Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / CompositionCommandSet.cs / 1 / CompositionCommandSet.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.ComponentModel; using System.Diagnostics; using System; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Collections; using Microsoft.Win32; ////// /// This class implements commands that are specific to the /// composition designer. /// internal class CompositionCommandSet : CommandSet { private Control compositionUI; private CommandSetItem[] commandSet; ////// /// Constructs a new composition command set object. /// // Since we don't override GetService it is okay to suppress this. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public CompositionCommandSet(Control compositionUI, ISite site) : base(site) { Debug.Assert(compositionUI != null, "Null compositionUI passed into CompositionCommandSet"); this.compositionUI = compositionUI; // Establish our set of commands // commandSet = new CommandSetItem[] { // Keyboard commands new CommandSetItem( this, new EventHandler(OnStatusAlways), new EventHandler(OnKeySelect), MenuCommands.KeySelectNext), new CommandSetItem( this, new EventHandler(OnStatusAlways), new EventHandler(OnKeySelect), MenuCommands.KeySelectPrevious), }; if (MenuService != null) { for (int i = 0; i < commandSet.Length; i++) { MenuService.AddCommand(commandSet[i]); } } } ////// /// Disposes of this object, removing all commands from the menu service. /// public override void Dispose() { if (MenuService != null) { for (int i = 0; i < commandSet.Length; i++) { MenuService.RemoveCommand(commandSet[i]); } } base.Dispose(); } ////// /// Called for the two cancel commands we support. /// protected override bool OnKeyCancel(object sender) { // The base implementation here just checks to see if we are dragging. // If we are, then we abort the drag. // if (!base.OnKeyCancel(sender)) { ISelectionService selSvc = SelectionService; IDesignerHost host = (IDesignerHost)GetService(typeof(IDesignerHost)); if (selSvc == null || host == null) { return true; } IComponent comp = host.RootComponent; selSvc.SetSelectedComponents(new object[] {comp}, SelectionTypes.Replace); return true; } return false; } ////// /// Called for selection via the tab key. /// protected void OnKeySelect(object sender, EventArgs e) { MenuCommand cmd = (MenuCommand)sender; bool reverse = (cmd.CommandID.Equals(MenuCommands.KeySelectPrevious)); RotateTabSelection(reverse); } ////// /// This is called when the selection has changed. Anyone using CommandSetItems /// that need to update their status based on selection changes should override /// this and update their own commands at this time. The base implementaion /// runs through all base commands and calls UpdateStatus on them. /// protected override void OnUpdateCommandStatus() { // Now whip through all of the commands and ask them to update. // for (int i = 0; i < commandSet.Length; i++) { commandSet[i].UpdateStatus(); } base.OnUpdateCommandStatus(); } ////// /// Rotates the selection to the element next in the tab index. If backwards /// is set, this will rotate to the previous tab index. /// private void RotateTabSelection(bool backwards) { ISelectionService selSvc; IComponent currentComponent; Control currentControl; ComponentTray.TrayControl nextControl = null; // First, get the currently selected component // selSvc = SelectionService; if (selSvc == null) { return; } IComponent primarySelection = selSvc.PrimarySelection as IComponent; if (primarySelection != null) { currentComponent = primarySelection; } else { currentComponent = null; ICollection selection = selSvc.GetSelectedComponents(); foreach(object obj in selection) { IComponent comp = obj as IComponent; if (comp != null) { currentComponent = comp; break; } } } // Now, if we have a selected component, get the composition UI for it and // find the next control on the UI. Otherwise, we just select the first // control on the UI. // if (currentComponent != null) { currentControl = ComponentTray.TrayControl.FromComponent(currentComponent); } else { currentControl = null; } if (currentControl != null) { Debug.Assert(compositionUI.Controls[0] is LinkLabel, "First item in the Composition designer is not a linklabel"); for (int i = 1; i < compositionUI.Controls.Count; i++) { if (compositionUI.Controls[i] == currentControl) { int next = i + 1; if (next >= compositionUI.Controls.Count) { next = 1; } ComponentTray.TrayControl tc = compositionUI.Controls[next] as ComponentTray.TrayControl; if (tc != null) { nextControl = tc; } else { continue; } break; } } } else { if (compositionUI.Controls.Count > 1) { Debug.Assert(compositionUI.Controls[0] is LinkLabel, "First item in the Composition designer is not a linklabel"); ComponentTray.TrayControl tc = compositionUI.Controls[1] as ComponentTray.TrayControl; if (tc != null) { nextControl = tc; } } } // If we got a "nextControl", then select the component inside of it. // if (nextControl != null) { selSvc.SetSelectedComponents(new object[] {nextControl.Component}, SelectionTypes.Replace); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
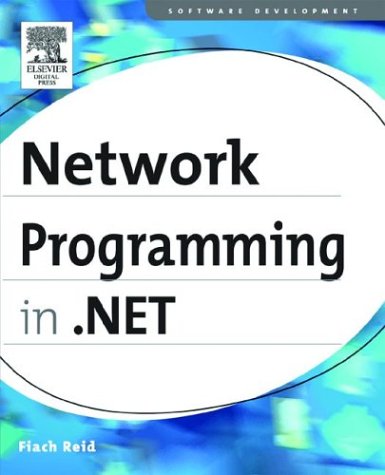
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DescriptionCreator.cs
- HelpEvent.cs
- PrivateFontCollection.cs
- MultiPartWriter.cs
- QilDataSource.cs
- TrackingCondition.cs
- ConfigurationValues.cs
- Vars.cs
- ServiceDocumentFormatter.cs
- CapabilitiesPattern.cs
- ExpressionLexer.cs
- FixedPageStructure.cs
- MachineKeySection.cs
- CopyNodeSetAction.cs
- CommandLibraryHelper.cs
- ArrayConverter.cs
- DataDocumentXPathNavigator.cs
- ValidatorCollection.cs
- DataKey.cs
- DtrList.cs
- ConstNode.cs
- ExpressionCopier.cs
- OpCellTreeNode.cs
- URI.cs
- WebPartExportVerb.cs
- CustomBinding.cs
- QueryableDataSource.cs
- SettingsPropertyCollection.cs
- LabelAutomationPeer.cs
- DesignerVerb.cs
- KeyToListMap.cs
- CacheRequest.cs
- SwitchAttribute.cs
- XmlCharCheckingReader.cs
- PolyLineSegment.cs
- CompiledRegexRunner.cs
- FtpWebResponse.cs
- BasicAsyncResult.cs
- FileUtil.cs
- OutputCacheProfile.cs
- DialogResultConverter.cs
- ISAPIWorkerRequest.cs
- DiagnosticTrace.cs
- CodeCommentStatementCollection.cs
- HttpException.cs
- SiteMapNodeCollection.cs
- PathParser.cs
- StringBuilder.cs
- TextMetrics.cs
- StatusCommandUI.cs
- ContentElement.cs
- DllHostedComPlusServiceHost.cs
- DragCompletedEventArgs.cs
- ExpanderAutomationPeer.cs
- LookupNode.cs
- IndentTextWriter.cs
- ViewBox.cs
- SqlNotificationEventArgs.cs
- PixelFormats.cs
- DataGridViewElement.cs
- ByValueEqualityComparer.cs
- ItemPager.cs
- WrappedIUnknown.cs
- _ContextAwareResult.cs
- WebPartVerbCollection.cs
- ProcessInputEventArgs.cs
- ToolBarButton.cs
- Error.cs
- MemberCollection.cs
- ping.cs
- ClipboardData.cs
- EditorZone.cs
- View.cs
- SaveLedgerEntryRequest.cs
- BrowserCapabilitiesFactoryBase.cs
- HttpPostLocalhostServerProtocol.cs
- WsdlBuildProvider.cs
- PerfService.cs
- QueryOutputWriter.cs
- Control.cs
- MSG.cs
- TextBox.cs
- TemplateControlParser.cs
- ConfigurationValues.cs
- TextEditorTyping.cs
- ProvidePropertyAttribute.cs
- TypedTableBase.cs
- DateTimeOffsetStorage.cs
- RelationalExpressions.cs
- UIElement3D.cs
- StringHandle.cs
- BaseCodeDomTreeGenerator.cs
- RelatedView.cs
- NonParentingControl.cs
- DelegatingTypeDescriptionProvider.cs
- SHA384Cng.cs
- MetadataItem_Static.cs
- SchemaElementLookUpTable.cs
- RequestBringIntoViewEventArgs.cs
- SqlClientMetaDataCollectionNames.cs