Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / FormatStringEditor.cs / 1 / FormatStringEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.FormatStringEditor..ctor()")] namespace System.Windows.Forms.Design { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms; ////// /// internal class FormatStringEditor : UITypeEditor { private FormatStringDialog formatStringDialog; ///Provides an editor to edit advanced binding objects. ////// /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { DataGridViewCellStyle dgvCellStyle = context.Instance as DataGridViewCellStyle; ListControl listControl = context.Instance as ListControl; Debug.Assert(listControl != null || dgvCellStyle != null, "this editor is used for the DataGridViewCellStyle::Format and the ListControl::FormatString properties"); if (formatStringDialog == null) { formatStringDialog = new FormatStringDialog(context); } if (listControl != null) { formatStringDialog.ListControl = listControl; } else { formatStringDialog.DataGridViewCellStyle = dgvCellStyle; } IComponentChangeService changeSvc = (IComponentChangeService)provider.GetService(typeof(IComponentChangeService)); if (changeSvc != null) { if (dgvCellStyle != null) { changeSvc.OnComponentChanging(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["Format"]); changeSvc.OnComponentChanging(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["NullValue"]); changeSvc.OnComponentChanging(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["FormatProvider"]); } else { changeSvc.OnComponentChanging(listControl, TypeDescriptor.GetProperties(listControl)["FormatString"]); changeSvc.OnComponentChanging(listControl, TypeDescriptor.GetProperties(listControl)["FormatInfo"]); } } edSvc.ShowDialog(formatStringDialog); formatStringDialog.End(); if (formatStringDialog.Dirty) { // since the bindings may have changed, the properties listed in the properties window // need to be refreshed TypeDescriptor.Refresh(context.Instance); if (changeSvc != null) { if (dgvCellStyle != null) { changeSvc.OnComponentChanged(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["Format"], null, null); changeSvc.OnComponentChanged(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["NullValue"], null, null); changeSvc.OnComponentChanged(dgvCellStyle, TypeDescriptor.GetProperties(dgvCellStyle)["FormatProvider"], null, null); } else { changeSvc.OnComponentChanged(listControl, TypeDescriptor.GetProperties(listControl)["FormatString"], null, null); changeSvc.OnComponentChanged(listControl, TypeDescriptor.GetProperties(listControl)["FormatInfo"], null, null); } } } } } return value; } ///Edits the specified value using the specified provider /// within the specified context. ////// /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Gets the edit style from the current context. ///
Link Menu
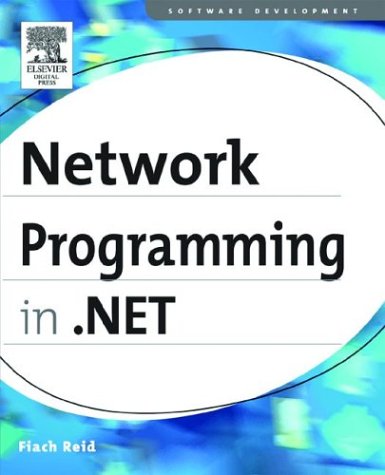
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LexicalChunk.cs
- OdbcParameterCollection.cs
- SqlCaseSimplifier.cs
- StringPropertyBuilder.cs
- WebPartConnectionsDisconnectVerb.cs
- ISFTagAndGuidCache.cs
- cache.cs
- SafeRightsManagementSessionHandle.cs
- ListSortDescription.cs
- FastEncoderWindow.cs
- ToolStripItemRenderEventArgs.cs
- ConnectionInterfaceCollection.cs
- EditorPartChrome.cs
- XmlSchemaAny.cs
- EntityContainer.cs
- SendActivity.cs
- ObjectResult.cs
- DocumentReference.cs
- PassportAuthentication.cs
- SymbolMethod.cs
- XmlEntity.cs
- SamlAttributeStatement.cs
- SqlIdentifier.cs
- Glyph.cs
- WindowsGraphicsCacheManager.cs
- ListControl.cs
- XPathParser.cs
- SystemColors.cs
- FreezableCollection.cs
- TextServicesProperty.cs
- ConstructorExpr.cs
- MarkupObject.cs
- ListViewItem.cs
- SuspendDesigner.cs
- Accessible.cs
- Properties.cs
- UInt16.cs
- SoapObjectWriter.cs
- ChangesetResponse.cs
- DiscoveryClientChannelBase.cs
- CollectionChangeEventArgs.cs
- EventSourceCreationData.cs
- ConsoleTraceListener.cs
- StylusButtonEventArgs.cs
- EditorZoneBase.cs
- BitmapMetadataBlob.cs
- SecurityAlgorithmSuiteConverter.cs
- PolicyStatement.cs
- LocatorGroup.cs
- WasAdminWrapper.cs
- GZipDecoder.cs
- ReservationCollection.cs
- TcpStreams.cs
- FigureParagraph.cs
- MenuItemBinding.cs
- XsltSettings.cs
- WebPartVerb.cs
- XmlTextWriter.cs
- XmlValidatingReaderImpl.cs
- ObjectDataSourceMethodEventArgs.cs
- MutexSecurity.cs
- NullableConverter.cs
- MetadataArtifactLoaderCompositeResource.cs
- SHA256Managed.cs
- DesignerMetadata.cs
- CompilationUtil.cs
- PersonalizablePropertyEntry.cs
- Graphics.cs
- ScriptControlManager.cs
- ImageKeyConverter.cs
- HwndSourceKeyboardInputSite.cs
- sqlpipe.cs
- ListViewHitTestInfo.cs
- Delegate.cs
- RoleManagerModule.cs
- ClientRuntimeConfig.cs
- PropertyInfoSet.cs
- GatewayDefinition.cs
- ProfileInfo.cs
- ellipse.cs
- QilPatternVisitor.cs
- CharConverter.cs
- DoubleConverter.cs
- ParameterCollection.cs
- TypefaceCollection.cs
- NavigationWindow.cs
- ProviderIncompatibleException.cs
- UrlMappingsModule.cs
- ActivitiesCollection.cs
- ToolStripDropDownItem.cs
- DataServiceEntityAttribute.cs
- DataGridViewRowCollection.cs
- COM2ComponentEditor.cs
- HostSecurityManager.cs
- DynamicValueConverter.cs
- ControlValuePropertyAttribute.cs
- OptimizerPatterns.cs
- XmlObjectSerializerReadContextComplex.cs
- XmlName.cs
- InputGestureCollection.cs