Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintPreviewGraphics.cs / 1305376 / PrintPreviewGraphics.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Drawing.Internal; using System.Drawing.Imaging; using System.Drawing.Text; using System.Drawing.Drawing2D; using System.Drawing.Printing; using System.Runtime.Versioning; ////// /// internal class PrintPreviewGraphics { private PrintPageEventArgs printPageEventArgs; private PrintDocument printDocument; public PrintPreviewGraphics(PrintDocument document, PrintPageEventArgs e) { printPageEventArgs = e; printDocument = document; } ///Retrives the printer graphics during preview. ////// /// Gets the Visible bounds of this graphics object. Used during print preview. /// public RectangleF VisibleClipBounds { [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] get { IntPtr hdevMode = printPageEventArgs.PageSettings.PrinterSettings.GetHdevmodeInternal(); using( DeviceContext dc = printPageEventArgs.PageSettings.PrinterSettings.CreateDeviceContext(hdevMode)) { using( Graphics graphics = Graphics.FromHdcInternal(dc.Hdc) ) { if (printDocument.OriginAtMargins) { // Adjust the origin of the graphics object to be at the user-specified margin location // Note: Graphics.FromHdc internally calls SaveDC(hdc), we can still use the saved hdc to get the resolution. int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); float hardMarginX = hardMarginX_DU * 100 / dpiX; float hardMarginY = hardMarginY_DU * 100 / dpiY; graphics.TranslateTransform(-hardMarginX, -hardMarginY); graphics.TranslateTransform(printDocument.DefaultPageSettings.Margins.Left, printDocument.DefaultPageSettings.Margins.Top); } return graphics.VisibleClipBounds; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Drawing.Internal; using System.Drawing.Imaging; using System.Drawing.Text; using System.Drawing.Drawing2D; using System.Drawing.Printing; using System.Runtime.Versioning; ////// /// internal class PrintPreviewGraphics { private PrintPageEventArgs printPageEventArgs; private PrintDocument printDocument; public PrintPreviewGraphics(PrintDocument document, PrintPageEventArgs e) { printPageEventArgs = e; printDocument = document; } ///Retrives the printer graphics during preview. ////// /// Gets the Visible bounds of this graphics object. Used during print preview. /// public RectangleF VisibleClipBounds { [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] get { IntPtr hdevMode = printPageEventArgs.PageSettings.PrinterSettings.GetHdevmodeInternal(); using( DeviceContext dc = printPageEventArgs.PageSettings.PrinterSettings.CreateDeviceContext(hdevMode)) { using( Graphics graphics = Graphics.FromHdcInternal(dc.Hdc) ) { if (printDocument.OriginAtMargins) { // Adjust the origin of the graphics object to be at the user-specified margin location // Note: Graphics.FromHdc internally calls SaveDC(hdc), we can still use the saved hdc to get the resolution. int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); float hardMarginX = hardMarginX_DU * 100 / dpiX; float hardMarginY = hardMarginY_DU * 100 / dpiY; graphics.TranslateTransform(-hardMarginX, -hardMarginY); graphics.TranslateTransform(printDocument.DefaultPageSettings.Margins.Left, printDocument.DefaultPageSettings.Margins.Top); } return graphics.VisibleClipBounds; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
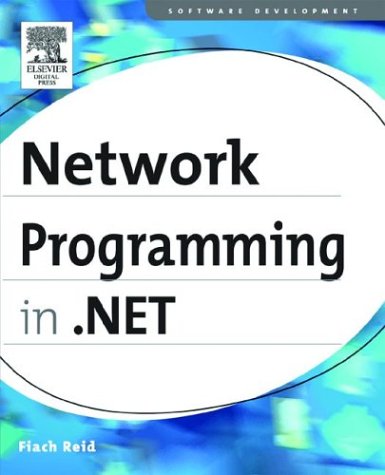
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Debugger.cs
- EventWaitHandle.cs
- PaintValueEventArgs.cs
- BindingParameterCollection.cs
- XsdDataContractImporter.cs
- Viewport2DVisual3D.cs
- DataGridPageChangedEventArgs.cs
- XmlAttributes.cs
- InvalidCastException.cs
- BitmapFrame.cs
- GlobalProxySelection.cs
- ReadOnlyTernaryTree.cs
- SessionIDManager.cs
- TrayIconDesigner.cs
- EntityParameterCollection.cs
- CompModSwitches.cs
- FormsAuthenticationUser.cs
- SyndicationSerializer.cs
- CmsInterop.cs
- FileIOPermission.cs
- Decimal.cs
- BrushProxy.cs
- InertiaRotationBehavior.cs
- AutoGeneratedFieldProperties.cs
- Operators.cs
- XmlSchemaInclude.cs
- ChameleonKey.cs
- SoundPlayer.cs
- Blend.cs
- LinqDataSource.cs
- TextRangeEditLists.cs
- ManagedWndProcTracker.cs
- MDIControlStrip.cs
- PeerMessageDispatcher.cs
- RoleGroup.cs
- Stroke2.cs
- Assembly.cs
- RawStylusSystemGestureInputReport.cs
- DoubleLinkListEnumerator.cs
- Button.cs
- TextAction.cs
- ConfigLoader.cs
- AttributeXamlType.cs
- Metafile.cs
- ArglessEventHandlerProxy.cs
- FormsAuthenticationModule.cs
- StreamingContext.cs
- ValueConversionAttribute.cs
- SupportingTokenSpecification.cs
- InvalidWorkflowException.cs
- KeyValueSerializer.cs
- AlgoModule.cs
- Polyline.cs
- AddInIpcChannel.cs
- ReflectEventDescriptor.cs
- XmlDomTextWriter.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- DataGridViewMethods.cs
- CultureData.cs
- TimeoutException.cs
- DeviceFiltersSection.cs
- PageVisual.cs
- CollectionDataContractAttribute.cs
- TextEffect.cs
- HyperLinkDataBindingHandler.cs
- Pair.cs
- LayoutDump.cs
- IsolatedStoragePermission.cs
- DBCommandBuilder.cs
- DocumentXPathNavigator.cs
- LiteralControl.cs
- Tile.cs
- MediaElementAutomationPeer.cs
- PreProcessor.cs
- OleDbException.cs
- AnnotationComponentChooser.cs
- AutomationElementIdentifiers.cs
- DataServiceRequestException.cs
- WorkItem.cs
- EntitySqlException.cs
- SqlMethodAttribute.cs
- GridErrorDlg.cs
- BinaryObjectInfo.cs
- TextAdaptor.cs
- BlurEffect.cs
- ReadOnlyNameValueCollection.cs
- PathFigure.cs
- FigureParagraph.cs
- BinHexDecoder.cs
- FontWeights.cs
- ViewStateModeByIdAttribute.cs
- XmlSchemaSimpleTypeList.cs
- RegexInterpreter.cs
- StorageSetMapping.cs
- EventlogProvider.cs
- itemelement.cs
- XmlSchemaObject.cs
- Item.cs
- TextStore.cs
- AmbiguousMatchException.cs