Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ImageKeyConverter.cs / 1305376 / ImageKeyConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Collections.Specialized; ////// /// ImageIndexConverter is a class that can be used to convert /// image index values one data type to another. /// public class ImageKeyConverter : StringConverter { private string parentImageListProperty = "Parent"; ///protected virtual bool IncludeNoneAsStandardValue { get { return true; } } /// /// this is the property to look at when there is no ImageList property /// on the current object. For example, in ToolBarButton - the ImageList is /// on the ToolBarButton.Parent property. In WinBarItem, the ImageList is on /// the WinBarItem.Owner property. /// internal string ParentImageListProperty { get { return parentImageListProperty; } set { parentImageListProperty = value; } } ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can convert an object in the /// given source type to a string using the specified context. ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { return (string)value; } if (value == null) { return ""; } return base.ConvertFrom(context, culture, value); } ///Converts the specified value object to a string object. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value != null && value is string && ((string)value).Length == 0) { return SR.GetString(SR.toStringNone); } else if (destinationType == typeof(string) && (value == null)) { return SR.GetString(SR.toStringNone); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { object instance = context.Instance; PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); while (instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { ImageList imageList = (ImageList)imageListProp.GetValue(instance); if (imageList != null) { // Create array to contain standard values // object[] values; int nImages = imageList.Images.Count; if (IncludeNoneAsStandardValue) { values = new object[nImages + 1]; values[nImages] = ""; } else { values = new object[nImages]; } // Fill in the array // StringCollection imageKeys = imageList.Images.Keys; for (int i = 0; i < imageKeys.Count; i++) { if ((imageKeys[i] != null) && (imageKeys[i].Length != 0)) values[i] = imageKeys[i]; } return new StandardValuesCollection(values); } } } if (IncludeNoneAsStandardValue) { return new StandardValuesCollection(new object[] {""}); } else { return new StandardValuesCollection(new object[0]); } } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return true; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
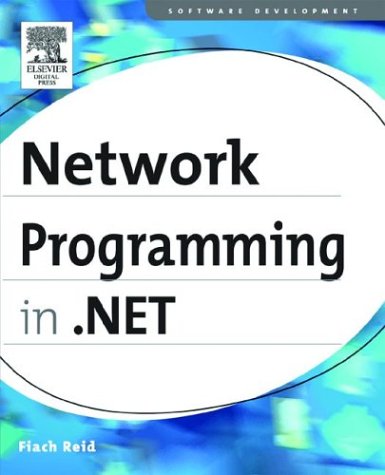
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Signature.cs
- ItemsPanelTemplate.cs
- unsafenativemethodsother.cs
- CharConverter.cs
- WindowsAuthenticationModule.cs
- CorrelationInitializer.cs
- QilInvokeEarlyBound.cs
- MappingMetadataHelper.cs
- SqlUDTStorage.cs
- ObjectStateFormatter.cs
- MetadataSerializer.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- WindowsIdentity.cs
- Html32TextWriter.cs
- COMException.cs
- OpenTypeCommon.cs
- InheritanceRules.cs
- AnnotationResourceCollection.cs
- InkCanvasSelection.cs
- _NetworkingPerfCounters.cs
- TabletCollection.cs
- bidPrivateBase.cs
- ComponentChangedEvent.cs
- ProtocolsConfigurationEntry.cs
- ContextBase.cs
- ImageBrush.cs
- DocumentPageView.cs
- FilterException.cs
- VersionPair.cs
- BitmapEffectGeneralTransform.cs
- XmlILAnnotation.cs
- WaitHandleCannotBeOpenedException.cs
- ReflectEventDescriptor.cs
- TaskFileService.cs
- XmlNavigatorStack.cs
- DiagnosticsConfigurationHandler.cs
- XmlUtilWriter.cs
- TextEditor.cs
- AuthStoreRoleProvider.cs
- PropertiesTab.cs
- FlowDocumentPageViewerAutomationPeer.cs
- LoginAutoFormat.cs
- Group.cs
- KeyInfo.cs
- Debug.cs
- TypeDependencyAttribute.cs
- AsymmetricKeyExchangeDeformatter.cs
- _NestedSingleAsyncResult.cs
- SystemIdentity.cs
- CodeAssignStatement.cs
- ReflectionPermission.cs
- VirtualPath.cs
- UserControl.cs
- JsonMessageEncoderFactory.cs
- ElementUtil.cs
- Currency.cs
- Int16.cs
- SqlClientMetaDataCollectionNames.cs
- RMEnrollmentPage2.cs
- SolidColorBrush.cs
- DataGridViewTextBoxColumn.cs
- FlowDocumentPaginator.cs
- Int32Animation.cs
- ValidationVisibilityAttribute.cs
- XmlSchemaChoice.cs
- ObjectContextServiceProvider.cs
- Convert.cs
- RequestResizeEvent.cs
- EdmComplexPropertyAttribute.cs
- OleDbReferenceCollection.cs
- SqlDuplicator.cs
- Focus.cs
- Fault.cs
- StructureChangedEventArgs.cs
- xamlnodes.cs
- DiscoveryClientProtocol.cs
- DbUpdateCommandTree.cs
- Misc.cs
- SerializerDescriptor.cs
- DebugController.cs
- BindingMAnagerBase.cs
- InvokeProviderWrapper.cs
- SafeFileMapViewHandle.cs
- SqlMethodTransformer.cs
- ServiceProviders.cs
- DependencyObjectProvider.cs
- MasterPageCodeDomTreeGenerator.cs
- Int32Converter.cs
- TaskFormBase.cs
- EFColumnProvider.cs
- SourceItem.cs
- CounterSampleCalculator.cs
- DrawingImage.cs
- SettingsSavedEventArgs.cs
- SqlClientWrapperSmiStreamChars.cs
- UIElementPropertyUndoUnit.cs
- NamespaceInfo.cs
- OdbcReferenceCollection.cs
- IndexerNameAttribute.cs
- XsdBuilder.cs