Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SafeRsaProviderHandle.cs / 1 / SafeRsaProviderHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.ComponentModel; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Security; using Microsoft.InfoCards.Diagnostics; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Provides a wrapper over a handle retrieved by CryptAcquireContext // internal class SafeRsaProviderHandle : SafeHandle { [DllImport( "advapi32.dll", EntryPoint ="CryptAcquireContextW", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] [SuppressUnmanagedCodeSecurity] private static extern bool CryptAcquireContext( [Out] out SafeRsaProviderHandle hProv, [In] string pszContainer, [In] string pszProvider, [In] uint dwProvType, [In] uint dwFlags ); [DllImport( "advapi32.dll", EntryPoint = "CryptReleaseContext", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern bool CryptReleaseContext( [In] IntPtr hProv, [In] uint dwFlags ); public static SafeRsaProviderHandle Construct() { // // The native provider we use to do encryption and decryption and associated constants to // set up the Microsoft Enhanced RSA and AES provider // const uint CRYPT_VERIFYCONTEXT = 0xF0000000; const byte PROV_RSA_AES = 24; // // We use a null provider and CRYPT_VERIFYCONTEXT as a flag // to force the use of ephemeral keys. See http://support.microsoft.com/default.aspx?scid=kb%3Ben-us%3B238187 // for details. // SafeRsaProviderHandle h; if( !SafeRsaProviderHandle.CryptAcquireContext( out h, null, null, PROV_RSA_AES, CRYPT_VERIFYCONTEXT ) ) { Win32Exception e = new Win32Exception( Marshal.GetLastWin32Error() ); IDT.CloseInvalidOutSafeHandle(h); IDT.TraceAndLogException( e ); throw IDT.ThrowHelperError( new CommunicationException( e.Message ) ); } return h; } private SafeRsaProviderHandle() : base( IntPtr.Zero, true ) { } public override bool IsInvalid { get { return ( IntPtr.Zero == base.handle ); } } protected override bool ReleaseHandle() { #pragma warning suppress 56523 return CryptReleaseContext( base.handle, 0 ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
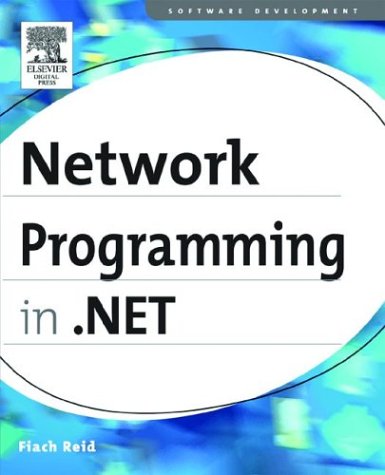
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JsonXmlDataContract.cs
- Blend.cs
- FtpRequestCacheValidator.cs
- AsyncDataRequest.cs
- regiisutil.cs
- ListView.cs
- ButtonChrome.cs
- RunWorkerCompletedEventArgs.cs
- HttpClientChannel.cs
- ReadOnlyCollection.cs
- BmpBitmapEncoder.cs
- XmlSchemaComplexContent.cs
- Events.cs
- TokenBasedSetEnumerator.cs
- ConfigXmlComment.cs
- StaticContext.cs
- LZCodec.cs
- TypeContext.cs
- XmlExceptionHelper.cs
- Assert.cs
- ToolStripLabel.cs
- PasswordDeriveBytes.cs
- DataKey.cs
- ValueCollectionParameterReader.cs
- ReaderWriterLock.cs
- PointLightBase.cs
- MemberCollection.cs
- InvalidPipelineStoreException.cs
- ClientSettingsSection.cs
- BaseTemplateCodeDomTreeGenerator.cs
- GeneralTransform3DCollection.cs
- _NTAuthentication.cs
- SortQueryOperator.cs
- Queue.cs
- InfoCardMasterKey.cs
- XhtmlBasicPanelAdapter.cs
- IndependentAnimationStorage.cs
- XmlSchemaRedefine.cs
- FormsAuthenticationModule.cs
- DocumentPageViewAutomationPeer.cs
- ImpersonationOption.cs
- IndependentAnimationStorage.cs
- DependencyPropertyValueSerializer.cs
- EntityDataSourceStatementEditor.cs
- cookieexception.cs
- LineBreakRecord.cs
- HMACSHA1.cs
- Section.cs
- IndependentlyAnimatedPropertyMetadata.cs
- Rect3D.cs
- Select.cs
- XmlSchemaNotation.cs
- ClrPerspective.cs
- TextServicesCompartment.cs
- FocusChangedEventArgs.cs
- LeaseManager.cs
- DbModificationClause.cs
- ControlDesignerState.cs
- SqlDataSourceCustomCommandPanel.cs
- ForeignKeyConstraint.cs
- TextSelectionProcessor.cs
- TemplateBindingExtensionConverter.cs
- ResourceDisplayNameAttribute.cs
- Base64Decoder.cs
- LastQueryOperator.cs
- PrintDialog.cs
- ElementProxy.cs
- OverflowException.cs
- AssemblyName.cs
- TextEditorMouse.cs
- ItemChangedEventArgs.cs
- OdbcInfoMessageEvent.cs
- InternalCache.cs
- GroupQuery.cs
- PersistenceTypeAttribute.cs
- XmlSequenceWriter.cs
- MsdtcWrapper.cs
- ExtensionFile.cs
- TypeSemantics.cs
- RectangleConverter.cs
- RemoteWebConfigurationHostStream.cs
- _NegotiateClient.cs
- PointValueSerializer.cs
- RemotingException.cs
- PageVisual.cs
- UserNameSecurityTokenAuthenticator.cs
- XmlUrlResolver.cs
- Point3DCollection.cs
- ConsumerConnectionPointCollection.cs
- PrintingPermissionAttribute.cs
- XmlAtomicValue.cs
- HostSecurityManager.cs
- FontDriver.cs
- DockingAttribute.cs
- InvalidCommandTreeException.cs
- ZipPackagePart.cs
- PrintingPermission.cs
- CqlLexer.cs
- MessageLoggingFilterTraceRecord.cs
- SHA1CryptoServiceProvider.cs