Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapSourceSafeMILHandle.cs / 1 / BitmapSourceSafeMILHandle.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A sub-class of SafeMILHandle that can estimate size for bitmap // source objects. //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using MS.Internal; using MS.Win32; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media.Imaging { ////// Constructor which computes size of the handle and delegates /// to baseclass safe handle. /// internal class BitmapSourceSafeMILHandle : SafeMILHandle { ////// Critical - initializes critical static field (autogenerated ctor) /// TreatAsSafe - sets them to the correct values, it's ok /// [SecurityCritical, SecurityTreatAsSafe] static BitmapSourceSafeMILHandle() { } ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal BitmapSourceSafeMILHandle() : base() { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: It is used to keep memory around /// [SecurityCritical] internal BitmapSourceSafeMILHandle(IntPtr handle) : base() { SetHandle(handle); } ////// Calculate the rough size for this handle /// ////// Critical - access unmanaged code /// TreatAsSafe - queries size of the bitmap, safe operation /// /// Attributes are required for UnsafeNativeMethods.* calls /// [SecurityCritical,SecurityTreatAsSafe] internal void CalculateSize() { UpdateEstimatedSize(ComputeEstimatedSize(handle)); } ////// Compute a rough estimate of the size in bytes for the image /// ////// Critical - access unmanaged code and takes an IntPtr /// [SecurityCritical] private static long ComputeEstimatedSize(IntPtr bitmapObject) { long estimatedSize = 0; if (bitmapObject != null && bitmapObject != IntPtr.Zero) { IntPtr wicBitmap; // // QueryInterface for the bitmap source to ensure we are // calling through the right vtable on the pinvoke. // int hr = UnsafeNativeMethods.MILUnknown.QueryInterface( bitmapObject, ref _uuidBitmap, out wicBitmap ); if (hr == HRESULT.S_OK) { Debug.Assert(wicBitmap != IntPtr.Zero); // The safe handle will release the ref added by the above QI SafeMILHandle bitmapSourceSafeHandle = new SafeMILHandle(wicBitmap, 0); uint pixelWidth = 0; uint pixelHeight = 0; hr = UnsafeNativeMethods.WICBitmapSource.GetSize( bitmapSourceSafeHandle, out pixelWidth, out pixelHeight); if (hr == HRESULT.S_OK) { Guid guidFormat; hr = UnsafeNativeMethods.WICBitmapSource.GetPixelFormat(bitmapSourceSafeHandle, out guidFormat); if (hr == HRESULT.S_OK) { // // Go to long space to avoid overflow and check for overlfow // PixelFormat pixelFormat = new PixelFormat(guidFormat); long scanlineSize = (long)pixelWidth * pixelFormat.InternalBitsPerPixel / 8; // // Check that scanlineSize is small enough that we can multiply by 2*pixelWidth // without an overflow. Since pixelHeight is a 32-bit value and we multiply by 2*pixelHeight, // then we can only have a 31-bit scanlineSize. Since we need a sign bit as well, // we need to check that scanlineSize can fit in 30 bits. // if (scanlineSize < 0x40000000) { // We often duplicate the image bits in a managed texture or elsewhere, so // estimate twice the natural size of the image. estimatedSize = 2*pixelHeight*scanlineSize; } } } } } return estimatedSize; } ////// Guid for IIDM_IMILBitmapSource /// ////// Critical - guid used for COM interop, need to be careful not to overwrite /// [SecurityCritical] private static Guid _uuidBitmap = MILGuidData.IID_IWICBitmap; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A sub-class of SafeMILHandle that can estimate size for bitmap // source objects. //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using MS.Internal; using MS.Win32; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media.Imaging { ////// Constructor which computes size of the handle and delegates /// to baseclass safe handle. /// internal class BitmapSourceSafeMILHandle : SafeMILHandle { ////// Critical - initializes critical static field (autogenerated ctor) /// TreatAsSafe - sets them to the correct values, it's ok /// [SecurityCritical, SecurityTreatAsSafe] static BitmapSourceSafeMILHandle() { } ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal BitmapSourceSafeMILHandle() : base() { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: It is used to keep memory around /// [SecurityCritical] internal BitmapSourceSafeMILHandle(IntPtr handle) : base() { SetHandle(handle); } ////// Calculate the rough size for this handle /// ////// Critical - access unmanaged code /// TreatAsSafe - queries size of the bitmap, safe operation /// /// Attributes are required for UnsafeNativeMethods.