Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / PointAnimation.cs / 1305600 / PointAnimation.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using MS.Internal.PresentationCore; namespace System.Windows.Media.Animation { ////// Animates the value of a Point property using linear interpolation /// between two values. The values are determined by the combination of /// From, To, or By values that are set on the animation. /// public partial class PointAnimation : PointAnimationBase { #region Data ////// This is used if the user has specified From, To, and/or By values. /// private Point[] _keyValues; private AnimationType _animationType; private bool _isAnimationFunctionValid; #endregion #region Constructors ////// Static ctor for PointAnimation establishes /// dependency properties, using as much shared data as possible. /// static PointAnimation() { Type typeofProp = typeof(Point?); Type typeofThis = typeof(PointAnimation); PropertyChangedCallback propCallback = new PropertyChangedCallback(AnimationFunction_Changed); ValidateValueCallback validateCallback = new ValidateValueCallback(ValidateFromToOrByValue); FromProperty = DependencyProperty.Register( "From", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); ToProperty = DependencyProperty.Register( "To", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); ByProperty = DependencyProperty.Register( "By", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); EasingFunctionProperty = DependencyProperty.Register( "EasingFunction", typeof(IEasingFunction), typeofThis); } ////// Creates a new PointAnimation with all properties set to /// their default values. /// public PointAnimation() : base() { } ////// Creates a new PointAnimation that will animate a /// Point property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public PointAnimation(Point toValue, Duration duration) : this() { To = toValue; Duration = duration; } ////// Creates a new PointAnimation that will animate a /// Point property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public PointAnimation(Point toValue, Duration duration, FillBehavior fillBehavior) : this() { To = toValue; Duration = duration; FillBehavior = fillBehavior; } ////// Creates a new PointAnimation that will animate a /// Point property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public PointAnimation(Point fromValue, Point toValue, Duration duration) : this() { From = fromValue; To = toValue; Duration = duration; } ////// Creates a new PointAnimation that will animate a /// Point property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public PointAnimation(Point fromValue, Point toValue, Duration duration, FillBehavior fillBehavior) : this() { From = fromValue; To = toValue; Duration = duration; FillBehavior = fillBehavior; } #endregion #region Freezable ////// Creates a copy of this PointAnimation /// ///The copy public new PointAnimation Clone() { return (PointAnimation)base.Clone(); } // // Note that we don't override the Clone virtuals (CloneCore, CloneCurrentValueCore, // GetAsFrozenCore, and GetCurrentValueAsFrozenCore) even though this class has state // not stored in a DP. // // We don't need to clone _animationType and _keyValues because they are the the cached // results of animation function validation, which can be recomputed. The other remaining // field, isAnimationFunctionValid, defaults to false, which causes this recomputation to happen. // ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PointAnimation(); } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Point GetCurrentValueCore(Point defaultOriginValue, Point defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); if (!