Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / CharConverter.cs / 1 / CharConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class CharConverter : TypeConverter { ///Provides /// a type converter to convert Unicode /// character objects to and from various other representations. ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to a Unicode character object using /// the specified context. ////// Converts the given object to another type. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && value is char) { if ((char)value == (char)0) { return ""; } } return base.ConvertTo(context, culture, value, destinationType); } ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = (string)value; if (text.Length > 1) { text = text.Trim(); } if (text != null && text.Length > 0) { if (text.Length != 1) { throw new FormatException(SR.GetString(SR.ConvertInvalidPrimitive, text, "Char")); } return text[0]; } return '\0'; } return base.ConvertFrom(context, culture, value); } } }Converts the given object to a Unicode character object. ///
Link Menu
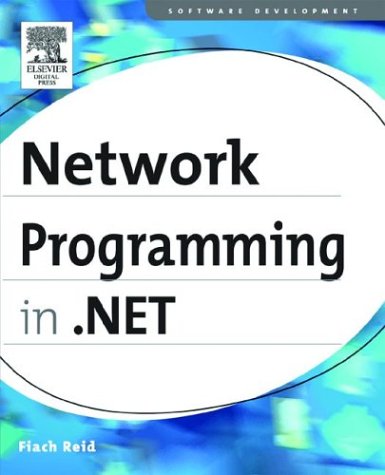
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KoreanCalendar.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- RegexRunner.cs
- SafeSerializationManager.cs
- WindowsImpersonationContext.cs
- EndpointBehaviorElement.cs
- Filter.cs
- NestPullup.cs
- WindowsContainer.cs
- ValidationHelper.cs
- hresults.cs
- Dictionary.cs
- SvcMapFileSerializer.cs
- WebPartConnectVerb.cs
- QueryStringConverter.cs
- DrawingImage.cs
- QuaternionAnimationBase.cs
- ScrollChrome.cs
- TextBlock.cs
- ControlBuilder.cs
- NamedPipeTransportSecurityElement.cs
- _LazyAsyncResult.cs
- AutomationPattern.cs
- XmlConvert.cs
- TemplateNameScope.cs
- RSAOAEPKeyExchangeFormatter.cs
- XmlILAnnotation.cs
- PointAnimationBase.cs
- FeatureSupport.cs
- HandleCollector.cs
- ConversionContext.cs
- DocumentApplicationJournalEntry.cs
- FormsAuthenticationTicket.cs
- LinqDataSourceDeleteEventArgs.cs
- LinqDataSource.cs
- Delegate.cs
- XslCompiledTransform.cs
- FlowDocumentReader.cs
- ToolBarTray.cs
- RectIndependentAnimationStorage.cs
- CalendarSelectionChangedEventArgs.cs
- XmlILAnnotation.cs
- ObjectDataSourceMethodEventArgs.cs
- LinqDataSourceValidationException.cs
- WindowsStatusBar.cs
- EntitySetRetriever.cs
- HtmlAnchor.cs
- WebPartVerbsEventArgs.cs
- DecoderReplacementFallback.cs
- ConfigurationManagerHelper.cs
- RichTextBox.cs
- SqlGenerator.cs
- DateTimeOffsetConverter.cs
- HttpValueCollection.cs
- ToolStripDropDownButton.cs
- ELinqQueryState.cs
- PaperSource.cs
- SingleKeyFrameCollection.cs
- ISAPIApplicationHost.cs
- SyndicationContent.cs
- CodeTypeReferenceCollection.cs
- SoapSchemaImporter.cs
- DetailsViewRowCollection.cs
- Wildcard.cs
- Parser.cs
- RoleService.cs
- PagedDataSource.cs
- RowToFieldTransformer.cs
- Interfaces.cs
- AddInActivator.cs
- IDQuery.cs
- FunctionDescription.cs
- ToolBarTray.cs
- FormsIdentity.cs
- nulltextnavigator.cs
- MenuCommandService.cs
- TextTreeInsertUndoUnit.cs
- UnitControl.cs
- Currency.cs
- MetaModel.cs
- ClientTargetSection.cs
- Compiler.cs
- XmlSubtreeReader.cs
- FieldValue.cs
- UpdateCompiler.cs
- FocusManager.cs
- CodeGenHelper.cs
- WebScriptClientGenerator.cs
- UIElement3D.cs
- precedingquery.cs
- RenderData.cs
- PenLineCapValidation.cs
- ViewGenResults.cs
- Label.cs
- ZeroOpNode.cs
- ContextStaticAttribute.cs
- StandardToolWindows.cs
- FixedHyperLink.cs
- ReferentialConstraint.cs
- FontWeights.cs