Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / xamlnodes.cs / 1 / xamlnodes.cs
/****************************************************************************\ * * File: XamlNodes.cs * * Purpose: Xaml Node definition class. Contains the different nodes * That can be returned by the XamlReader * * History: * 11/06/02: rogerg Created * 5/27/03: [....] Ported to wcp * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; #if !PBTCOMPILER using System.Windows; using System.Windows.Threading; #endif using MS.Utility; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { #region XamlNodeTypeDefitions ////// Identifier for XamlNodes /// internal enum XamlNodeType { ////// Unknown Node /// Unknown, ////// Start Document Node /// DocumentStart, ////// End Document Node /// DocumentEnd, ////// Start Element Node, which may be a CLR object or a DependencyObject /// ElementStart, ////// End Element Node /// ElementEnd, ////// Property Node, which may be a CLR property or a DependencyProperty /// Property, ////// Complex Property Node /// PropertyComplexStart, ////// End Complex Property Node /// PropertyComplexEnd, ////// Start Array Property Node /// PropertyArrayStart, ////// End Array Property Node /// PropertyArrayEnd, ////// Star IList Property Node /// PropertyIListStart, ////// End IListProperty Node /// PropertyIListEnd, ////// Start IDictionary Property Node /// PropertyIDictionaryStart, ////// End IDictionary Property Node /// PropertyIDictionaryEnd, ////// A property whose value is a simple MarkupExtension object /// PropertyWithExtension, ////// A property whose value is a Type object /// PropertyWithType, ////// LiteralContent Node /// LiteralContent, ////// Text Node /// Text, ////// RoutedEventNode /// RoutedEvent, ////// ClrEvent Node /// ClrEvent, ////// XmlnsProperty Node /// XmlnsProperty, ////// XmlAttribute Node /// XmlAttribute, ////// Processing Intstruction Node /// ProcessingInstruction, ////// Comment Node /// Comment, ////// DefTag Node /// DefTag, ////// DefAttribute Node /// DefAttribute, ////// PresentationOptionsAttribute Node /// PresentationOptionsAttribute, ////// x:Key attribute that is resolved to a Type /// DefKeyTypeAttribute, ////// EndAttributes Node /// EndAttributes, ////// PI xml - clr namespace mapping /// PIMapping, ////// Unknown tag /// UnknownTagStart, ////// Unknown tag /// UnknownTagEnd, ////// Unknown attribute /// UnknownAttribute, ////// Start of an element tree used /// to identify a key in an IDictionary. /// KeyElementStart, ////// End of an element tree used /// to identify a key in an IDictionary. /// KeyElementEnd, ////// Start of a section that contains one or more constructor parameters /// ConstructorParametersStart, ////// Start of a section that contains one or more constructor parameters /// ConstructorParametersEnd, ////// Constructor parameter that has been resolved to a Type at compile time. /// ConstructorParameterType, ////// Node to set the content property /// ContentProperty, } ////// Base Node in which all others derive /// internal class XamlNode { ////// Constructor /// internal XamlNode( XamlNodeType tokenType, int lineNumber, int linePosition, int depth) { _token = tokenType; _lineNumber = lineNumber; _linePosition = linePosition; _depth = depth; } ////// Token Type of the Node /// internal XamlNodeType TokenType { get { return _token; } } ////// LineNumber of Node in File /// internal int LineNumber { get { return _lineNumber; } } ////// LinePosition of Node in File /// internal int LinePosition { get { return _linePosition; } } ////// Depth of the Node /// internal int Depth { get { return _depth; } } ////// An array of xamlnodes that represent the start of scoped portions of the xaml file /// internal static XamlNodeType[] ScopeStartTokens = new XamlNodeType[]{ XamlNodeType.DocumentStart, XamlNodeType.ElementStart, XamlNodeType.PropertyComplexStart, XamlNodeType.PropertyArrayStart, XamlNodeType.PropertyIListStart, XamlNodeType.PropertyIDictionaryStart, }; ////// An array of xamlnodes that represent the end of scoped portions of the xaml file /// internal static XamlNodeType[] ScopeEndTokens = new XamlNodeType[]{ XamlNodeType.DocumentEnd, XamlNodeType.ElementEnd, XamlNodeType.PropertyComplexEnd, XamlNodeType.PropertyArrayEnd, XamlNodeType.PropertyIListEnd, XamlNodeType.PropertyIDictionaryEnd, }; XamlNodeType _token; int _lineNumber; int _linePosition; int _depth; } ////// XamlDocument start node /// internal class XamlDocumentStartNode : XamlNode { ////// Constructor /// internal XamlDocumentStartNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.DocumentStart,lineNumber,linePosition,depth) { } } ////// XamlDocument end node /// internal class XamlDocumentEndNode : XamlNode { ////// Constructor /// ///internal XamlDocumentEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.DocumentEnd,lineNumber,linePosition,depth) { } } /// /// Xaml Text Node /// [DebuggerDisplay("Text:{_text}")] internal class XamlTextNode : XamlNode { ////// Constructor /// internal XamlTextNode( int lineNumber, int linePosition, int depth, string textContent, Type converterType) : base (XamlNodeType.Text,lineNumber,linePosition,depth) { _text = textContent; _converterType = converterType; } ////// Text for the TextNode /// internal string Text { get { return _text; } } ////// Type of Converter to be used to convert this text value into an object /// internal Type ConverterType { get { return _converterType; } } ////// internal function so Tokenizer can just update a text /// node after whitespace processing /// internal void UpdateText(string text) { _text = text; } string _text; Type _converterType = null; } ////// Base class for XamlPropertyNode and XamlPropertyComplexStartNode /// [DebuggerDisplay("Prop:{_typeFullName}.