_isAnimationFunctionValid) { ValidateAnimationFunction(); } double progress = animationClock.CurrentProgress.Value; IEasingFunction easingFunction = EasingFunction; if (easingFunction != null) { progress = easingFunction.Ease(progress); } Point from = new Point(); Point to = new Point(); Point accumulated = new Point(); Point foundation = new Point(); // need to validate the default origin and destination values if // the animation uses them as the from, to, or foundation values bool validateOrigin = false; bool validateDestination = false; switch(_animationType) { case AnimationType.Automatic: from = defaultOriginValue; to = defaultDestinationValue; validateOrigin = true; validateDestination = true; break; case AnimationType.From: from = _keyValues[0]; to = defaultDestinationValue; validateDestination = true; break; case AnimationType.To: from = defaultOriginValue; to = _keyValues[0]; validateOrigin = true; break; case AnimationType.By: // According to the SMIL specification, a By animation is // always additive. But we don't force this so that a // user can re-use a By animation and have it replace the // animations that precede it in the list without having // to manually set the From value to the base value. to = _keyValues[0]; foundation = defaultOriginValue; validateOrigin = true; break; case AnimationType.FromTo: from = _keyValues[0]; to = _keyValues[1]; if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; case AnimationType.FromBy: from = _keyValues[0]; to = AnimatedTypeHelpers.AddPoint(_keyValues[0], _keyValues[1]); if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; default: Debug.Fail("Unknown animation type."); break; } if (validateOrigin && !AnimatedTypeHelpers.IsValidAnimationValuePoint(defaultOriginValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "origin", defaultOriginValue.ToString(CultureInfo.InvariantCulture))); } if (validateDestination && !AnimatedTypeHelpers.IsValidAnimationValuePoint(defaultDestinationValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "destination", defaultDestinationValue.ToString(CultureInfo.InvariantCulture))); } if (IsCumulative) { double currentRepeat = (double)(animationClock.CurrentIteration - 1); if (currentRepeat > 0.0) { Point accumulator = AnimatedTypeHelpers.SubtractPoint(to, from); accumulated = AnimatedTypeHelpers.ScalePoint(accumulator, currentRepeat); } } // return foundation + accumulated + from + ((to - from) * progress) return AnimatedTypeHelpers.AddPoint( foundation, AnimatedTypeHelpers.AddPoint( accumulated, AnimatedTypeHelpers.InterpolatePoint(from, to, progress))); } private void ValidateAnimationFunction() { _animationType = AnimationType.Automatic; _keyValues = null; if (From.HasValue) { if (To.HasValue) { _animationType = AnimationType.FromTo; _keyValues = new Point[2]; _keyValues[0] = From.Value; _keyValues[1] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.FromBy; _keyValues = new Point[2]; _keyValues[0] = From.Value; _keyValues[1] = By.Value; } else { _animationType = AnimationType.From; _keyValues = new Point[1]; _keyValues[0] = From.Value; } } else if (To.HasValue) { _animationType = AnimationType.To; _keyValues = new Point[1]; _keyValues[0] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.By; _keyValues = new Point[1]; _keyValues[0] = By.Value; } _isAnimationFunctionValid = true; } #endregion #region Properties private static void AnimationFunction_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointAnimation a = (PointAnimation)d; a._isAnimationFunctionValid = false; a.PropertyChanged(e.Property); } private static bool ValidateFromToOrByValue(object value) { Point? typedValue = (Point?)value; if (typedValue.HasValue) { return AnimatedTypeHelpers.IsValidAnimationValuePoint(typedValue.Value); } else { return true; } } ////// FromProperty /// public static readonly DependencyProperty FromProperty; ////// From /// public Point? From { get { return (Point?)GetValue(FromProperty); } set { SetValueInternal(FromProperty, value); } } ////// ToProperty /// public static readonly DependencyProperty ToProperty; ////// To /// public Point? To { get { return (Point?)GetValue(ToProperty); } set { SetValueInternal(ToProperty, value); } } ////// ByProperty /// public static readonly DependencyProperty ByProperty; ////// By /// public Point? By { get { return (Point?)GetValue(ByProperty); } set { SetValueInternal(ByProperty, value); } } ////// EasingFunctionProperty /// public static readonly DependencyProperty EasingFunctionProperty; ////// EasingFunction /// public IEasingFunction EasingFunction { get { return (IEasingFunction)GetValue(EasingFunctionProperty); } set { SetValueInternal(EasingFunctionProperty, value); } } ////// If this property is set to true the animation will add its value to /// the base value instead of replacing it entirely. /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValueInternal(IsAdditiveProperty, BooleanBoxes.Box(value)); } } ////// It this property is set to true, the animation will accumulate its /// value over repeats. For instance if you have a From value of 0.0 and /// a To value of 1.0, the animation return values from 1.0 to 2.0 over /// the second reteat cycle, and 2.0 to 3.0 over the third, etc. /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValueInternal(IsCumulativeProperty, BooleanBoxes.Box(value)); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using MS.Internal.PresentationCore; namespace System.Windows.Media.Animation { ////// Animates the value of a Point property using linear interpolation /// between two values. The values are determined by the combination of /// From, To, or By values that are set on the animation. /// public partial class PointAnimation : PointAnimationBase { #region Data ////// This is used if the user has specified From, To, and/or By values. /// private Point[] _keyValues; private AnimationType _animationType; private bool _isAnimationFunctionValid; #endregion #region Constructors ////// Static ctor for PointAnimation establishes /// dependency properties, using as much shared data as possible. /// static PointAnimation() { Type typeofProp = typeof(Point?); Type typeofThis = typeof(PointAnimation); PropertyChangedCallback propCallback = new PropertyChangedCallback(AnimationFunction_Changed); ValidateValueCallback validateCallback = new ValidateValueCallback(ValidateFromToOrByValue); FromProperty = DependencyProperty.Register( "From", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); ToProperty = DependencyProperty.Register( "To", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); ByProperty = DependencyProperty.Register( "By", typeofProp, typeofThis, new PropertyMetadata((Point?)null, propCallback), validateCallback); EasingFunctionProperty = DependencyProperty.Register( "EasingFunction", typeof(IEasingFunction), typeofThis); } ////// Creates a new PointAnimation with all properties set to /// their default values. /// public PointAnimation() : base() { } ////// Creates a new PointAnimation that will animate a /// Point property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public PointAnimation(Point toValue, Duration duration) : this() { To = toValue; Duration = duration; } ////// Creates a new PointAnimation that will animate a /// Point property from its base value to the value specified /// by the "toValue" parameter of this constructor. /// public PointAnimation(Point toValue, Duration duration, FillBehavior fillBehavior) : this() { To = toValue; Duration = duration; FillBehavior = fillBehavior; } ////// Creates a new PointAnimation that will animate a /// Point property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public PointAnimation(Point fromValue, Point toValue, Duration duration) : this() { From = fromValue; To = toValue; Duration = duration; } ////// Creates a new PointAnimation that will animate a /// Point property from the "fromValue" parameter of this constructor /// to the "toValue" parameter. /// public PointAnimation(Point fromValue, Point toValue, Duration duration, FillBehavior fillBehavior) : this() { From = fromValue; To = toValue; Duration = duration; FillBehavior = fillBehavior; } #endregion #region Freezable ////// Creates a copy of this PointAnimation /// ///The copy public new PointAnimation Clone() { return (PointAnimation)base.Clone(); } // // Note that we don't override the Clone virtuals (CloneCore, CloneCurrentValueCore, // GetAsFrozenCore, and GetCurrentValueAsFrozenCore) even though this class has state // not stored in a DP. // // We don't need to clone _animationType and _keyValues because they are the the cached // results of animation function validation, which can be recomputed. The other remaining // field, isAnimationFunctionValid, defaults to false, which causes this recomputation to happen. // ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PointAnimation(); } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Point GetCurrentValueCore(Point defaultOriginValue, Point defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); if (!