Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / GiveFeedbackEventArgs.cs / 1 / GiveFeedbackEventArgs.cs
//---------------------------------------------------------------------------- // // File: GiveFeedbackEventArgs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: GiveFeedbackEventArgs for drag-and-drop operation.// // // History: // 08/19/2004 : sangilj Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Windows { ////// The GiveFeedbackEventArgs class represents a type of RoutedEventArgs that /// are relevant to GiveFeedback. /// public sealed class GiveFeedbackEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initializes a new instance of the GiveFeedbackEventArgs class. /// /// /// The effect of the drag operation. /// /// /// Use the default cursors. /// internal GiveFeedbackEventArgs(DragDropEffects effects, bool useDefaultCursors) { if (!DragDrop.IsValidDragDropEffects(effects)) { Debug.Assert(false, "Invalid effects"); } this._effects = effects; this._useDefaultCursors = useDefaultCursors; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// The effects of drag operation /// public DragDropEffects Effects { get { return _effects; } } ////// Use the default cursors. /// public bool UseDefaultCursors { get { return _useDefaultCursors; } set { _useDefaultCursors = value; } } #endregion Public Methods #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { GiveFeedbackEventHandler handler = (GiveFeedbackEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private DragDropEffects _effects; private bool _useDefaultCursors; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: GiveFeedbackEventArgs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: GiveFeedbackEventArgs for drag-and-drop operation.// // // History: // 08/19/2004 : sangilj Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Windows { ////// The GiveFeedbackEventArgs class represents a type of RoutedEventArgs that /// are relevant to GiveFeedback. /// public sealed class GiveFeedbackEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initializes a new instance of the GiveFeedbackEventArgs class. /// /// /// The effect of the drag operation. /// /// /// Use the default cursors. /// internal GiveFeedbackEventArgs(DragDropEffects effects, bool useDefaultCursors) { if (!DragDrop.IsValidDragDropEffects(effects)) { Debug.Assert(false, "Invalid effects"); } this._effects = effects; this._useDefaultCursors = useDefaultCursors; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// The effects of drag operation /// public DragDropEffects Effects { get { return _effects; } } ////// Use the default cursors. /// public bool UseDefaultCursors { get { return _useDefaultCursors; } set { _useDefaultCursors = value; } } #endregion Public Methods #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { GiveFeedbackEventHandler handler = (GiveFeedbackEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private DragDropEffects _effects; private bool _useDefaultCursors; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
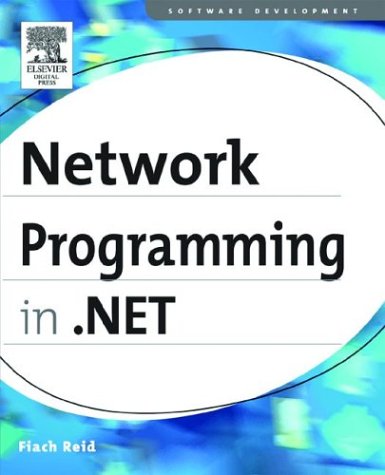
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessInputEventArgs.cs
- FixedSOMLineCollection.cs
- DashStyles.cs
- NativeMethods.cs
- PaintValueEventArgs.cs
- ISCIIEncoding.cs
- IfAction.cs
- Identity.cs
- WebConfigurationManager.cs
- UrlAuthorizationModule.cs
- ExtentKey.cs
- IndependentAnimationStorage.cs
- FileDialog_Vista_Interop.cs
- CultureInfo.cs
- ViewGenerator.cs
- AnchoredBlock.cs
- webproxy.cs
- BitmapEncoder.cs
- CryptoApi.cs
- ApplicationInfo.cs
- RowToParametersTransformer.cs
- IndexOutOfRangeException.cs
- TextBlockAutomationPeer.cs
- CombinedHttpChannel.cs
- XmlWrappingWriter.cs
- ContentAlignmentEditor.cs
- ListViewItemMouseHoverEvent.cs
- BulletedList.cs
- XmlRawWriterWrapper.cs
- FrameAutomationPeer.cs
- EventPropertyMap.cs
- EDesignUtil.cs
- SaveFileDialog.cs
- HttpClientProtocol.cs
- IODescriptionAttribute.cs
- SafeEventLogWriteHandle.cs
- RelativeSource.cs
- ValidationErrorCollection.cs
- WorkflowWebHostingModule.cs
- ColumnResizeUndoUnit.cs
- ButtonDesigner.cs
- CharStorage.cs
- StaticTextPointer.cs
- MulticastDelegate.cs
- DockPatternIdentifiers.cs
- ReadOnlyDictionary.cs
- SingleConverter.cs
- FileChangesMonitor.cs
- OdbcCommandBuilder.cs
- SizeF.cs
- HtmlTableCellCollection.cs
- RuntimeConfigLKG.cs
- OdbcEnvironment.cs
- KeyTime.cs
- BinaryQueryOperator.cs
- WebFormDesignerActionService.cs
- CredentialSelector.cs
- DesignerForm.cs
- ToolStripLocationCancelEventArgs.cs
- ProcessModelSection.cs
- OpenTypeCommon.cs
- DataGridItem.cs
- SharedDp.cs
- OracleBinary.cs
- ExpandSegmentCollection.cs
- EventBindingService.cs
- basemetadatamappingvisitor.cs
- XmlCodeExporter.cs
- BindingMemberInfo.cs
- ValidationError.cs
- JsonStringDataContract.cs
- ManagementClass.cs
- DataGridViewToolTip.cs
- InternalBufferOverflowException.cs
- HashHelper.cs
- SiteMapNodeItemEventArgs.cs
- isolationinterop.cs
- ValueSerializer.cs
- CommonRemoteMemoryBlock.cs
- CatalogZoneBase.cs
- WebContentFormatHelper.cs
- SqlStream.cs
- QuaternionConverter.cs
- GraphicsPathIterator.cs
- PartialCachingControl.cs
- ClientRolePrincipal.cs
- AuthenticationServiceManager.cs
- AddInControllerImpl.cs
- ClassImporter.cs
- GPPOINTF.cs
- MostlySingletonList.cs
- Win32KeyboardDevice.cs
- Point3DAnimationBase.cs
- Task.cs
- SourceExpressionException.cs
- PasswordTextNavigator.cs
- PropertyConverter.cs
- SocketPermission.cs
- DataBoundLiteralControl.cs
- KeyFrames.cs