Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Attributes.cs / 2 / Attributes.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System.Runtime.InteropServices; namespace System.Security { // DynamicSecurityMethodAttribute: // Indicates that calling the target method requires space for a security // object to be allocated on the callers stack. This attribute is only ever // set on certain security methods defined within mscorlib. [AttributeUsage(AttributeTargets.Method, AllowMultiple = true, Inherited = false )] sealed internal class DynamicSecurityMethodAttribute : System.Attribute { } // SuppressUnmanagedCodeSecurityAttribute: // Indicates that the target P/Invoke method(s) should skip the per-call // security checked for unmanaged code permission. [AttributeUsage(AttributeTargets.Method | AttributeTargets.Class | AttributeTargets.Interface | AttributeTargets.Delegate, AllowMultiple = true, Inherited = false )] [System.Runtime.InteropServices.ComVisible(true)] sealed public class SuppressUnmanagedCodeSecurityAttribute : System.Attribute { } // UnverifiableCodeAttribute: // Indicates that the target module contains unverifiable code. [AttributeUsage(AttributeTargets.Module, AllowMultiple = true, Inherited = false )] [System.Runtime.InteropServices.ComVisible(true)] sealed public class UnverifiableCodeAttribute : System.Attribute { } // AllowPartiallyTrustedCallersAttribute: // Indicates that the Assembly is secure and can be used by untrusted // and semitrusted clients // For v.1, this is valid only on Assemblies, but could be expanded to // include Module, Method, class [AttributeUsage(AttributeTargets.Assembly, AllowMultiple = false, Inherited = false )] [System.Runtime.InteropServices.ComVisible(true)] sealed public class AllowPartiallyTrustedCallersAttribute : System.Attribute { public AllowPartiallyTrustedCallersAttribute () { } } public enum SecurityCriticalScope { Explicit = 0, Everything = 0x1 } // SecurityCriticalAttribute // Indicates that the decorated code or assembly performs security [AttributeUsage(AttributeTargets.Assembly | AttributeTargets.Module | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Enum | AttributeTargets.Constructor | AttributeTargets.Method | AttributeTargets.Property | AttributeTargets.Field | AttributeTargets.Event | AttributeTargets.Interface | AttributeTargets.Delegate, AllowMultiple = false, Inherited = false )] sealed public class SecurityCriticalAttribute : System.Attribute { internal SecurityCriticalScope _val; public SecurityCriticalAttribute () {} public SecurityCriticalAttribute(SecurityCriticalScope scope) { _val = scope; } public SecurityCriticalScope Scope { get { return _val; } } } // SecurityTreatAsSafeAttribute: // Indicates that the code may contain violations to the security [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false )] sealed public class SecurityTreatAsSafeAttribute : System.Attribute { public SecurityTreatAsSafeAttribute () { } } // SecuritySafeCriticalAttribute: // Indicates that the code may contain violations to the security [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false )] sealed public class SecuritySafeCriticalAttribute : System.Attribute { public SecuritySafeCriticalAttribute () { } } // SecurityTransparentAttribute: // Indicates the assembly contains only transparent code. // Security [AttributeUsage(AttributeTargets.Assembly, AllowMultiple = false, Inherited = false )] sealed public class SecurityTransparentAttribute : System.Attribute { public SecurityTransparentAttribute () {} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
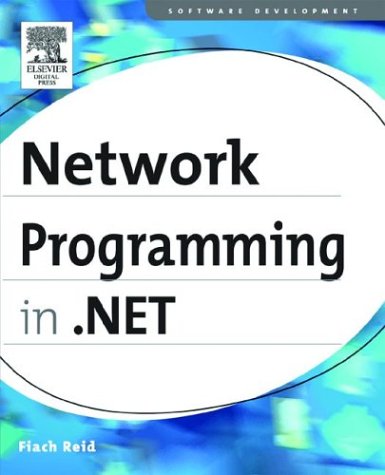
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GestureRecognitionResult.cs
- SerializerProvider.cs
- PageBuildProvider.cs
- SharedDp.cs
- DocumentXPathNavigator.cs
- DataGridViewMethods.cs
- ISessionStateStore.cs
- ProxyManager.cs
- ListViewTableRow.cs
- InstanceDataCollectionCollection.cs
- PriorityChain.cs
- WindowsSolidBrush.cs
- Size.cs
- _SslSessionsCache.cs
- FragmentNavigationEventArgs.cs
- ContentElement.cs
- UriSectionReader.cs
- CornerRadiusConverter.cs
- ParameterModifier.cs
- ColorConverter.cs
- SqlAliaser.cs
- DrawTreeNodeEventArgs.cs
- WebConfigurationHost.cs
- ListBoxItemWrapperAutomationPeer.cs
- OracleInfoMessageEventArgs.cs
- MimeTypeAttribute.cs
- TypeNameHelper.cs
- ServiceCredentialsSecurityTokenManager.cs
- Freezable.cs
- RtType.cs
- GroupDescription.cs
- CodeNamespace.cs
- ExpressionPrefixAttribute.cs
- RestHandler.cs
- FloaterParagraph.cs
- CipherData.cs
- DragAssistanceManager.cs
- CodeObjectCreateExpression.cs
- KeyEvent.cs
- ViewManager.cs
- CopyNodeSetAction.cs
- InnerItemCollectionView.cs
- Predicate.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- TransformPattern.cs
- AppearanceEditorPart.cs
- CharacterBufferReference.cs
- RegexParser.cs
- httpstaticobjectscollection.cs
- ShapeTypeface.cs
- InvalidBodyAccessException.cs
- InternalBufferOverflowException.cs
- SpellerError.cs
- GridViewCancelEditEventArgs.cs
- HostedTcpTransportManager.cs
- WaitingCursor.cs
- PermissionListSet.cs
- UserValidatedEventArgs.cs
- MailMessage.cs
- GlyphInfoList.cs
- DebuggerAttributes.cs
- MaskedTextBoxTextEditorDropDown.cs
- VBIdentifierNameEditor.cs
- HtmlInputButton.cs
- XmlUtilWriter.cs
- PeerToPeerException.cs
- DispatcherEventArgs.cs
- AnimatedTypeHelpers.cs
- ObjectQueryProvider.cs
- TPLETWProvider.cs
- TextPatternIdentifiers.cs
- OleDbCommandBuilder.cs
- baseshape.cs
- Set.cs
- ToggleButton.cs
- FixedPosition.cs
- EventLogTraceListener.cs
- ZoomPercentageConverter.cs
- ApplicationManager.cs
- X509UI.cs
- MappingModelBuildProvider.cs
- SequentialOutput.cs
- InternalException.cs
- TableSectionStyle.cs
- FeatureManager.cs
- AdornerDecorator.cs
- ReliableSession.cs
- OutputCacheModule.cs
- ConnectionPoolManager.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- AnnotationDocumentPaginator.cs
- PocoEntityKeyStrategy.cs
- XslAst.cs
- AutomationIdentifierGuids.cs
- EntityContainerEmitter.cs
- AssemblyBuilder.cs
- BuildProvider.cs
- StatusBar.cs
- SelectionListDesigner.cs
- Root.cs