Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / NetworkInformation / SystemIPGlobalProperties.cs / 1 / SystemIPGlobalProperties.cs
namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System.Security.Permissions; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Threading; internal class SystemIPGlobalProperties:IPGlobalProperties { private FixedInfo fixedInfo; private bool fixedInfoInitialized = false; //changing these require a reboot, so we'll cache them instead. private static string hostName = null; private static string domainName = null; static object syncObject = new object(); internal SystemIPGlobalProperties() { } internal static FixedInfo GetFixedInfo(){ uint size = 0; SafeLocalFree buffer = null; FixedInfo fixedInfo = new FixedInfo(); //first we need to get the size of the buffer uint result = UnsafeNetInfoNativeMethods.GetNetworkParams(SafeLocalFree.Zero,ref size); while (result == IpHelperErrors.ErrorBufferOverflow) { try { //now we allocate the buffer and read the network parameters. buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetNetworkParams(buffer,ref size); if ( result == IpHelperErrors.Success ) { fixedInfo = new FixedInfo( (FIXED_INFO)Marshal.PtrToStructure(buffer.DangerousGetHandle(),typeof(FIXED_INFO))); } } finally { if(buffer != null){ buffer.Close(); } } } //if the result include there being no information, we'll still throw if (result != IpHelperErrors.Success) { throw new NetworkInformationException((int)result); } return fixedInfo; } internal FixedInfo FixedInfo { get { if (!fixedInfoInitialized) { lock(this){ if (!fixedInfoInitialized) { fixedInfo = GetFixedInfo(); fixedInfoInitialized = true; } } } return fixedInfo; } } ///Specifies the host name for the local computer. public override string HostName{ get { if(hostName == null){ lock(syncObject){ if(hostName == null){ hostName = FixedInfo.HostName; domainName = FixedInfo.DomainName; } } } return hostName; } } ///Specifies the domain in which the local computer is registered. public override string DomainName{ get { if(domainName == null){ lock(syncObject){ if(domainName == null){ hostName = FixedInfo.HostName; domainName = FixedInfo.DomainName; } } } return domainName; } } ////// The type of node. /// ////// The exact mechanism by which NetBIOS names are resolved to IP addresses /// depends on the node's configured NetBIOS Node Type. Broadcast - uses broadcast /// NetBIOS Name Queries for name registration and resolution. /// PeerToPeer - uses a NetBIOS name server (NBNS), such as Windows Internet /// Name Service (WINS), to resolve NetBIOS names. /// Mixed - uses Broadcast then PeerToPeer. /// Hybrid - uses PeerToPeer then Broadcast. /// public override NetBiosNodeType NodeType{get { return (NetBiosNodeType)FixedInfo.NodeType;} } ///Specifies the DHCP scope name. public override string DhcpScopeName{get { return FixedInfo.ScopeId;} } ///Specifies whether the local computer is acting as an WINS proxy. public override bool IsWinsProxy{get { return (FixedInfo.EnableProxy);} } public override TcpConnectionInformation[] GetActiveTcpConnections(){ ArrayList list = new ArrayList(); TcpConnectionInformation[] connections = GetAllTcpConnections(); foreach(TcpConnectionInformation connection in connections){ if(connection.State != TcpState.Listen){ list.Add(connection); } } connections = new TcpConnectionInformation[list.Count]; for (int i=0; i< list.Count;i++) { connections[i]=(TcpConnectionInformation)list[i]; } return connections; } public override IPEndPoint[] GetActiveTcpListeners (){ ArrayList list = new ArrayList(); TcpConnectionInformation[] connections = GetAllTcpConnections(); foreach(TcpConnectionInformation connection in connections){ if(connection.State == TcpState.Listen){ list.Add(connection.LocalEndPoint); } } IPEndPoint[] endPoints = new IPEndPoint[list.Count]; for (int i=0; i< list.Count;i++) { endPoints[i]=(IPEndPoint)list[i]; } return endPoints; } ////// Gets the active tcp connections. Uses the native GetTcpTable api. private TcpConnectionInformation[] GetAllTcpConnections(){ uint size = 0; SafeLocalFree buffer = null; SystemTcpConnectionInformation[] tcpConnections = null; //get the size of buffer needed uint result = UnsafeNetInfoNativeMethods.GetTcpTable(SafeLocalFree.Zero,ref size,true); while (result == IpHelperErrors.ErrorInsufficientBuffer) { try { //allocate the buffer and get the tcptable buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetTcpTable(buffer,ref size,true); if ( result == IpHelperErrors.Success ) { //the table info just gives us the number of rows. IntPtr newPtr = buffer.DangerousGetHandle(); MibTcpTable tcpTableInfo = (MibTcpTable)Marshal.PtrToStructure(newPtr,typeof(MibTcpTable)); if (tcpTableInfo.numberOfEntries > 0) { tcpConnections = new SystemTcpConnectionInformation[tcpTableInfo.numberOfEntries]; //we need to skip over the tableinfo to get the inline rows newPtr = (IntPtr)((long)newPtr + Marshal.SizeOf(tcpTableInfo.numberOfEntries)); for (int i=0;iGets the active udp listeners. Uses the native GetUdpTable api. public override IPEndPoint[] GetActiveUdpListeners(){ uint size = 0; SafeLocalFree buffer = null; IPEndPoint[] udpListeners = null; //get the size of buffer needed uint result = UnsafeNetInfoNativeMethods.GetUdpTable(SafeLocalFree.Zero,ref size,true); while (result == IpHelperErrors.ErrorInsufficientBuffer) { try { //allocate the buffer and get the udptable buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetUdpTable(buffer,ref size,true); if ( result == IpHelperErrors.Success) { //the table info just gives us the number of rows. IntPtr newPtr = buffer.DangerousGetHandle(); MibUdpTable udpTableInfo = (MibUdpTable)Marshal.PtrToStructure(newPtr,typeof(MibUdpTable)); if (udpTableInfo.numberOfEntries > 0) { udpListeners = new IPEndPoint[udpTableInfo.numberOfEntries]; //we need to skip over the tableinfo to get the inline rows newPtr = (IntPtr)((long)newPtr + Marshal.SizeOf(udpTableInfo.numberOfEntries)); for (int i=0;i Specifies the host name for the local computer. public override string HostName{ get { if(hostName == null){ lock(syncObject){ if(hostName == null){ hostName = FixedInfo.HostName; domainName = FixedInfo.DomainName; } } } return hostName; } } /// Specifies the domain in which the local computer is registered. public override string DomainName{ get { if(domainName == null){ lock(syncObject){ if(domainName == null){ hostName = FixedInfo.HostName; domainName = FixedInfo.DomainName; } } } return domainName; } } ////// The type of node. /// ////// The exact mechanism by which NetBIOS names are resolved to IP addresses /// depends on the node's configured NetBIOS Node Type. Broadcast - uses broadcast /// NetBIOS Name Queries for name registration and resolution. /// PeerToPeer - uses a NetBIOS name server (NBNS), such as Windows Internet /// Name Service (WINS), to resolve NetBIOS names. /// Mixed - uses Broadcast then PeerToPeer. /// Hybrid - uses PeerToPeer then Broadcast. /// public override NetBiosNodeType NodeType{get { return (NetBiosNodeType)FixedInfo.NodeType;} } ///Specifies the DHCP scope name. public override string DhcpScopeName{get { return FixedInfo.ScopeId;} } ///Specifies whether the local computer is acting as an WINS proxy. public override bool IsWinsProxy{get { return (FixedInfo.EnableProxy);} } public override TcpConnectionInformation[] GetActiveTcpConnections(){ ArrayList list = new ArrayList(); TcpConnectionInformation[] connections = GetAllTcpConnections(); foreach(TcpConnectionInformation connection in connections){ if(connection.State != TcpState.Listen){ list.Add(connection); } } connections = new TcpConnectionInformation[list.Count]; for (int i=0; i< list.Count;i++) { connections[i]=(TcpConnectionInformation)list[i]; } return connections; } public override IPEndPoint[] GetActiveTcpListeners (){ ArrayList list = new ArrayList(); TcpConnectionInformation[] connections = GetAllTcpConnections(); foreach(TcpConnectionInformation connection in connections){ if(connection.State == TcpState.Listen){ list.Add(connection.LocalEndPoint); } } IPEndPoint[] endPoints = new IPEndPoint[list.Count]; for (int i=0; i< list.Count;i++) { endPoints[i]=(IPEndPoint)list[i]; } return endPoints; } ////// Gets the active tcp connections. Uses the native GetTcpTable api. private TcpConnectionInformation[] GetAllTcpConnections(){ uint size = 0; SafeLocalFree buffer = null; SystemTcpConnectionInformation[] tcpConnections = null; //get the size of buffer needed uint result = UnsafeNetInfoNativeMethods.GetTcpTable(SafeLocalFree.Zero,ref size,true); while (result == IpHelperErrors.ErrorInsufficientBuffer) { try { //allocate the buffer and get the tcptable buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetTcpTable(buffer,ref size,true); if ( result == IpHelperErrors.Success ) { //the table info just gives us the number of rows. IntPtr newPtr = buffer.DangerousGetHandle(); MibTcpTable tcpTableInfo = (MibTcpTable)Marshal.PtrToStructure(newPtr,typeof(MibTcpTable)); if (tcpTableInfo.numberOfEntries > 0) { tcpConnections = new SystemTcpConnectionInformation[tcpTableInfo.numberOfEntries]; //we need to skip over the tableinfo to get the inline rows newPtr = (IntPtr)((long)newPtr + Marshal.SizeOf(tcpTableInfo.numberOfEntries)); for (int i=0;iGets the active udp listeners. Uses the native GetUdpTable api. public override IPEndPoint[] GetActiveUdpListeners(){ uint size = 0; SafeLocalFree buffer = null; IPEndPoint[] udpListeners = null; //get the size of buffer needed uint result = UnsafeNetInfoNativeMethods.GetUdpTable(SafeLocalFree.Zero,ref size,true); while (result == IpHelperErrors.ErrorInsufficientBuffer) { try { //allocate the buffer and get the udptable buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetUdpTable(buffer,ref size,true); if ( result == IpHelperErrors.Success) { //the table info just gives us the number of rows. IntPtr newPtr = buffer.DangerousGetHandle(); MibUdpTable udpTableInfo = (MibUdpTable)Marshal.PtrToStructure(newPtr,typeof(MibUdpTable)); if (udpTableInfo.numberOfEntries > 0) { udpListeners = new IPEndPoint[udpTableInfo.numberOfEntries]; //we need to skip over the tableinfo to get the inline rows newPtr = (IntPtr)((long)newPtr + Marshal.SizeOf(udpTableInfo.numberOfEntries)); for (int i=0;i
Link Menu
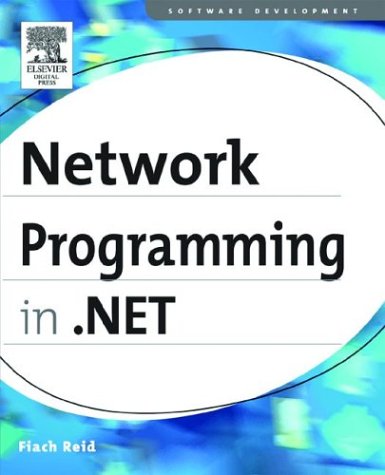
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AppDomainManager.cs
- ProxyWebPart.cs
- UnsafeNativeMethods.cs
- WindowsPrincipal.cs
- Helpers.cs
- XmlNamedNodeMap.cs
- CompilerErrorCollection.cs
- ParseHttpDate.cs
- ExcludeFromCodeCoverageAttribute.cs
- Collection.cs
- Paragraph.cs
- SqlCacheDependencyDatabaseCollection.cs
- SupportsEventValidationAttribute.cs
- ThousandthOfEmRealDoubles.cs
- DbDeleteCommandTree.cs
- ReadOnlyTernaryTree.cs
- InputProcessorProfiles.cs
- WebBaseEventKeyComparer.cs
- DocumentSequenceHighlightLayer.cs
- DesignerActionUIService.cs
- Int64KeyFrameCollection.cs
- GorillaCodec.cs
- ConfigurationValues.cs
- WebEventCodes.cs
- ResourceContainer.cs
- ComponentEvent.cs
- SectionInput.cs
- CriticalExceptions.cs
- Attributes.cs
- BooleanFunctions.cs
- SQLMoney.cs
- SecurityElementBase.cs
- TableCellAutomationPeer.cs
- DbCommandTree.cs
- CfgSemanticTag.cs
- ScriptResourceAttribute.cs
- ProfileInfo.cs
- ThreadStaticAttribute.cs
- EntitySqlQueryState.cs
- FragmentNavigationEventArgs.cs
- DriveInfo.cs
- ComponentChangingEvent.cs
- ToolBar.cs
- FieldCollectionEditor.cs
- PointF.cs
- GridSplitter.cs
- ConfigurationSettings.cs
- GeometryHitTestResult.cs
- CachedPathData.cs
- HealthMonitoringSectionHelper.cs
- DelegateHelpers.cs
- CorrelationValidator.cs
- ClientTargetCollection.cs
- WindowsImpersonationContext.cs
- ChannelEndpointElement.cs
- MediaTimeline.cs
- Rfc4050KeyFormatter.cs
- EpmContentDeSerializer.cs
- IsolatedStorageFilePermission.cs
- LoadMessageLogger.cs
- QueryRelOp.cs
- InternalMappingException.cs
- LayoutEngine.cs
- DLinqAssociationProvider.cs
- MarkupObject.cs
- MatrixAnimationUsingKeyFrames.cs
- CompiledXpathExpr.cs
- ElapsedEventArgs.cs
- RefreshInfo.cs
- TemplateLookupAction.cs
- BaseHashHelper.cs
- Freezable.cs
- DetailsViewPagerRow.cs
- HierarchicalDataBoundControlAdapter.cs
- HitTestWithGeometryDrawingContextWalker.cs
- Executor.cs
- SerializerProvider.cs
- CheckBoxBaseAdapter.cs
- SimpleLine.cs
- DetailsViewDeletedEventArgs.cs
- PathFigure.cs
- LinkedResourceCollection.cs
- Serialization.cs
- StatusCommandUI.cs
- RawStylusInput.cs
- XmlNodeComparer.cs
- FileEnumerator.cs
- ResourceAssociationType.cs
- IResourceProvider.cs
- _NestedSingleAsyncResult.cs
- TreeViewImageIndexConverter.cs
- ConnectionManagementElementCollection.cs
- OletxCommittableTransaction.cs
- HttpUnhandledOperationInvoker.cs
- XamlSerializerUtil.cs
- UnmanagedMarshal.cs
- ConstructorArgumentAttribute.cs
- CommandBindingCollection.cs
- RecordsAffectedEventArgs.cs
- CompositionTarget.cs