Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / SizeChangedEventArgs.cs / 1 / SizeChangedEventArgs.cs
using System; namespace System.Windows { ////// The SizeChangedEventArgs class is used by SizeChangedEventHandler. /// This handler is used for ComputedWidthChanged and ComputedHeightChanged events /// on UIElement. /// public class SizeChangedEventArgs : RoutedEventArgs { ////// Initializes a new instance of the SizeChangedEventArgs class. /// /// /// The UIElement which has its size changed by layout engine/>. /// /// /// The SizeChangeInfo that is used by. /// internal SizeChangedEventArgs(UIElement element, SizeChangedInfo info) { if (info == null) { throw new ArgumentNullException("info"); } if (element == null) { throw new ArgumentNullException("element"); } _element = element; _previousSize = info.PreviousSize; if(info.WidthChanged) _bits |= _widthChangedBit; if(info.HeightChanged) _bits |= _heightChangedBit; } /// /// Read-only access to the previous Size /// public Size PreviousSize { get { return _previousSize; } } ////// Read-only access to the new Size /// public Size NewSize { get { return _element.RenderSize; } } ////// Read-only access to the flag indicating that Width component of the size changed. /// Note that due to double math /// effects, the it may be (previousSize.Width != newSize.Width) and widthChanged = true. /// This may happen in layout when sizes of objects are fluctuating because of a precision "jitter" of /// the input parameters, but the overall scene is considered to be "the same" so no visible changes /// will be detected. Typically, the handler of SizeChangedEvent should check this bit to avoid /// invalidation of layout if the dimension didn't change. /// public bool WidthChanged { get { return ((_bits & _widthChangedBit) != 0); } } ////// Read-only access to the flag indicating that Height component of the size changed. /// Note that due to double math /// effects, the it may be (previousSize.Height != newSize.Height) and heightChanged = true. /// This may happen in layout when sizes of objects are fluctuating because of a precision "jitter" of /// the input parameters, but the overall scene is considered to be "the same" so no visible changes /// will be detected. Typically, the handler of SizeChangedEvent should check this bit to avoid /// invalidation of layout if the dimension didn't change. /// public bool HeightChanged { get { return ((_bits & _heightChangedBit) != 0); } } private Size _previousSize; private UIElement _element; private byte _bits; private static byte _widthChangedBit = 0x1; private static byte _heightChangedBit = 0x2; ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// ///protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { SizeChangedEventHandler handler = (SizeChangedEventHandler) genericHandler; handler(genericTarget, this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows { /// /// The SizeChangedEventArgs class is used by SizeChangedEventHandler. /// This handler is used for ComputedWidthChanged and ComputedHeightChanged events /// on UIElement. /// public class SizeChangedEventArgs : RoutedEventArgs { ////// Initializes a new instance of the SizeChangedEventArgs class. /// /// /// The UIElement which has its size changed by layout engine/>. /// /// /// The SizeChangeInfo that is used by. /// internal SizeChangedEventArgs(UIElement element, SizeChangedInfo info) { if (info == null) { throw new ArgumentNullException("info"); } if (element == null) { throw new ArgumentNullException("element"); } _element = element; _previousSize = info.PreviousSize; if(info.WidthChanged) _bits |= _widthChangedBit; if(info.HeightChanged) _bits |= _heightChangedBit; } /// /// Read-only access to the previous Size /// public Size PreviousSize { get { return _previousSize; } } ////// Read-only access to the new Size /// public Size NewSize { get { return _element.RenderSize; } } ////// Read-only access to the flag indicating that Width component of the size changed. /// Note that due to double math /// effects, the