Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ResourceAssociationType.cs / 1305376 / ResourceAssociationType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Simple couple of classes to keep association descriptions // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System.Diagnostics; using System.Collections.Generic; ////// Stores information about a association and its ends /// internal class ResourceAssociationType { ///FullName of the association. private readonly string fullName; ///Name of the association private readonly string name; ///end1 for this association. private readonly ResourceAssociationTypeEnd end1; ///end2 for this association. private readonly ResourceAssociationTypeEnd end2; ////// Creates a new instance of AssociationInfo to store information about an association. /// /// name of the association. /// namespaceName of the association. /// first end of the association. /// second end of the association. internal ResourceAssociationType(string name, string namespaceName, ResourceAssociationTypeEnd end1, ResourceAssociationTypeEnd end2) { Debug.Assert(!String.IsNullOrEmpty(name), "!String.IsNullOrEmpty(name)"); Debug.Assert(end1 != null && end2 != null, "end1 != null && end2 != null"); this.name = name; this.fullName = namespaceName + "." + name; this.end1 = end1; this.end2 = end2; } ///FullName of the association. internal string FullName { get { return this.fullName; } } ///Name of the association. internal string Name { get { return this.name; } } ///end1 for this association. internal ResourceAssociationTypeEnd End1 { get { return this.end1; } } ///end2 for this association. internal ResourceAssociationTypeEnd End2 { get { return this.end2; } } ////// Retrieve the end for the given resource set, type and property. /// /// resource type for the end /// resource property for the end ///Association type end for the given parameters internal ResourceAssociationTypeEnd GetResourceAssociationTypeEnd(ResourceType resourceType, ResourceProperty resourceProperty) { Debug.Assert(resourceType != null, "resourceType != null"); foreach (ResourceAssociationTypeEnd end in new[] { this.end1, this.end2 }) { if (end.ResourceType == resourceType && end.ResourceProperty == resourceProperty) { return end; } } return null; } ////// Retrieve the related end for the given resource set, type and property. /// /// resource type for the source end /// resource property for the source end ///Related association type end for the given parameters internal ResourceAssociationTypeEnd GetRelatedResourceAssociationSetEnd(ResourceType resourceType, ResourceProperty resourceProperty) { Debug.Assert(resourceType != null, "resourceType != null"); ResourceAssociationTypeEnd thisEnd = this.GetResourceAssociationTypeEnd(resourceType, resourceProperty); if (thisEnd != null) { foreach (ResourceAssociationTypeEnd end in new[] { this.end1, this.end2 }) { if (end != thisEnd) { return end; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Simple couple of classes to keep association descriptions // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System.Diagnostics; using System.Collections.Generic; ////// Stores information about a association and its ends /// internal class ResourceAssociationType { ///FullName of the association. private readonly string fullName; ///Name of the association private readonly string name; ///end1 for this association. private readonly ResourceAssociationTypeEnd end1; ///end2 for this association. private readonly ResourceAssociationTypeEnd end2; ////// Creates a new instance of AssociationInfo to store information about an association. /// /// name of the association. /// namespaceName of the association. /// first end of the association. /// second end of the association. internal ResourceAssociationType(string name, string namespaceName, ResourceAssociationTypeEnd end1, ResourceAssociationTypeEnd end2) { Debug.Assert(!String.IsNullOrEmpty(name), "!String.IsNullOrEmpty(name)"); Debug.Assert(end1 != null && end2 != null, "end1 != null && end2 != null"); this.name = name; this.fullName = namespaceName + "." + name; this.end1 = end1; this.end2 = end2; } ///FullName