Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapVisualManager.cs / 1 / BitmapVisualManager.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: BitmapVisualManager.cs // //----------------------------------------------------------------------------- using System; using System.Windows; using System.Threading; using System.Windows.Threading; using System.Diagnostics; using System.Collections; using System.Runtime.InteropServices; using System.IO; using MS.Internal; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Win32.PresentationCore; namespace System.Windows.Media.Imaging { #region public class BitmapVisualManager ////// BitmapVisualManager holds state and context for a drawing a visual to an bitmap. /// internal class BitmapVisualManager : DispatcherObject { #region Constructors private BitmapVisualManager() { } ////// Create an BitmapVisualManager for drawing a visual to the bitmap. /// /// Where the resulting bitmap is rendered public BitmapVisualManager(RenderTargetBitmap bitmapTarget) { if (bitmapTarget == null) { throw new ArgumentNullException("bitmapTarget"); } if (bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen, null)); } _bitmapTarget = bitmapTarget; } #endregion #region Public methods ////// Render visual to printer. /// /// Root of the visual to render public void Render(Visual visual) { Render(visual, Matrix.Identity, Rect.Empty, false); } ////// Render visual to printer. /// /// Root of the visual to render /// World transform to apply to the root visual /// The window clip of the outermost window or Empty /// True if we are rendering the visual /// to apply an effect to it /// ////// Critical - Deals with bitmap handles /// TreatAsSafe - validates all parameters, uses safe wrappers /// [SecurityCritical,SecurityTreatAsSafe] internal void Render(Visual visual, Matrix worldTransform, Rect windowClip, bool fRenderForBitmapEffect) { if (visual == null) { throw new ArgumentNullException("visual"); } // If the bitmapTarget we're writing to is frozen then we can't proceed. Note that // it's possible for the BitmapVisualManager to be constructed with a mutable BitmapImage // and for the app to later freeze it. Such an application is misbehaving if // they subsequently try to render to the BitmapImage. if (_bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen)); } int sizeX = _bitmapTarget.PixelWidth; int sizeY = _bitmapTarget.PixelHeight; double dpiX = _bitmapTarget.DpiX; double dpiY = _bitmapTarget.DpiY; Debug.Assert ((sizeX > 0) && (sizeY > 0)); Debug.Assert ((dpiX > 0) && (dpiY > 0)); // validate the data if ((sizeX <= 0) || (sizeY <= 0)) { return; // nothing to draw } if ((dpiX <= 0) || (dpiY <= 0)) { dpiX = 96; dpiY = 96; } SafeMILHandle renderTargetBitmap = _bitmapTarget.MILRenderTarget; Debug.Assert (renderTargetBitmap != null, "Render Target is null"); IntPtr pIRenderTargetBitmap = IntPtr.Zero; try { // // Allocate a fresh synchronous channel. // MediaContext mctx = MediaContext.CurrentMediaContext; DUCE.Channel channel = mctx.AllocateSyncChannel(); // // Acquire the target bitmap. // Guid iidRTB = MILGuidData.IID_IMILRenderTargetBitmap; HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( renderTargetBitmap, ref iidRTB, out pIRenderTargetBitmap)); // // Render the visual on the synchronous channel. // Renderer.Render( pIRenderTargetBitmap, channel, visual, sizeX, sizeY, dpiX, dpiY, worldTransform, windowClip, fRenderForBitmapEffect); // // Release the synchronous channel. This way we can // re-use that channel later. // mctx.ReleaseSyncChannel(channel); } finally { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref pIRenderTargetBitmap); } _bitmapTarget.RenderTargetContentsChanged(); } #endregion #region Member Variables private RenderTargetBitmap _bitmapTarget = null; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: BitmapVisualManager.cs // //----------------------------------------------------------------------------- using System; using System.Windows; using System.Threading; using System.Windows.Threading; using System.Diagnostics; using System.Collections; using System.Runtime.InteropServices; using System.IO; using MS.Internal; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Win32.PresentationCore; namespace System.Windows.Media.Imaging { #region public class BitmapVisualManager ////// BitmapVisualManager holds state and context for a drawing a visual to an bitmap. /// internal class BitmapVisualManager : DispatcherObject { #region Constructors private BitmapVisualManager() { } ////// Create an BitmapVisualManager for drawing a visual to the bitmap. /// /// Where the resulting bitmap is rendered public BitmapVisualManager(RenderTargetBitmap bitmapTarget) { if (bitmapTarget == null) { throw new ArgumentNullException("bitmapTarget"); } if (bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen, null)); } _bitmapTarget = bitmapTarget; } #endregion #region Public methods ////// Render visual to printer. /// /// Root