Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / SessionPageStatePersister.cs / 1 / SessionPageStatePersister.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.IO; using System.Text; using System.Web.SessionState; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SessionPageStatePersister : PageStatePersister { private const string _viewStateSessionKey = "__SESSIONVIEWSTATE"; private const string _viewStateQueueKey = "__VIEWSTATEQUEUE"; public SessionPageStatePersister(Page page) : base (page) { HttpSessionState session = null; try { session = page.Session; } catch { // ignore, throw if session is null. } if (session == null) { throw new ArgumentException(SR.GetString(SR.SessionPageStatePersister_SessionMustBeEnabled)); } } public override void Load() { NameValueCollection requestValueCollection = Page.RequestValueCollection; if (requestValueCollection == null) { return; } try { string combinedSerializedStateString = Page.RequestViewStateString; string persistedStateID = null; bool controlStateInSession = false; // SessionState will persist a Pair of, // where if requiresControlStateInSession is true, second will just be the sessionID, as // we will store both control state and view state in session. Otherwise, we store just the // view state in session and the pair will be if (!String.IsNullOrEmpty(combinedSerializedStateString)) { Pair combinedState = (Pair)Util.DeserializeWithAssert(StateFormatter, combinedSerializedStateString); // Check if we are storing control state in session as well if ((bool)combinedState.First) { // So the second is the persistedID persistedStateID = (string)combinedState.Second; controlStateInSession = true; } else { // Second is Pair pair = (Pair)combinedState.Second; persistedStateID = (string)pair.First; ControlState = pair.Second; } } if (persistedStateID != null) { object sessionData = Page.Session[_viewStateSessionKey + persistedStateID]; if (controlStateInSession) { Pair combinedState = sessionData as Pair; if (combinedState != null) { ViewState = combinedState.First; ControlState = combinedState.Second; } } else { ViewState = sessionData; } } } catch (Exception e) { // Setup the formatter for this exception, to make sure this message shows up // in an error page as opposed to the inner-most exception's message. HttpException newException = new HttpException(SR.GetString(SR.Invalid_ControlState), e); newException.SetFormatter(new UseLastUnhandledErrorFormatter(newException)); throw newException; } } /// /// To be supplied. /// public override void Save() { bool requiresControlStateInSession = false; object clientData = null; Triplet vsTrip = ViewState as Triplet; // no session view state to store. if ((ControlState != null) || ((vsTrip == null || vsTrip.Second != null || vsTrip.Third != null) && ViewState != null)) { HttpSessionState session = Page.Session; string sessionViewStateID = Convert.ToString(DateTime.Now.Ticks, 16); object state = null; requiresControlStateInSession = Page.Request.Browser.RequiresControlStateInSession; if (requiresControlStateInSession) { // ClientState will just be sessionID state = new Pair(ViewState, ControlState); clientData = sessionViewStateID; } else { // ClientState will be astate = ViewState; clientData = new Pair(sessionViewStateID, ControlState); } string sessionKey = _viewStateSessionKey + sessionViewStateID; session[sessionKey] = state; Queue queue = session[_viewStateQueueKey] as Queue; if (queue == null) { queue = new Queue(); session[_viewStateQueueKey] = queue; } queue.Enqueue(sessionKey); SessionPageStateSection cfg = RuntimeConfig.GetConfig(Page.Request.Context).SessionPageState; int queueCount = queue.Count; if (cfg != null && queueCount > cfg.HistorySize || cfg == null && queueCount > SessionPageStateSection.DefaultHistorySize) { string oldSessionKey = (string)queue.Dequeue(); session.Remove(oldSessionKey); } } if (clientData != null) { Page.ClientState = Util.SerializeWithAssert(StateFormatter, new Pair(requiresControlStateInSession, clientData)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.IO; using System.Text; using System.Web.SessionState; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SessionPageStatePersister : PageStatePersister { private const string _viewStateSessionKey = "__SESSIONVIEWSTATE"; private const string _viewStateQueueKey = "__VIEWSTATEQUEUE"; public SessionPageStatePersister(Page page) : base (page) { HttpSessionState session = null; try { session = page.Session; } catch { // ignore, throw if session is null. } if (session == null) { throw new ArgumentException(SR.GetString(SR.SessionPageStatePersister_SessionMustBeEnabled)); } } public override void Load() { NameValueCollection requestValueCollection = Page.RequestValueCollection; if (requestValueCollection == null) { return; } try { string combinedSerializedStateString = Page.RequestViewStateString; string persistedStateID = null; bool controlStateInSession = false; // SessionState will persist a Pair of, // where if requiresControlStateInSession is true, second will just be the sessionID, as // we will store both control state and view state in session. Otherwise, we store just the // view state in session and the pair will