Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / RadioButton.cs / 1 / RadioButton.cs
using System; using System.Collections; using System.ComponentModel; using System.Threading; using System.Windows.Threading; using System.Windows.Automation; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows; using System.Windows.Input; using System.Windows.Media; using MS.Utility; // Disable CS3001: Warning as Error: not CLS-compliant #pragma warning disable 3001 namespace System.Windows.Controls { ////// RadioButton implements option button with two states: true or false /// [Localizability(LocalizationCategory.RadioButton)] public class RadioButton : ToggleButton { #region Constructors static RadioButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(RadioButton), new FrameworkPropertyMetadata(typeof(RadioButton))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(RadioButton)); KeyboardNavigation.AcceptsReturnProperty.OverrideMetadata(typeof(RadioButton), new FrameworkPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); } ////// Default RadioButton constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public RadioButton() : base() { } #endregion #region private helpers private static void OnGroupNameChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RadioButton radioButton = (RadioButton)d; string groupName = e.NewValue as string; string currentlyRegisteredGroupName = _currentlyRegisteredGroupName.GetValue(radioButton); if (groupName != currentlyRegisteredGroupName) { // Unregister the old group name if set if (!string.IsNullOrEmpty(currentlyRegisteredGroupName)) Unregister(currentlyRegisteredGroupName, radioButton); // Register the new group name is set if (!string.IsNullOrEmpty(groupName)) Register(groupName, radioButton); } } private static void Register(string groupName, RadioButton radioButton) { if (_groupNameToElements == null) _groupNameToElements = new Hashtable(1); lock (_groupNameToElements) { ArrayList elements = (ArrayList)_groupNameToElements[groupName]; if (elements == null) { elements = new ArrayList(1); _groupNameToElements[groupName] = elements; } else { // There were some elements there, remove dead ones PurgeDead(elements, null); } elements.Add(new WeakReference(radioButton)); } _currentlyRegisteredGroupName.SetValue(radioButton, groupName); } private static void Unregister(string groupName, RadioButton radioButton) { if (_groupNameToElements == null) return; lock (_groupNameToElements) { // Get all elements bound to this key and remove this element ArrayList elements = (ArrayList)_groupNameToElements[groupName]; if (elements != null) { PurgeDead(elements, radioButton); if (elements.Count == 0) { _groupNameToElements.Remove(groupName); } } } _currentlyRegisteredGroupName.SetValue(radioButton, null); } private static void PurgeDead(ArrayList elements, object elementToRemove) { for (int i = 0; i < elements.Count; ) { WeakReference weakReference = (WeakReference)elements[i]; object element = weakReference.Target; if (element == null || element == elementToRemove) { elements.RemoveAt(i); } else { i++; } } } private void UpdateRadioButtonGroup() { string groupName = GroupName; if (!string.IsNullOrEmpty(groupName)) { Visual rootScope = KeyboardNavigation.GetVisualRoot(this); if (_groupNameToElements == null) _groupNameToElements = new Hashtable(1); lock (_groupNameToElements) { // Get all elements bound to this key and remove this element ArrayList elements = (ArrayList)_groupNameToElements[groupName]; for (int i = 0; i < elements.Count; ) { WeakReference weakReference = (WeakReference)elements[i]; RadioButton rb = weakReference.Target as RadioButton; if (rb == null) { // Remove dead instances elements.RemoveAt(i); } else { // Uncheck all checked RadioButtons different from the current one if (rb != this && (rb.IsChecked == true) && rootScope == KeyboardNavigation.GetVisualRoot(rb)) rb.UncheckRadioButton(); i++; } } } } else // Logical parent should be the group { DependencyObject parent = this.Parent; if (parent != null) { // Traverse logical children IEnumerable children = LogicalTreeHelper.GetChildren(parent); IEnumerator itor = children.GetEnumerator(); while (itor.MoveNext()) { RadioButton rb = itor.Current as RadioButton; if (rb != null && rb != this && string.IsNullOrEmpty(rb.GroupName) && (rb.IsChecked == true)) rb.UncheckRadioButton(); } } } } private void UncheckRadioButton() { ClearValue(IsCheckedProperty); // If IsChecked is still true and the value source is not a trigger - set local value to false