Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Runtime / Serialization / ObjectToIdCache.cs / 1305376 / ObjectToIdCache.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Serialization { using System.Collections; using System.Collections.Generic; using System.Runtime.CompilerServices; using System.Security; class ObjectToIdCache { internal int m_currentCount; internal int []m_ids; internal Object []m_objs; public ObjectToIdCache() { m_currentCount=1; m_ids = new int[GetPrime(1)]; m_objs = new Object[m_ids.Length]; } public int GetId(object obj, ref bool newId) { bool isEmpty; int pos = FindElement(obj, out isEmpty); if(!isEmpty) { newId = false; return m_ids[pos]; } if(!newId) return -1; int id = m_currentCount++; m_objs[pos]=obj; m_ids[pos]= id; if (m_currentCount >= (m_objs.Length-1)) Rehash(); return id; } #if NotUsed public bool Remove(object obj) { bool isEmpty; int pos = FindElement(obj, out isEmpty); if(isEmpty) return false; RemoveAt(pos); return true; } #endif // (oldObjId, oldObj-id, newObj-newObjId) => (oldObj-oldObjId, newObj-id, newObjId ) public int ReassignId(int oldObjId, object oldObj, object newObj) { bool isEmpty; int pos = FindElement(oldObj, out isEmpty); if(isEmpty) return 0; int id = m_ids[pos]; if (oldObjId > 0) m_ids[pos] = oldObjId; else RemoveAt(pos); pos = FindElement(newObj, out isEmpty); int newObjId = 0; if (!isEmpty) newObjId = m_ids[pos]; m_objs[pos] = newObj; m_ids[pos] = id; return newObjId; } private int FindElement(object obj, out bool isEmpty) { int hashcode = RuntimeHelpers.GetHashCode(obj); int pos = ((hashcode&0x7FFFFFFF)%m_objs.Length); for(int i = pos; i != (pos-1); i++) { if (m_objs[i] == null) { isEmpty=true; return i; } if (m_objs[i] == obj) { isEmpty=false; return i; } if(i == (m_objs.Length-1)) i = -1; } // m_obj must ALWAYS have atleast one slot empty (null). Fx.Assert("Object table overflow"); throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ObjectTableOverflow))); } private void RemoveAt(int pos) { int hashcode = RuntimeHelpers.GetHashCode(m_objs[pos]); for (int i = pos, j;i != (pos-1); i = j) { j = (i+1) % m_objs.Length; if (m_objs[j] == null || RuntimeHelpers.GetHashCode(m_objs[j]) != hashcode) { m_objs[pos] = m_objs[i]; m_ids[pos] = m_ids[i]; m_objs[i] = null; m_ids[i] = 0; return; } } // m_obj must ALWAYS have atleast one slot empty (null). Fx.Assert("Object table overflow"); throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ObjectTableOverflow))); } private void Rehash() { int size = GetPrime(m_objs.Length * 2); int[] oldIds = m_ids; object[] oldObjs = m_objs; m_ids = new int[size]; m_objs = new Object[size]; for (int j=0; j= min) return prime; } //outside of our predefined table. //compute the hard way. for (int i = (min | 1); i < Int32.MaxValue;i+=2) { if (IsPrime(i)) return i; } return min; } static bool IsPrime(int candidate) { if ((candidate & 1) != 0) { int limit = (int)Math.Sqrt (candidate); for (int divisor = 3; divisor <= limit; divisor+=2) { if ((candidate % divisor) == 0) return false; } return true; } return (candidate == 