Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / _OSSOCK.cs / 1 / _OSSOCK.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Net.Sockets; using System.Runtime.InteropServices; // // Argument structure for IP_ADD_MEMBERSHIP and IP_DROP_MEMBERSHIP. // [StructLayout(LayoutKind.Sequential)] internal struct IPMulticastRequest { internal int MulticastAddress; // IP multicast address of group internal int InterfaceAddress; // local IP address of interface internal static readonly int Size = Marshal.SizeOf(typeof(IPMulticastRequest)); } [StructLayout(LayoutKind.Sequential)] internal struct Linger { internal short OnOff; // option on/off internal short Time; // linger time } [StructLayout(LayoutKind.Sequential)] internal struct WSABuffer { internal int Length; // Length of Buffer internal IntPtr Pointer;// Pointer to Buffer } [StructLayout(LayoutKind.Sequential)] internal class TransmitFileBuffers { internal IntPtr preBuffer;// Pointer to Buffer internal int preBufferLength; // Length of Buffer internal IntPtr postBuffer;// Pointer to Buffer internal int postBufferLength; // Length of Buffer } [StructLayout(LayoutKind.Sequential)] internal struct WSAData { internal short wVersion; internal short wHighVersion; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=257)] internal string szDescription; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=129)] internal string szSystemStatus; internal short iMaxSockets; internal short iMaxUdpDg; internal IntPtr lpVendorInfo; } // data structures and types needed for getaddrinfo calls. [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Ansi)] internal unsafe struct AddressInfo { internal AddressInfoHints ai_flags; internal AddressFamily ai_family; internal SocketType ai_socktype; internal ProtocolFamily ai_protocol; internal int ai_addrlen; internal sbyte* ai_canonname; // Ptr to the cannonical name - check for NULL internal byte* ai_addr; // Ptr to the sockaddr structure internal AddressInfo* ai_next; // Ptr to the next AddressInfo structure } [Flags] internal enum AddressInfoHints { AI_PASSIVE = 0x01, /* Socket address will be used in bind() call */ AI_CANONNAME = 0x02, /* Return canonical name in first ai_canonname */ AI_NUMERICHOST = 0x04, /* Nodename must be a numeric address string */ } [Flags] internal enum NameInfoFlags { NI_NOFQDN = 0x01, /* Only return nodename portion for local hosts */ NI_NUMERICHOST = 0x02, /* Return numeric form of the host's address */ NI_NAMEREQD = 0x04, /* Error if the host's name not in DNS */ NI_NUMERICSERV = 0x08, /* Return numeric form of the service (port #) */ NI_DGRAM = 0x10, /* Service is a datagram service */ } // Argument structure for IPV6_ADD_MEMBERSHIP and IPV6_DROP_MEMBERSHIP. [StructLayout(LayoutKind.Sequential)] internal struct IPv6MulticastRequest { [MarshalAs(UnmanagedType.ByValArray,SizeConst=16)] internal byte[] MulticastAddress; // IP address of group internal int InterfaceIndex; // local interface index internal static readonly int Size = Marshal.SizeOf(typeof(IPv6MulticastRequest)); } // // used as last parameter to WSASocket call // [Flags] internal enum SocketConstructorFlags { WSA_FLAG_OVERLAPPED = 0x01, WSA_FLAG_MULTIPOINT_C_ROOT = 0x02, WSA_FLAG_MULTIPOINT_C_LEAF = 0x04, WSA_FLAG_MULTIPOINT_D_ROOT = 0x08, WSA_FLAG_MULTIPOINT_D_LEAF = 0x10, } } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Net.Sockets; using System.Runtime.InteropServices; // // Argument structure for IP_ADD_MEMBERSHIP and IP_DROP_MEMBERSHIP. // [StructLayout(LayoutKind.Sequential)] internal struct IPMulticastRequest { internal int MulticastAddress; // IP multicast address of group internal int InterfaceAddress; // local IP address of interface internal static readonly int Size = Marshal.SizeOf(typeof(IPMulticastRequest)); } [StructLayout(LayoutKind.Sequential)] internal struct Linger { internal short OnOff; // option on/off internal short Time; // linger time } [StructLayout(LayoutKind.Sequential)] internal struct WSABuffer { internal int Length; // Length of Buffer internal IntPtr Pointer;// Pointer to Buffer } [StructLayout(LayoutKind.Sequential)] internal class TransmitFileBuffers { internal IntPtr preBuffer;// Pointer to Buffer internal int preBufferLength; // Length of Buffer internal IntPtr postBuffer;// Pointer to Buffer internal int postBufferLength; // Length of Buffer } [StructLayout(LayoutKind.Sequential)] internal struct WSAData { internal short wVersion; internal short wHighVersion; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=257)] internal string