_isAnimationFunctionValid) { ValidateAnimationFunction(); } double progress = animationClock.CurrentProgress.Value; IEasingFunction easingFunction = EasingFunction; if (easingFunction != null) { progress = easingFunction.Ease(progress); } Point from = new Point(); Point to = new Point(); Point accumulated = new Point(); Point foundation = new Point(); // need to validate the default origin and destination values if // the animation uses them as the from, to, or foundation values bool validateOrigin = false; bool validateDestination = false; switch(_animationType) { case AnimationType.Automatic: from = defaultOriginValue; to = defaultDestinationValue; validateOrigin = true; validateDestination = true; break; case AnimationType.From: from = _keyValues[0]; to = defaultDestinationValue; validateDestination = true; break; case AnimationType.To: from = defaultOriginValue; to = _keyValues[0]; validateOrigin = true; break; case AnimationType.By: // According to the SMIL specification, a By animation is // always additive. But we don't force this so that a // user can re-use a By animation and have it replace the // animations that precede it in the list without having // to manually set the From value to the base value. to = _keyValues[0]; foundation = defaultOriginValue; validateOrigin = true; break; case AnimationType.FromTo: from = _keyValues[0]; to = _keyValues[1]; if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; case AnimationType.FromBy: from = _keyValues[0]; to = AnimatedTypeHelpers.AddPoint(_keyValues[0], _keyValues[1]); if (IsAdditive) { foundation = defaultOriginValue; validateOrigin = true; } break; default: Debug.Fail("Unknown animation type."); break; } if (validateOrigin && !AnimatedTypeHelpers.IsValidAnimationValuePoint(defaultOriginValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "origin", defaultOriginValue.ToString(CultureInfo.InvariantCulture))); } if (validateDestination && !AnimatedTypeHelpers.IsValidAnimationValuePoint(defaultDestinationValue)) { throw new InvalidOperationException( SR.Get( SRID.Animation_Invalid_DefaultValue, this.GetType(), "destination", defaultDestinationValue.ToString(CultureInfo.InvariantCulture))); } if (IsCumulative) { double currentRepeat = (double)(animationClock.CurrentIteration - 1); if (currentRepeat > 0.0) { Point accumulator = AnimatedTypeHelpers.SubtractPoint(to, from); accumulated = AnimatedTypeHelpers.ScalePoint(accumulator, currentRepeat); } } // return foundation + accumulated + from + ((to - from) * progress) return AnimatedTypeHelpers.AddPoint( foundation, AnimatedTypeHelpers.AddPoint( accumulated, AnimatedTypeHelpers.InterpolatePoint(from, to, progress))); } private void ValidateAnimationFunction() { _animationType = AnimationType.Automatic; _keyValues = null; if (From.HasValue) { if (To.HasValue) { _animationType = AnimationType.FromTo; _keyValues = new Point[2]; _keyValues[0] = From.Value; _keyValues[1] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.FromBy; _keyValues = new Point[2]; _keyValues[0] = From.Value; _keyValues[1] = By.Value; } else { _animationType = AnimationType.From; _keyValues = new Point[1]; _keyValues[0] = From.Value; } } else if (To.HasValue) { _animationType = AnimationType.To; _keyValues = new Point[1]; _keyValues[0] = To.Value; } else if (By.HasValue) { _animationType = AnimationType.By; _keyValues = new Point[1]; _keyValues[0] = By.Value; } _isAnimationFunctionValid = true; } #endregion #region Properties private static void AnimationFunction_Changed(DependencyObject d, DependencyPropertyChangedEventArgs e) { PointAnimation a = (PointAnimation)d; a._isAnimationFunctionValid = false; a.PropertyChanged(e.Property); } private static bool ValidateFromToOrByValue(object value) { Point? typedValue = (Point?)value; if (typedValue.HasValue) { return