Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / CngProvider.cs / 1305376 / CngProvider.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.CodeAnalysis; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ////// Utility class to strongly type providers used with CNG. Since all CNG APIs which require a /// provider name take the name as a string, we use this string wrapper class to specifically mark /// which parameters are expected to be providers. We also provide a list of well known provider /// names, which helps Intellisense users find a set of good providernames to use. /// [Serializable] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngProvider : IEquatable{ private static CngProvider s_msSmartCardKsp; private static CngProvider s_msSoftwareKsp; private string m_provider; public CngProvider(string provider) { Contract.Ensures(!String.IsNullOrEmpty(m_provider)); if (provider == null) { throw new ArgumentNullException("provider"); } if (provider.Length == 0) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidProviderName, provider), "provider"); } m_provider = provider; } /// /// Name of the CNG provider /// public string Provider { get { Contract.Ensures(!String.IsNullOrEmpty(Contract.Result())); return m_provider; } } public static bool operator ==(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return Object.ReferenceEquals(right, null); } return left.Equals(right); } [Pure] public static bool operator !=(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return !Object.ReferenceEquals(right, null); } return !left.Equals(right); } public override bool Equals(object obj) { Contract.Assert(m_provider != null); return Equals(obj as CngProvider); } public bool Equals(CngProvider other) { if (Object.ReferenceEquals(other, null)) { return false; } return m_provider.Equals(other.Provider); } public override int GetHashCode() { Contract.Assert(m_provider != null); return m_provider.GetHashCode(); } public override string ToString() { Contract.Assert(m_provider != null); return m_provider.ToString(); } // // Well known NCrypt KSPs // [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "CardKey", Justification = "This is not 'Smart Cardkey', but 'Smart Card Key'")] [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "SmartCard", Justification = "Smart Card is two words in the ncrypt usage")] public static CngProvider MicrosoftSmartCardKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSmartCardKsp == null) { s_msSmartCardKsp = new CngProvider("Microsoft Smart Card Key Storage Provider"); // MS_SMART_CARD_KEY_STORAGE_PROVIDER } return s_msSmartCardKsp; } } public static CngProvider MicrosoftSoftwareKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSoftwareKsp == null) { s_msSoftwareKsp = new CngProvider("Microsoft Software Key Storage Provider"); // MS_KEY_STORAGE_PROVIDER } return s_msSoftwareKsp; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
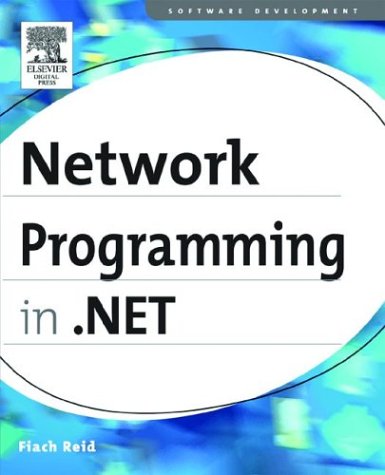
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BackgroundFormatInfo.cs
- RightsManagementResourceHelper.cs
- Simplifier.cs
- Form.cs
- ModelItemImpl.cs
- ControlPropertyNameConverter.cs
- EventWaitHandle.cs
- DataGridBoundColumn.cs
- GenericIdentity.cs
- SendMessageContent.cs
- SessionViewState.cs
- SQLInt32Storage.cs
- Clock.cs
- InvalidOperationException.cs
- SelectionRangeConverter.cs
- QuaternionRotation3D.cs
- ProxyWebPartManager.cs
- ApplyTemplatesAction.cs
- RangeBaseAutomationPeer.cs
- WorkflowTimerService.cs
- EntitySetDataBindingList.cs
- OleServicesContext.cs
- RequiredAttributeAttribute.cs
- TextTreeRootTextBlock.cs
- LogStore.cs
- ActivityTypeCodeDomSerializer.cs
- ListViewDeleteEventArgs.cs
- DefaultPrintController.cs
- FrameworkContentElement.cs
- VariableBinder.cs
- CompressStream.cs
- CodeSubDirectoriesCollection.cs
- HttpDebugHandler.cs
- QuaternionKeyFrameCollection.cs
- MailMessageEventArgs.cs
- SuppressMergeCheckAttribute.cs
- ActivityMarkupSerializer.cs
- DataTableMappingCollection.cs
- DataRelation.cs
- ElementHostAutomationPeer.cs
- RadialGradientBrush.cs
- SHA384.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- GridViewRowCollection.cs
- BoundsDrawingContextWalker.cs
- DocumentPageHost.cs
- GridViewRowCollection.cs
- input.cs
- OracleCommandBuilder.cs
- ProviderUtil.cs
- ResourceExpression.cs
- CollectionBase.cs
- dataprotectionpermission.cs
- ReadOnlyActivityGlyph.cs
- WebPartMenu.cs
- DisposableCollectionWrapper.cs
- OutOfProcStateClientManager.cs
- ClientConfigurationSystem.cs
- SmiEventSink_DeferedProcessing.cs
- ExpressionBuilder.cs
- TimeoutHelper.cs
- WebPartConnectionsCancelVerb.cs
- LeaseManager.cs
- DocumentSequenceHighlightLayer.cs
- FormViewPagerRow.cs
- DataGridViewCellStyleChangedEventArgs.cs
- InternalConfigRoot.cs
- WebConfigurationManager.cs
- VisualStyleElement.cs
- PingReply.cs
- ToolStripSystemRenderer.cs
- ParagraphVisual.cs
- ReceiveMessageAndVerifySecurityAsyncResultBase.cs
- DesignerCategoryAttribute.cs
- PkcsUtils.cs
- updatecommandorderer.cs
- ElementHostPropertyMap.cs
- ControlUtil.cs
- WebPartDisplayMode.cs
- Screen.cs
- DataGridRelationshipRow.cs
- SQLBytes.cs
- CapabilitiesUse.cs
- GridViewPageEventArgs.cs
- SoapAttributeAttribute.cs
- SQLDateTimeStorage.cs
- DesignerCapabilities.cs
- MetadataItemCollectionFactory.cs
- CreateUserWizardAutoFormat.cs
- TypeGeneratedEventArgs.cs
- CompilerError.cs
- Emitter.cs
- WebControl.cs
- TextRenderer.cs
- SQLInt16.cs
- InstanceHandleConflictException.cs
- CodeGeneratorOptions.cs
- CompilerTypeWithParams.cs
- JsonFormatReaderGenerator.cs
- BaseTemplateParser.cs