it may be (previousSize.Width != newSize.Width) and widthChanged = true. /// This may happen in layout when sizes of objects are fluctuating because of a precision "jitter" of /// the input parameters, but the overall scene is considered to be "the same" so no visible changes /// will be detected. Typically, the handler of SizeChangedEvent should check this bit to avoid /// invalidation of layout if the dimension didn't change. /// public bool WidthChanged { get { return ((_bits & _widthChangedBit) != 0); } } ////// Read-only access to the flag indicating that Height component of the size changed. /// Note that due to double math /// effects, the it may be (previousSize.Height != newSize.Height) and heightChanged = true. /// This may happen in layout when sizes of objects are fluctuating because of a precision "jitter" of /// the input parameters, but the overall scene is considered to be "the same" so no visible changes /// will be detected. Typically, the handler of SizeChangedEvent should check this bit to avoid /// invalidation of layout if the dimension didn't change. /// public bool HeightChanged { get { return ((_bits & _heightChangedBit) != 0); } } private Size _previousSize; private UIElement _element; private byte _bits; private static byte _widthChangedBit = 0x1; private static byte _heightChangedBit = 0x2; ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// ///protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { SizeChangedEventHandler handler = (SizeChangedEventHandler) genericHandler; handler(genericTarget, this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
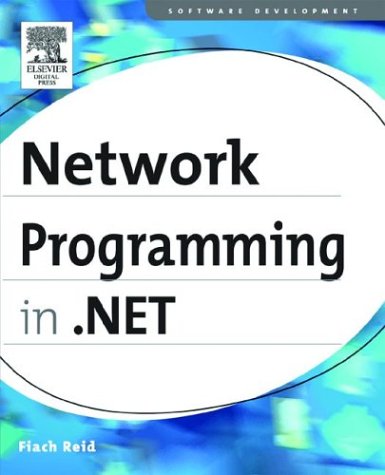
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMAC.cs
- RsaKeyIdentifierClause.cs
- SimpleType.cs
- XPathPatternParser.cs
- ValidationErrorEventArgs.cs
- DataSourceCacheDurationConverter.cs
- XmlReflectionImporter.cs
- WorkflowPrinting.cs
- LinearKeyFrames.cs
- FeatureSupport.cs
- XmlElementAttributes.cs
- ConfigurationException.cs
- CustomSignedXml.cs
- HitTestWithPointDrawingContextWalker.cs
- DataColumnPropertyDescriptor.cs
- Process.cs
- Ticks.cs
- SqlXml.cs
- EndOfStreamException.cs
- InputLanguage.cs
- XPathNodeInfoAtom.cs
- BitmapVisualManager.cs
- DeviceSpecificDesigner.cs
- ContainerParaClient.cs
- _UncName.cs
- RecordsAffectedEventArgs.cs
- BitStack.cs
- Timer.cs
- WebSysDefaultValueAttribute.cs
- MailMessage.cs
- RectangleF.cs
- JapaneseCalendar.cs
- LinkLabelLinkClickedEvent.cs
- BindValidator.cs
- UnsafeNativeMethods.cs
- HttpInputStream.cs
- Panel.cs
- PathFigure.cs
- CornerRadiusConverter.cs
- ProjectionPathSegment.cs
- XmlDictionaryReaderQuotas.cs
- UxThemeWrapper.cs
- Adorner.cs
- SplitterEvent.cs
- CapabilitiesState.cs
- InvalidContentTypeException.cs
- SqlDataSourceParameterParser.cs
- BooleanFunctions.cs
- DoubleAnimationUsingKeyFrames.cs
- DataRowView.cs
- OracleMonthSpan.cs
- XmlArrayItemAttributes.cs
- shaperfactoryquerycachekey.cs
- GiveFeedbackEventArgs.cs
- ConstantCheck.cs
- Emitter.cs
- ModuleElement.cs
- XmlChildNodes.cs
- formatstringdialog.cs
- Point3DAnimation.cs
- XmlElementAttribute.cs
- PagesChangedEventArgs.cs
- TransactionFilter.cs
- ProxyManager.cs
- CategoryNameCollection.cs
- AmbientEnvironment.cs
- EmbeddedMailObject.cs
- DataGridDesigner.cs
- UIElementAutomationPeer.cs
- StrokeNodeEnumerator.cs
- LockRecursionException.cs
- ProcessHostMapPath.cs
- RuntimeVariableList.cs
- PointAnimation.cs
- ThicknessKeyFrameCollection.cs
- DropTarget.cs
- CustomAttributeFormatException.cs
- XmlArrayAttribute.cs
- ContentPlaceHolder.cs
- ChangeToolStripParentVerb.cs
- Timeline.cs
- CoTaskMemHandle.cs
- ToolStripOverflowButton.cs
- SchemaExporter.cs
- DependencyObject.cs
- EmptyEnumerator.cs
- TraceLog.cs
- XPathParser.cs
- DataGridViewUtilities.cs
- BindingListCollectionView.cs
- CanExecuteRoutedEventArgs.cs
- OleDbErrorCollection.cs
- Formatter.cs
- GeometryCollection.cs
- TransactionScope.cs
- EntityCommandCompilationException.cs
- XmlAttribute.cs
- ToolBarOverflowPanel.cs
- DebugView.cs
- ConsoleCancelEventArgs.cs