* calls /// [SecurityCritical,SecurityTreatAsSafe] internal void CalculateSize() { UpdateEstimatedSize(ComputeEstimatedSize(handle)); } ////// Compute a rough estimate of the size in bytes for the image /// ////// Critical - access unmanaged code and takes an IntPtr /// [SecurityCritical] private static long ComputeEstimatedSize(IntPtr bitmapObject) { long estimatedSize = 0; if (bitmapObject != null && bitmapObject != IntPtr.Zero) { IntPtr wicBitmap; // // QueryInterface for the bitmap source to ensure we are // calling through the right vtable on the pinvoke. // int hr = UnsafeNativeMethods.MILUnknown.QueryInterface( bitmapObject, ref _uuidBitmap, out wicBitmap ); if (hr == HRESULT.S_OK) { Debug.Assert(wicBitmap != IntPtr.Zero); // The safe handle will release the ref added by the above QI SafeMILHandle bitmapSourceSafeHandle = new SafeMILHandle(wicBitmap, 0); uint pixelWidth = 0; uint pixelHeight = 0; hr = UnsafeNativeMethods.WICBitmapSource.GetSize( bitmapSourceSafeHandle, out pixelWidth, out pixelHeight); if (hr == HRESULT.S_OK) { Guid guidFormat; hr = UnsafeNativeMethods.WICBitmapSource.GetPixelFormat(bitmapSourceSafeHandle, out guidFormat); if (hr == HRESULT.S_OK) { // // Go to long space to avoid overflow and check for overlfow // PixelFormat pixelFormat = new PixelFormat(guidFormat); long scanlineSize = (long)pixelWidth * pixelFormat.InternalBitsPerPixel / 8; // // Check that scanlineSize is small enough that we can multiply by 2*pixelWidth // without an overflow. Since pixelHeight is a 32-bit value and we multiply by 2*pixelHeight, // then we can only have a 31-bit scanlineSize. Since we need a sign bit as well, // we need to check that scanlineSize can fit in 30 bits. // if (scanlineSize < 0x40000000) { // We often duplicate the image bits in a managed texture or elsewhere, so // estimate twice the natural size of the image. estimatedSize = 2*pixelHeight*scanlineSize; } } } } } return estimatedSize; } ////// Guid for IIDM_IMILBitmapSource /// ////// Critical - guid used for COM interop, need to be careful not to overwrite /// [SecurityCritical] private static Guid _uuidBitmap = MILGuidData.IID_IWICBitmap; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
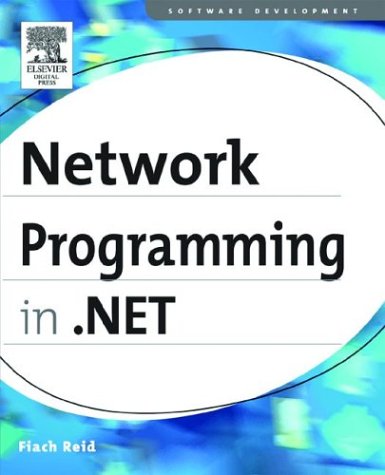
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontNameEditor.cs
- wmiprovider.cs
- HtmlTitle.cs
- BitmapVisualManager.cs
- SoapElementAttribute.cs
- CodePageEncoding.cs
- CodeIdentifier.cs
- HtmlInputImage.cs
- SpellerStatusTable.cs
- MenuEventArgs.cs
- DocumentSequenceHighlightLayer.cs
- ApplicationServicesHostFactory.cs
- XmlSerializationWriter.cs
- DataControlFieldTypeEditor.cs
- PixelFormats.cs
- GridEntry.cs
- ScriptRegistrationManager.cs
- SafeNativeMethods.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- XmlTextReader.cs
- Column.cs
- Brush.cs
- Random.cs
- DashStyles.cs
- SyntaxCheck.cs
- XmlDocumentSerializer.cs
- XDRSchema.cs
- SHA512CryptoServiceProvider.cs
- SmiTypedGetterSetter.cs
- CollectionViewGroupRoot.cs
- PopupRootAutomationPeer.cs
- HashAlgorithm.cs
- WebProxyScriptElement.cs
- ImageBrush.cs
- KnownBoxes.cs
- RuntimeConfig.cs
- SystemIPv4InterfaceProperties.cs
- BadImageFormatException.cs
- UidPropertyAttribute.cs
- XmlSchemaObjectCollection.cs
- AuthenticationService.cs
- AuthenticationService.cs
- ValidatorCompatibilityHelper.cs
- EntryWrittenEventArgs.cs
- WhileDesigner.cs
- NonSerializedAttribute.cs
- GridViewUpdatedEventArgs.cs
- FontCacheUtil.cs
- DefaultIfEmptyQueryOperator.cs
- DecimalConverter.cs
- XmlSchemaAnnotation.cs
- HashHelper.cs
- _emptywebproxy.cs
- SamlAssertionKeyIdentifierClause.cs
- ResXResourceSet.cs
- BasicKeyConstraint.cs
- TrackingProfile.cs
- DbConnectionClosed.cs
- DbParameterHelper.cs
- ModelTreeManager.cs
- DateTimeValueSerializerContext.cs
- XPathBinder.cs
- Pkcs7Signer.cs
- SafeRegistryHandle.cs
- DataSourceConverter.cs
- Mapping.cs
- TypeInfo.cs
- StringCollection.cs
- CompositeScriptReferenceEventArgs.cs
- SerializerWriterEventHandlers.cs
- MSG.cs
- RouteData.cs
- FrameworkReadOnlyPropertyMetadata.cs
- PageContentCollection.cs
- StylusPointCollection.cs
- XmlDictionaryWriter.cs
- HierarchicalDataBoundControl.cs
- SuppressIldasmAttribute.cs
- TextTreeFixupNode.cs
- InvariantComparer.cs
- ListViewCancelEventArgs.cs
- GroupBoxRenderer.cs
- NullNotAllowedCollection.cs
- XPathExpr.cs
- ReferentialConstraint.cs
- Authorization.cs
- SubclassTypeValidator.cs
- TokenBasedSet.cs
- AdornedElementPlaceholder.cs
- WebResourceUtil.cs
- Convert.cs
- Function.cs
- DES.cs
- BamlLocalizabilityResolver.cs
- DataGridTable.cs
- DictionaryGlobals.cs
- CodeValidator.cs
- InputLangChangeRequestEvent.cs
- DbConvert.cs
- UnsafeNativeMethods.cs