{_propName}")] internal class XamlPropertyBaseNode : XamlNode { ////// Constructor /// internal XamlPropertyBaseNode( XamlNodeType token, int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty or MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (token, lineNumber, linePosition, depth) { if (typeFullName == null) { throw new ArgumentNullException("typeFullName"); } if (propertyName == null) { throw new ArgumentNullException("propertyName"); } _propertyMember = propertyMember; _assemblyName = assemblyName; _typeFullName = typeFullName; _propName = propertyName; } #if PBTCOMPILER ////// PropertyInfo for the property for the Node. This may be null. /// internal PropertyInfo PropInfo { get { return _propertyMember as PropertyInfo;} } #endif ////// Assembly of the type that owns or has declared the Property /// internal string AssemblyName { get { return _assemblyName; } } ////// TypeFullName of type that owns or has declared the Property /// internal string TypeFullName { get { return _typeFullName; } } ////// Name of the Property /// internal string PropName { get { return _propName;} } ////// Type of the owner or declarer of this Property /// internal Type PropDeclaringType { get { // Lazy initialize this to avoid addition reflection if it // is not needed. if (_declaringType == null && _propertyMember != null) { _declaringType = XamlTypeMapper.GetDeclaringType(_propertyMember); } return _declaringType; } } ////// Valid Type of the Property /// internal Type PropValidType { get { // Lazy initialize this to avoid addition reflection if it // is not needed. if (_validType == null) { _validType = XamlTypeMapper.GetPropertyType(_propertyMember); } return _validType; } } ////// Property methodinfo or propertyinfo /// internal object PropertyMember { get { return _propertyMember; } } object _propertyMember; string _assemblyName; string _typeFullName; string _propName; Type _validType; Type _declaringType; } ////// Xaml Complex Property Node, which is a property of the /// form / internal class XamlPropertyComplexStartNode : XamlPropertyBaseNode { ///. This can pertain to any type of object. /// /// Constructor /// internal XamlPropertyComplexStartNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty or MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (XamlNodeType.PropertyComplexStart, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { } ////// Internal Constructor /// internal XamlPropertyComplexStartNode( XamlNodeType token, int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty or MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (token, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { } } ////// Xaml Complex Property Node, which is a property of the /// form / internal class XamlPropertyComplexEndNode : XamlNode { ///. This can pertain to any type of object. /// /// Contstructor /// internal XamlPropertyComplexEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.PropertyComplexEnd,lineNumber,linePosition,depth) { } ////// Contstructor /// internal XamlPropertyComplexEndNode( XamlNodeType token, int lineNumber, int linePosition, int depth) : base (token,lineNumber,linePosition,depth) { } } ////// Xaml Property Node, whose value is a simple MarkupExtension. /// internal class XamlPropertyWithExtensionNode : XamlPropertyBaseNode { internal XamlPropertyWithExtensionNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName, string value, short extensionTypeId, bool isValueNestedExtension, bool isValueTypeExtension) : base(XamlNodeType.PropertyWithExtension, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { _value = value; _extensionTypeId = extensionTypeId; _isValueNestedExtension = isValueNestedExtension; _isValueTypeExtension = isValueTypeExtension; _defaultTargetType = null; } internal short ExtensionTypeId { get { return _extensionTypeId; } } internal string Value { get { return _value; } } internal bool IsValueNestedExtension { get { return _isValueNestedExtension; } } internal bool IsValueTypeExtension { get { return _isValueTypeExtension; } } internal Type DefaultTargetType { get { return _defaultTargetType; } set { _defaultTargetType = value; } } short _extensionTypeId; string _value; bool _isValueNestedExtension; bool _isValueTypeExtension; Type _defaultTargetType; } ////// Xaml Property Node, which can be a DependencyProperty, CLR property or /// hold a reference to a static property set method. /// internal class XamlPropertyNode : XamlPropertyBaseNode { ////// Constructor /// internal XamlPropertyNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName, string value, BamlAttributeUsage attributeUsage, bool complexAsSimple) : base (XamlNodeType.Property, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { _value = value; _attributeUsage = attributeUsage; _complexAsSimple = complexAsSimple; } internal XamlPropertyNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName, string value, BamlAttributeUsage attributeUsage, bool complexAsSimple, bool isDefinitionName) : this(lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName, value, attributeUsage, complexAsSimple) { _isDefinitionName = isDefinitionName; } #if PBTCOMPILER internal bool IsDefinitionName { get { return _isDefinitionName; } } #endif ////// Value for the attribute /// internal string Value { get { return _value;} } ////// Change the value stored in the xaml node. For internal use only. /// internal void SetValue(string value) { _value = value; } ////// Return the declaring type to use when resolving the type converter /// or serializer for this property value. /// internal Type ValueDeclaringType { get { if (_valueDeclaringType == null) { return PropDeclaringType; } else { return _valueDeclaringType; } } set { _valueDeclaringType = value; } } ////// Return the property name to use when resolving the type converter /// or serializer for this property value. This may be different than /// the actual property name for this property /// internal string ValuePropertyName { get { if (_valuePropertyName == null) { return PropName; } else { return _valuePropertyName; } } set { _valuePropertyName = value; } } ////// Return the property type to use when resolving the type converter /// or serializer for this property value. This may be different than /// the actual property type for this property. /// internal Type ValuePropertyType { get { if (_valuePropertyType == null) { return PropValidType; } else { return _valuePropertyType; } } set { _valuePropertyType = value; } } ////// Return the property member info to use when resolving the type converter /// or serializer for this property value. This may be different than /// the actual property member info for this property. /// internal object ValuePropertyMember { get { if (_valuePropertyMember == null) { return PropertyMember; } else { return _valuePropertyMember; } } set { _valuePropertyMember = value; } } // Indicates if the valueId been explcitly set internal bool HasValueId { get { return _hasValueId; } } // This is either a known dependency property Id or a // TypeId of the resolved owner type of a DP that is the // value of this property node. internal short ValueId { get { return _valueId; } set { _valueId = value; _hasValueId = true; } } // If ValueId is a TypeId, this stores the name of the DP. internal string MemberName { get { return _memberName; } set { _memberName = value; } } // The type to resolve the DP value against if Onwer is not explicitly specified. internal Type DefaultTargetType { get { return _defaultTargetType; } set { _defaultTargetType = value; } } ////// Gives the specific usage of this property /// ////// Some properties are not only set on an element, but have some other effects /// such as setting the xml:lang or xml:space values in the parser context. /// The AttributeUsage describes addition effects or usage for this property. /// internal BamlAttributeUsage AttributeUsage { get { return _attributeUsage; } } ////// A XamlPropertyNode is created from a property=value assignment, /// or it may be created from a complex subtree which contains text, but is /// represented as a simple property. In the latter case, ComplexAsSimple /// should be set to True. /// internal bool ComplexAsSimple { get { return _complexAsSimple; } } string _value; BamlAttributeUsage _attributeUsage; bool _complexAsSimple; bool _isDefinitionName; // Variables for holding property info when this property's value is // resolved using the attributes of another property. Type _valueDeclaringType; string _valuePropertyName; Type _valuePropertyType; object _valuePropertyMember; bool _hasValueId = false; short _valueId = 0; string _memberName = null; Type _defaultTargetType; } ////// Xaml Property Node, which can be a DependencyProperty, CLR property or /// hold a reference to a static property set method. /// internal class XamlPropertyWithTypeNode : XamlPropertyBaseNode { ////// Constructor /// internal XamlPropertyWithTypeNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName, string valueTypeFullName, // String value of type, in the form Namespace.Typename string valueAssemblyName, // Assembly name where type value is defined. Type valueElementType, // Actual type of the valueTypeFullname. string valueSerializerTypeFullName, string valueSerializerTypeAssemblyName) : base (XamlNodeType.PropertyWithType, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { _valueTypeFullname = valueTypeFullName; _valueTypeAssemblyName = valueAssemblyName; _valueElementType = valueElementType; _valueSerializerTypeFullName = valueSerializerTypeFullName; _valueSerializerTypeAssemblyName = valueSerializerTypeAssemblyName; } ////// Value for the property's value, which should resolve to a Type. /// This is in the form Namespace.LocalTypeName /// internal string ValueTypeFullName { get { return _valueTypeFullname;} } ////// Name of the assembly where the resolved value's type is declared. /// internal string ValueTypeAssemblyName { get { return _valueTypeAssemblyName;} } ////// Cached value of the