AnimatedTypeHelpers.IsValidAnimationValuePoint(typedValue.Value); } else { return true; } } ////// FromProperty /// public static readonly DependencyProperty FromProperty; ////// From /// public Point? From { get { return (Point?)GetValue(FromProperty); } set { SetValueInternal(FromProperty, value); } } ////// ToProperty /// public static readonly DependencyProperty ToProperty; ////// To /// public Point? To { get { return (Point?)GetValue(ToProperty); } set { SetValueInternal(ToProperty, value); } } ////// ByProperty /// public static readonly DependencyProperty ByProperty; ////// By /// public Point? By { get { return (Point?)GetValue(ByProperty); } set { SetValueInternal(ByProperty, value); } } ////// EasingFunctionProperty /// public static readonly DependencyProperty EasingFunctionProperty; ////// EasingFunction /// public IEasingFunction EasingFunction { get { return (IEasingFunction)GetValue(EasingFunctionProperty); } set { SetValueInternal(EasingFunctionProperty, value); } } ////// If this property is set to true the animation will add its value to /// the base value instead of replacing it entirely. /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValueInternal(IsAdditiveProperty, BooleanBoxes.Box(value)); } } ////// It this property is set to true, the animation will accumulate its /// value over repeats. For instance if you have a From value of 0.0 and /// a To value of 1.0, the animation return values from 1.0 to 2.0 over /// the second reteat cycle, and 2.0 to 3.0 over the third, etc. /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValueInternal(IsCumulativeProperty, BooleanBoxes.Box(value)); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
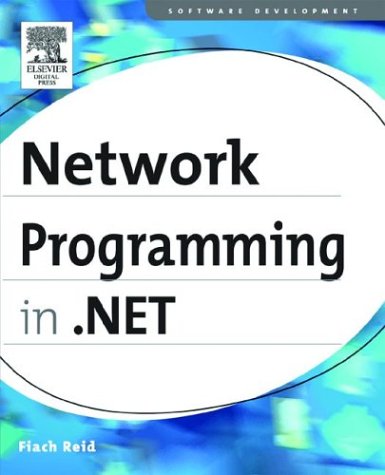
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TokenCreationException.cs
- Logging.cs
- EventDescriptor.cs
- DigitalSignatureProvider.cs
- XamlParser.cs
- CreateUserWizard.cs
- UdpChannelListener.cs
- FormViewCommandEventArgs.cs
- QilLiteral.cs
- AttributeUsageAttribute.cs
- EntitySqlQueryState.cs
- TypeFieldSchema.cs
- DataKeyArray.cs
- RegexInterpreter.cs
- TraceXPathNavigator.cs
- BackStopAuthenticationModule.cs
- Int32.cs
- HttpRequestTraceRecord.cs
- BufferedReadStream.cs
- InfocardInteractiveChannelInitializer.cs
- MenuAutomationPeer.cs
- ConstNode.cs
- ApplicationSecurityInfo.cs
- DependencyObjectProvider.cs
- PointCollection.cs
- EventLogPermissionEntryCollection.cs
- ImmutableCommunicationTimeouts.cs
- SecurityManager.cs
- VectorAnimation.cs
- InvalidateEvent.cs
- PolicyLevel.cs
- SmtpCommands.cs
- OwnerDrawPropertyBag.cs
- NativeActivityTransactionContext.cs
- RegexInterpreter.cs
- RubberbandSelector.cs
- TemplateBuilder.cs
- ListChangedEventArgs.cs
- GridSplitter.cs
- ToolboxBitmapAttribute.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- InkCanvas.cs
- Trace.cs
- FastEncoderWindow.cs
- TypeLibConverter.cs
- DataGridViewRowsRemovedEventArgs.cs
- TextParaLineResult.cs
- CompilerErrorCollection.cs
- DBSqlParserColumnCollection.cs
- ValueProviderWrapper.cs
- Visual3D.cs
- CompilerResults.cs
- Msmq.cs
- CompiledQuery.cs
- DataGridToolTip.cs
- Terminate.cs
- GridViewRowPresenterBase.cs
- BridgeDataRecord.cs
- MessageSecurityVersion.cs
- OracleException.cs
- DataGridViewRowsRemovedEventArgs.cs
- HttpClientCertificate.cs
- DeferredReference.cs
- SettingsAttributeDictionary.cs
- Html32TextWriter.cs
- ExpressionEditorAttribute.cs
- RequestQueue.cs
- Vector3DCollection.cs
- securestring.cs
- Wizard.cs
- ResizeGrip.cs
- LabelEditEvent.cs
- ValueSerializer.cs
- _ChunkParse.cs
- DrawingBrush.cs
- AmbientValueAttribute.cs
- SynchronizationLockException.cs
- TraceUtility.cs
- StoreItemCollection.cs
- TransactionContextValidator.cs
- WindowsTokenRoleProvider.cs
- MediaSystem.cs
- TokenFactoryFactory.cs
- WebPartZoneAutoFormat.cs
- DetailsViewPageEventArgs.cs
- TopClause.cs
- CqlQuery.cs
- ContentTextAutomationPeer.cs
- PEFileEvidenceFactory.cs
- NativeCompoundFileAPIs.cs
- SByte.cs
- Configuration.cs
- TdsParserSessionPool.cs
- KeyEventArgs.cs
- DataRelationPropertyDescriptor.cs
- DataGridViewCellStyleConverter.cs
- ImageMetadata.cs
- ToolStripDropDownClosedEventArgs.cs
- IIS7UserPrincipal.cs