be if (!String.IsNullOrEmpty(combinedSerializedStateString)) { Pair combinedState = (Pair)Util.DeserializeWithAssert(StateFormatter, combinedSerializedStateString); // Check if we are storing control state in session as well if ((bool)combinedState.First) { // So the second is the persistedID persistedStateID = (string)combinedState.Second; controlStateInSession = true; } else { // Second is Pair pair = (Pair)combinedState.Second; persistedStateID = (string)pair.First; ControlState = pair.Second; } } if (persistedStateID != null) { object sessionData = Page.Session[_viewStateSessionKey + persistedStateID]; if (controlStateInSession) { Pair combinedState = sessionData as Pair; if (combinedState != null) { ViewState = combinedState.First; ControlState = combinedState.Second; } } else { ViewState = sessionData; } } } catch (Exception e) { // Setup the formatter for this exception, to make sure this message shows up // in an error page as opposed to the inner-most exception's message. HttpException newException = new HttpException(SR.GetString(SR.Invalid_ControlState), e); newException.SetFormatter(new UseLastUnhandledErrorFormatter(newException)); throw newException; } } /// /// To be supplied. /// public override void Save() { bool requiresControlStateInSession = false; object clientData = null; Triplet vsTrip = ViewState as Triplet; // no session view state to store. if ((ControlState != null) || ((vsTrip == null || vsTrip.Second != null || vsTrip.Third != null) && ViewState != null)) { HttpSessionState session = Page.Session; string sessionViewStateID = Convert.ToString(DateTime.Now.Ticks, 16); object state = null; requiresControlStateInSession = Page.Request.Browser.RequiresControlStateInSession; if (requiresControlStateInSession) { // ClientState will just be sessionID state = new Pair(ViewState, ControlState); clientData = sessionViewStateID; } else { // ClientState will be astate = ViewState; clientData = new Pair(sessionViewStateID, ControlState); } string sessionKey = _viewStateSessionKey + sessionViewStateID; session[sessionKey] = state; Queue queue = session[_viewStateQueueKey] as Queue; if (queue == null) { queue = new Queue(); session[_viewStateQueueKey] = queue; } queue.Enqueue(sessionKey); SessionPageStateSection cfg = RuntimeConfig.GetConfig(Page.Request.Context).SessionPageState; int queueCount = queue.Count; if (cfg != null && queueCount > cfg.HistorySize || cfg == null && queueCount > SessionPageStateSection.DefaultHistorySize) { string oldSessionKey = (string)queue.Dequeue(); session.Remove(oldSessionKey); } } if (clientData != null) { Page.ClientState = Util.SerializeWithAssert(StateFormatter, new Pair(requiresControlStateInSession, clientData)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
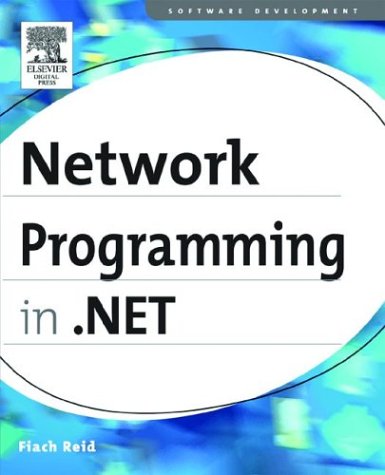
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StatusBarDrawItemEvent.cs
- DataGridRelationshipRow.cs
- AsymmetricSignatureDeformatter.cs
- ObjectComplexPropertyMapping.cs
- FlowDocumentPageViewerAutomationPeer.cs
- EdmConstants.cs
- EllipseGeometry.cs
- CharacterBuffer.cs
- XmlnsCache.cs
- TypeCollectionDesigner.xaml.cs
- StorageEntityContainerMapping.cs
- Deserializer.cs
- ITextView.cs
- BasicViewGenerator.cs
- Paragraph.cs
- DataPagerField.cs
- Blend.cs
- ConsoleEntryPoint.cs
- BehaviorEditorPart.cs
- CompiledIdentityConstraint.cs
- SiteMap.cs
- ListArgumentProvider.cs
- IssuedSecurityTokenParameters.cs
- NameHandler.cs
- CategoryGridEntry.cs
- InputScopeConverter.cs
- FlowLayoutPanel.cs
- ActivityExecutorOperation.cs
- DBCommandBuilder.cs
- CodeDOMProvider.cs
- CqlParser.cs
- RenderDataDrawingContext.cs
- GenericsNotImplementedException.cs
- ParseHttpDate.cs
- SecurityTokenValidationException.cs
- Math.cs
- Variable.cs
- QuotedPrintableStream.cs
- XmlSchemaSubstitutionGroup.cs
- TextProviderWrapper.cs
- WizardForm.cs
- RelatedImageListAttribute.cs
- TypeToken.cs
- GacUtil.cs
- GlobalAclOperationRequirement.cs
- InlineUIContainer.cs
- Exceptions.cs
- Win32Exception.cs
- WebPartConnectionsDisconnectVerb.cs
- QueryCursorEventArgs.cs
- EdmFunction.cs
- FastPropertyAccessor.cs
- SyndicationItemFormatter.cs
- WorkerRequest.cs
- HostUtils.cs
- connectionpool.cs
- SHA1Managed.cs
- HttpRuntimeSection.cs
- SetStoryboardSpeedRatio.cs
- UtilityExtension.cs
- WindowsMenu.cs
- ListViewHitTestInfo.cs
- DataListGeneralPage.cs
- ItemsControl.cs
- StateChangeEvent.cs
- BatchParser.cs
- PageStatePersister.cs
- CanonicalFontFamilyReference.cs
- ImageMapEventArgs.cs
- OperandQuery.cs
- BitmapMetadata.cs
- UnsafeNativeMethods.cs
- SettingsContext.cs
- ImmComposition.cs
- IPPacketInformation.cs
- ISAPIRuntime.cs
- Rule.cs
- ContextItemManager.cs
- ObjectDataSourceEventArgs.cs
- XmlBaseWriter.cs
- HtmlWindow.cs
- SqlUtils.cs
- SystemIcmpV4Statistics.cs
- SimpleType.cs
- ApplicationDirectoryMembershipCondition.cs
- CommandValueSerializer.cs
- ImageSource.cs
- RenderDataDrawingContext.cs
- Span.cs
- ResourcesBuildProvider.cs
- ErrorFormatterPage.cs
- DetailsViewPageEventArgs.cs
- dataobject.cs
- HttpListenerPrefixCollection.cs
- DynamicAttribute.cs
- InternalConfigEventArgs.cs
- SqlCommandSet.cs
- PersonalizationProvider.cs
- ClientType.cs
- SqlDataSourceCommandParser.cs