Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Configuration / System / Configuration / SimpleBitVector32.cs / 1 / SimpleBitVector32.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; // // This is a cut down copy of System.Collections.Specialized.BitVector32. The // reason this is here is because it is used rather intensively by Control and // WebControl. As a result, being able to inline this operations results in a // measurable performance gain, at the expense of some maintainability. // [Serializable] internal struct SimpleBitVector32 { private int data; internal SimpleBitVector32(int data) { this.data = data; } internal int Data { get { return data; } #if UNUSED_CODE set { data = value; } #endif } internal bool this[int bit] { get { return (data & bit) == bit; } set { int _data = data; if(value) { data = _data | bit; } else { data = _data & ~bit; } } } #if UNUSED_CODE internal void Set(int bit) { data |= bit; } internal void Clear(int bit) { data &= ~bit; } internal void Toggle(int bit) { data ^= bit; } /* * COPY_FLAG copies the value of flags from a source field * into a destination field. * * In the macro: * + "&flag" limits the outer xor operation to just the flag we're interested in. * + These are the results of the two xor operations: * * fieldDst fieldSrc inner xor outer xor * 0 0 0 0 * 0 1 1 1 * 1 0 1 0 * 1 1 0 1 */ internal void Copy(SimpleBitVector32 src, int bit) { data ^= (data ^ src.data) & bit; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; // // This is a cut down copy of System.Collections.Specialized.BitVector32. The // reason this is here is because it is used rather intensively by Control and // WebControl. As a result, being able to inline this operations results in a // measurable performance gain, at the expense of some maintainability. // [Serializable] internal struct SimpleBitVector32 { private int data; internal SimpleBitVector32(int data) { this.data = data; } internal int Data { get { return data; } #if UNUSED_CODE set { data = value; } #endif } internal bool this[int bit] { get { return (data & bit) == bit; } set { int _data = data; if(value) { data = _data | bit; } else { data = _data & ~bit; } } } #if UNUSED_CODE internal void Set(int bit) { data |= bit; } internal void Clear(int bit) { data &= ~bit; } internal void Toggle(int bit) { data ^= bit; } /* * COPY_FLAG copies the value of flags from a source field * into a destination field. * * In the macro: * + "&flag" limits the outer xor operation to just the flag we're interested in. * + These are the results of the two xor operations: * * fieldDst fieldSrc inner xor outer xor * 0 0 0 0 * 0 1 1 1 * 1 0 1 0 * 1 1 0 1 */ internal void Copy(SimpleBitVector32 src, int bit) { data ^= (data ^ src.data) & bit; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
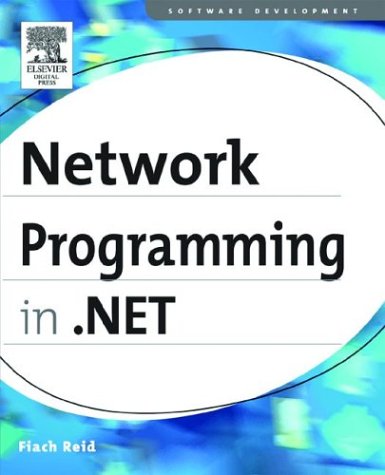
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncryptedData.cs
- AmbientLight.cs
- ObjectSecurity.cs
- HtmlMobileTextWriter.cs
- CommandEventArgs.cs
- WebControl.cs
- CatalogPartChrome.cs
- GeneralTransform.cs
- SmtpFailedRecipientException.cs
- GroupBox.cs
- TextRunProperties.cs
- InteropDesigner.xaml.cs
- WCFBuildProvider.cs
- TextDecorationCollection.cs
- ArraySegment.cs
- HtmlTextViewAdapter.cs
- ReadOnlyMetadataCollection.cs
- ProtocolsConfigurationHandler.cs
- DictionaryContent.cs
- ValidateNames.cs
- Operand.cs
- DataSvcMapFileSerializer.cs
- SessionEndingCancelEventArgs.cs
- ExternalException.cs
- ACL.cs
- StylusEventArgs.cs
- Typography.cs
- MultipleViewPattern.cs
- DataSourceXmlTextReader.cs
- PreviousTrackingServiceAttribute.cs
- TextParaClient.cs
- AutomationProperties.cs
- SHA512Managed.cs
- Literal.cs
- DetailsViewPagerRow.cs
- HelpInfo.cs
- TextChangedEventArgs.cs
- RegexTree.cs
- CultureTableRecord.cs
- PropertyChangedEventManager.cs
- IProvider.cs
- TrackingConditionCollection.cs
- DataTableReader.cs
- DependencyPropertyKind.cs
- SubMenuStyle.cs
- EditBehavior.cs
- BaseContextMenu.cs
- FormsAuthenticationConfiguration.cs
- HideDisabledControlAdapter.cs
- TextProperties.cs
- DashStyle.cs
- WebPartVerbsEventArgs.cs
- RequestStatusBarUpdateEventArgs.cs
- CompilerScope.cs
- MouseCaptureWithinProperty.cs
- SoapTypeAttribute.cs
- BuilderInfo.cs
- DataGridViewCellConverter.cs
- infer.cs
- SqlServices.cs
- XXXOnTypeBuilderInstantiation.cs
- MediaSystem.cs
- PrivilegeNotHeldException.cs
- TextBoxBase.cs
- DynamicILGenerator.cs
- WindowsProgressbar.cs
- XamlClipboardData.cs
- AsyncContentLoadedEventArgs.cs
- DPTypeDescriptorContext.cs
- TriggerActionCollection.cs
- LayoutDump.cs
- DataGridViewDataErrorEventArgs.cs
- XmlnsCompatibleWithAttribute.cs
- LoginName.cs
- Parameter.cs
- ResourceDictionary.cs
- BamlMapTable.cs
- InstanceCollisionException.cs
- ScrollableControl.cs
- HtmlDocument.cs
- WorkflowTransactionService.cs
- ResourceKey.cs
- Point3DConverter.cs
- HttpProcessUtility.cs
- jithelpers.cs
- StreamAsIStream.cs
- DelayedRegex.cs
- InvalidAsynchronousStateException.cs
- CustomCategoryAttribute.cs
- FixedLineResult.cs
- ResourceDisplayNameAttribute.cs
- BatchWriter.cs
- BooleanExpr.cs
- StrongName.cs
- InProcStateClientManager.cs
- XmlCodeExporter.cs
- ObjectList.cs
- IntegerValidator.cs
- ComponentChangedEvent.cs
- SQLConvert.cs