Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / XPath / Internal / Axis.cs / 1 / Axis.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal class Axis : AstNode { private AxisType axisType; private AstNode input; private string prefix; private string name; private XPathNodeType nodeType; protected bool abbrAxis; public enum AxisType { Ancestor, AncestorOrSelf, Attribute, Child, Descendant, DescendantOrSelf, Following, FollowingSibling, Namespace, Parent, Preceding, PrecedingSibling, Self, None }; // constructor public Axis(AxisType axisType, AstNode input, string prefix, string name, XPathNodeType nodetype) { Debug.Assert(prefix != null); Debug.Assert(name != null); this.axisType = axisType; this.input = input; this.prefix = prefix; this.name = name; this.nodeType = nodetype; } // constructor public Axis(AxisType axisType, AstNode input) : this(axisType, input, string.Empty, string.Empty, XPathNodeType.All) { this.abbrAxis = true; } public override AstType Type { get {return AstType.Axis;} } public override XPathResultType ReturnType { get {return XPathResultType.NodeSet;} } public AstNode Input { get {return input;} set {input = value;} } public string Prefix { get { return prefix; } } public string Name { get { return name; } } public XPathNodeType NodeType { get { return nodeType; } } public AxisType TypeOfAxis { get { return axisType; } } public bool AbbrAxis { get { return abbrAxis; } } // Used by AstTree in Schema private string urn = string.Empty; public string Urn { get { return urn; } set { urn = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal class Axis : AstNode { private AxisType axisType; private AstNode input; private string prefix; private string name; private XPathNodeType nodeType; protected bool abbrAxis; public enum AxisType { Ancestor, AncestorOrSelf, Attribute, Child, Descendant, DescendantOrSelf, Following, FollowingSibling, Namespace, Parent, Preceding, PrecedingSibling, Self, None }; // constructor public Axis(AxisType axisType, AstNode input, string prefix, string name, XPathNodeType nodetype) { Debug.Assert(prefix != null); Debug.Assert(name != null); this.axisType = axisType; this.input = input; this.prefix = prefix; this.name = name; this.nodeType = nodetype; } // constructor public Axis(AxisType axisType, AstNode input) : this(axisType, input, string.Empty, string.Empty, XPathNodeType.All) { this.abbrAxis = true; } public override AstType Type { get {return AstType.Axis;} } public override XPathResultType ReturnType { get {return XPathResultType.NodeSet;} } public AstNode Input { get {return input;} set {input = value;} } public string Prefix { get { return prefix; } } public string Name { get { return name; } } public XPathNodeType NodeType { get { return nodeType; } } public AxisType TypeOfAxis { get { return axisType; } } public bool AbbrAxis { get { return abbrAxis; } } // Used by AstTree in Schema private string urn = string.Empty; public string Urn { get { return urn; } set { urn = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
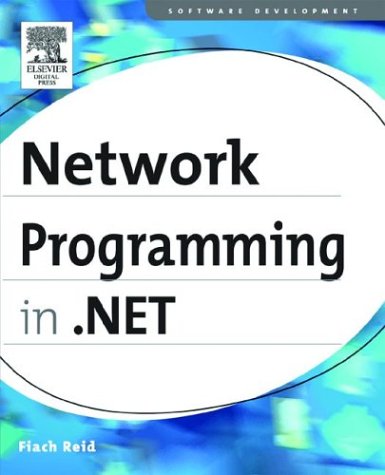
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadNeutralSemaphore.cs
- XmlSerializerFactory.cs
- _FtpDataStream.cs
- TdsEnums.cs
- ViewStateException.cs
- VectorCollectionConverter.cs
- GeometryCollection.cs
- DriveInfo.cs
- DataGridRowHeaderAutomationPeer.cs
- RegexInterpreter.cs
- RtType.cs
- MediaSystem.cs
- LinkedResource.cs
- Int16.cs
- mediaeventargs.cs
- ColorConvertedBitmap.cs
- TraceUtility.cs
- X509ChainPolicy.cs
- DesignerCommandAdapter.cs
- XsltQilFactory.cs
- PropertyOrder.cs
- StringFormat.cs
- regiisutil.cs
- ProfileEventArgs.cs
- _NativeSSPI.cs
- SafeNativeMethods.cs
- SchemaNames.cs
- InputProcessorProfiles.cs
- ServiceManager.cs
- PageAsyncTaskManager.cs
- XsdBuilder.cs
- ContextBase.cs
- StreamUpdate.cs
- NativeMethods.cs
- ToolStripPanelRenderEventArgs.cs
- SaveFileDialog.cs
- SchemaConstraints.cs
- BinaryFormatter.cs
- ContentValidator.cs
- JumpPath.cs
- StateBag.cs
- RegistryPermission.cs
- BooleanConverter.cs
- ViewGenResults.cs
- DynamicValueConverter.cs
- DecimalAnimation.cs
- HtmlControl.cs
- Cursor.cs
- PageThemeParser.cs
- TextTabProperties.cs
- XmlRawWriter.cs
- PolyQuadraticBezierSegment.cs
- PaintEvent.cs
- DocumentViewer.cs
- DefaultTypeArgumentAttribute.cs
- CodeChecksumPragma.cs
- ToolStripManager.cs
- AdornerDecorator.cs
- TabRenderer.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- ConsumerConnectionPointCollection.cs
- StringBuilder.cs
- HelpHtmlBuilder.cs
- ContentTextAutomationPeer.cs
- AuthenticatingEventArgs.cs
- EventArgs.cs
- EntityWrapper.cs
- ECDiffieHellmanCng.cs
- IERequestCache.cs
- SimpleHandlerFactory.cs
- SecurityTokenReferenceStyle.cs
- ReversePositionQuery.cs
- WebPartsPersonalizationAuthorization.cs
- CharacterBufferReference.cs
- PtsCache.cs
- ParserOptions.cs
- InkCanvasFeedbackAdorner.cs
- CultureNotFoundException.cs
- SurrogateSelector.cs
- GCHandleCookieTable.cs
- WebPartZoneCollection.cs
- RelatedEnd.cs
- SID.cs
- ChildDocumentBlock.cs
- Drawing.cs
- DiscreteKeyFrames.cs
- ReferenceConverter.cs
- UnsafeNativeMethods.cs
- AccessorTable.cs
- MouseGestureConverter.cs
- NameSpaceEvent.cs
- DescendantOverDescendantQuery.cs
- uribuilder.cs
- CodeTypeReference.cs
- CompilationUtil.cs
- CollectionsUtil.cs
- ConsoleCancelEventArgs.cs
- OptimalBreakSession.cs
- LinqDataSourceUpdateEventArgs.cs
- HtmlCommandAdapter.cs