannedCells.Length; Debug.Assert(_cells.Count <= _formatCellCount); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// RowGroup owner accessor /// internal TableRowGroup RowGroup { get { return (Parent as TableRowGroup); } } ////// Table owner accessor /// internal Table Table { get { return (RowGroup != null ? RowGroup.Table : null); } } ////// Returns the row's cell collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public TableCellCollection Cells { get { return (_cells); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeCells() { return (Cells.Count > 0); } ////// Row's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } int IAcceptInsertion.InsertionIndex { get { return this.InsertionIndex; } set { this.InsertionIndex = value; } } ////// Stores temporary data for where to insert a new cell /// internal int InsertionIndex { get { return _cellInsertionIndex; } set { _cellInsertionIndex = value; } } ////// Returns span cells vector /// internal TableCell[] SpannedCells { get { return (_spannedCells); } } ////// Count of columns in the table /// internal int ColumnCount { get { return (_columnCount); } } ////// Returns "true" if there are row spanned cells belonging to previous rows /// internal bool HasForeignCells { get { return (CheckFlags(Flags.HasForeignCells)); } } ////// Returns "true" if there are row spanned cells belonging to previous rows /// internal bool HasRealCells { get { return (CheckFlags(Flags.HasRealCells)); } } ////// Count of columns in the table /// internal int FormatCellCount { get { return (_formatCellCount); } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Private ctor time initialization. /// private void PrivateInitialize() { _cells = new TableCellCollection(this); _parentIndex = -1; _cellInsertionIndex = -1; } ////// SetFlags is used to set or unset one or multiple flags on the row. /// private void SetFlags(bool value, Flags flags) { _flags = value ? (_flags | flags) : (_flags & (~flags)); } ////// CheckFlags returns true if all flags in the bitmask flags are set. /// private bool CheckFlags(Flags flags) { return ((_flags & flags) == flags); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private TableCellCollection _cells; // collection of cells belonging to the row private TableCell[] _spannedCells; // row spanned cell storage private int _parentIndex; // row's index in parent's children collection private int _cellInsertionIndex; // Insertion index a cell private int _columnCount; private Flags _flags; // flags reflecting various aspects of row's state private int _formatCellCount; // count of the cell to be formatted in this row #endregion Private Fields //------------------------------------------------------ // // Private Structures / Classes // //----------------------------------------------------- #region Private Structures Classes [System.Flags] private enum Flags { // // state flags // HasForeignCells = 0x00000010, // there are hanging cells from the previous rows HasRealCells = 0x00000020, // real cells in row (not just spanning) (Only known by validation, not format) } #endregion Private Structures Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table row implementation // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Security; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Markup; using System.Collections.Generic; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Table row /// [ContentProperty("Cells")] public class TableRow : TextElement, IAddChild, IIndexedChild, IAcceptInsertion { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates an instance of a Row /// public TableRow() : base() { PrivateInitialize(); } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// void IAddChild.AddChild(object value) { if (value == null) { throw new ArgumentNullException("value"); } TableCell cell = value as TableCell; if (cell != null) { Cells.Add(cell); return; } throw (new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableCell)), "value")); } ////// /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } ////// /// Called when tablerow gets new parent /// /// /// New parent of cell /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; if (newParent != null && !