of the visual to render public void Render(Visual visual) { Render(visual, Matrix.Identity, Rect.Empty, false); } ////// Render visual to printer. /// /// Root of the visual to render /// World transform to apply to the root visual /// The window clip of the outermost window or Empty /// True if we are rendering the visual /// to apply an effect to it /// ////// Critical - Deals with bitmap handles /// TreatAsSafe - validates all parameters, uses safe wrappers /// [SecurityCritical,SecurityTreatAsSafe] internal void Render(Visual visual, Matrix worldTransform, Rect windowClip, bool fRenderForBitmapEffect) { if (visual == null) { throw new ArgumentNullException("visual"); } // If the bitmapTarget we're writing to is frozen then we can't proceed. Note that // it's possible for the BitmapVisualManager to be constructed with a mutable BitmapImage // and for the app to later freeze it. Such an application is misbehaving if // they subsequently try to render to the BitmapImage. if (_bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen)); } int sizeX = _bitmapTarget.PixelWidth; int sizeY = _bitmapTarget.PixelHeight; double dpiX = _bitmapTarget.DpiX; double dpiY = _bitmapTarget.DpiY; Debug.Assert ((sizeX > 0) && (sizeY > 0)); Debug.Assert ((dpiX > 0) && (dpiY > 0)); // validate the data if ((sizeX <= 0) || (sizeY <= 0)) { return; // nothing to draw } if ((dpiX <= 0) || (dpiY <= 0)) { dpiX = 96; dpiY = 96; } SafeMILHandle renderTargetBitmap = _bitmapTarget.MILRenderTarget; Debug.Assert (renderTargetBitmap != null, "Render Target is null"); IntPtr pIRenderTargetBitmap = IntPtr.Zero; try { // // Allocate a fresh synchronous channel. // MediaContext mctx = MediaContext.CurrentMediaContext; DUCE.Channel channel = mctx.AllocateSyncChannel(); // // Acquire the target bitmap. // Guid iidRTB = MILGuidData.IID_IMILRenderTargetBitmap; HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( renderTargetBitmap, ref iidRTB, out pIRenderTargetBitmap)); // // Render the visual on the synchronous channel. // Renderer.Render( pIRenderTargetBitmap, channel, visual, sizeX, sizeY, dpiX, dpiY, worldTransform, windowClip, fRenderForBitmapEffect); // // Release the synchronous channel. This way we can // re-use that channel later. // mctx.ReleaseSyncChannel(channel); } finally { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref pIRenderTargetBitmap); } _bitmapTarget.RenderTargetContentsChanged(); } #endregion #region Member Variables private RenderTargetBitmap _bitmapTarget = null; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
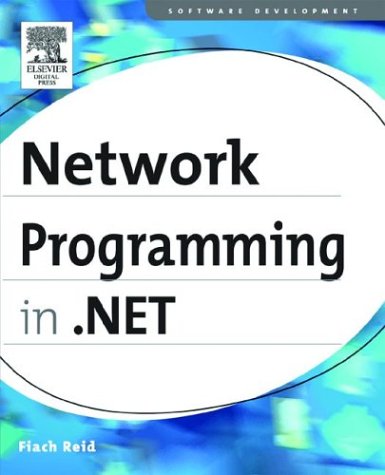
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignOnlyAttribute.cs
- SpeakInfo.cs
- StylusPointProperty.cs
- SrgsNameValueTag.cs
- ListViewItemMouseHoverEvent.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- CounterCreationDataCollection.cs
- sitestring.cs
- NativeMethods.cs
- ParserExtension.cs
- MonitorWrapper.cs
- GZipDecoder.cs
- TheQuery.cs
- ChildChangedEventArgs.cs
- StopStoryboard.cs
- EntityContainer.cs
- TextEndOfLine.cs
- XXXInfos.cs
- RectAnimationBase.cs
- MdImport.cs
- DataServicePagingProviderWrapper.cs
- MappingSource.cs
- AssemblyHash.cs
- ToolStripContainer.cs
- thaishape.cs
- SemanticResolver.cs
- CompoundFileDeflateTransform.cs
- UInt32.cs
- ResXFileRef.cs
- WebEventCodes.cs
- AsyncResult.cs
- Cast.cs
- SiteMapSection.cs
- DataTemplate.cs
- PropertyItemInternal.cs
- AppSettings.cs
- BitmapEffectGeneralTransform.cs
- ConnectionConsumerAttribute.cs
- MessageContractAttribute.cs
- WindowsListView.cs
- TypedServiceChannelBuilder.cs
- SelectionListDesigner.cs
- ShellProvider.cs
- PersonalizationStateQuery.cs
- ToolStripOverflowButton.cs
- DefaultPropertyAttribute.cs
- DocumentOrderQuery.cs
- ObjectDataSourceStatusEventArgs.cs
- Point.cs
- SplineKeyFrames.cs
- DataControlButton.cs
- ReliableChannelFactory.cs
- HelpPage.cs
- ConfigurationStrings.cs
- ToolStripStatusLabel.cs
- QuaternionRotation3D.cs
- dbdatarecord.cs
- DeleteHelper.cs
- XmlReaderSettings.cs
- XmlLanguageConverter.cs
- HttpRequestWrapper.cs
- UpdateException.cs
- DecoderBestFitFallback.cs
- InternalPermissions.cs
- SqlMethodCallConverter.cs
- SchemaImporter.cs
- HttpCacheVaryByContentEncodings.cs
- ModelPropertyCollectionImpl.cs
- FileEnumerator.cs
- CompositeControl.cs
- BuilderPropertyEntry.cs
- Set.cs
- MailMessageEventArgs.cs
- DataPointer.cs
- Italic.cs
- HttpCapabilitiesBase.cs
- FixedElement.cs
- Path.cs
- SQlBooleanStorage.cs
- TextOptions.cs
- ModelVisual3D.cs
- AuditLog.cs
- DesignerHelpers.cs
- HierarchicalDataTemplate.cs
- DesignerCommandSet.cs
- ComplusEndpointConfigContainer.cs
- SamlSerializer.cs
- ListControlActionList.cs
- DynamicQueryStringParameter.cs
- XmlIlGenerator.cs
- ConnectionStringsSection.cs
- ReachSerializer.cs
- ThrowHelper.cs
- SkipStoryboardToFill.cs
- Function.cs
- ToggleButtonAutomationPeer.cs
- ErasingStroke.cs
- safesecurityhelperavalon.cs
- ZipIOBlockManager.cs
- ParameterCollection.cs