Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / StateMachines / StateMachine.cs / 1 / StateMachine.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains definitions and default implementations for the various // state machines in the protocol provider using System; using System.Collections; using System.Diagnostics; using System.Text; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.StateMachines { abstract partial class StateMachine : ITimerRecipient { protected TransactionEnlistment enlistment; protected ProtocolState state; protected SynchronizationManager synchronization; State current; StateMachineHistory history; protected StateMachine(TransactionEnlistment enlistment) { this.enlistment = enlistment; this.state = enlistment.State; this.synchronization = new SynchronizationManager(this); if (DebugTrace.Warning || DiagnosticUtility.ShouldTraceWarning) { this.history = new StateMachineHistory(); } } public override string ToString() { return this.GetType().Name; } public State State { get { return this.current; } } public abstract State AbortedState { get; } public TransactionEnlistment Enlistment { get { return this.enlistment; } } public StateMachineHistory History { get { return this.history; } } public Guid UniqueId { get { return this.enlistment.EnlistmentId; } } public void Enqueue (SynchronizationEvent e) { this.synchronization.Enqueue (e); } public void Dispatch (SynchronizationEvent e) { if (this.history != null) { this.history.AddEvent (e.ToString()); } e.Execute(this); } public void ChangeState (State newState) { if (this.history != null) { this.history.AddState (newState.ToString()); } // Deliver leave event if (this.current != null) { if (DebugTrace.Info) { DebugTrace.TxTrace(TraceLevel.Info, this.enlistment.EnlistmentId, "Leaving [{0}]", this.current); } this.current.Leave (this); } if (DebugTrace.Info) { DebugTrace.TxTrace (TraceLevel.Info, this.enlistment.EnlistmentId, "Entering [{0}]", newState); } // Set current state this.current = newState; // Deliver enter event this.current.Enter (this); } public void TraceInvalidEvent (SynchronizationEvent e, bool fatal) { // Debug tracing if (DebugTrace.Error) { if (this.history != null) { DebugTrace.TxTrace ( TraceLevel.Error, e.Enlistment.EnlistmentId, "The {0} was not expected by the {1} state. The state machine history history follows:\n\n{2}", e, this.current, this.history.ToString() ); } else { DebugTrace.TxTrace ( TraceLevel.Error, e.Enlistment.EnlistmentId, "The {0} was not expected by the {1} state", e, this.current ); } } // Diagnostic tracing if (fatal) { FatalUnexpectedStateMachineEventRecord.TraceAndLog ( this.enlistment.EnlistmentId, this.enlistment.Enlistment.RemoteTransactionId, this.ToString(), this.current.ToString(), this.history, e.ToString(), null // It would have been nice to have added context-sensitive details here. // Maybe next time... See MB 19006 for more details ); } else { NonFatalUnexpectedStateMachineEventRecord.TraceAndLog ( this.enlistment.EnlistmentId, this.enlistment.Enlistment.RemoteTransactionId, this.ToString(), this.current.ToString(), this.history, e.ToString(), null // It would have been nice to have added context-sensitive details here. // Maybe next time... See MB 19006 for more details ); } } public virtual void Cleanup() { this.enlistment.OnStateMachineComplete(); } } abstract class ParticipantStateMachine : StateMachine { ParticipantEnlistment participant; public ParticipantStateMachine (ParticipantEnlistment participant) : base (participant) { this.participant = participant; } protected override void OnTimer(TimerProfile profile) { base.OnTimer(profile); this.synchronization.Enqueue(new TimerParticipantEvent(participant, profile)); } } class DurableStateMachine : ParticipantStateMachine { public DurableStateMachine (ParticipantEnlistment participant) : base (participant) {} public override State AbortedState { get { return state.States.DurableAborted; } } } class VolatileStateMachine : ParticipantStateMachine { public VolatileStateMachine (ParticipantEnlistment participant) : base (participant) {} public override State AbortedState { get { return state.States.VolatileAborted; } } } class SubordinateStateMachine : StateMachine { public SubordinateStateMachine(ParticipantEnlistment participant) : base(participant) { } public override State AbortedState { get { return state.States.SubordinateFinished; } } } class CompletionStateMachine : StateMachine { public CompletionStateMachine (CompletionEnlistment completion) : base (completion) { } public override State AbortedState { get { return state.States.CompletionAborted; } } } class CoordinatorStateMachine : StateMachine { CoordinatorEnlistment coordinator; public CoordinatorStateMachine (CoordinatorEnlistment coordinator) : base (coordinator) { this.coordinator = coordinator; } public override State AbortedState { get { return state.States.CoordinatorAborted; } } protected override void OnTimer(TimerProfile profile) { base.OnTimer(profile); this.synchronization.Enqueue (new TimerCoordinatorEvent (this.coordinator, profile)); } } class TransactionContextStateMachine : StateMachine { TransactionContextManager contextManager; public TransactionContextStateMachine(TransactionContextManager contextManager) : base(contextManager) { this.contextManager = contextManager; } public override State AbortedState { get { return state.States.TransactionContextFinished; } } public TransactionContextManager ContextManager { get { return this.contextManager; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
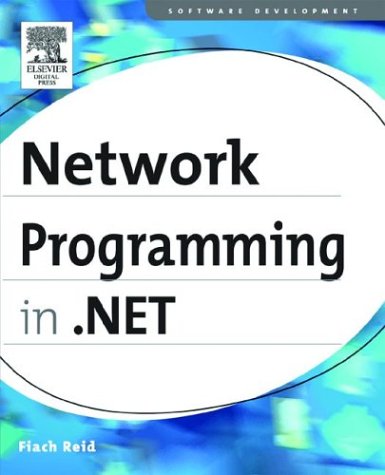
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerProperties.cs
- RepeaterItemCollection.cs
- ObjectStateFormatter.cs
- xdrvalidator.cs
- SqlTriggerAttribute.cs
- DataRowExtensions.cs
- HttpClientCredentialType.cs
- SelectionEditingBehavior.cs
- DockPanel.cs
- HMACSHA384.cs
- PanningMessageFilter.cs
- MemberRestriction.cs
- Journal.cs
- HostSecurityManager.cs
- InvalidDataException.cs
- SymbolEqualComparer.cs
- AsyncPostBackTrigger.cs
- Parameter.cs
- ClientConfigPaths.cs
- RelationshipConstraintValidator.cs
- PageHandlerFactory.cs
- Site.cs
- TcpAppDomainProtocolHandler.cs
- X509CertificateValidator.cs
- ImageMapEventArgs.cs
- CalendarDataBindingHandler.cs
- MatrixTransform3D.cs
- ListControlConvertEventArgs.cs
- TextSelectionHelper.cs
- LinkedResourceCollection.cs
- CodeCommentStatementCollection.cs
- PowerModeChangedEventArgs.cs
- CodeDomLocalizationProvider.cs
- TableLayoutColumnStyleCollection.cs
- ClientTargetCollection.cs
- UIElementPropertyUndoUnit.cs
- SerializationInfoEnumerator.cs
- XmlSchemaDatatype.cs
- SimpleFieldTemplateUserControl.cs
- Expressions.cs
- GcHandle.cs
- EntityDataSourceConfigureObjectContext.cs
- XmlTypeAttribute.cs
- RenamedEventArgs.cs
- BindingExpressionUncommonField.cs
- TaskFileService.cs
- DocumentGridPage.cs
- CheckBoxField.cs
- TypeAccessException.cs
- HtmlImage.cs
- KeyToListMap.cs
- TextSelectionHighlightLayer.cs
- precedingquery.cs
- ZipIOCentralDirectoryBlock.cs
- SoapIncludeAttribute.cs
- RequestCachePolicy.cs
- OleDbFactory.cs
- ConditionChanges.cs
- BaseTemplateParser.cs
- XmlRootAttribute.cs
- DataGridLengthConverter.cs
- _DomainName.cs
- WindowAutomationPeer.cs
- ThemeInfoAttribute.cs
- XmlSchemaExporter.cs
- ProgressiveCrcCalculatingStream.cs
- XmlImplementation.cs
- CrossContextChannel.cs
- Sql8ConformanceChecker.cs
- NullExtension.cs
- ComEventsSink.cs
- AudienceUriMode.cs
- XsltFunctions.cs
- Rethrow.cs
- AutoGeneratedFieldProperties.cs
- RowUpdatedEventArgs.cs
- ParsedRoute.cs
- ButtonFieldBase.cs
- MembershipSection.cs
- hwndwrapper.cs
- XmlElement.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DataGridDetailsPresenterAutomationPeer.cs
- ExpressionEditorAttribute.cs
- DataSourceHelper.cs
- ByteFacetDescriptionElement.cs
- VariableElement.cs
- SwitchExpression.cs
- SerialStream.cs
- FlowLayoutPanel.cs
- XmlUrlEditor.cs
- RepeaterItemCollection.cs
- MailMessageEventArgs.cs
- ParseHttpDate.cs
- ExpressionEditorAttribute.cs
- InputGestureCollection.cs
- ColumnMap.cs
- FlagsAttribute.cs
- Base64WriteStateInfo.cs
- DLinqColumnProvider.cs