if (IsChecked == true) { bool hasModifiers; BaseValueSourceInternal valueSource = GetValueSource(IsCheckedProperty, null, out hasModifiers); if (valueSource != BaseValueSourceInternal.ThemeStyleTrigger && valueSource != BaseValueSourceInternal.TemplateTrigger && valueSource != BaseValueSourceInternal.ParentTemplateTrigger) { IsChecked = false; } } } #endregion #region Properties and Events ////// The DependencyID for the GroupName property. /// Default Value: "String.Empty" /// public static readonly DependencyProperty GroupNameProperty = DependencyProperty.Register( "GroupName", typeof(string), typeof(RadioButton), new FrameworkPropertyMetadata(String.Empty, new PropertyChangedCallback(OnGroupNameChanged))); ////// GroupName determine mutually excusive radiobutton groups /// [DefaultValue("")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public string GroupName { get { return (string)GetValue(GroupNameProperty); } set { SetValue(GroupNameProperty, value); } } #endregion #region Override methods ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.RadioButtonAutomationPeer(this); } ///) /// /// This method is invoked when the IsChecked becomes true. /// /// RoutedEventArgs. protected override void OnChecked(RoutedEventArgs e) { // If RadioButton is checked we should uncheck the others in the same group UpdateRadioButtonGroup(); base.OnChecked(e); } ////// This override method is called from OnClick(). /// RadioButton implements its own toggle behavior /// protected internal override void OnToggle() { IsChecked = true; } ////// The Access key for this control was invoked. /// /// protected override void OnAccessKey(AccessKeyEventArgs e) { if (!IsKeyboardFocused) { Focus(); } base.OnAccessKey(e); } #endregion #region Accessibility #endregion Accessibility #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey #region private data [ThreadStatic] private static Hashtable _groupNameToElements; private static readonly UncommonField_currentlyRegisteredGroupName = new UncommonField (); #endregion private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
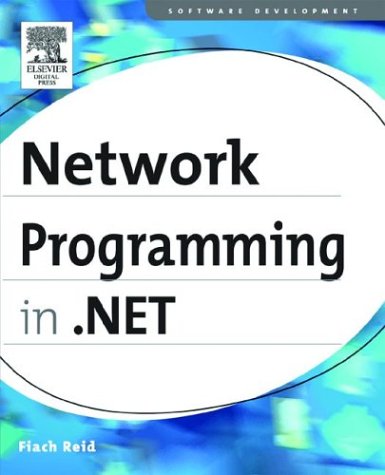
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Inline.cs
- PathParser.cs
- AnimationClock.cs
- TemplatedControlDesigner.cs
- RoutedEvent.cs
- EarlyBoundInfo.cs
- XmlBinaryReader.cs
- ThemeInfoAttribute.cs
- CheckBoxRenderer.cs
- CollectionView.cs
- CodeDomSerializerException.cs
- XamlSerializerUtil.cs
- SafeProcessHandle.cs
- UInt16.cs
- SafeRightsManagementPubHandle.cs
- _SingleItemRequestCache.cs
- URIFormatException.cs
- StringArrayConverter.cs
- XmlConvert.cs
- IndexOutOfRangeException.cs
- EntityDataSourceWizardForm.cs
- DomainUpDown.cs
- StatusBarDrawItemEvent.cs
- SBCSCodePageEncoding.cs
- HtmlInputPassword.cs
- CodeComment.cs
- Events.cs
- MetaType.cs
- MarkerProperties.cs
- SynchronizationFilter.cs
- ObjectDataSourceFilteringEventArgs.cs
- TabPanel.cs
- MimeTypeAttribute.cs
- DrawingServices.cs
- WorkflowServiceInstance.cs
- WsdlContractConversionContext.cs
- OleDbConnection.cs
- BitmapSizeOptions.cs
- ArgIterator.cs
- TCPClient.cs
- filewebresponse.cs
- WindowPatternIdentifiers.cs
- RemoteWebConfigurationHostStream.cs
- DataTable.cs
- BitmapEffectDrawing.cs
- DataGridViewCellCollection.cs
- ReferentialConstraint.cs
- EntityDesignerUtils.cs
- HMACRIPEMD160.cs
- DesignTimeVisibleAttribute.cs
- updateconfighost.cs
- AttributeEmitter.cs
- FormViewCommandEventArgs.cs
- Debug.cs
- GridViewSelectEventArgs.cs
- SecurityKeyIdentifier.cs
- MenuItemStyle.cs
- Themes.cs
- PriorityBindingExpression.cs
- DataAccessor.cs
- SchemaTableColumn.cs
- PtsCache.cs
- UnsafeNativeMethods.cs
- CodeCompiler.cs
- WorkflowServiceBehavior.cs
- RequestQueue.cs
- ChildTable.cs
- RoutedEventConverter.cs
- DocumentAutomationPeer.cs
- GrammarBuilderDictation.cs
- PageHandlerFactory.cs
- TemplatedAdorner.cs
- ViewStateModeByIdAttribute.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- FilterQueryOptionExpression.cs
- StatusBarItemAutomationPeer.cs
- Unit.cs
- Screen.cs
- ResourceKey.cs
- AddInServer.cs
- Compiler.cs
- DetailsViewUpdatedEventArgs.cs
- TypefaceCollection.cs
- BindingNavigator.cs
- HotCommands.cs
- SimpleBitVector32.cs
- Configuration.cs
- WriteableBitmap.cs
- SQLDoubleStorage.cs
- PerfCounters.cs
- ConfigViewGenerator.cs
- RelatedCurrencyManager.cs
- prompt.cs
- TextBoxRenderer.cs
- WebExceptionStatus.cs
- FunctionImportMapping.cs
- FontFamilyConverter.cs
- AnonymousIdentificationModule.cs
- ReachFixedDocumentSerializerAsync.cs
- OutKeywords.cs