Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / ExpressionBindings.cs / 1 / ExpressionBindings.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Describes a binding for an expression. Conceptually similar to a foreach loop /// in C#. The DbExpression property defines the collection being iterated over, /// while the Var property provides a means to reference the current element /// of the collection during the iteration. DbExpressionBinding is used to describe the set arguments /// to relational expressions such as ///, /// and . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; internal DbExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable name is non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if(!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_CollectionRequired, "input"); } Debug.Assert(elementType.IsReadOnly, "DbExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; } /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } internal static void Check(string strName, DbExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } ///that references the element variable. /// /// Defines the binding for the input set to a [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; private string _groupVarName; internal DbGroupExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName, string groupVarName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); EntityUtil.CheckArgumentNull(groupVarName, "groupVarName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable and Group names are both non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } if (string.IsNullOrEmpty(groupVarName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_GroupVariableNameNotValid, "groupVarName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if (!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_CollectionRequired, "input"); } Debug.Assert((elementType.IsReadOnly), "DbGroupExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; _groupVarName = groupVarName; } ///. /// In addition to the properties of , DbGroupExpressionBinding /// also provides access to the group element via the variable reference. /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } ////// Gets the name assigned to the group element variable. /// public string GroupVariableName { get { return _groupVarName; } } ////// Gets the type metadata of the group element variable. /// public TypeUsage GroupVariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the group element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression GroupVariable { get { return this.Expression.CommandTree.CreateVariableReferenceExpression(_groupVarName, _varType); } } internal static void Check(string strName, DbGroupExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Describes a binding for an expression. Conceptually similar to a foreach loop /// in C#. The DbExpression property defines the collection being iterated over, /// while the Var property provides a means to reference the current element /// of the collection during the iteration. DbExpressionBinding is used to describe the set arguments /// to relational expressions such as ///, /// and . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; internal DbExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable name is non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if(!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_CollectionRequired, "input"); } Debug.Assert(elementType.IsReadOnly, "DbExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; } /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } internal static void Check(string strName, DbExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } ///that references the element variable. /// /// Defines the binding for the input set to a [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; private string _groupVarName; internal DbGroupExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName, string groupVarName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); EntityUtil.CheckArgumentNull(groupVarName, "groupVarName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable and Group names are both non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } if (string.IsNullOrEmpty(groupVarName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_GroupVariableNameNotValid, "groupVarName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if (!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_CollectionRequired, "input"); } Debug.Assert((elementType.IsReadOnly), "DbGroupExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; _groupVarName = groupVarName; } ///. /// In addition to the properties of , DbGroupExpressionBinding /// also provides access to the group element via the variable reference. /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } ////// Gets the name assigned to the group element variable. /// public string GroupVariableName { get { return _groupVarName; } } ////// Gets the type metadata of the group element variable. /// public TypeUsage GroupVariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the group element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression GroupVariable { get { return this.Expression.CommandTree.CreateVariableReferenceExpression(_groupVarName, _varType); } } internal static void Check(string strName, DbGroupExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
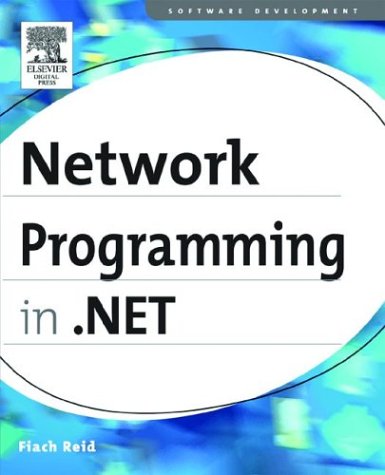
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextSpanModifier.cs
- MarkupCompiler.cs
- ConfigurationElementCollection.cs
- AutomationElementIdentifiers.cs
- TextSpanModifier.cs
- MarkupCompilePass1.cs
- ArcSegment.cs
- IgnoreSection.cs
- SpecularMaterial.cs
- TransactedBatchingBehavior.cs
- XmlSerializationReader.cs
- webclient.cs
- Style.cs
- precedingsibling.cs
- DataGridViewSortCompareEventArgs.cs
- Win32SafeHandles.cs
- StylusPointPropertyInfo.cs
- StrongNamePublicKeyBlob.cs
- ServicePointManager.cs
- CodeLabeledStatement.cs
- BitmapEffectGeneralTransform.cs
- OracleInfoMessageEventArgs.cs
- CommonDialog.cs
- WebEventTraceProvider.cs
- OracleColumn.cs
- FtpCachePolicyElement.cs
- FieldToken.cs
- RegistryHandle.cs
- DataGridViewColumnCollectionEditor.cs
- TextLineBreak.cs
- ColorConverter.cs
- Util.cs
- BamlMapTable.cs
- Stopwatch.cs
- MediaPlayerState.cs
- ListBox.cs
- GuidTagList.cs
- PropertyMetadata.cs
- Rules.cs
- Atom10FormatterFactory.cs
- GradientStop.cs
- ImportCatalogPart.cs
- FixedSOMPage.cs
- PrtCap_Base.cs
- ScrollBar.cs
- ExceptionUtil.cs
- SoapServerProtocol.cs
- HtmlInputReset.cs
- __Filters.cs
- PictureBox.cs
- Single.cs
- XmlObjectSerializerReadContext.cs
- HashJoinQueryOperatorEnumerator.cs
- PropertyRef.cs
- ToolStripPanelCell.cs
- CanExecuteRoutedEventArgs.cs
- Root.cs
- MultiAsyncResult.cs
- HtmlWindowCollection.cs
- SQLInt64.cs
- TargetConverter.cs
- UpdateProgress.cs
- WebPageTraceListener.cs
- XpsS0ValidatingLoader.cs
- InternalConfigConfigurationFactory.cs
- EdmProviderManifest.cs
- SortAction.cs
- XmlQueryType.cs
- SvcMapFileLoader.cs
- IntPtr.cs
- DrawingAttributes.cs
- PropertyTabAttribute.cs
- SyndicationElementExtension.cs
- HttpHandlerAction.cs
- FrameworkElement.cs
- UnaryOperationBinder.cs
- ModuleElement.cs
- DeadCharTextComposition.cs
- FixedMaxHeap.cs
- WebServiceHandler.cs
- XhtmlStyleClass.cs
- LinkArea.cs
- FontStretches.cs
- AlternateViewCollection.cs
- RegexBoyerMoore.cs
- StylusButtonEventArgs.cs
- VerticalAlignConverter.cs
- NodeCounter.cs
- SqlBulkCopy.cs
- NestPullup.cs
- PrimaryKeyTypeConverter.cs
- NotFiniteNumberException.cs
- CompilerHelpers.cs
- ImageListImageEditor.cs
- SHA512CryptoServiceProvider.cs
- Page.cs
- _OSSOCK.cs
- PopupControlService.cs
- SRDisplayNameAttribute.cs
- ChangeNode.cs