type of the value that is resolved at compile time /// internal Type ValueElementType { get { return _valueElementType;} } ////// Name of the serializer to use when parsing an object that is of the /// type of ValueElementType /// internal string ValueSerializerTypeFullName { get { return _valueSerializerTypeFullName;} } ////// Name of the assembly where the serializer to use when parsing an object /// that is of the type of ValueElementType is declared. /// internal string ValueSerializerTypeAssemblyName { get { return _valueSerializerTypeAssemblyName;} } string _valueTypeFullname; string _valueTypeAssemblyName; Type _valueElementType; string _valueSerializerTypeFullName; string _valueSerializerTypeAssemblyName; } ////// Start of an Unknown attribute in Xaml. This may be handled by /// a custom Serializer /// internal class XamlUnknownAttributeNode : XamlAttributeNode { ////// Constructor /// internal XamlUnknownAttributeNode( int lineNumber, int linePosition, int depth, string xmlNamespace, string name, string value, BamlAttributeUsage attributeUsage) : base(XamlNodeType.UnknownAttribute,lineNumber,linePosition, depth,value) { _xmlNamespace = xmlNamespace; _name = name; _attributeUsage = attributeUsage; } ////// XmlNamespace associated with the unknown attribute /// internal string XmlNamespace { get { return _xmlNamespace;} } ////// Name of the unknown property /// internal string Name { get { return _name;} } #if PBTCOMPILER ////// Gives the specific usage of this property /// ////// Some properties are not only set on an element, but have some other effects /// such as setting the xml:lang or xml:space values in the parser context. /// The AttributeUsage describes addition effects or usage for this property. /// internal BamlAttributeUsage AttributeUsage { get { return _attributeUsage; } } ////// Full TypeName of the unknown owner of this property /// internal string OwnerTypeFullName { get { return _ownerTypeFullName; } set { _ownerTypeFullName = value; } } string _ownerTypeFullName; #endif string _xmlNamespace; string _name; BamlAttributeUsage _attributeUsage; } ////// Xaml start element Node, which is any type of object /// [DebuggerDisplay("Elem:{_typeFullName}")] internal class XamlElementStartNode : XamlNode { ////// Constructor /// internal XamlElementStartNode( int lineNumber, int linePosition, int depth, string assemblyName, string typeFullName, Type elementType, Type serializerType) : this (XamlNodeType.ElementStart, lineNumber, linePosition, depth, assemblyName, typeFullName, elementType, serializerType, false /*isEmptyElement*/, false /*needsDictionaryKey*/, false /*isInjected*/) { } internal XamlElementStartNode( XamlNodeType tokenType, int lineNumber, int linePosition, int depth, string assemblyName, string typeFullName, Type elementType, Type serializerType, bool isEmptyElement, bool needsDictionaryKey, bool isInjected) : base (tokenType,lineNumber,linePosition,depth) { _assemblyName = assemblyName; _typeFullName = typeFullName; _elementType = elementType; _serializerType = serializerType; _isEmptyElement = isEmptyElement; _needsDictionaryKey = needsDictionaryKey; _useTypeConverter = false; IsInjected = isInjected; } ////// Assembly Object is in /// internal string AssemblyName { get { return _assemblyName; } } ////// TypeFullName of the Object /// internal string TypeFullName { get { return _typeFullName; } } ////// Type for the Object /// internal Type ElementType { get { return _elementType; } } ////// Type of serializer to use when serializing or deserializing /// the element. The serializer can be used to override the default /// deserialization action taken by the Avalon parser. /// If there is no custom serializer, then this is null. /// internal Type SerializerType { get { return _serializerType; } } ////// Type full name of serializer to use when serializing or deserializing /// the element. The serializer can be used to override the default /// deserialization action taken by the Avalon parser. /// If there is no custom serializer, then this is an empty string. /// internal string SerializerTypeFullName { get { return _serializerType == null ? string.Empty : _serializerType.FullName; } } ////// Whether we plan on creating an instance of this element via a /// TypeConverter using a Text node that follows. /// internal bool CreateUsingTypeConverter { get { return _useTypeConverter; } set { _useTypeConverter = value; } } #if PBTCOMPILER // True if this element is the top level element in a dictionary, which // means that we have to have a key associated with it. internal bool NeedsDictionaryKey { get { return _needsDictionaryKey; } } #endif internal bool IsInjected { get { return _isInjected; } set { _isInjected = value; } } string _assemblyName; string _typeFullName; Type _elementType; Type _serializerType; bool _isEmptyElement; bool _needsDictionaryKey; bool _useTypeConverter; bool _isInjected; } ////// Start of a constructor parameters section /// internal class XamlConstructorParametersStartNode : XamlNode { ////// Constructor /// internal XamlConstructorParametersStartNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.ConstructorParametersStart,lineNumber,linePosition,depth) { } } ////// End of a constructor parameters section /// internal class XamlConstructorParametersEndNode : XamlNode { ////// Constructor /// internal XamlConstructorParametersEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.ConstructorParametersEnd,lineNumber,linePosition,depth) { } } #if PBTCOMPILER ////// Constructor parameter that is a Type object /// internal class XamlConstructorParameterTypeNode : XamlNode { ////// Constructor /// internal XamlConstructorParameterTypeNode( int lineNumber, int linePosition, int depth, string valueTypeFullName, // String value of type, in the form Namespace.Typename string valueAssemblyName, // Assembly name where type value is defined. Type valueElementType) // Actual type of the valueTypeFullname. : base (XamlNodeType.ConstructorParameterType,lineNumber,linePosition,depth) { _valueTypeFullname = valueTypeFullName; _valueTypeAssemblyName = valueAssemblyName; _valueElementType = valueElementType; } ////// Value for the parameter, which should resolve to a Type. /// This is in the form Namespace.LocalTypeName /// internal string ValueTypeFullName { get { return _valueTypeFullname;} } ////// Name of the assembly where the resolved value's type is declared. /// internal string ValueTypeAssemblyName { get { return _valueTypeAssemblyName;} } ////// Cached value of the type of the value that is resolved at compile time /// internal Type ValueElementType { get { return _valueElementType;} } string _valueTypeFullname; string _valueTypeAssemblyName; Type _valueElementType; } #endif ////// Xaml End Element Node /// internal class XamlElementEndNode : XamlNode { ////// Constructor /// internal XamlElementEndNode( int lineNumber, int linePosition, int depth) : this (XamlNodeType.ElementEnd,lineNumber,linePosition,depth) { } ////// Constructor /// internal XamlElementEndNode( XamlNodeType tokenType, int lineNumber, int linePosition, int depth) : base (tokenType,lineNumber,linePosition,depth) { } } ////// XamlLiteralContentNode /// [DebuggerDisplay("Cont:{_content}")] internal class XamlLiteralContentNode : XamlNode { ////// Constructor /// internal XamlLiteralContentNode( int lineNumber, int linePosition, int depth, string content) : base (XamlNodeType.LiteralContent,lineNumber,linePosition,depth) { _content = content; } ////// The Literal Content /// internal string Content { get { return _content; } } string _content; } ////// XamlAttributeNode /// [DebuggerDisplay("Attr:{_value}")] internal class XamlAttributeNode : XamlNode { ////// Constructor /// internal XamlAttributeNode( XamlNodeType tokenType, int lineNumber, int linePosition, int depth, string value) : base(tokenType,lineNumber,linePosition,depth) { _value = value; } ////// Value for the attribute /// internal string Value { get { return _value;} } string _value; } ////// Start of an Unknown element tag in Xaml. This may be handled by /// a custom Serializer /// internal class XamlUnknownTagStartNode : XamlAttributeNode { ////// Constructor /// internal XamlUnknownTagStartNode( int lineNumber, int linePosition, int depth, string xmlNamespace, string value) : base(XamlNodeType.UnknownTagStart,lineNumber,linePosition,depth, value) { _xmlNamespace = xmlNamespace; } ////// XmlNamespace associated with the unknown tag /// internal string XmlNamespace { get { return _xmlNamespace;} } string _xmlNamespace; } ////// End of an Unknown element tag in Xaml. This may be handled by /// a custom Serializer /// internal class XamlUnknownTagEndNode : XamlNode { ////// Constructor /// internal XamlUnknownTagEndNode( int lineNumber, int linePosition, int depth, string localName, string xmlNamespace) : base (XamlNodeType.UnknownTagEnd,lineNumber,linePosition,depth) { _localName = localName; _xmlNamespace = xmlNamespace; } #if PBTCOMPILER ////// LocalName associated with the unknown tag /// internal string LocalName { get { return _localName; } } ////// Xml Namespace associated with the unknown tag /// internal string XmlNamespace { get { return _xmlNamespace; } } #endif // PBTCOMPILER string _localName; string _xmlNamespace; } #if !PBTCOMPILER ////// XamlRoutedEventNode /// internal class XamlRoutedEventNode : XamlAttributeNode { ////// Constructor /// internal XamlRoutedEventNode( int lineNumber, int linePosition, int depth, RoutedEvent routedEvent, string assemblyName, string typeFullName, string routedEventName, string value) : base (XamlNodeType.RoutedEvent,lineNumber,linePosition,depth,value) { _routedEvent = routedEvent; _assemblyName = assemblyName; _typeFullName = typeFullName; _routedEventName = routedEventName; } ////// RoutedEvent ID for this node /// internal RoutedEvent Event { get { return _routedEvent;} } ////// Assembly that contains the owner Type /// internal string AssemblyName { get { return _assemblyName; } } ////// TypeFullName of the owner /// internal string TypeFullName { get { return _typeFullName; } } ////// EventName /// internal string EventName { get { return _routedEventName;} } RoutedEvent _routedEvent; string _assemblyName; string _typeFullName; string _routedEventName; } #endif ////// XamlXmlnsPropertyNode /// [DebuggerDisplay("Xmlns:{_prefix)={_xmlNamespace}")] internal class XamlXmlnsPropertyNode : XamlNode { ////// Constructor /// internal XamlXmlnsPropertyNode( int lineNumber, int linePosition, int depth, string