Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / TextChangedEventArgs.cs / 1 / TextChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
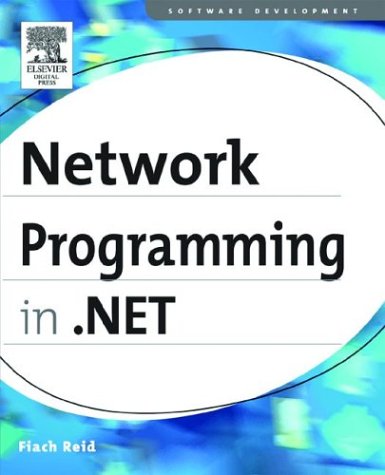
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeRecognizer.cs
- WebBrowsableAttribute.cs
- NeutralResourcesLanguageAttribute.cs
- FlatButtonAppearance.cs
- GroupBox.cs
- DynamicQueryableWrapper.cs
- ImageListUtils.cs
- TimeSpanParse.cs
- DataSourceCache.cs
- SessionStateSection.cs
- SqlClientFactory.cs
- StoreItemCollection.cs
- QilTypeChecker.cs
- autovalidator.cs
- Keywords.cs
- ScriptResourceDefinition.cs
- ObjectView.cs
- EndOfStreamException.cs
- RoleGroupCollection.cs
- EtwProvider.cs
- GiveFeedbackEventArgs.cs
- Enlistment.cs
- BasicHttpSecurityElement.cs
- NameScopePropertyAttribute.cs
- DataGridViewIntLinkedList.cs
- AvtEvent.cs
- PeoplePickerWrapper.cs
- Visual.cs
- StrokeDescriptor.cs
- EdmProviderManifest.cs
- DataServiceException.cs
- DesignerAttribute.cs
- DrawingAttributes.cs
- StreamingContext.cs
- Latin1Encoding.cs
- TraceUtils.cs
- ButtonBase.cs
- DriveNotFoundException.cs
- QilStrConcatenator.cs
- CollectionView.cs
- HttpSessionStateWrapper.cs
- ParserStreamGeometryContext.cs
- ElementHostAutomationPeer.cs
- Transform.cs
- TableLayoutSettingsTypeConverter.cs
- ScopelessEnumAttribute.cs
- SystemThemeKey.cs
- NodeInfo.cs
- DeferredSelectedIndexReference.cs
- _ServiceNameStore.cs
- AQNBuilder.cs
- XmlNullResolver.cs
- SchemaInfo.cs
- SvcMapFile.cs
- InternalControlCollection.cs
- NotificationContext.cs
- XdrBuilder.cs
- PerfCounterSection.cs
- HttpUnhandledOperationInvoker.cs
- FileChangesMonitor.cs
- EntityModelSchemaGenerator.cs
- Random.cs
- IPAddressCollection.cs
- ScrollChrome.cs
- ConfigXmlText.cs
- ObjectParameter.cs
- XPathParser.cs
- TextEditor.cs
- Int32.cs
- TreeViewAutomationPeer.cs
- ModifierKeysConverter.cs
- UnicastIPAddressInformationCollection.cs
- StreamDocument.cs
- X509Chain.cs
- mediapermission.cs
- DragEvent.cs
- controlskin.cs
- TemplatedWizardStep.cs
- InvalidPrinterException.cs
- TraceSource.cs
- DBNull.cs
- PageHandlerFactory.cs
- HtmlContainerControl.cs
- XmlLanguage.cs
- OracleException.cs
- SafeFileHandle.cs
- InstanceKeyCollisionException.cs
- AspNetHostingPermission.cs
- MarkupProperty.cs
- RegexStringValidator.cs
- EventLogTraceListener.cs
- ModuleBuilder.cs
- Exception.cs
- GridViewRow.cs
- ViewBox.cs
- RuntimeHandles.cs
- TextReader.cs
- ToolStripLabel.cs
- RadioButtonRenderer.cs
- DynamicResourceExtensionConverter.cs