Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / DataObjectSettingDataEventArgs.cs / 1 / DataObjectSettingDataEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataObjectSettingData event arguments // //--------------------------------------------------------------------------- using System; namespace System.Windows { ////// Arguments for the DataObject.SettingData event. /// The DataObject.SettingData event is raised during /// Copy (or Drag start) command when an editor /// is going to start data conversion for some /// of data formats. By handling this event an application /// can prevent from editon doing that thus making /// Copy performance better. /// public sealed class DataObjectSettingDataEventArgs : DataObjectEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a DataObjectSettingDataEventArgs. /// /// /// DataObject to which a new data format is going to be added. /// /// /// Format which is going to be added to the DataObject. /// public DataObjectSettingDataEventArgs(IDataObject dataObject, string format) // : base(System.Windows.DataObject.SettingDataEvent, /*isDragDrop:*/false) { if (dataObject == null) { throw new ArgumentNullException("dataObject"); } if (format == null) { throw new ArgumentNullException("format"); } _dataObject = dataObject; _format = format; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// DataObject to which a new data format is going to be added. /// public IDataObject DataObject { get { return _dataObject; } } ////// Format which is going to be added to the DataObject. /// public string Format { get { return _format; } } #endregion Public Properties #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DataObjectSettingDataEventHandler handler = (DataObjectSettingDataEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private IDataObject _dataObject; private string _format; #endregion Private Fields } ////// The delegate to use for handlers that receive the DataObject.QueryingCopy/QueryingPaste events. /// ////// A handler for a DataObject.SettingData event. /// Te event is fired as part of Copy (or Drag) command /// once for each of data formats added to a DataObject. /// The purpose of this handler is mostly copy command /// optimization. With the help of it application /// can filter some formats from being added to DataObject. /// The other opportunity of doing that exists in /// DataObject.Copying event, which could set all undesirable /// formats to null, but in this case the work for data /// conversion is already done, which may be too expensive. /// By handling DataObject.SettingData event an application /// can prevent from each particular data format conversion. /// By calling DataObjectSettingDataEventArgs.CancelCommand /// method the handler tells an editor to skip one particular /// data format (identified by DataObjectSettingDataEventArgs.Format /// property). Note that calling CancelCommand method /// for this event does not cancel the whole Copy or Drag /// command. /// public delegate void DataObjectSettingDataEventHandler(object sender, DataObjectSettingDataEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
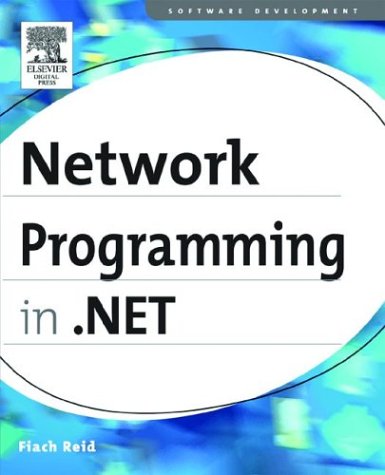
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeneralTransform3DCollection.cs
- DBDataPermission.cs
- SqlGenericUtil.cs
- AccessDataSourceView.cs
- AssociationEndMember.cs
- Tokenizer.cs
- SizeAnimation.cs
- MSAAWinEventWrap.cs
- ConfigurationManagerHelper.cs
- MenuScrollingVisibilityConverter.cs
- CheckPair.cs
- ScrollBar.cs
- OpenFileDialog.cs
- Properties.cs
- ClientSettingsStore.cs
- _AutoWebProxyScriptEngine.cs
- PeerHelpers.cs
- DataGridViewCellConverter.cs
- SafeMemoryMappedFileHandle.cs
- Assembly.cs
- SoapEnvelopeProcessingElement.cs
- WebBrowserHelper.cs
- SchemaImporterExtensionsSection.cs
- TextSearch.cs
- StringCollection.cs
- HistoryEventArgs.cs
- DataGridViewComboBoxCell.cs
- RegexWriter.cs
- SqlParameterCollection.cs
- SoapCodeExporter.cs
- IdentityHolder.cs
- SystemWebExtensionsSectionGroup.cs
- CompositeTypefaceMetrics.cs
- HttpListenerResponse.cs
- ConnectionPointCookie.cs
- ValidationErrorCollection.cs
- FormatException.cs
- SessionPageStatePersister.cs
- DivideByZeroException.cs
- LocatorPartList.cs
- ErrorStyle.cs
- HMACSHA256.cs
- Empty.cs
- PeerServiceMessageContracts.cs
- RpcResponse.cs
- ToolStripDropDownClosingEventArgs.cs
- GridViewRowCollection.cs
- WebPartConnectionsCancelEventArgs.cs
- DispatchProxy.cs
- ThreadAbortException.cs
- ApplicationSecurityInfo.cs
- DbModificationCommandTree.cs
- CriticalFinalizerObject.cs
- BatchWriter.cs
- SizeAnimationUsingKeyFrames.cs
- DrawingAttributes.cs
- EventData.cs
- InvokeGenerator.cs
- ScanQueryOperator.cs
- PipelineModuleStepContainer.cs
- ParameterBuilder.cs
- PagedControl.cs
- AttachmentCollection.cs
- SurrogateEncoder.cs
- DefaultPrintController.cs
- SoapInteropTypes.cs
- Wizard.cs
- SignatureHelper.cs
- LineBreakRecord.cs
- XmlSchemaAttributeGroupRef.cs
- AuthenticationConfig.cs
- ProfileGroupSettingsCollection.cs
- SponsorHelper.cs
- DbMetaDataFactory.cs
- PasswordBox.cs
- DataGridViewControlCollection.cs
- TypeDescriptionProviderAttribute.cs
- _ListenerResponseStream.cs
- CustomCategoryAttribute.cs
- BridgeDataRecord.cs
- InteropAutomationProvider.cs
- UriScheme.cs
- SqlInfoMessageEvent.cs
- OptimizedTemplateContent.cs
- ButtonBase.cs
- ToolStripRenderEventArgs.cs
- PointHitTestResult.cs
- StringExpressionSet.cs
- Stylesheet.cs
- HashHelper.cs
- SqlBuilder.cs
- ConfigurationLockCollection.cs
- CodeCompiler.cs
- BufferedGraphicsContext.cs
- SpotLight.cs
- Utils.cs
- FlatButtonAppearance.cs
- BuilderPropertyEntry.cs
- Evidence.cs
- SBCSCodePageEncoding.cs