Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / SHA384CryptoServiceProvider.cs / 1305376 / SHA384CryptoServiceProvider.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ////// Wrapper around the CAPI implementation of the SHA-384 hashing algorithm /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class SHA384CryptoServiceProvider : SHA384 { private CapiHashAlgorithm m_hashAlgorithm; //// [System.Security.SecurityCritical] public SHA384CryptoServiceProvider() { Contract.Ensures(m_hashAlgorithm != null); m_hashAlgorithm = new CapiHashAlgorithm(CapiNative.ProviderNames.MicrosoftEnhancedRsaAes, CapiNative.ProviderType.RsaAes, CapiNative.AlgorithmId.Sha384); } //// // [System.Security.SecurityCritical] protected override void Dispose(bool disposing) { try { if (disposing) { m_hashAlgorithm.Dispose(); } } finally { base.Dispose(disposing); } } ///// /// Reset the hash algorithm to begin hashing a new set of data /// //// [System.Security.SecurityCritical] public override void Initialize() { Contract.Assert(m_hashAlgorithm != null); m_hashAlgorithm.Initialize(); } ///// /// Hash a block of data /// //// [System.Security.SecurityCritical] protected override void HashCore(byte[] array, int ibStart, int cbSize) { Contract.Assert(m_hashAlgorithm != null); m_hashAlgorithm.HashCore(array, ibStart, cbSize); } ///// /// Complete the hash, returning its value /// //// [System.Security.SecurityCritical] protected override byte[] HashFinal() { Contract.Assert(m_hashAlgorithm != null); return m_hashAlgorithm.HashFinal(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ///// /// Wrapper around the CAPI implementation of the SHA-384 hashing algorithm /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class SHA384CryptoServiceProvider : SHA384 { private CapiHashAlgorithm m_hashAlgorithm; //// [System.Security.SecurityCritical] public SHA384CryptoServiceProvider() { Contract.Ensures(m_hashAlgorithm != null); m_hashAlgorithm = new CapiHashAlgorithm(CapiNative.ProviderNames.MicrosoftEnhancedRsaAes, CapiNative.ProviderType.RsaAes, CapiNative.AlgorithmId.Sha384); } //// // [System.Security.SecurityCritical] protected override void Dispose(bool disposing) { try { if (disposing) { m_hashAlgorithm.Dispose(); } } finally { base.Dispose(disposing); } } ///// /// Reset the hash algorithm to begin hashing a new set of data /// //// [System.Security.SecurityCritical] public override void Initialize() { Contract.Assert(m_hashAlgorithm != null); m_hashAlgorithm.Initialize(); } ///// /// Hash a block of data /// //// [System.Security.SecurityCritical] protected override void HashCore(byte[] array, int ibStart, int cbSize) { Contract.Assert(m_hashAlgorithm != null); m_hashAlgorithm.HashCore(array, ibStart, cbSize); } ///// /// Complete the hash, returning its value /// //// [System.Security.SecurityCritical] protected override byte[] HashFinal() { Contract.Assert(m_hashAlgorithm != null); return m_hashAlgorithm.HashFinal(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.//
Link Menu
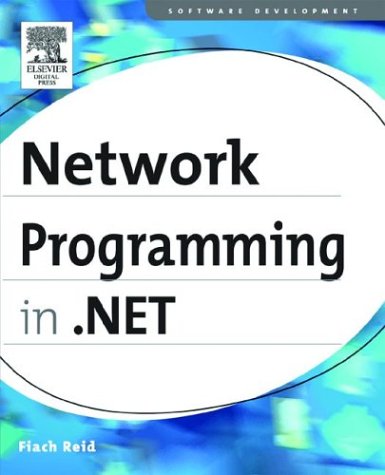
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Binding.cs
- WebPartDisplayModeCancelEventArgs.cs
- FillRuleValidation.cs
- ResourceDescriptionAttribute.cs
- AttributedMetaModel.cs
- GridViewRowPresenter.cs
- SpellerError.cs
- SpellerHighlightLayer.cs
- ObjectStateEntryDbDataRecord.cs
- AdornedElementPlaceholder.cs
- ZipIOModeEnforcingStream.cs
- DomainUpDown.cs
- PrinterResolution.cs
- TypeSystem.cs
- ParserOptions.cs
- ScrollPattern.cs
- ReferenceTypeElement.cs
- DataFieldConverter.cs
- EntityDataSourceWrapperCollection.cs
- GridItemPattern.cs
- PopupRootAutomationPeer.cs
- XsdBuildProvider.cs
- MetadataItemEmitter.cs
- RuntimeConfigurationRecord.cs
- QueryContinueDragEvent.cs
- ConfigurationLoaderException.cs
- Focus.cs
- MergePropertyDescriptor.cs
- XmlComment.cs
- DetailsViewDesigner.cs
- UserValidatedEventArgs.cs
- Point3DCollectionConverter.cs
- BevelBitmapEffect.cs
- OpacityConverter.cs
- CheckBoxFlatAdapter.cs
- DbConnectionPoolGroup.cs
- DependencyObjectPropertyDescriptor.cs
- EpmSyndicationContentDeSerializer.cs
- RowTypePropertyElement.cs
- TreeNodeEventArgs.cs
- XPathSelfQuery.cs
- TdsParserStaticMethods.cs
- SimpleLine.cs
- ResourcePool.cs
- SqlVisitor.cs
- ScrollContentPresenter.cs
- LinqDataSource.cs
- CodeSubDirectory.cs
- ConditionalAttribute.cs
- TextViewBase.cs
- InfiniteTimeSpanConverter.cs
- WebPartConnectionsDisconnectVerb.cs
- BrowserCapabilitiesFactory.cs
- VerificationException.cs
- DataGridViewTopLeftHeaderCell.cs
- CrossContextChannel.cs
- DrawingVisualDrawingContext.cs
- GenericPrincipal.cs
- TextParentUndoUnit.cs
- XmlSortKeyAccumulator.cs
- SqlMethodAttribute.cs
- DocumentGridContextMenu.cs
- UniqueEventHelper.cs
- QueryAccessibilityHelpEvent.cs
- EntityDataSourceContextDisposingEventArgs.cs
- ColumnWidthChangingEvent.cs
- AddInPipelineAttributes.cs
- RootBuilder.cs
- ValidationHelper.cs
- HostedTransportConfigurationManager.cs
- ProcessHostConfigUtils.cs
- GenericAuthenticationEventArgs.cs
- EdmPropertyAttribute.cs
- WebControlsSection.cs
- ComProxy.cs
- BufferedGraphicsContext.cs
- MaskedTextBox.cs
- HasCopySemanticsAttribute.cs
- EncodingDataItem.cs
- SendMailErrorEventArgs.cs
- ReferencedCollectionType.cs
- FileLevelControlBuilderAttribute.cs
- CharConverter.cs
- BookmarkUndoUnit.cs
- ProfileManager.cs
- XmlReaderDelegator.cs
- GridViewSelectEventArgs.cs
- xmlsaver.cs
- ClaimComparer.cs
- X509CertificateRecipientClientCredential.cs
- RuleAttributes.cs
- CompiledRegexRunnerFactory.cs
- ViewSimplifier.cs
- Size3D.cs
- Utilities.cs
- GetMemberBinder.cs
- Glyph.cs
- MethodBody.cs
- MissingMemberException.cs
- URIFormatException.cs