Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / Design / HelpKeywordAttribute.cs / 1 / HelpKeywordAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System; using System.Security.Permissions; ////// Allows specification of the context keyword that will be specified for this class or member. By default, /// the help keyword for a class is the Type's full name, and for a member it's the full name of the type that declared the property, /// plus the property name itself. /// /// For example, consider System.Windows.Forms.Button and it's Text property: /// /// The class keyword is "System.Windows.Forms.Button", but the Text property keyword is "System.Windows.Forms.Control.Text", because the Text /// property is declared on the System.Windows.Forms.Control class rather than the Button class itself; the Button class inherits the property. /// By contrast, the DialogResult property is declared on the Button so its keyword would be "System.Windows.Forms.Button.DialogResult". /// /// When the help system gets the keywords, it will first look at this attribute. At the class level, it will return the string specified by the /// HelpContextAttribute. Note this will not be used for members of the Type in question. They will still reflect the declaring Type's actual /// full name, plus the member name. To override this, place the attribute on the member itself. /// /// Example: /// /// [HelpKeywordAttribute(typeof(Component))] /// public class MyComponent : Component { /// /// /// public string Property1 { get{return "";}; /// /// [HelpKeywordAttribute("SomeNamespace.SomeOtherClass.Property2")] /// public string Property2 { get{return "";}; /// /// } /// /// /// For the above class (default without attribution): /// /// Class keyword: "System.ComponentModel.Component" ("MyNamespace.MyComponent') /// Property1 keyword: "MyNamespace.MyComponent.Property1" (default) /// Property2 keyword: "SomeNamespace.SomeOtherClass.Property2" ("MyNamespace.MyComponent.Property2") /// /// [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false)] [Serializable] public sealed class HelpKeywordAttribute : Attribute { ////// Default value for HelpKeywordAttribute, which is null. /// public static readonly HelpKeywordAttribute Default = new HelpKeywordAttribute(); private string contextKeyword; ////// Default constructor, which creates an attribute with a null HelpKeyword. /// public HelpKeywordAttribute() { } ////// Creates a HelpKeywordAttribute with the value being the given keyword string. /// public HelpKeywordAttribute(string keyword) { if (keyword == null) { throw new ArgumentNullException("keyword"); } this.contextKeyword = keyword; } ////// Creates a HelpKeywordAttribute with the value being the full name of the given type. /// public HelpKeywordAttribute(Type t) { if (t == null) { throw new ArgumentNullException("t"); } this.contextKeyword = t.FullName; } ////// Retrieves the HelpKeyword this attribute supplies. /// public string HelpKeyword { get { return contextKeyword; } } ////// Two instances of a HelpKeywordAttribute are equal if they're HelpKeywords are equal. /// public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is HelpKeywordAttribute)) { return ((HelpKeywordAttribute)obj).HelpKeyword == HelpKeyword; } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns true if this Attribute's HelpKeyword is null. /// public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System; using System.Security.Permissions; ////// Allows specification of the context keyword that will be specified for this class or member. By default, /// the help keyword for a class is the Type's full name, and for a member it's the full name of the type that declared the property, /// plus the property name itself. /// /// For example, consider System.Windows.Forms.Button and it's Text property: /// /// The class keyword is "System.Windows.Forms.Button", but the Text property keyword is "System.Windows.Forms.Control.Text", because the Text /// property is declared on the System.Windows.Forms.Control class rather than the Button class itself; the Button class inherits the property. /// By contrast, the DialogResult property is declared on the Button so its keyword would be "System.Windows.Forms.Button.DialogResult". /// /// When the help system gets the keywords, it will first look at this attribute. At the class level, it will return the string specified by the /// HelpContextAttribute. Note this will not be used for members of the Type in question. They will still reflect the declaring Type's actual /// full name, plus the