prefix, string xmlNamespace) : base (XamlNodeType.XmlnsProperty,lineNumber,linePosition,depth) { _prefix = prefix; _xmlNamespace = xmlNamespace; } ////// Namespace Prefx /// internal string Prefix { get { return _prefix;} } ////// XmlNamespace associated with the prefix /// internal string XmlNamespace { get { return _xmlNamespace;} } string _prefix; string _xmlNamespace; } ////// XamlPIMappingNode which maps an xml namespace to a clr namespace and assembly /// [DebuggerDisplay("PIMap:{_xmlns}={_clrns};{_assy}")] internal class XamlPIMappingNode : XamlNode { ////// Cosntructor /// internal XamlPIMappingNode( int lineNumber, int linePosition, int depth, string xmlNamespace, string clrNamespace, string assemblyName) : base (XamlNodeType.PIMapping,lineNumber,linePosition,depth) { _xmlns = xmlNamespace; _clrns = clrNamespace; _assy = assemblyName; } ////// Xml namespace for this mapping instruction /// internal string XmlNamespace { get { return _xmlns;} } ////// Clr namespace that maps to the Xml namespace for this mapping instruction /// internal string ClrNamespace { get { return _clrns;} } ////// Assembly where the CLR namespace object can be found /// internal string AssemblyName { get { return _assy;} #if PBTCOMPILER set { _assy = value;} #endif } string _xmlns; string _clrns; string _assy; } ////// XamlClrEventNode, which is a clr event on any object. Note that /// this may be on a DependencyObject, or any other object type. /// internal class XamlClrEventNode : XamlAttributeNode { ////// Constructor /// internal XamlClrEventNode( int lineNumber, int linePosition, int depth, string eventName, MemberInfo eventMember, // Could either be an eventinfo or a methodinfo for the AddHandler method string value) : base (XamlNodeType.ClrEvent,lineNumber,linePosition,depth,value) { #if PBTCOMPILER _eventName = eventName; _eventMember = eventMember; #if HANDLEDEVENTSTOO _handledEventsToo = false; #endif #endif } #if PBTCOMPILER /// /// Name of the Event /// internal string EventName { get { return _eventName;} } ////// EventInfo for the for this event or /// MethodInfo for the Add{EventName}Handler method /// internal MemberInfo EventMember { get { return _eventMember; } } // // The type of the listener. This is relevant for // attached events, where the type of the listener is // different from the type of the event. This is used // by the markup compiler. // internal Type ListenerType { get { return _listenerType; } set { _listenerType = value; } } #if HANDLEDEVENTSTOO ////// HandledEventsToo flag for this event /// internal bool HandledEventsToo { get { return _handledEventsToo; } set { _handledEventsToo = value; } } #endif ////// This event is specified via an EventSetter in a Style. /// internal bool IsStyleSetterEvent { get { return _isStyleSetterEvent; } set { _isStyleSetterEvent = value; } } ////// This event is an event attribute inside a template. /// internal bool IsTemplateEvent { get { return _isTemplateEvent; } set { _isTemplateEvent = value; } } ////// An intermediary parser should skip processing this event if false /// internal bool IsOriginatingEvent { get { return _isOriginatingEvent; } set { _isOriginatingEvent = value; } } ////// true if this event needs to be added to the current scope /// false if a new scope needs to be started and a new connectionId /// is generated /// internal bool IsSameScope { get { return _isSameScope; } set { _isSameScope = value; } } ////// The short name of the local assembly if this event is locally defined /// internal string LocalAssemblyName { get { return _localAssemblyName; } set { _localAssemblyName = value; } } ////// The connectionId identifying the scope to which this event belongs. /// internal Int32 ConnectionId { get { return _connectionId; } set { _connectionId = value; } } #if HANDLEDEVENTSTOO bool _handledEventsToo; #endif private Type _listenerType; bool _isStyleSetterEvent; bool _isTemplateEvent; bool _isOriginatingEvent = true; bool _isSameScope; string _localAssemblyName; Int32 _connectionId; string _eventName; MemberInfo _eventMember; #endif } ////// Xaml Array Start Property Node /// internal class XamlPropertyArrayStartNode : XamlPropertyComplexStartNode { ////// Constructor /// internal XamlPropertyArrayStartNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (XamlNodeType.PropertyArrayStart, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { } } ////// Xaml IList or IAddChild Start Property Node /// ////// This is meant for properties that have a list-like interface for adding items, and /// are read-only properties on an object. The list-like interface may be IList, /// IEnumerator or IAddChild. Note that if the property itself does not actually /// support IList or IAddChild, then the object that contains the property can implement /// IAddChild, and that will be used to add items. /// internal class XamlPropertyIListStartNode : XamlPropertyComplexStartNode { ////// Constructor /// internal XamlPropertyIListStartNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (XamlNodeType.PropertyIListStart, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { } } ////// XamlIDictionaryPropertyNode /// internal class XamlPropertyIDictionaryStartNode : XamlPropertyComplexStartNode { ////// Cosntructor /// internal XamlPropertyIDictionaryStartNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty, MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (XamlNodeType.PropertyIDictionaryStart, lineNumber, linePosition, depth, propertyMember, assemblyName, typeFullName, propertyName) { } } ////// Xaml End Array Property Node /// internal class XamlPropertyArrayEndNode : XamlPropertyComplexEndNode { ////// Constructor /// internal XamlPropertyArrayEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.PropertyArrayEnd,lineNumber,linePosition,depth) { } } ////// Xaml End IList or IAddChild Property Node /// ////// This is meant for properties that have a list-like interface for adding items, and /// are read-only properties on an object. The list-like interface may be IList, /// IEnumerator or IAddChild. Note that if the property itself does not actually /// support IList or IAddChild, then the object that contains the property can implement /// IAddChild, and that will be used to add items. /// internal class XamlPropertyIListEndNode : XamlPropertyComplexEndNode { ////// Constructor /// internal XamlPropertyIListEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.PropertyIListEnd,lineNumber,linePosition,depth) { } } ////// Xaml End IDictionary Property Node /// internal class XamlPropertyIDictionaryEndNode : XamlPropertyComplexEndNode { ////// Constructor /// internal XamlPropertyIDictionaryEndNode(int lineNumber,int linePosition,int depth) : base (XamlNodeType.PropertyIDictionaryEnd,lineNumber,linePosition,depth) { } } ////// Xaml End Attributes Node /// internal class XamlEndAttributesNode : XamlNode { ////// Constructor /// internal XamlEndAttributesNode( int lineNumber, int linePosition, int depth, bool compact) : base (XamlNodeType.EndAttributes,lineNumber,linePosition,depth) { _compact = compact; } ////// True if this node ends the attributes of a compact attribute /// internal bool IsCompact { get { return _compact; } } bool _compact; } ////// XamlDefTagNode /// internal class XamlDefTagNode : XamlAttributeNode { ////// Constructor /// internal XamlDefTagNode( int lineNumber, int linePosition, int depth, bool isEmptyElement, XmlReader xmlReader, string defTagName) : base (XamlNodeType.DefTag,lineNumber,linePosition,depth,defTagName) { #if PBTCOMPILER _xmlReader = xmlReader; _isEmptyElement = isEmptyElement; #endif } #if PBTCOMPILER ////// XmlReader to use when reading the nodes /// internal XmlReader XmlReader { get { return _xmlReader; } } ////// True if Reader is on an Empty element /// internal bool IsEmptyElement { get { return _isEmptyElement; } } XmlReader _xmlReader; bool _isEmptyElement; #endif } ////// XamlDefAttributeNode /// internal class XamlDefAttributeNode : XamlAttributeNode { #if !PBTCOMPILER ////// Constructor /// internal XamlDefAttributeNode( int lineNumber, int linePosition, int depth, string name, string value) : base (XamlNodeType.DefAttribute,lineNumber,linePosition,depth,value) { _attributeUsage = BamlAttributeUsage.Default; _name = name; } #endif ////// Constructor /// internal XamlDefAttributeNode( int lineNumber, int linePosition, int depth, string name, string value, BamlAttributeUsage bamlAttributeUsage) : base (XamlNodeType.DefAttribute,lineNumber,linePosition,depth,value) { _attributeUsage = bamlAttributeUsage; _name = name; } ////// Name of the Attribute /// internal string Name { get { return _name; } } ////// Gives the specific usage of this property /// ////// Some properties are not only set on an element, but have some other effects /// such as setting the xml:lang or xml:space values in the parser context. /// The AttributeUsage describes addition effects or usage for this property. /// internal BamlAttributeUsage AttributeUsage { get { return _attributeUsage; } } BamlAttributeUsage _attributeUsage; string _name; } ////// XamlDefAttributeNode /// internal class XamlDefAttributeKeyTypeNode : XamlAttributeNode { ////// Constructor /// internal XamlDefAttributeKeyTypeNode( int lineNumber, int linePosition, int depth, string value, // Full type name of the type string assemblyName, // Full assembly name where the value type is defined Type valueType) // Actual Type, if known. : base (XamlNodeType.DefKeyTypeAttribute,lineNumber,linePosition,depth,value) { _assemblyName = assemblyName; _valueType = valueType; } ////// Name of the Assembly where the value type is defined. /// internal string AssemblyName { get { return _assemblyName; } } ////// Gives the specific usage of this property /// internal Type ValueType { get { return _valueType; } } string _assemblyName; Type _valueType; } ////// XamlPresentationOptionsAttributeNode /// internal class XamlPresentationOptionsAttributeNode : XamlAttributeNode { ////// Constructor /// internal XamlPresentationOptionsAttributeNode( int lineNumber, int linePosition, int depth, string name, string value) : base (XamlNodeType.PresentationOptionsAttribute,lineNumber,linePosition,depth,value) { _name = name; } ////// Name of the Attribute /// internal string Name { get { return _name; } } ////// Gives the specific usage of this property /// ////// Some properties are not only set on an element, but have some other effects /// such as setting the xml:lang or xml:space values in the parser context. /// The