2); } [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - Static fields are marked SecurityCritical or readonly to prevent" + " data from being modified or leaked to other components in appdomain.")] internal static readonly int[] primes = { 3, 7, 17, 37, 89, 197, 431, 919, 1931, 4049, 8419, 17519, 36353, 75431, 156437, 324449, 672827, 1395263, 2893249, 5999471, }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Serialization { using System.Collections; using System.Collections.Generic; using System.Runtime.CompilerServices; using System.Security; class ObjectToIdCache { internal int m_currentCount; internal int []m_ids; internal Object []m_objs; public ObjectToIdCache() { m_currentCount=1; m_ids = new int[GetPrime(1)]; m_objs = new Object[m_ids.Length]; } public int GetId(object obj, ref bool newId) { bool isEmpty; int pos = FindElement(obj, out isEmpty); if(!isEmpty) { newId = false; return m_ids[pos]; } if(!newId) return -1; int id = m_currentCount++; m_objs[pos]=obj; m_ids[pos]= id; if (m_currentCount >= (m_objs.Length-1)) Rehash(); return id; } #if NotUsed public bool Remove(object obj) { bool isEmpty; int pos = FindElement(obj, out isEmpty); if(isEmpty) return false; RemoveAt(pos); return true; } #endif // (oldObjId, oldObj-id, newObj-newObjId) => (oldObj-oldObjId, newObj-id, newObjId ) public int ReassignId(int oldObjId, object oldObj, object newObj) { bool isEmpty; int pos = FindElement(oldObj, out isEmpty); if(isEmpty) return 0; int id = m_ids[pos]; if (oldObjId > 0) m_ids[pos] = oldObjId; else RemoveAt(pos); pos = FindElement(newObj, out isEmpty); int newObjId = 0; if (!isEmpty) newObjId = m_ids[pos]; m_objs[pos] = newObj; m_ids[pos] = id; return newObjId; } private int FindElement(object obj, out bool isEmpty) { int hashcode = RuntimeHelpers.GetHashCode(obj); int pos = ((hashcode&0x7FFFFFFF)%m_objs.Length); for(int i = pos; i != (pos-1); i++) { if (m_objs[i] == null) { isEmpty=true; return i; } if (m_objs[i] == obj) { isEmpty=false; return i; } if(i == (m_objs.Length-1)) i = -1; } // m_obj must ALWAYS have atleast one slot empty (null). Fx.Assert("Object table overflow"); throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ObjectTableOverflow))); } private void RemoveAt(int pos) { int hashcode = RuntimeHelpers.GetHashCode(m_objs[pos]); for (int i = pos, j;i != (pos-1); i = j) { j = (i+1) % m_objs.Length; if (m_objs[j] == null || RuntimeHelpers.GetHashCode(m_objs[j]) != hashcode) { m_objs[pos] = m_objs[i]; m_ids[pos] = m_ids[i]; m_objs[i] = null; m_ids[i] = 0; return; } } // m_obj must ALWAYS have atleast one slot empty (null). Fx.Assert("Object table overflow"); throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ObjectTableOverflow))); } private void Rehash() { int size = GetPrime(m_objs.Length * 2); int[] oldIds = m_ids; object[] oldObjs = m_objs; m_ids = new int[size]; m_objs = new Object[size]; for (int j=0; j = min) return prime; } //outside of our predefined table. //compute the hard way. for (int i = (min | 1); i < Int32.MaxValue;i+=2) { if (IsPrime(i)) return i; } return min; } static bool