Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachObjectContext.cs / 1 / ReachObjectContext.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachObjectContext.cs Abstract: This file contains the implementation representing the context of a Serializable object. This woudl include information about the object itself and all the properties contained within that object Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { internal class SerializableObjectContext : BasicContext { #region Constructor static SerializableObjectContext( ) { _recycableSerializableObjectContexts = new Stack(); } ////// Instantiates an ObjectContext /// /// /// The name of type of the object. /// /// /// The perfix (namespace) of the type of the object. /// /// /// The instance of the object which contained this object as /// one of its properties. /// /// /// The property from which this object was driven to serialization. /// public SerializableObjectContext( string name, string prefix, object target, SerializablePropertyContext serializablePropertyContext ) : base(name, prefix) { // // Validate Input Arguments // if (target == null) { throw new ArgumentNullException("target"); } _targetObject = target; _isComplexValue = false; _backingPropertyContext = serializablePropertyContext; } ////// Instantiates an ObjectContext /// /// /// The instance of the object which contained this object as /// one of its properties. /// /// /// The property from which this object was driven to serialization. /// public SerializableObjectContext( object target, SerializablePropertyContext serializablePropertyContext ) { Initialize(target, serializablePropertyContext); } #endregion Constructor #region Internal Methods ////// Factory method to create ObjectContexts /// /// /// The manager controllig the serialization process. /// /// /// The instance of the object which contained this object as /// one of its properties. /// /// /// The ObjectContext of the parent object of this object. /// /// /// The property from which this object was driven to serialization. /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] internal static SerializableObjectContext CreateContext( PackageSerializationManager serializationManager, object serializableObject, SerializableObjectContext serializableObjectParentContext, SerializablePropertyContext serializablePropertyContext ) { // // Check for element pre-existance to avoid infinite loops // in the process of serialization // int stackIndex = 0; object currentObject = null; for(currentObject = serializationManager.GraphContextStack[stackIndex]; currentObject != null; currentObject = serializationManager.GraphContextStack[++stackIndex]) { SerializableObjectContext currentObjectContext = currentObject as SerializableObjectContext; if(currentObjectContext!=null && currentObjectContext.TargetObject == serializableObject) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_CycleDetectedInSerialization)); } } SerializableObjectContext serializableObjectContext = _recycableSerializableObjectContexts.Count == 0 ? null : (SerializableObjectContext)_recycableSerializableObjectContexts.Pop(); if(serializableObjectContext == null) { serializableObjectContext = new SerializableObjectContext(serializableObject, serializablePropertyContext); } else { serializableObjectContext.Initialize(serializableObject, serializablePropertyContext); } { // // Namespace related creation within the context // MetroSerializationNamespaceTable parentNamespaceTable = serializableObjectParentContext != null ? serializableObjectParentContext.NamespaceTable : null; if (serializableObjectContext.NamespaceTable == null) { serializableObjectContext.NamespaceTable = new MetroSerializationNamespaceTable(parentNamespaceTable); } } { // // Properties related creation within the context // if(serializableObjectContext.PropertiesCollection == null) { serializableObjectContext.PropertiesCollection = new SerializablePropertyCollection(serializationManager, serializableObject); } else { serializableObjectContext.PropertiesCollection.Initialize(serializationManager, serializableObject); } } serializableObjectContext.Name = serializableObjectContext.TargetObject.GetType().Name; return serializableObjectContext; } ////// To optimize, we build a cache of created contexts /// and recycle them instead of desposing them. /// /// /// Context to recycle. /// internal static void RecycleContext( SerializableObjectContext serializableObjectContext ) { serializableObjectContext.Clear(); _recycableSerializableObjectContexts.Push(serializableObjectContext); } #endregion