AttributeUsage describes addition effects or usage for this property. /// //internal BamlAttributeUsage AttributeUsage //{ // get { return BamlAttributeUsage.Default; } //} string _name; } ////// Xaml start element Node that is used to define a section that is /// the key object for an IDictionary /// internal class XamlKeyElementStartNode : XamlElementStartNode { ////// Constructor /// internal XamlKeyElementStartNode( int lineNumber, int linePosition, int depth, string assemblyName, string typeFullName, Type elementType, Type serializerType) : base (XamlNodeType.KeyElementStart, lineNumber, linePosition, depth, assemblyName, typeFullName, elementType, serializerType, false, false, false) { } } ////// XamlKeyElementEndNode /// internal class XamlKeyElementEndNode : XamlElementEndNode { ////// Constructor /// internal XamlKeyElementEndNode( int lineNumber, int linePosition, int depth) : base (XamlNodeType.KeyElementEnd,lineNumber,linePosition,depth) { } } internal class XamlContentPropertyNode : XamlNode { ////// Constructor /// internal XamlContentPropertyNode( int lineNumber, int linePosition, int depth, object propertyMember, // DependencyProperty or MethodInfo or PropertyInfo string assemblyName, string typeFullName, string propertyName) : base (XamlNodeType.ContentProperty, lineNumber, linePosition, depth) { if (typeFullName == null) { throw new ArgumentNullException("typeFullName"); } if (propertyName == null) { throw new ArgumentNullException("propertyName"); } _propertyMember = propertyMember; _assemblyName = assemblyName; _typeFullName = typeFullName; _propName = propertyName; } ////// Assembly of the type that owns or has declared the Property /// internal string AssemblyName { get { return _assemblyName; } } ////// TypeFullName of type that owns or has declared the Property /// internal string TypeFullName { get { return _typeFullName; } } ////// Name of the Property /// internal string PropName { get { return _propName; } } ////// Type of the owner or declarer of this Property /// internal Type PropDeclaringType { get { // Lazy initialize this to avoid addition reflection if it // is not needed. if (_declaringType == null && _propertyMember != null) { _declaringType = XamlTypeMapper.GetDeclaringType(_propertyMember); } return _declaringType; } } ////// Valid Type of the Property /// internal Type PropValidType { get { // Lazy initialize this to avoid addition reflection if it // is not needed. if (_validType == null) { _validType = XamlTypeMapper.GetPropertyType(_propertyMember); } return _validType; } } Type _declaringType; Type _validType; object _propertyMember; string _assemblyName; string _typeFullName; string _propName; } #endregion XamlNodeTypeDefitions } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
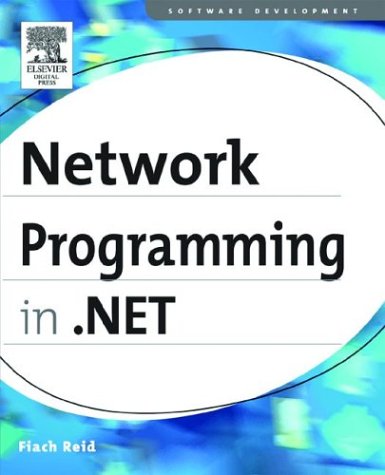
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionNode.cs
- DynamicDocumentPaginator.cs
- ResXBuildProvider.cs
- FileLogRecordHeader.cs
- DataListCommandEventArgs.cs
- ByteViewer.cs
- DiscardableAttribute.cs
- ZipIORawDataFileBlock.cs
- ProfileEventArgs.cs
- TypedTableHandler.cs
- AnimatedTypeHelpers.cs
- FilterableAttribute.cs
- AppDomain.cs
- SecurityTokenResolver.cs
- DecoderBestFitFallback.cs
- ASCIIEncoding.cs
- TextComposition.cs
- PropertyMetadata.cs
- ArrayConverter.cs
- Metafile.cs
- OutputCacheProfileCollection.cs
- TreeViewCancelEvent.cs
- UInt32.cs
- RelationshipNavigation.cs
- ParserExtension.cs
- HttpRuntimeSection.cs
- DayRenderEvent.cs
- FileRegion.cs
- ColumnWidthChangingEvent.cs
- Operator.cs
- GroupDescription.cs
- PrtCap_Public_Simple.cs
- WmlTextViewAdapter.cs
- EventLogPropertySelector.cs
- PropertyGridCommands.cs
- OleServicesContext.cs
- WmpBitmapEncoder.cs
- TimeSpan.cs
- SafeFindHandle.cs
- DataGridPageChangedEventArgs.cs
- PageTheme.cs
- DrawingContextWalker.cs
- KnownTypes.cs
- XMLSchema.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- UrlMapping.cs
- QueryOperationResponseOfT.cs
- PerformanceCountersElement.cs
- RetrieveVirtualItemEventArgs.cs
- ContainerSelectorActiveEvent.cs
- ValueSerializerAttribute.cs
- StringConcat.cs
- WSHttpBindingCollectionElement.cs
- EventArgs.cs
- KeyGesture.cs
- DefaultCommandConverter.cs
- ZipIOExtraFieldPaddingElement.cs
- SqlGatherConsumedAliases.cs
- PropertyItemInternal.cs
- PathFigureCollectionConverter.cs
- UndoManager.cs
- XmlnsCompatibleWithAttribute.cs
- DocumentGrid.cs
- ExpressionBinding.cs
- CheckBoxField.cs
- JsonEnumDataContract.cs
- MediaEntryAttribute.cs
- RayHitTestParameters.cs
- ServiceAuthorizationManager.cs
- TextProviderWrapper.cs
- COSERVERINFO.cs
- TextRangeEditLists.cs
- input.cs
- Screen.cs
- SafePEFileHandle.cs
- XmlSchemaAttributeGroup.cs
- Paragraph.cs
- ApplicationSettingsBase.cs
- DivideByZeroException.cs
- DataControlFieldTypeEditor.cs
- SortDescription.cs
- DataGridLinkButton.cs
- XmlNodeChangedEventManager.cs
- Polyline.cs
- DocumentGrid.cs
- OperationFormatUse.cs
- PngBitmapDecoder.cs
- RectKeyFrameCollection.cs
- IxmlLineInfo.cs
- HtmlControlPersistable.cs
- ExpressionVisitor.cs
- DeferredReference.cs
- Column.cs
- RuntimeWrappedException.cs
- _BufferOffsetSize.cs
- CallContext.cs
- IteratorFilter.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- TypefaceMetricsCache.cs
- UnauthorizedWebPart.cs