Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / BatchWriter.cs / 2 / BatchWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class for DataWeb services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System; using System.Diagnostics; using System.IO; #endregion Namespaces. ////// Static helper class to write responses for batch requests /// internal static class BatchWriter { ////// Writes the start of the changeset response /// /// writer to which the response needs to be written /// batch boundary /// changeset boundary internal static void WriteStartBatchBoundary(StreamWriter writer, string batchBoundary, string changesetBoundary) { WriterStartBoundary(writer, batchBoundary); writer.WriteLine( "{0}: {1}; {2}={3}", XmlConstants.HttpContentType, XmlConstants.MimeMultiPartMixed, XmlConstants.HttpMultipartBoundary, changesetBoundary); writer.WriteLine(); // NewLine to seperate the header from message } ///Write the boundary and header information. /// writer to which the response needs to be written /// host containing the value of the response headers /// boundary string that needs to be written internal static void WriteBoundaryAndHeaders(StreamWriter writer, IDataServiceHost host, string boundary) { Debug.Assert(writer != null, "writer != null"); Debug.Assert(host != null, "host != null"); Debug.Assert(boundary != null, "boundary != null"); WriterStartBoundary(writer, boundary); // First write the headers to indicate that the payload below is a http request WriteHeaderValue(writer, XmlConstants.HttpContentType, XmlConstants.MimeApplicationHttp); WriteHeaderValue(writer, XmlConstants.HttpContentTransferEncoding, XmlConstants.BatchRequestContentTransferEncoding); writer.WriteLine(); // NewLine to seperate the batch headers from http headers // In error cases, we create a dummy host, which has no request header information. // Hence we need to handle the case here. writer.WriteLine("{0} {1} {2}", XmlConstants.HttpVersionInBatching, host.ResponseStatusCode, WebUtil.GetStatusCodeText(host.ResponseStatusCode)); BatchServiceHost batch = (BatchServiceHost)host; if (null != batch.ContentId) { WriteHeaderValue(writer, XmlConstants.HttpContentID, batch.ContentId); } WriteHeaderValue(writer, XmlConstants.HttpContentType, host.ResponseContentType); WriteHeaderValue(writer, XmlConstants.HttpResponseCacheControl, host.ResponseCacheControl); WriteHeaderValue(writer, XmlConstants.HttpResponseETag, host.ResponseETag); WriteHeaderValue(writer, XmlConstants.HttpResponseLocation, host.ResponseLocation); WriteHeaderValue(writer, XmlConstants.HttpDataServiceVersion, host.ResponseVersion); BatchServiceHost batchHost = host as BatchServiceHost; Debug.Assert(batchHost != null, "batchHost != null -- only batch host currently supported."); WriteHeaderValue(writer, XmlConstants.HttpResponseAllow, batchHost.ResponseAllowHeader); writer.WriteLine(); // NewLine to seperate the header from message } ////// Write the end boundary /// /// writer to which the response needs to be written /// end boundary string. internal static void WriteEndBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}--", boundary); } ////// Write the start boundary /// /// writer to which the response needs to be written /// boundary string. private static void WriterStartBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}", boundary); } ////// Write the header name and value /// /// writer to which the response needs to be written /// name of the header whose value needs to be written. /// value of the header that needs to be written. private static void WriteHeaderValue(StreamWriter writer, string headerName, object headerValue) { if (headerValue != null) { string text = Convert.ToString(headerValue, System.Globalization.CultureInfo.InvariantCulture); if (!String.IsNullOrEmpty(text)) { writer.WriteLine("{0}: {1}", headerName, text); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class for DataWeb services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System; using System.Diagnostics; using System.IO; #endregion Namespaces. ////// Static helper class to write responses for batch requests /// internal static class BatchWriter { ////// Writes the start of the changeset response /// /// writer to which the response needs to be written /// batch boundary /// changeset boundary internal static void WriteStartBatchBoundary(StreamWriter writer, string batchBoundary, string changesetBoundary) { WriterStartBoundary(writer, batchBoundary); writer.WriteLine( "{0}: {1}; {2}={3}", XmlConstants.HttpContentType, XmlConstants.MimeMultiPartMixed, XmlConstants.HttpMultipartBoundary, changesetBoundary); writer.WriteLine(); // NewLine to seperate the header from message } ///Write the boundary and header information. /// writer to which the response needs to be written /// host containing the value of the response headers /// boundary string that needs to be written internal static void WriteBoundaryAndHeaders(StreamWriter writer, IDataServiceHost host, string boundary) { Debug.Assert(writer != null, "writer != null"); Debug.Assert(host != null, "host != null"); Debug.Assert(boundary != null, "boundary != null"); WriterStartBoundary(writer, boundary); // First write the headers to indicate that the payload below is a http request WriteHeaderValue(writer, XmlConstants.HttpContentType, XmlConstants.MimeApplicationHttp); WriteHeaderValue(writer, XmlConstants.HttpContentTransferEncoding, XmlConstants.BatchRequestContentTransferEncoding); writer.WriteLine(); // NewLine to seperate the batch headers from http headers // In error cases, we create a dummy host, which has no request header information. // Hence we need to handle the case here. writer.WriteLine("{0} {1} {2}", XmlConstants.HttpVersionInBatching, host.ResponseStatusCode, WebUtil.GetStatusCodeText(host.ResponseStatusCode)); BatchServiceHost batch = (BatchServiceHost)host; if (null != batch.ContentId) { WriteHeaderValue(writer, XmlConstants.HttpContentID, batch.ContentId); } WriteHeaderValue(writer, XmlConstants.HttpContentType, host.ResponseContentType); WriteHeaderValue(writer, XmlConstants.HttpResponseCacheControl, host.ResponseCacheControl); WriteHeaderValue(writer, XmlConstants.HttpResponseETag, host.ResponseETag); WriteHeaderValue(writer, XmlConstants.HttpResponseLocation, host.ResponseLocation); WriteHeaderValue(writer, XmlConstants.HttpDataServiceVersion, host.ResponseVersion); BatchServiceHost batchHost = host as BatchServiceHost; Debug.Assert(batchHost != null, "batchHost != null -- only batch host currently supported."); WriteHeaderValue(writer, XmlConstants.HttpResponseAllow, batchHost.ResponseAllowHeader); writer.WriteLine(); // NewLine to seperate the header from message } ////// Write the end boundary /// /// writer to which the response needs to be written /// end boundary string. internal static void WriteEndBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}--", boundary); } ////// Write the start boundary /// /// writer to which the response needs to be written /// boundary string. private static void WriterStartBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}", boundary); } ////// Write the header name and value /// /// writer to which the response needs to be written /// name of the header whose value needs to be written. /// value of the header that needs to be written. private static void WriteHeaderValue(StreamWriter writer, string headerName, object headerValue) { if (headerValue != null) { string text = Convert.ToString(headerValue, System.Globalization.CultureInfo.InvariantCulture); if (!String.IsNullOrEmpty(text)) { writer.WriteLine("{0}: {1}", headerName, text); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
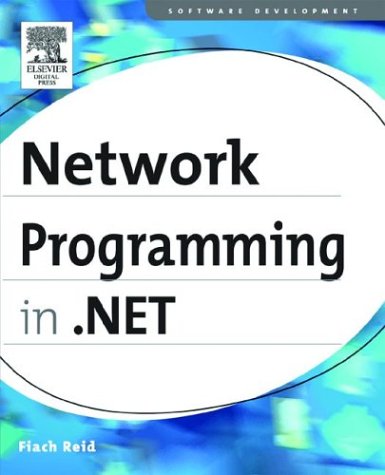
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Parser.cs
- ReverseInheritProperty.cs
- ArrayElementGridEntry.cs
- DataServiceRequestArgs.cs
- TextRangeSerialization.cs
- DataTemplateSelector.cs
- DynamicControlParameter.cs
- CurrencyManager.cs
- TextEditorCharacters.cs
- EntityDataSourceReferenceGroup.cs
- TypeDelegator.cs
- FrameDimension.cs
- FrameworkTemplate.cs
- DataGridViewCellCancelEventArgs.cs
- FilterableAttribute.cs
- DetailsViewUpdatedEventArgs.cs
- IntegrationExceptionEventArgs.cs
- MessageProperties.cs
- PositiveTimeSpanValidator.cs
- VariableAction.cs
- ClonableStack.cs
- DrawingContextWalker.cs
- TypeExtension.cs
- ScrollBarAutomationPeer.cs
- SettingsProviderCollection.cs
- ContourSegment.cs
- DurationConverter.cs
- SHA384CryptoServiceProvider.cs
- MediaSystem.cs
- handlecollector.cs
- TableStyle.cs
- SiteMapNode.cs
- KeyValuePairs.cs
- HandlerFactoryWrapper.cs
- ComplexTypeEmitter.cs
- CryptoKeySecurity.cs
- ToolboxItemCollection.cs
- EventSinkHelperWriter.cs
- DbCommandDefinition.cs
- HtmlSelect.cs
- storepermission.cs
- ParseNumbers.cs
- uribuilder.cs
- DataErrorValidationRule.cs
- WebServiceMethodData.cs
- DataStreams.cs
- KeyProperty.cs
- FixedPosition.cs
- ObservableDictionary.cs
- WinEventHandler.cs
- UserInitiatedNavigationPermission.cs
- DataPagerFieldItem.cs
- TileModeValidation.cs
- HelloOperation11AsyncResult.cs
- WebConfigurationManager.cs
- OlePropertyStructs.cs
- HandlerFactoryCache.cs
- ConfigXmlWhitespace.cs
- LeafCellTreeNode.cs
- RequiredAttributeAttribute.cs
- ProgressiveCrcCalculatingStream.cs
- Directory.cs
- OrderedHashRepartitionStream.cs
- PanelStyle.cs
- ConvertersCollection.cs
- BufferBuilder.cs
- XmlC14NWriter.cs
- ProfilePropertySettings.cs
- CollectionBuilder.cs
- AutomationIdentifier.cs
- ComboBoxRenderer.cs
- ProxyAssemblyNotLoadedException.cs
- SiteMap.cs
- WaitHandle.cs
- QueryNode.cs
- ExpandoClass.cs
- MappingException.cs
- documentsequencetextview.cs
- PropertyDescriptorComparer.cs
- AlternateView.cs
- RawStylusSystemGestureInputReport.cs
- SqlDataAdapter.cs
- FontDialog.cs
- DependencyObjectProvider.cs
- LinqDataSourceView.cs
- XmlEntityReference.cs
- SqlLiftIndependentRowExpressions.cs
- BuiltInExpr.cs
- StretchValidation.cs
- MimeReflector.cs
- MembershipPasswordException.cs
- EntityRecordInfo.cs
- AccessedThroughPropertyAttribute.cs
- SmtpFailedRecipientsException.cs
- ConditionalAttribute.cs
- NativeCppClassAttribute.cs
- PerformanceCounterCategory.cs
- TextChangedEventArgs.cs
- HandledMouseEvent.cs
- NetSectionGroup.cs