member name. To override this, place the attribute on the member itself. /// /// Example: /// /// [HelpKeywordAttribute(typeof(Component))] /// public class MyComponent : Component { /// /// /// public string Property1 { get{return "";}; /// /// [HelpKeywordAttribute("SomeNamespace.SomeOtherClass.Property2")] /// public string Property2 { get{return "";}; /// /// } /// /// /// For the above class (default without attribution): /// /// Class keyword: "System.ComponentModel.Component" ("MyNamespace.MyComponent') /// Property1 keyword: "MyNamespace.MyComponent.Property1" (default) /// Property2 keyword: "SomeNamespace.SomeOtherClass.Property2" ("MyNamespace.MyComponent.Property2") /// /// [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false)] [Serializable] public sealed class HelpKeywordAttribute : Attribute { ////// Default value for HelpKeywordAttribute, which is null. /// public static readonly HelpKeywordAttribute Default = new HelpKeywordAttribute(); private string contextKeyword; ////// Default constructor, which creates an attribute with a null HelpKeyword. /// public HelpKeywordAttribute() { } ////// Creates a HelpKeywordAttribute with the value being the given keyword string. /// public HelpKeywordAttribute(string keyword) { if (keyword == null) { throw new ArgumentNullException("keyword"); } this.contextKeyword = keyword; } ////// Creates a HelpKeywordAttribute with the value being the full name of the given type. /// public HelpKeywordAttribute(Type t) { if (t == null) { throw new ArgumentNullException("t"); } this.contextKeyword = t.FullName; } ////// Retrieves the HelpKeyword this attribute supplies. /// public string HelpKeyword { get { return contextKeyword; } } ////// Two instances of a HelpKeywordAttribute are equal if they're HelpKeywords are equal. /// public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is HelpKeywordAttribute)) { return ((HelpKeywordAttribute)obj).HelpKeyword == HelpKeyword; } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns true if this Attribute's HelpKeyword is null. /// public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
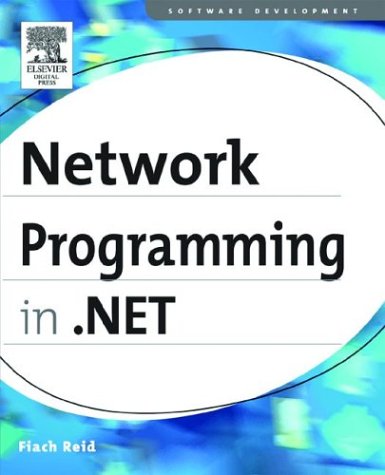
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlTitle.cs
- ExpandableObjectConverter.cs
- NumberFunctions.cs
- MouseActionValueSerializer.cs
- HasActivatableWorkflowEvent.cs
- XPathAncestorIterator.cs
- ping.cs
- CryptoKeySecurity.cs
- EntityDataReader.cs
- CodeVariableDeclarationStatement.cs
- SystemIPv4InterfaceProperties.cs
- ZipPackage.cs
- SimpleApplicationHost.cs
- DesignerDataTableBase.cs
- ContextBase.cs
- ConnectorEditor.cs
- SerializerWriterEventHandlers.cs
- BuildDependencySet.cs
- BufferBuilder.cs
- PageVisual.cs
- SoapIgnoreAttribute.cs
- BuildResultCache.cs
- Solver.cs
- WriteLine.cs
- grammarelement.cs
- XpsFixedDocumentReaderWriter.cs
- HelpProvider.cs
- oledbmetadatacolumnnames.cs
- XmlWrappingReader.cs
- SuppressIldasmAttribute.cs
- FlowDecisionLabelFeature.cs
- CompilationUnit.cs
- ReachFixedDocumentSerializer.cs
- SqlUtils.cs
- RenderData.cs
- RotationValidation.cs
- ContextConfiguration.cs
- EntityProviderFactory.cs
- Label.cs
- TreeViewDesigner.cs
- x509store.cs
- Mutex.cs
- ComPlusInstanceContextInitializer.cs
- ValueChangedEventManager.cs
- EnumDataContract.cs
- DataSpaceManager.cs
- SatelliteContractVersionAttribute.cs
- SynchronizationLockException.cs
- CompModSwitches.cs
- RepeaterCommandEventArgs.cs
- OdbcErrorCollection.cs
- DataGridViewLinkCell.cs
- TimeSpanParse.cs
- graph.cs
- SchemaElement.cs
- DataGridHeaderBorder.cs
- MdiWindowListStrip.cs
- ToolStripDropTargetManager.cs
- ContentDesigner.cs
- OleDbCommand.cs
- XmlConvert.cs
- InputBindingCollection.cs
- ControlUtil.cs
- WSSecurityPolicy12.cs
- CodeIdentifier.cs
- ProtocolsConfigurationEntry.cs
- NeutralResourcesLanguageAttribute.cs
- SqlStream.cs
- DatatypeImplementation.cs
- updatecommandorderer.cs
- InfiniteIntConverter.cs
- FunctionDescription.cs
- BidPrivateBase.cs
- FileDetails.cs
- ListViewGroupItemCollection.cs
- WebSysDisplayNameAttribute.cs
- AccessibleObject.cs
- ImageSource.cs
- PlatformCulture.cs
- GlyphManager.cs
- XmlNodeChangedEventArgs.cs
- RadialGradientBrush.cs
- EntityContainerEmitter.cs
- TripleDES.cs
- SectionInput.cs
- ListControlActionList.cs
- CompensatableSequenceActivity.cs
- CatalogZoneBase.cs
- TraversalRequest.cs
- AsnEncodedData.cs
- HostExecutionContextManager.cs
- EmissiveMaterial.cs
- FixedTextBuilder.cs
- SqlErrorCollection.cs
- StylusCollection.cs
- OracleBoolean.cs
- SystemKeyConverter.cs
- ProvidersHelper.cs
- ParameterModifier.cs
- Geometry3D.cs