Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmSyndicationContentSerializer.cs / 1305376 / EpmSyndicationContentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Classes used for serializing EntityPropertyMappingAttribute content for // syndication specific mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Data.Services.Providers; using System.Data.Services.Serializers; using System.Globalization; using System.ServiceModel.Syndication; using System.Diagnostics; #endregion ////// Base visitor class for performing serialization of syndication specific content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmSyndicationContentSerializer : EpmContentSerializerBase, IDisposable { ////// Syndication author object resulting from mapping /// private SyndicationPerson author; ////// Is author mapping provided in the target tree /// private bool authorInfoPresent; ////// Is author name provided in the target tree /// private bool authorNamePresent; ///Collection of null valued properties for this current serialization private EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties; ////// Constructor initializes the base class be identifying itself as a syndication serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added /// Null valued properties found during serialization internal EpmSyndicationContentSerializer(EpmTargetTree tree, object element, SyndicationItem target, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties) : base(tree, true, element, target) { this.nullValuedProperties = nullValuedProperties; } ////// Used for housecleaning once every node has been visited, creates an author syndication element if none has been /// created by the visitor /// public void Dispose() { this.CreateAuthor(true); if (!this.authorNamePresent) { this.author.Name = String.Empty; this.authorNamePresent = true; } this.Target.Authors.Add(this.author); } ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize /// Data Service provider used for rights verification. protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) { if (targetSegment.HasContent) { EntityPropertyMappingInfo epmInfo = targetSegment.EpmInfo; Object propertyValue; try { propertyValue = epmInfo.PropValReader.DynamicInvoke(this.Element, provider); } catch (System.Reflection.TargetInvocationException e) { ErrorHandler.HandleTargetInvocationException(e); throw; } if (propertyValue == null) { this.nullValuedProperties.Add(epmInfo); } String textPropertyValue = propertyValue != null ? PlainXmlSerializer.PrimitiveToString(propertyValue) : String.Empty; TextSyndicationContentKind contentKind = (TextSyndicationContentKind)epmInfo.Attribute.TargetTextContentKind; switch (epmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: this.CreateAuthor(false); this.author.Email = textPropertyValue; break; case SyndicationItemProperty.AuthorName: this.CreateAuthor(false); this.author.Name = textPropertyValue; this.authorNamePresent = true; break; case SyndicationItemProperty.AuthorUri: this.CreateAuthor(false); this.author.Uri = textPropertyValue; break; case SyndicationItemProperty.ContributorEmail: case SyndicationItemProperty.ContributorName: case SyndicationItemProperty.ContributorUri: this.SetContributorProperty(epmInfo.Attribute.TargetSyndicationItem, textPropertyValue); break; case SyndicationItemProperty.Updated: this.Target.LastUpdatedTime = EpmSyndicationContentSerializer.GetDate(propertyValue, Strings.EpmSerializer_UpdatedHasWrongType); break; case SyndicationItemProperty.Published: this.Target.PublishDate = EpmSyndicationContentSerializer.GetDate(propertyValue, Strings.EpmSerializer_PublishedHasWrongType); break; case SyndicationItemProperty.Rights: this.Target.Copyright = new TextSyndicationContent(textPropertyValue, contentKind); break; case SyndicationItemProperty.Summary: this.Target.Summary = new TextSyndicationContent(textPropertyValue, contentKind); break; case SyndicationItemProperty.Title: this.Target.Title = new TextSyndicationContent(textPropertyValue, contentKind); break; default: Debug.Fail("Unhandled SyndicationItemProperty enum value - should never get here."); break; } } else { base.Serialize(targetSegment, kind, provider); } } ////// Given an object returns the corresponding DateTimeOffset value through conversions /// /// Object containing property value /// Message of the thrown exception if thecannot be converted to DateTimeOffset. /// DateTimeOffset after conversion private static DateTimeOffset GetDate(object propertyValue, string exceptionMsg) { if (propertyValue == null) { return DateTimeOffset.Now; } DateTimeOffset date; if (propertyValue is DateTimeOffset) { date = (DateTimeOffset)propertyValue; } else if (propertyValue is DateTime) { // DateTimeOffset takes care of DateTimes of Unspecified kind so we won't end up // with datetime without timezone info mapped to atom:updated or atom:published element. date = new DateTimeOffset((DateTime)propertyValue); } else if (propertyValue is String) { if (!DateTimeOffset.TryParse((String)propertyValue, out date)) { DateTime result; if (!DateTime.TryParse((String)propertyValue, out result)) { throw new DataServiceException(500, null, exceptionMsg, null, null); } date = new DateTimeOffset(result); } } else { try { date = new DateTimeOffset(Convert.ToDateTime(propertyValue, CultureInfo.InvariantCulture)); } catch (Exception e) { throw new DataServiceException(500, null, exceptionMsg, null, e); } } return date; } ////// Creates a new author syndication specific object /// /// Whether to create a null author or a non-null author private void CreateAuthor(bool createNull) { if (!this.authorInfoPresent) { this.author = createNull ? new SyndicationPerson(null, String.Empty, null) : new SyndicationPerson(); this.authorInfoPresent = true; } } ////// Set a property of atom:contributor element. atom:contributor is created if does not exist. /// /// Property to be set. /// Value of the property. private void SetContributorProperty(SyndicationItemProperty propertyToSet, string textPropertyValue) { if (this.Target.Contributors.Count == 0) { this.Target.Contributors.Add(new SyndicationPerson()); } // We can have at most one contributor switch (propertyToSet) { case SyndicationItemProperty.ContributorEmail: this.Target.Contributors[0].Email = textPropertyValue; break; case SyndicationItemProperty.ContributorName: this.Target.Contributors[0].Name = textPropertyValue; break; case SyndicationItemProperty.ContributorUri: this.Target.Contributors[0].Uri = textPropertyValue; break; default: Debug.Fail("propertyToSet is not a Contributor property."); break; } Debug.Assert(this.Target.Contributors.Count == 1, "There should be one and only one contributor."); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Classes used for serializing EntityPropertyMappingAttribute content for // syndication specific mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Data.Services.Providers; using System.Data.Services.Serializers; using System.Globalization; using System.ServiceModel.Syndication; using System.Diagnostics; #endregion ////// Base visitor class for performing serialization of syndication specific content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmSyndicationContentSerializer : EpmContentSerializerBase, IDisposable { ////// Syndication author object resulting from mapping /// private SyndicationPerson author; ////// Is author mapping provided in the target tree /// private bool authorInfoPresent; ////// Is author name provided in the target tree /// private bool authorNamePresent; ///Collection of null valued properties for this current serialization private EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties; ////// Constructor initializes the base class be identifying itself as a syndication serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added /// Null valued properties found during serialization internal EpmSyndicationContentSerializer(EpmTargetTree