of the association. internal string FullName { get { return this.fullName; } } ///Name of the association. internal string Name { get { return this.name; } } ///end1 for this association. internal ResourceAssociationTypeEnd End1 { get { return this.end1; } } ///end2 for this association. internal ResourceAssociationTypeEnd End2 { get { return this.end2; } } ////// Retrieve the end for the given resource set, type and property. /// /// resource type for the end /// resource property for the end ///Association type end for the given parameters internal ResourceAssociationTypeEnd GetResourceAssociationTypeEnd(ResourceType resourceType, ResourceProperty resourceProperty) { Debug.Assert(resourceType != null, "resourceType != null"); foreach (ResourceAssociationTypeEnd end in new[] { this.end1, this.end2 }) { if (end.ResourceType == resourceType && end.ResourceProperty == resourceProperty) { return end; } } return null; } ////// Retrieve the related end for the given resource set, type and property. /// /// resource type for the source end /// resource property for the source end ///Related association type end for the given parameters internal ResourceAssociationTypeEnd GetRelatedResourceAssociationSetEnd(ResourceType resourceType, ResourceProperty resourceProperty) { Debug.Assert(resourceType != null, "resourceType != null"); ResourceAssociationTypeEnd thisEnd = this.GetResourceAssociationTypeEnd(resourceType, resourceProperty); if (thisEnd != null) { foreach (ResourceAssociationTypeEnd end in new[] { this.end1, this.end2 }) { if (end != thisEnd) { return end; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
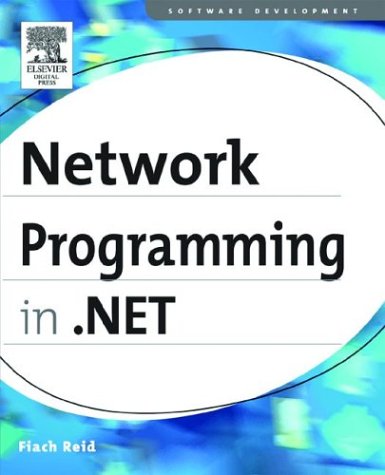
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Currency.cs
- ArraySortHelper.cs
- RuntimeResourceSet.cs
- NullableFloatMinMaxAggregationOperator.cs
- XmlEnumAttribute.cs
- SspiHelper.cs
- Logging.cs
- Cell.cs
- LinqDataSourceStatusEventArgs.cs
- SupportsPreviewControlAttribute.cs
- listitem.cs
- CompositeTypefaceMetrics.cs
- MULTI_QI.cs
- sqlstateclientmanager.cs
- Transactions.cs
- CardSpaceShim.cs
- TransformGroup.cs
- SecurityPolicySection.cs
- _Connection.cs
- WindowsTitleBar.cs
- EdmItemCollection.cs
- EntityContainerEmitter.cs
- Matrix.cs
- EmbossBitmapEffect.cs
- InputLangChangeRequestEvent.cs
- DnsEndpointIdentity.cs
- Splitter.cs
- CompilerErrorCollection.cs
- NullableDoubleSumAggregationOperator.cs
- SplashScreen.cs
- FontStretches.cs
- TargetConverter.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- ReflectionHelper.cs
- DataControlFieldCell.cs
- ButtonFlatAdapter.cs
- Psha1DerivedKeyGeneratorHelper.cs
- DictionaryCustomTypeDescriptor.cs
- GACMembershipCondition.cs
- KeyBinding.cs
- XmlParserContext.cs
- SoapInteropTypes.cs
- CharacterBufferReference.cs
- GC.cs
- RichTextBoxAutomationPeer.cs
- DataAdapter.cs
- CompositeFontFamily.cs
- SuppressMessageAttribute.cs
- VisualStyleElement.cs
- TreeNodeBinding.cs
- SystemIPInterfaceStatistics.cs
- CancellationToken.cs
- SplayTreeNode.cs
- mongolianshape.cs
- ListViewEditEventArgs.cs
- RequestTimeoutManager.cs
- HwndAppCommandInputProvider.cs
- MappedMetaModel.cs
- PolyLineSegmentFigureLogic.cs
- SQlBooleanStorage.cs
- XmlSchemaSimpleTypeRestriction.cs
- OutOfMemoryException.cs
- DatePicker.cs
- AccessibleObject.cs
- EntityDataSourceWizardForm.cs
- PeerOutputChannel.cs
- ReaderWriterLockWrapper.cs
- EventRouteFactory.cs
- PrintingPermission.cs
- Rect3DConverter.cs
- NamedPipeTransportManager.cs
- AnnouncementInnerClient11.cs
- AVElementHelper.cs
- ReadOnlyPermissionSet.cs
- IISUnsafeMethods.cs
- String.cs
- ObfuscationAttribute.cs
- XmlConverter.cs
- WebConfigurationHost.cs
- ThrowHelper.cs
- GradientSpreadMethodValidation.cs
- FontFamilyIdentifier.cs
- Solver.cs
- SQLConvert.cs
- XsdBuilder.cs
- DataServiceQueryOfT.cs
- MembershipAdapter.cs
- EmptyReadOnlyDictionaryInternal.cs
- PropertyItem.cs
- DBPropSet.cs
- IItemProperties.cs
- NotificationContext.cs
- _AutoWebProxyScriptEngine.cs
- HandlerFactoryWrapper.cs
- PreProcessInputEventArgs.cs
- WizardSideBarListControlItemEventArgs.cs
- ClientCultureInfo.cs
- DataListComponentEditor.cs
- RecognizedPhrase.cs
- PointKeyFrameCollection.cs