Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / RequestCachePolicyConverter.cs / 1 / RequestCachePolicyConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2007 // // File: RequesetCachePolicyConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Net.Cache; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RequestCachePolicyConverter Parses a RequestCachePolicy. /// public sealed class RequestCachePolicyConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - attempt to convert to a RequestCachePolicy from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a RequestCachePolicy. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } HttpRequestCacheLevel level = (HttpRequestCacheLevel)Enum.Parse(typeof(HttpRequestCacheLevel), s, true); return new HttpRequestCachePolicy(level); } ////// ConvertTo - Attempt to convert to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The policy to convert. /// The type to which to convert the policy. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RequestCachePolicy/HttpRequestCachePolicy, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } HttpRequestCachePolicy httpPolicy = value as HttpRequestCachePolicy; if(httpPolicy != null) { if (destinationType == typeof(string)) { return httpPolicy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(HttpRequestCachePolicy).GetConstructor(new Type[] { typeof(HttpRequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { httpPolicy.Level }); } } //if it's not an HttpRequestCachePolicy, try a regular RequestCachePolicy RequestCachePolicy policy = value as RequestCachePolicy; if (policy != null) { if (destinationType == typeof(string)) { return policy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(RequestCachePolicy).GetConstructor(new Type[] { typeof(RequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { policy.Level }); } } throw GetConvertToException(value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
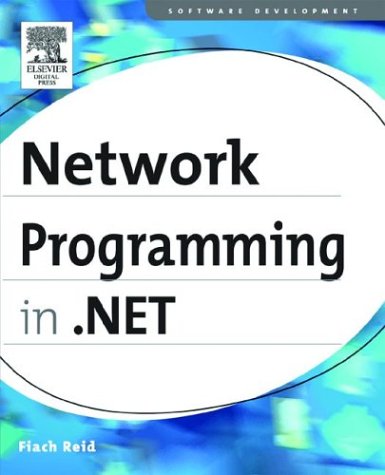
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SourceFileBuildProvider.cs
- ListBindingHelper.cs
- ProgressiveCrcCalculatingStream.cs
- HeaderFilter.cs
- VectorAnimation.cs
- DataGridParentRows.cs
- EventPropertyMap.cs
- SortExpressionBuilder.cs
- XamlToRtfWriter.cs
- StatusBar.cs
- SourceItem.cs
- AxisAngleRotation3D.cs
- XpsSerializerFactory.cs
- MemberMaps.cs
- WsdlBuildProvider.cs
- Array.cs
- VisualBrush.cs
- EnvelopedSignatureTransform.cs
- TableRowCollection.cs
- ConfigsHelper.cs
- C14NUtil.cs
- CollectionDataContract.cs
- BamlResourceDeserializer.cs
- TargetConverter.cs
- SamlSerializer.cs
- WsdlHelpGeneratorElement.cs
- ListBox.cs
- WebMethodAttribute.cs
- Operator.cs
- DataGridViewCellCancelEventArgs.cs
- RNGCryptoServiceProvider.cs
- WorkflowApplication.cs
- VBIdentifierName.cs
- HostingMessageProperty.cs
- RIPEMD160.cs
- PrintingPermissionAttribute.cs
- BaseValidatorDesigner.cs
- PrimaryKeyTypeConverter.cs
- PopupRoot.cs
- XslTransform.cs
- ComboBox.cs
- TypeConverterHelper.cs
- Animatable.cs
- DayRenderEvent.cs
- OutputScope.cs
- ContentType.cs
- ReaderWriterLockWrapper.cs
- ZipFileInfoCollection.cs
- ChtmlMobileTextWriter.cs
- QueryRewriter.cs
- AnnotationObservableCollection.cs
- XmlSiteMapProvider.cs
- SymbolEqualComparer.cs
- HttpHandlerActionCollection.cs
- HashAlgorithm.cs
- CollectionContainer.cs
- InsufficientMemoryException.cs
- NetStream.cs
- LinkConverter.cs
- NavigationHelper.cs
- UnknownBitmapEncoder.cs
- ISessionStateStore.cs
- ControlTemplate.cs
- SQLInt16Storage.cs
- TreeViewDataItemAutomationPeer.cs
- TextRenderer.cs
- EmulateRecognizeCompletedEventArgs.cs
- Helpers.cs
- DynamicAttribute.cs
- StringHandle.cs
- ExpressionPrinter.cs
- ResourceReferenceExpression.cs
- LocalFileSettingsProvider.cs
- NavigationProgressEventArgs.cs
- Transform.cs
- TouchEventArgs.cs
- WebControl.cs
- FixedNode.cs
- CheckBoxStandardAdapter.cs
- JoinTreeNode.cs
- LogSwitch.cs
- BodyGlyph.cs
- TextTreeTextNode.cs
- ResourceType.cs
- IOException.cs
- NodeLabelEditEvent.cs
- DataListItem.cs
- Themes.cs
- SqlFormatter.cs
- ScriptModule.cs
- SqlReferenceCollection.cs
- ProxyGenerationError.cs
- Pen.cs
- TriggerAction.cs
- StylusPointPropertyId.cs
- Hashtable.cs
- MenuItemAutomationPeer.cs
- MarginsConverter.cs
- UserPreferenceChangingEventArgs.cs
- ThousandthOfEmRealPoints.cs