szDescription; [MarshalAs(UnmanagedType.ByValTStr, SizeConst=129)] internal string szSystemStatus; internal short iMaxSockets; internal short iMaxUdpDg; internal IntPtr lpVendorInfo; } // data structures and types needed for getaddrinfo calls. [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Ansi)] internal unsafe struct AddressInfo { internal AddressInfoHints ai_flags; internal AddressFamily ai_family; internal SocketType ai_socktype; internal ProtocolFamily ai_protocol; internal int ai_addrlen; internal sbyte* ai_canonname; // Ptr to the cannonical name - check for NULL internal byte* ai_addr; // Ptr to the sockaddr structure internal AddressInfo* ai_next; // Ptr to the next AddressInfo structure } [Flags] internal enum AddressInfoHints { AI_PASSIVE = 0x01, /* Socket address will be used in bind() call */ AI_CANONNAME = 0x02, /* Return canonical name in first ai_canonname */ AI_NUMERICHOST = 0x04, /* Nodename must be a numeric address string */ } [Flags] internal enum NameInfoFlags { NI_NOFQDN = 0x01, /* Only return nodename portion for local hosts */ NI_NUMERICHOST = 0x02, /* Return numeric form of the host's address */ NI_NAMEREQD = 0x04, /* Error if the host's name not in DNS */ NI_NUMERICSERV = 0x08, /* Return numeric form of the service (port #) */ NI_DGRAM = 0x10, /* Service is a datagram service */ } // Argument structure for IPV6_ADD_MEMBERSHIP and IPV6_DROP_MEMBERSHIP. [StructLayout(LayoutKind.Sequential)] internal struct IPv6MulticastRequest { [MarshalAs(UnmanagedType.ByValArray,SizeConst=16)] internal byte[] MulticastAddress; // IP address of group internal int InterfaceIndex; // local interface index internal static readonly int Size = Marshal.SizeOf(typeof(IPv6MulticastRequest)); } // // used as last parameter to WSASocket call // [Flags] internal enum SocketConstructorFlags { WSA_FLAG_OVERLAPPED = 0x01, WSA_FLAG_MULTIPOINT_C_ROOT = 0x02, WSA_FLAG_MULTIPOINT_C_LEAF = 0x04, WSA_FLAG_MULTIPOINT_D_ROOT = 0x08, WSA_FLAG_MULTIPOINT_D_LEAF = 0x10, } } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
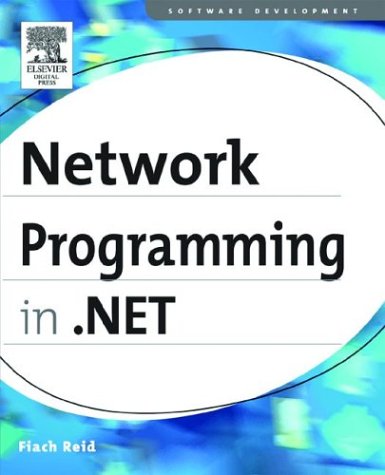
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinqDataSourceStatusEventArgs.cs
- XmlSchemas.cs
- BuilderElements.cs
- PrintPreviewControl.cs
- BevelBitmapEffect.cs
- PointLight.cs
- DefaultMemberAttribute.cs
- TextRangeEditLists.cs
- ToolStripCollectionEditor.cs
- HttpModuleActionCollection.cs
- MessageBuffer.cs
- TextSerializer.cs
- Automation.cs
- ListViewGroup.cs
- XmlSchemaProviderAttribute.cs
- SQLDecimalStorage.cs
- CodeGenerator.cs
- SqlNodeAnnotations.cs
- CodeSubDirectoriesCollection.cs
- EmptyEnumerator.cs
- Panel.cs
- ContextProperty.cs
- EventProxy.cs
- XamlStackWriter.cs
- sqlser.cs
- CultureTableRecord.cs
- DataKey.cs
- SoapClientMessage.cs
- HealthMonitoringSectionHelper.cs
- ListViewContainer.cs
- BaseComponentEditor.cs
- SmtpFailedRecipientsException.cs
- StringConcat.cs
- BufferedOutputStream.cs
- XmlTextEncoder.cs
- TemplateControl.cs
- SourceElementsCollection.cs
- SystemTcpConnection.cs
- AccessDataSourceDesigner.cs
- LassoHelper.cs
- XDeferredAxisSource.cs
- DataGridColumnHeaderAutomationPeer.cs
- UserControlDocumentDesigner.cs
- MobilePage.cs
- CqlBlock.cs
- CellLabel.cs
- XPathDocumentNavigator.cs
- MouseCaptureWithinProperty.cs
- ObjectReaderCompiler.cs
- Point3DAnimationUsingKeyFrames.cs
- UdpChannelFactory.cs
- IntSecurity.cs
- counter.cs
- ListBox.cs
- ParseChildrenAsPropertiesAttribute.cs
- CachedFontFace.cs
- WindowsFormsHelpers.cs
- NavigationPropertyEmitter.cs
- IPAddress.cs
- HttpResponse.cs
- IsolatedStorage.cs
- ToolStripLocationCancelEventArgs.cs
- ProgressBarBrushConverter.cs
- OdbcInfoMessageEvent.cs
- RedistVersionInfo.cs
- UserNameSecurityTokenProvider.cs
- HtmlControlPersistable.cs
- ListViewUpdatedEventArgs.cs
- ProfileModule.cs
- FormsAuthenticationUserCollection.cs
- FragmentQueryProcessor.cs
- SortDescription.cs
- SizeAnimationBase.cs
- UnhandledExceptionEventArgs.cs
- Border.cs
- SequentialWorkflowRootDesigner.cs
- Content.cs
- SelectionEditingBehavior.cs
- CompatibleIComparer.cs
- HttpHandlersSection.cs
- StaticFileHandler.cs
- RetrieveVirtualItemEventArgs.cs
- LateBoundBitmapDecoder.cs
- parserscommon.cs
- KeysConverter.cs
- WebPartAuthorizationEventArgs.cs
- MemberDomainMap.cs
- HMACSHA512.cs
- SeverityFilter.cs
- SourceFileBuildProvider.cs
- FloaterBaseParagraph.cs
- SevenBitStream.cs
- TypeInitializationException.cs
- StringSource.cs
- CodeConstructor.cs
- PersonalizablePropertyEntry.cs
- DelayedRegex.cs
- Tuple.cs
- CodeDomExtensionMethods.cs
- XDRSchema.cs