Internal Methods #region Public Properties ////// Query target object /// public object TargetObject { get { return _targetObject; } } ////// Query/Set namespace informaiton table /// public MetroSerializationNamespaceTable NamespaceTable { get { return _namespaceTable; } set { _namespaceTable = value; } } ////// Query/Set the collection containing all /// the properties of the current object /// public SerializablePropertyCollection PropertiesCollection { get { return _propertiesCollection; } set { _propertiesCollection = value; } } ////// Query/Set the type of the property / object being /// considered for serialization. /// public bool IsComplexValue { get { return _isComplexValue; } set { _isComplexValue = value; } } ////// Query / Set the readability type of the object /// public bool IsReadOnlyValue { get { return _isReadOnlyValue; } set { _isReadOnlyValue = value; } } #endregion Public Properties #region Public Methods ////// Initialize the context /// public void Initialize( object target, SerializablePropertyContext serializablePropertyContext ) { Initialize(); _targetObject = target; _isComplexValue = false; _backingPropertyContext = serializablePropertyContext; if(_backingPropertyContext!=null) { _isComplexValue = _backingPropertyContext.IsComplex; _isReadOnlyValue = _backingPropertyContext.IsReadOnly; } else { _isComplexValue = true; _isReadOnlyValue = false; } } ////// Clear the Context /// public override void Clear() { _targetObject = null; _isComplexValue = false; _isReadOnlyValue = false; _namespaceTable = null; if (_propertiesCollection != null) { _propertiesCollection.Clear(); } base.Clear(); } #endregion Public Methods #region Private Data private object _targetObject; private MetroSerializationNamespaceTable _namespaceTable; private SerializablePropertyCollection _propertiesCollection; private bool _isComplexValue; private bool _isReadOnlyValue; private SerializablePropertyContext _backingPropertyContext; static Stack _recycableSerializableObjectContexts; #endregion Private Data }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
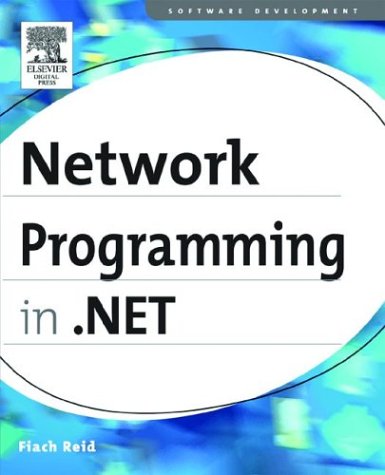
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LambdaCompiler.ControlFlow.cs
- DynamicResourceExtension.cs
- ProtocolsSection.cs
- EventManager.cs
- CatalogZone.cs
- PlainXmlWriter.cs
- MessageQueueAccessControlEntry.cs
- VectorValueSerializer.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- SHA256Managed.cs
- EntryPointNotFoundException.cs
- ScriptMethodAttribute.cs
- PersistChildrenAttribute.cs
- MetadataWorkspace.cs
- SiteMapNode.cs
- TextBox.cs
- SqlParameter.cs
- HtmlShim.cs
- WebBrowserHelper.cs
- FormViewUpdatedEventArgs.cs
- TextTrailingWordEllipsis.cs
- DataGridViewToolTip.cs
- NeutralResourcesLanguageAttribute.cs
- WebPartEditVerb.cs
- EntityDataSourceConfigureObjectContext.cs
- OracleConnectionString.cs
- DbProviderFactory.cs
- VirtualPathUtility.cs
- TakeOrSkipQueryOperator.cs
- CodeDesigner.cs
- HitTestParameters3D.cs
- SwitchAttribute.cs
- VirtualDirectoryMappingCollection.cs
- COM2Properties.cs
- UrlSyndicationContent.cs
- ChannelServices.cs
- followingsibling.cs
- SafeEventHandle.cs
- CodeIdentifier.cs
- ColorContext.cs
- ConfigPathUtility.cs
- EncoderReplacementFallback.cs
- DataGridColumnCollection.cs
- Quad.cs
- ItemCheckEvent.cs
- ConsoleKeyInfo.cs
- Pair.cs
- WebPartDisplayMode.cs
- TemplateXamlTreeBuilder.cs
- ClientConfigurationSystem.cs
- DateTimePicker.cs
- TextUtf8RawTextWriter.cs
- DefaultProxySection.cs
- ResXBuildProvider.cs
- NamespaceEmitter.cs
- AspNetHostingPermission.cs
- TableLayoutStyleCollection.cs
- httpapplicationstate.cs
- InheritanceRules.cs
- TrayIconDesigner.cs
- IListConverters.cs
- SQLInt32Storage.cs
- GestureRecognitionResult.cs
- MetadataSource.cs
- AddingNewEventArgs.cs
- dtdvalidator.cs
- CodeTypeConstructor.cs
- MethodExpr.cs
- FunctionImportMapping.cs
- ProxyManager.cs
- XPathBuilder.cs
- StringBuilder.cs
- MembershipSection.cs
- VerticalAlignConverter.cs
- StrokeNodeOperations.cs
- ExtentKey.cs
- EntityClientCacheEntry.cs
- StyleSheetDesigner.cs
- RemotingServices.cs
- PropertyInformation.cs
- XmlSchemaSet.cs
- QilSortKey.cs
- SafeWaitHandle.cs
- CalendarBlackoutDatesCollection.cs
- TraceHandler.cs
- XPathSingletonIterator.cs
- FullTrustAssemblyCollection.cs
- EdmItemError.cs
- EmbeddedMailObject.cs
- DirectoryLocalQuery.cs
- DataTableNameHandler.cs
- Baml2006KeyRecord.cs
- DataGridViewTextBoxColumn.cs
- MediaScriptCommandRoutedEventArgs.cs
- TablePatternIdentifiers.cs
- UrlRoutingModule.cs
- Pts.cs
- CompilerHelpers.cs
- ColorPalette.cs
- BezierSegment.cs