Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / XamlIntegration / FuncTypeConverter.cs / 1305376 / FuncTypeConverter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.XamlIntegration { using System; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Runtime; using System.Windows.Markup; using System.Xaml; public class FuncDeferringLoader : XamlDeferringLoader { public override object Load(XamlReader xamlReader, IServiceProvider context) { IXamlObjectWriterFactory objectWriterFactory = context.GetService(typeof(IXamlObjectWriterFactory)) as IXamlObjectWriterFactory; IProvideValueTarget provideValueService = context.GetService(typeof(IProvideValueTarget)) as IProvideValueTarget; Type propertyType = null; // // IProvideValueTarget.TargetProperty can return DP, Attached Property or MemberInfo for clr property // In this case it should always be a regular clr property here - we are always targeting Activity.Body. PropertyInfo propertyInfo = provideValueService.TargetProperty as PropertyInfo; if (propertyInfo != null) { propertyType = propertyInfo.PropertyType; } object instance = Activator.CreateInstance( typeof(FuncFactory<>).MakeGenericType(propertyType.GetGenericArguments()), objectWriterFactory, xamlReader); return Delegate.CreateDelegate(propertyType, instance, instance.GetType().GetMethod("Evaluate")); } public override XamlReader Save(object value, IServiceProvider serviceProvider) { throw FxTrace.Exception.AsError(new NotSupportedException(SR.SavingActivityToXamlNotSupported)); } abstract class FuncFactory { public XamlNodeList Nodes { get; set; } } class FuncFactory: FuncFactory { IXamlObjectWriterFactory objectWriterFactory; public FuncFactory(IXamlObjectWriterFactory objectWriterFactory, XamlReader reader) { this.objectWriterFactory = objectWriterFactory; this.Nodes = new XamlNodeList(reader.SchemaContext); XamlServices.Transform(reader, this.Nodes.Writer); } public T Evaluate() { XamlObjectWriter writer = this.objectWriterFactory.GetXamlObjectWriter(new XamlObjectWriterSettings()); XamlServices.Transform(this.Nodes.GetReader(), writer); return (T)writer.Result; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
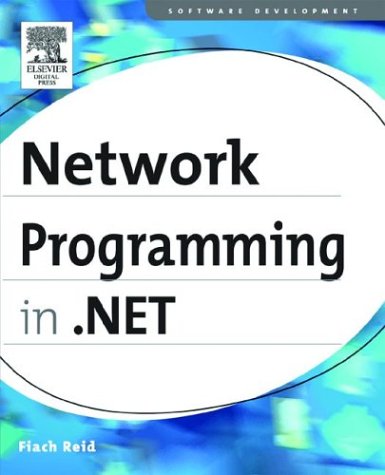
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentOrderComparer.cs
- WebProxyScriptElement.cs
- RealizationContext.cs
- DesignTimeParseData.cs
- AutoResetEvent.cs
- MdImport.cs
- ComponentResourceKey.cs
- WorkflowViewManager.cs
- ItemsControlAutomationPeer.cs
- SharedUtils.cs
- ResourceSet.cs
- SelectionRange.cs
- Size.cs
- DesignerDataStoredProcedure.cs
- DataGridViewCheckBoxCell.cs
- FieldBuilder.cs
- SafeRightsManagementHandle.cs
- GlobalAclOperationRequirement.cs
- PaperSource.cs
- Point3DConverter.cs
- BufferModeSettings.cs
- OutputCacheProfileCollection.cs
- PostBackOptions.cs
- MaterializeFromAtom.cs
- WindowsStatusBar.cs
- LocalClientSecuritySettings.cs
- StrokeFIndices.cs
- NamespaceDisplay.xaml.cs
- ExtentKey.cs
- SymbolType.cs
- StdValidatorsAndConverters.cs
- XmlSubtreeReader.cs
- RootBrowserWindow.cs
- ProgressBarRenderer.cs
- ReadContentAsBinaryHelper.cs
- UnsafeNativeMethods.cs
- ExceptionHandlers.cs
- messageonlyhwndwrapper.cs
- SHA256.cs
- TextEvent.cs
- AccessText.cs
- _HelperAsyncResults.cs
- SessionParameter.cs
- TextCompositionEventArgs.cs
- UnsignedPublishLicense.cs
- GrammarBuilder.cs
- MinimizableAttributeTypeConverter.cs
- HttpStreamFormatter.cs
- PointCollectionValueSerializer.cs
- ArgumentOutOfRangeException.cs
- XmlSchemaExternal.cs
- DataObjectMethodAttribute.cs
- SchemaMapping.cs
- TreeViewImageKeyConverter.cs
- Pair.cs
- CategoryValueConverter.cs
- DnsPermission.cs
- ExpandableObjectConverter.cs
- WebReferencesBuildProvider.cs
- WindowHideOrCloseTracker.cs
- _UriSyntax.cs
- EdmRelationshipRoleAttribute.cs
- SqlUdtInfo.cs
- Type.cs
- BindingSource.cs
- cookieexception.cs
- OracleCommandBuilder.cs
- ManifestResourceInfo.cs
- ParameterBinding.cs
- TemplateComponentConnector.cs
- PassportAuthenticationEventArgs.cs
- BitmapVisualManager.cs
- ClientApiGenerator.cs
- ClosableStream.cs
- FacetDescription.cs
- wgx_exports.cs
- BindableTemplateBuilder.cs
- OptimalTextSource.cs
- Dictionary.cs
- DataGridViewRowConverter.cs
- ThicknessKeyFrameCollection.cs
- TextRangeProviderWrapper.cs
- XmlImplementation.cs
- MLangCodePageEncoding.cs
- safelink.cs
- IOException.cs
- CustomBindingCollectionElement.cs
- NamespaceCollection.cs
- Operator.cs
- Stack.cs
- MobileControlDesigner.cs
- ToolStripSettings.cs
- RepeaterItem.cs
- PagerSettings.cs
- PageThemeBuildProvider.cs
- QuadTree.cs
- SymmetricCryptoHandle.cs
- Wizard.cs
- GridErrorDlg.cs
- AppDomainAttributes.cs