Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DLinq / Dlinq / CompiledQuery.cs / 1305376 / CompiledQuery.cs
using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Data.Linq.Mapping; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; MappingSource mappingSource; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static FuncCompile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14, TArg15 arg15) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14, arg15}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); this.mappingSource = context.Mapping.MappingSource; } } } else { if (context.Mapping.MappingSource != this.mappingSource) throw Error.QueryWasCompiledForDifferentMappingSource(); } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Data.Linq.Mapping; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; MappingSource mappingSource; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14, TArg15 arg15) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14, arg15}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); this.mappingSource = context.Mapping.MappingSource; } } } else { if (context.Mapping.MappingSource != this.mappingSource) throw Error.QueryWasCompiledForDifferentMappingSource(); } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
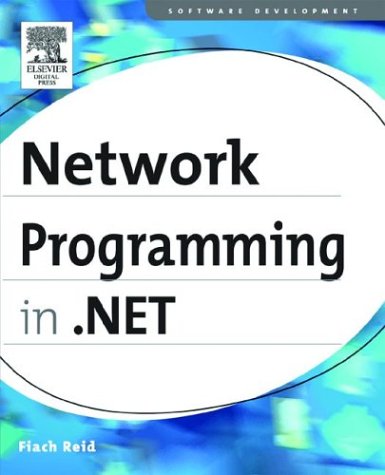
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseParser.cs
- PrintControllerWithStatusDialog.cs
- FixedSOMElement.cs
- Label.cs
- TileBrush.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TrackingMemoryStreamFactory.cs
- ByteStack.cs
- WindowsScrollBarBits.cs
- ResizeGrip.cs
- IisTraceListener.cs
- SafeSecurityHandles.cs
- StreamMarshaler.cs
- BamlStream.cs
- AdPostCacheSubstitution.cs
- CodePrimitiveExpression.cs
- CSharpCodeProvider.cs
- ping.cs
- ContainerVisual.cs
- CapabilitiesSection.cs
- XamlPoint3DCollectionSerializer.cs
- ComNativeDescriptor.cs
- ProviderCommandInfoUtils.cs
- PropertyEntry.cs
- ItemPager.cs
- AppSettings.cs
- ByteStreamMessageUtility.cs
- IntranetCredentialPolicy.cs
- util.cs
- OverflowException.cs
- ExpressionsCollectionConverter.cs
- prefixendpointaddressmessagefiltertable.cs
- RefreshEventArgs.cs
- DesignTimeTemplateParser.cs
- LiteralTextParser.cs
- PathData.cs
- MenuItemCollection.cs
- TreeNodeStyleCollection.cs
- _ConnectStream.cs
- AsymmetricAlgorithm.cs
- MimeTypeAttribute.cs
- Subordinate.cs
- Rect3D.cs
- SqlCacheDependencyDatabaseCollection.cs
- ReceiveCompletedEventArgs.cs
- XsdDataContractExporter.cs
- InnerItemCollectionView.cs
- MaterialCollection.cs
- AssemblyNameProxy.cs
- Convert.cs
- SqlGatherProducedAliases.cs
- _ConnectStream.cs
- SignedInfo.cs
- SettingsPropertyCollection.cs
- RemotingConfiguration.cs
- PersonalizationProvider.cs
- BindToObject.cs
- ReadOnlyHierarchicalDataSourceView.cs
- PropertyGridView.cs
- VerificationException.cs
- ListenerSingletonConnectionReader.cs
- InputProcessorProfiles.cs
- PolyLineSegment.cs
- TemplatedMailWebEventProvider.cs
- Keywords.cs
- _SecureChannel.cs
- CheckBoxList.cs
- JapaneseCalendar.cs
- DockingAttribute.cs
- SystemWebExtensionsSectionGroup.cs
- ConfigurationStrings.cs
- ModifiableIteratorCollection.cs
- VirtualizingPanel.cs
- FamilyMap.cs
- ToolStripDropDownClosedEventArgs.cs
- TemplatePagerField.cs
- COM2ExtendedTypeConverter.cs
- FixedTextSelectionProcessor.cs
- XmlSchemaElement.cs
- SynchronizationLockException.cs
- EventsTab.cs
- ViewBase.cs
- CheckBoxFlatAdapter.cs
- BinaryObjectInfo.cs
- ScrollItemProviderWrapper.cs
- SqlWebEventProvider.cs
- HashHelpers.cs
- RoutedEvent.cs
- UserControl.cs
- CompilerErrorCollection.cs
- JsonGlobals.cs
- PhonemeConverter.cs
- TextInfo.cs
- ServiceEndpointCollection.cs
- UnmanagedBitmapWrapper.cs
- ImageMetadata.cs
- XPathExpr.cs
- ImportedNamespaceContextItem.cs
- HitTestWithGeometryDrawingContextWalker.cs