IsPrime(int candidate) { if ((candidate & 1) != 0) { int limit = (int)Math.Sqrt (candidate); for (int divisor = 3; divisor <= limit; divisor+=2) { if ((candidate % divisor) == 0) return false; } return true; } return (candidate == 2); } [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview - Static fields are marked SecurityCritical or readonly to prevent" + " data from being modified or leaked to other components in appdomain.")] internal static readonly int[] primes = { 3, 7, 17, 37, 89, 197, 431, 919, 1931, 4049, 8419, 17519, 36353, 75431, 156437, 324449, 672827, 1395263, 2893249, 5999471, }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
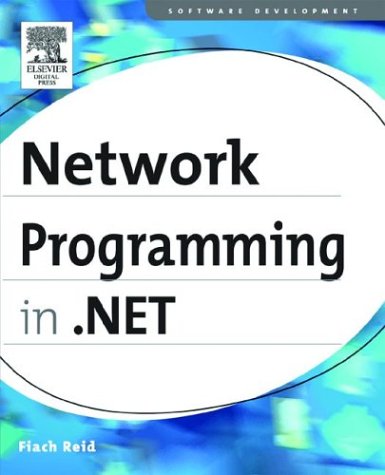
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollChrome.cs
- HtmlTitle.cs
- FileSystemInfo.cs
- CheckBox.cs
- ToggleButtonAutomationPeer.cs
- Executor.cs
- pingexception.cs
- LazyTextWriterCreator.cs
- ReceiveActivity.cs
- HexParser.cs
- BackStopAuthenticationModule.cs
- AtomServiceDocumentSerializer.cs
- LineGeometry.cs
- ContentPlaceHolder.cs
- WebPartDisplayMode.cs
- PtsHelper.cs
- Int32CollectionConverter.cs
- QueryCacheManager.cs
- Matrix3DValueSerializer.cs
- XmlSerializableWriter.cs
- MemoryFailPoint.cs
- TriggerCollection.cs
- TextElementCollection.cs
- MailSettingsSection.cs
- StatusBarPanel.cs
- HMACSHA384.cs
- OutputCacheSection.cs
- BitmapMetadataEnumerator.cs
- SecurityTokenReferenceStyle.cs
- SqlDataSourceTableQuery.cs
- DataSvcMapFile.cs
- Opcode.cs
- DocumentViewerBaseAutomationPeer.cs
- XmlReflectionMember.cs
- DescendantQuery.cs
- ServicePointManager.cs
- PenContext.cs
- WindowsIdentity.cs
- HtmlSelect.cs
- InheritanceRules.cs
- Page.cs
- Point3DKeyFrameCollection.cs
- TypeSemantics.cs
- QuotedPairReader.cs
- FileDialogPermission.cs
- ObservableCollection.cs
- MultipartContentParser.cs
- XPathNodeList.cs
- UIPropertyMetadata.cs
- Border.cs
- FormsAuthenticationTicket.cs
- ViewStateModeByIdAttribute.cs
- FormView.cs
- SHA384.cs
- HttpWriter.cs
- SyndicationFeedFormatter.cs
- Single.cs
- ClientScriptManagerWrapper.cs
- SqlRetyper.cs
- ResXDataNode.cs
- DataGridItem.cs
- QueryOptionExpression.cs
- SoapIgnoreAttribute.cs
- DesignerToolStripControlHost.cs
- TriggerCollection.cs
- XsltQilFactory.cs
- SubpageParaClient.cs
- _SecureChannel.cs
- WinEventHandler.cs
- ObjectContextServiceProvider.cs
- ClientOptions.cs
- NameValueCollection.cs
- RenamedEventArgs.cs
- WindowsFormsHostPropertyMap.cs
- _StreamFramer.cs
- PropertyValueEditor.cs
- InvokeWebService.cs
- CompilationSection.cs
- Helpers.cs
- DeploymentExceptionMapper.cs
- SrgsRuleRef.cs
- CanonicalXml.cs
- PerformanceCounterPermissionAttribute.cs
- ContentHostHelper.cs
- ProvideValueServiceProvider.cs
- CanExecuteRoutedEventArgs.cs
- cookieexception.cs
- DetailsViewInsertEventArgs.cs
- DataListItem.cs
- ConfigurationStrings.cs
- TextCompositionEventArgs.cs
- DataServiceHostFactory.cs
- TextHidden.cs
- WeakRefEnumerator.cs
- BitmapMetadataEnumerator.cs
- TailCallAnalyzer.cs
- MessageQueueKey.cs
- CellTreeNodeVisitors.cs
- securitymgrsite.cs
- EventProvider.cs