Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / PeerInputChannel.cs / 1 / PeerInputChannel.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Diagnostics; using System.Threading; class PeerInputChannel : InputChannel { EndpointAddress to; Uri via; PeerNode peerNode; bool released = false; public PeerInputChannel(PeerNodeImplementation peerNode, PeerNodeImplementation.Registration registration, ChannelManagerBase channelManager, EndpointAddress localAddress, Uri via) : base(channelManager, localAddress) { PeerNodeImplementation.ValidateVia(via); if(registration != null) { peerNode = PeerNodeImplementation.Get(via, registration); } this.peerNode = new PeerNode(peerNode); this.to = localAddress; this.via = via; } public override T GetProperty() { if(typeof(T) == typeof(PeerNode)) { return (T) (object) this.peerNode; } else if(typeof(T) == typeof(PeerNodeImplementation)) { return (T) (object) this.peerNode.InnerNode; } else if(typeof(T) == typeof(IOnlineStatus)) { return (T) (object) this.peerNode; } else if (typeof(T) == typeof(FaultConverter)) { return (T)(object)FaultConverter.GetDefaultFaultConverter(MessageVersion.Soap12WSAddressing10); } return base.GetProperty (); } protected override void OnAbort() { base.OnAbort(); if (this.State < CommunicationState.Closed) { try { this.peerNode.InnerNode.Abort(); } catch(Exception e) { if(DiagnosticUtility.IsFatal(e)) throw; DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { // first close the node, then the base return new ChainedAsyncResult(timeout, callback, state, OnBeginCloseNode, OnEndCloseNode, base.OnBeginClose, base.OnEndClose); } // fisrt step in the chained async close IAsyncResult OnBeginCloseNode(TimeSpan timeout, AsyncCallback callback, object state) { return this.peerNode.InnerNode.BeginClose(timeout, callback, state); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { // open the base, then the node return new ChainedAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, OnBeginOpenNode, OnEndOpenNode); } // second step in the chained async open IAsyncResult OnBeginOpenNode(TimeSpan timeout, AsyncCallback callback, object state) { IAsyncResult result = this.peerNode.InnerNode.BeginOpen(timeout, callback, state, true); return result; } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); this.peerNode.InnerNode.Close(timeoutHelper.RemainingTime()); base.OnClose(timeoutHelper.RemainingTime()); } protected override void OnClosing() { base.OnClosing(); ReleaseNode(); } void ReleaseNode() { if (!this.released) { bool release = false; lock(ThisLock) { if (!this.released) { release = this.released = true; } } if (release) { this.peerNode.InnerNode.Release(); } } } protected override void OnEndClose(IAsyncResult result) { ChainedAsyncResult.End(result); } void OnEndCloseNode(IAsyncResult result) { PeerNodeImplementation.EndClose(result); } protected override void OnEndOpen(IAsyncResult result) { ChainedAsyncResult.End(result); } void OnEndOpenNode(IAsyncResult result) { PeerNodeImplementation.EndOpen(result); } protected override void OnEnqueueItem(Message message) { // set the message's via to the uri on which it was received message.Properties.Via = this.via; if (DiagnosticUtility.ShouldTraceInformation) { TraceUtility.TraceEvent(TraceEventType.Information, TraceCode.PeerChannelMessageReceived, this, message); } } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); this.peerNode.OnOpen(); this.peerNode.InnerNode.Open(timeoutHelper.RemainingTime(), true); } protected override void OnFaulted() { base.OnFaulted(); ReleaseNode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
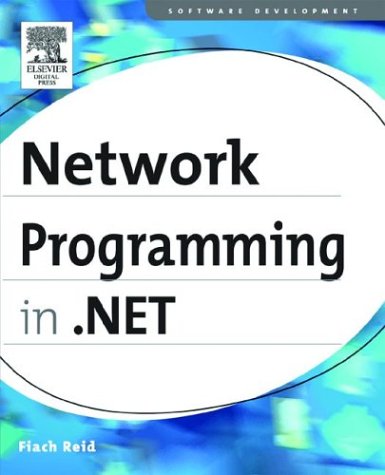
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TTSEvent.cs
- DomainConstraint.cs
- DataMemberConverter.cs
- HyperLinkColumn.cs
- ProfileSettingsCollection.cs
- UiaCoreApi.cs
- ToolStripOverflowButton.cs
- EndGetFileNameFromUserRequest.cs
- ProtocolState.cs
- FloaterParagraph.cs
- ScanQueryOperator.cs
- ParsedAttributeCollection.cs
- _NegoStream.cs
- GraphicsPathIterator.cs
- HMACSHA1.cs
- OpenTypeCommon.cs
- ExceptionHandlerDesigner.cs
- RecordManager.cs
- RegexWorker.cs
- ImageInfo.cs
- TemplateControlBuildProvider.cs
- _ShellExpression.cs
- TickBar.cs
- SchemaElementDecl.cs
- MD5HashHelper.cs
- AsymmetricKeyExchangeDeformatter.cs
- EncoderFallback.cs
- SecUtil.cs
- DesigntimeLicenseContext.cs
- PersistenceTypeAttribute.cs
- Binding.cs
- ViewBase.cs
- DataGridCommandEventArgs.cs
- NativeCppClassAttribute.cs
- KnownTypesHelper.cs
- TopClause.cs
- _DisconnectOverlappedAsyncResult.cs
- XmlLinkedNode.cs
- TailPinnedEventArgs.cs
- DrawingAttributesDefaultValueFactory.cs
- SwitchElementsCollection.cs
- User.cs
- XmlAnyAttributeAttribute.cs
- Padding.cs
- RequestChannelBinder.cs
- HttpApplication.cs
- EventDescriptorCollection.cs
- TemplateControlParser.cs
- PageVisual.cs
- TargetException.cs
- GridViewUpdateEventArgs.cs
- ImpersonationContext.cs
- CompoundFileIOPermission.cs
- QueryableDataSourceHelper.cs
- RelationshipEnd.cs
- SafeRightsManagementQueryHandle.cs
- ExceptionAggregator.cs
- ChildChangedEventArgs.cs
- CompiledIdentityConstraint.cs
- InlinedAggregationOperator.cs
- TemplateControl.cs
- TreeViewImageKeyConverter.cs
- WebPartMenu.cs
- FormDesigner.cs
- MessageQueuePermissionEntry.cs
- WebProxyScriptElement.cs
- FileDialog.cs
- SingleKeyFrameCollection.cs
- ServiceEndpointElement.cs
- SpellerInterop.cs
- Buffer.cs
- FileSecurity.cs
- SelectionEditingBehavior.cs
- SecurityCriticalDataForSet.cs
- FormViewInsertedEventArgs.cs
- SizeConverter.cs
- XmlQueryContext.cs
- AncillaryOps.cs
- LongValidator.cs
- EditorAttribute.cs
- DesignTimeParseData.cs
- BitHelper.cs
- ExchangeUtilities.cs
- HttpCookie.cs
- TextEditor.cs
- Guid.cs
- OleDbParameterCollection.cs
- _StreamFramer.cs
- OleCmdHelper.cs
- SubqueryRules.cs
- ComponentManagerBroker.cs
- SettingsAttributes.cs
- WebHttpEndpoint.cs
- ActiveDesignSurfaceEvent.cs
- SafeArrayRankMismatchException.cs
- DocumentXmlWriter.cs
- ScrollProviderWrapper.cs
- RSACryptoServiceProvider.cs
- AddressAccessDeniedException.cs
- TextBoxBase.cs