(newParent is TableRowGroup)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if (oldParent != null) { ((TableRowGroup)oldParent).Rows.InternalRemove(this); } base.OnNewParent(newParent); if (newParent != null) { ((TableRowGroup)newParent).Rows.InternalAdd(this); } } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #region IIndexedChild implementation ////// Callback used to notify about entering model tree. /// void IIndexedChild.OnEnterParentTree() { this.OnEnterParentTree(); } /// /// Callback used to notify about exitting model tree. /// void IIndexedChild.OnExitParentTree() { this.OnExitParentTree(); } void IIndexedChild .OnAfterExitParentTree(TableRowGroup parent) { this.OnAfterExitParentTree(parent); } int IIndexedChild .Index { get { return this.Index; } set { this.Index = value; } } #endregion IIndexedChild implementation /// /// Callback used to notify the Row about entering model tree. /// internal void OnEnterParentTree() { if (Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the Row about exitting model tree. /// internal void OnAfterExitParentTree(TableRowGroup rowGroup) { if (rowGroup.Table != null) { Table.OnStructureChanged(); } } ////// ValidateStructure /// internal void ValidateStructure(RowSpanVector rowSpanVector) { Debug.Assert(rowSpanVector != null); SetFlags(!rowSpanVector.Empty(), Flags.HasForeignCells); SetFlags(false, Flags.HasRealCells); _formatCellCount = 0; _columnCount = 0; int firstAvailableIndex; int firstOccupiedIndex; rowSpanVector.GetFirstAvailableRange(out firstAvailableIndex, out firstOccupiedIndex); for (int i = 0; i < _cells.Count; ++i) { TableCell cell = _cells[i]; // Get cloumn span and row span. Row span is limited to the number of rows in the row group. // Since we do not know the number of columns in the table at this point, column span is limited only // by internal constants int columnSpan = cell.ColumnSpan; int rowSpan = cell.RowSpan; while (firstAvailableIndex + columnSpan > firstOccupiedIndex) { rowSpanVector.GetNextAvailableRange(out firstAvailableIndex, out firstOccupiedIndex); } Debug.Assert(i <= firstAvailableIndex); cell.ValidateStructure(firstAvailableIndex); if (rowSpan > 1) { rowSpanVector.Register(cell); } else { _formatCellCount++; } firstAvailableIndex += columnSpan; } _columnCount = firstAvailableIndex; bool isLastRowOfAnySpan = false; rowSpanVector.GetSpanCells(out _spannedCells, out isLastRowOfAnySpan); Debug.Assert(_spannedCells != null); if ((_formatCellCount > 0) || isLastRowOfAnySpan == true) { SetFlags(true, Flags.HasRealCells); } _formatCellCount += _spannedCells.Length; Debug.Assert(_cells.Count <= _formatCellCount); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// RowGroup owner accessor /// internal TableRowGroup RowGroup { get { return (Parent as TableRowGroup); } } ////// Table owner accessor /// internal Table Table { get { return (RowGroup != null ? RowGroup.Table : null); } } ////// Returns the row's cell collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public TableCellCollection Cells { get { return (_cells); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeCells() { return (Cells.Count > 0); } ////// Row's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } int IAcceptInsertion.InsertionIndex { get { return this.InsertionIndex; } set { this.InsertionIndex = value; } } ////// Stores temporary data for where to insert a new cell /// internal int InsertionIndex { get { return _cellInsertionIndex; } set { _cellInsertionIndex = value; } } ////// Returns span cells vector /// internal TableCell[] SpannedCells { get { return (_spannedCells); } } ////// Count of columns in the table /// internal int ColumnCount { get { return (_columnCount); } } ////// Returns "true" if there are row spanned cells belonging to previous rows /// internal bool HasForeignCells { get { return (CheckFlags(Flags.HasForeignCells)); } } ////// Returns "true" if there are row spanned cells belonging to previous rows /// internal bool HasRealCells { get { return (CheckFlags(Flags.HasRealCells)); } } ////// Count of columns in the table /// internal int FormatCellCount { get { return (_formatCellCount); } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Private ctor time initialization. /// private void PrivateInitialize() { _cells = new TableCellCollection(this); _parentIndex = -1; _cellInsertionIndex = -1; } ////// SetFlags is used to set or unset one or multiple flags on the row. /// private void SetFlags(bool value, Flags flags) { _flags = value ? (_flags | flags) : (_flags & (~flags)); } ////// CheckFlags returns true if all flags in the bitmask flags are set. /// private bool CheckFlags(Flags flags) { return ((_flags & flags) == flags); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private TableCellCollection _cells; // collection of cells belonging to the row private TableCell[] _spannedCells; // row spanned cell storage private int _parentIndex; // row's index in parent's children collection private int _cellInsertionIndex; // Insertion index a cell private int _columnCount; private Flags _flags; // flags reflecting various aspects of row's state private int _formatCellCount; // count of the cell to be formatted in this row #endregion Private Fields //------------------------------------------------------ // // Private Structures / Classes // //----------------------------------------------------- #region Private Structures Classes [System.Flags] private enum Flags { // // state flags // HasForeignCells = 0x00000010, // there are hanging cells from the previous rows HasRealCells = 0x00000020, // real cells in row (not just spanning) (Only known by validation, not format) } #endregion Private Structures Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
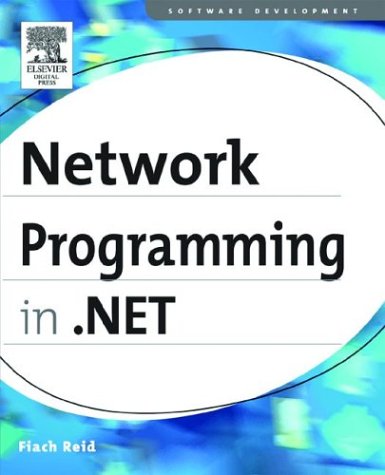
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathNodeIterator.cs
- DataSourceSelectArguments.cs
- HebrewCalendar.cs
- FrugalList.cs
- CryptoConfig.cs
- NetworkCredential.cs
- QilBinary.cs
- TargetFrameworkUtil.cs
- CallbackException.cs
- PreviewControlDesigner.cs
- OleDbRowUpdatedEvent.cs
- XmlQualifiedNameTest.cs
- connectionpool.cs
- SpellerError.cs
- PropertyGridEditorPart.cs
- BulletedListEventArgs.cs
- ToolStripItemCollection.cs
- OleDbInfoMessageEvent.cs
- PersistNameAttribute.cs
- MimeWriter.cs
- NavigationWindowAutomationPeer.cs
- SpecialTypeDataContract.cs
- StickyNote.cs
- InputLanguageCollection.cs
- Column.cs
- PlacementWorkspace.cs
- ServiceConfigurationTraceRecord.cs
- RectangleGeometry.cs
- BasicExpressionVisitor.cs
- GeometryModel3D.cs
- BlockUIContainer.cs
- UnmanagedMarshal.cs
- PageContent.cs
- LoginCancelEventArgs.cs
- WebConfigManager.cs
- CallTemplateAction.cs
- XmlSchema.cs
- ScrollableControl.cs
- SqlDataReaderSmi.cs
- SqlServer2KCompatibilityAnnotation.cs
- RequestCachePolicyConverter.cs
- ServiceDebugElement.cs
- CodeVariableReferenceExpression.cs
- EntityContainerEmitter.cs
- TrustSection.cs
- ConfigurationStrings.cs
- FilterQueryOptionExpression.cs
- EntityContainerRelationshipSetEnd.cs
- OpenTypeCommon.cs
- ConsumerConnectionPoint.cs
- Drawing.cs
- TimelineGroup.cs
- ErrorFormatterPage.cs
- ThemeDirectoryCompiler.cs
- TransactionInformation.cs
- MetadataArtifactLoaderResource.cs
- DateTimeParse.cs
- RightsManagementSuppressedStream.cs
- NavigationWindowAutomationPeer.cs
- CompiledRegexRunnerFactory.cs
- RC2CryptoServiceProvider.cs
- ToolStripItemClickedEventArgs.cs
- WindowsComboBox.cs
- path.cs
- ConfigXmlElement.cs
- WebPartMovingEventArgs.cs
- DeviceContexts.cs
- ColorConverter.cs
- HashSetDebugView.cs
- GridViewColumnHeaderAutomationPeer.cs
- WindowAutomationPeer.cs
- SetMemberBinder.cs
- IOException.cs
- TextDecoration.cs
- WebControlsSection.cs
- PartialArray.cs
- EventPropertyMap.cs
- XmlEntityReference.cs
- XmlDocumentType.cs
- SoapCodeExporter.cs
- EventLogRecord.cs
- CatalogZone.cs
- M3DUtil.cs
- BitmapFrameDecode.cs
- EdmSchemaError.cs
- Label.cs
- ToolStripProgressBar.cs
- HtmlLink.cs
- CodeSnippetExpression.cs
- DesignColumn.cs
- _HelperAsyncResults.cs
- EntityProviderFactory.cs
- ReadOnlyPropertyMetadata.cs
- DoubleCollectionConverter.cs
- ExtenderProvidedPropertyAttribute.cs
- nulltextnavigator.cs
- ActivationArguments.cs
- RectValueSerializer.cs
- TabItemAutomationPeer.cs
- UnsupportedPolicyOptionsException.cs