tree, object element, SyndicationItem target, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties) : base(tree, true, element, target) { this.nullValuedProperties = nullValuedProperties; } ////// Used for housecleaning once every node has been visited, creates an author syndication element if none has been /// created by the visitor /// public void Dispose() { this.CreateAuthor(true); if (!this.authorNamePresent) { this.author.Name = String.Empty; this.authorNamePresent = true; } this.Target.Authors.Add(this.author); } ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize /// Data Service provider used for rights verification. protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) { if (targetSegment.HasContent) { EntityPropertyMappingInfo epmInfo = targetSegment.EpmInfo; Object propertyValue; try { propertyValue = epmInfo.PropValReader.DynamicInvoke(this.Element, provider); } catch (System.Reflection.TargetInvocationException e) { ErrorHandler.HandleTargetInvocationException(e); throw; } if (propertyValue == null) { this.nullValuedProperties.Add(epmInfo); } String textPropertyValue = propertyValue != null ? PlainXmlSerializer.PrimitiveToString(propertyValue) : String.Empty; TextSyndicationContentKind contentKind = (TextSyndicationContentKind)epmInfo.Attribute.TargetTextContentKind; switch (epmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: this.CreateAuthor(false); this.author.Email = textPropertyValue; break; case SyndicationItemProperty.AuthorName: this.CreateAuthor(false); this.author.Name = textPropertyValue; this.authorNamePresent = true; break; case SyndicationItemProperty.AuthorUri: this.CreateAuthor(false); this.author.Uri = textPropertyValue; break; case SyndicationItemProperty.ContributorEmail: case SyndicationItemProperty.ContributorName: case SyndicationItemProperty.ContributorUri: this.SetContributorProperty(epmInfo.Attribute.TargetSyndicationItem, textPropertyValue); break; case SyndicationItemProperty.Updated: this.Target.LastUpdatedTime = EpmSyndicationContentSerializer.GetDate(propertyValue, Strings.EpmSerializer_UpdatedHasWrongType); break; case SyndicationItemProperty.Published: this.Target.PublishDate = EpmSyndicationContentSerializer.GetDate(propertyValue, Strings.EpmSerializer_PublishedHasWrongType); break; case SyndicationItemProperty.Rights: this.Target.Copyright = new TextSyndicationContent(textPropertyValue, contentKind); break; case SyndicationItemProperty.Summary: this.Target.Summary = new TextSyndicationContent(textPropertyValue, contentKind); break; case SyndicationItemProperty.Title: this.Target.Title = new TextSyndicationContent(textPropertyValue, contentKind); break; default: Debug.Fail("Unhandled SyndicationItemProperty enum value - should never get here."); break; } } else { base.Serialize(targetSegment, kind, provider); } } ////// Given an object returns the corresponding DateTimeOffset value through conversions /// /// Object containing property value /// Message of the thrown exception if thecannot be converted to DateTimeOffset. /// DateTimeOffset after conversion private static DateTimeOffset GetDate(object propertyValue, string exceptionMsg) { if (propertyValue == null) { return DateTimeOffset.Now; } DateTimeOffset date; if (propertyValue is DateTimeOffset) { date = (DateTimeOffset)propertyValue; } else if (propertyValue is DateTime) { // DateTimeOffset takes care of DateTimes of Unspecified kind so we won't end up // with datetime without timezone info mapped to atom:updated or atom:published element. date = new DateTimeOffset((DateTime)propertyValue); } else if (propertyValue is String) { if (!DateTimeOffset.TryParse((String)propertyValue, out date)) { DateTime result; if (!DateTime.TryParse((String)propertyValue, out result)) { throw new DataServiceException(500, null, exceptionMsg, null, null); } date = new DateTimeOffset(result); } } else { try { date = new DateTimeOffset(Convert.ToDateTime(propertyValue, CultureInfo.InvariantCulture)); } catch (Exception e) { throw new DataServiceException(500, null, exceptionMsg, null, e); } } return date; } ////// Creates a new author syndication specific object /// /// Whether to create a null author or a non-null author private void CreateAuthor(bool createNull) { if (!this.authorInfoPresent) { this.author = createNull ? new SyndicationPerson(null, String.Empty, null) : new SyndicationPerson(); this.authorInfoPresent = true; } } ////// Set a property of atom:contributor element. atom:contributor is created if does not exist. /// /// Property to be set. /// Value of the property. private void SetContributorProperty(SyndicationItemProperty propertyToSet, string textPropertyValue) { if (this.Target.Contributors.Count == 0) { this.Target.Contributors.Add(new SyndicationPerson()); } // We can have at most one contributor switch (propertyToSet) { case SyndicationItemProperty.ContributorEmail: this.Target.Contributors[0].Email = textPropertyValue; break; case SyndicationItemProperty.ContributorName: this.Target.Contributors[0].Name = textPropertyValue; break; case SyndicationItemProperty.ContributorUri: this.Target.Contributors[0].Uri = textPropertyValue; break; default: Debug.Fail("propertyToSet is not a Contributor property."); break; } Debug.Assert(this.Target.Contributors.Count == 1, "There should be one and only one contributor."); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
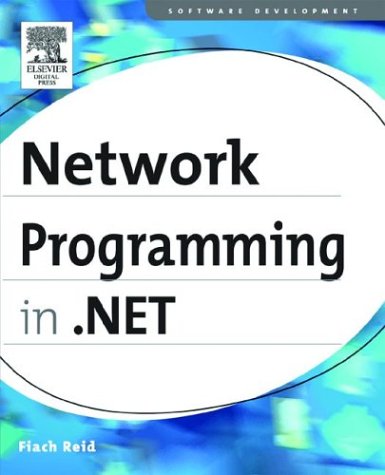
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SSmlParser.cs
- control.ime.cs
- EllipticalNodeOperations.cs
- TypeLibraryHelper.cs
- FlowDocumentScrollViewer.cs
- XPathMessageFilterElementCollection.cs
- Binding.cs
- SmiRecordBuffer.cs
- GenericXmlSecurityTokenAuthenticator.cs
- PerfCounters.cs
- FirewallWrapper.cs
- MemberAccessException.cs
- OracleEncoding.cs
- WebServiceErrorEvent.cs
- TextLineBreak.cs
- TypeRefElement.cs
- ProfileServiceManager.cs
- UnknownBitmapDecoder.cs
- UnsafeNativeMethods.cs
- SectionInformation.cs
- XmlTextEncoder.cs
- ConfigUtil.cs
- TargetFrameworkUtil.cs
- ItemCheckedEvent.cs
- TraceHwndHost.cs
- HierarchicalDataTemplate.cs
- PropertyCollection.cs
- SystemColors.cs
- ResourceManager.cs
- PropertyFilter.cs
- QilLoop.cs
- PropertyItemInternal.cs
- ProcessHost.cs
- PersonalizableAttribute.cs
- DbConnectionHelper.cs
- XComponentModel.cs
- PhysicalFontFamily.cs
- MembershipValidatePasswordEventArgs.cs
- CodeDesigner.cs
- ProviderCommandInfoUtils.cs
- ColorEditor.cs
- HideDisabledControlAdapter.cs
- CheckBoxAutomationPeer.cs
- IndicFontClient.cs
- HtmlInputButton.cs
- DefaultObjectMappingItemCollection.cs
- Version.cs
- DispatcherExceptionFilterEventArgs.cs
- RootNamespaceAttribute.cs
- NumericUpDownAcceleration.cs
- RectAnimation.cs
- PolyQuadraticBezierSegment.cs
- WizardPanel.cs
- UpDownBase.cs
- RestClientProxyHandler.cs
- ZipIOExtraFieldZip64Element.cs
- IApplicationTrustManager.cs
- _DisconnectOverlappedAsyncResult.cs
- ProfileManager.cs
- HtmlForm.cs
- TrackBar.cs
- VirtualizingStackPanel.cs
- DetailsViewDeleteEventArgs.cs
- CreateUserWizardStep.cs
- ObjectStateManagerMetadata.cs
- DataRowExtensions.cs
- ThousandthOfEmRealPoints.cs
- PrintPreviewDialog.cs
- LoadItemsEventArgs.cs
- RectConverter.cs
- followingquery.cs
- FrameSecurityDescriptor.cs
- XmlEncodedRawTextWriter.cs
- EDesignUtil.cs
- XamlSerializer.cs
- MD5.cs
- ValidateNames.cs
- ContextStack.cs
- XmlNamespaceDeclarationsAttribute.cs
- HScrollProperties.cs
- XmlSchemaAttributeGroup.cs
- CancellationTokenRegistration.cs
- ResizingMessageFilter.cs
- QueryExpr.cs
- StreamReader.cs
- TypeConverterHelper.cs
- PackageRelationshipSelector.cs
- XPathScanner.cs
- EntityDataSourceWrapper.cs
- Funcletizer.cs
- InvalidEnumArgumentException.cs
- LifetimeServices.cs
- AttributeCollection.cs
- SqlDependency.cs
- DataGridCaption.cs
- ActivityExecutionFilter.cs
- StretchValidation.cs
- TextElementEnumerator.cs
- ListViewInsertionMark.cs
- SqlFacetAttribute.cs