Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RemoteX509AsymmetricSecurityKey.cs / 1 / RemoteX509AsymmetricSecurityKey.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Remotes all PrivateKey operations to the RPC service running in the agent. // Any public key operations are performed with the local public key // class RemoteX509AsymmetricSecurityKey : X509AsymmetricSecurityKey, IDisposable { X509Certificate2 m_cert; object m_sync; bool m_disposed; public RemoteX509AsymmetricSecurityKey(X509Certificate2 cert) : base( cert ) { m_cert = cert; // // If the key is not our special remote key, we shouldn't be using // this object. // if( !( m_cert.PrivateKey is RemoteCryptoRsaServiceProvider) ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } m_sync = new object(); } // // Summary: // Return a specified key set. // // Arguments: // algorithmUri: the name of the algorithm // privateKey: indicates if the private key (remote key) is returned. // public override AsymmetricAlgorithm GetAsymmetricAlgorithm(string algorithmUri, bool privateKey) { if( !IsSupportedAlgorithm( algorithmUri ) ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } return base.GetAsymmetricAlgorithm( algorithmUri, privateKey ); } // // Summary: // Returns the Signature verifier // public override AsymmetricSignatureDeformatter GetSignatureDeformatter(string algorithmUri) { if( !IsSupportedAlgorithm( algorithmUri ) ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } // // This will use the public key, which we don't need to wrap. // return base.GetSignatureDeformatter( algorithmUri ); } // // Summary: // Returns the Signature creator // public override AsymmetricSignatureFormatter GetSignatureFormatter(string algorithmUri) { if( !IsSupportedAlgorithm( algorithmUri ) ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } // // The private key object must be our special private key. // RemoteAsymmetricSignatureFormatter formatter = new RemoteAsymmetricSignatureFormatter(); formatter.SetKey( m_cert.PrivateKey ); return formatter; } // // Summary: // Tests if a specific algorithm is supported. // public override bool IsSupportedAlgorithm(string algorithmUri) { switch (algorithmUri) { case SignedXml.XmlDsigRSASHA1Url: case EncryptedXml.XmlEncRSA15Url: case EncryptedXml.XmlEncRSAOAEPUrl: return base.IsSupportedAlgorithm( algorithmUri ); case SignedXml.XmlDsigDSAUrl: default: return false; } } void IDisposable.Dispose() { if ( m_disposed ) { return; } lock( m_sync ) { if( m_disposed ) { return; } m_disposed = true; IDisposable disposable = m_cert.PrivateKey as IDisposable; if( null != disposable ) { disposable.Dispose(); } disposable = m_cert.PublicKey.Key as IDisposable; if( null != disposable ) { disposable.Dispose(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
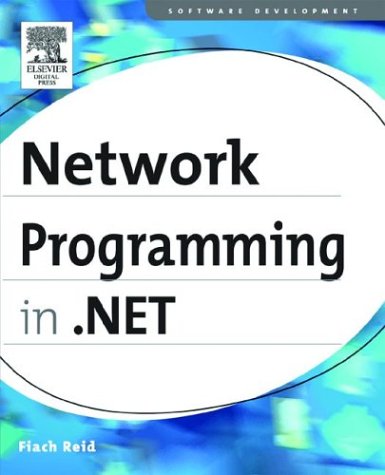
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OpCodes.cs
- SystemFonts.cs
- InteropBitmapSource.cs
- ControlIdConverter.cs
- FileUtil.cs
- LinqDataView.cs
- PageHandlerFactory.cs
- XslTransform.cs
- StartUpEventArgs.cs
- JavaScriptString.cs
- HttpWrapper.cs
- PointAnimationUsingPath.cs
- RequestQueryParser.cs
- ProfileGroupSettings.cs
- ProfileSettingsCollection.cs
- RowType.cs
- PenLineJoinValidation.cs
- ChangePassword.cs
- ValidationRuleCollection.cs
- SharedUtils.cs
- HttpListenerPrefixCollection.cs
- EventlogProvider.cs
- ShaderEffect.cs
- AssociationSet.cs
- MimeTypeAttribute.cs
- CalendarDateRangeChangingEventArgs.cs
- InvalidOperationException.cs
- CodeArrayIndexerExpression.cs
- CodeFieldReferenceExpression.cs
- Color.cs
- BindingNavigatorDesigner.cs
- RegexFCD.cs
- RequestSecurityTokenForGetBrowserToken.cs
- SourceChangedEventArgs.cs
- TableAutomationPeer.cs
- UnionExpr.cs
- SafeNativeMethods.cs
- DeadCharTextComposition.cs
- SizeChangedEventArgs.cs
- Helper.cs
- HttpModuleCollection.cs
- StrokeCollectionDefaultValueFactory.cs
- RegexBoyerMoore.cs
- MailAddress.cs
- TouchEventArgs.cs
- MasterPageParser.cs
- ItemContainerGenerator.cs
- login.cs
- SplashScreenNativeMethods.cs
- ByteKeyFrameCollection.cs
- StackOverflowException.cs
- ListItemViewAttribute.cs
- CodeTryCatchFinallyStatement.cs
- DataBoundLiteralControl.cs
- TemplateBindingExpressionConverter.cs
- ThrowHelper.cs
- RecordManager.cs
- MainMenu.cs
- RTTypeWrapper.cs
- ContactManager.cs
- TextLineBreak.cs
- MimeBasePart.cs
- CompositeControl.cs
- LoginUtil.cs
- XmlSchemaNotation.cs
- XmlRootAttribute.cs
- DrawingAttributeSerializer.cs
- MarkupCompiler.cs
- HwndHost.cs
- ProcessHost.cs
- FullTextBreakpoint.cs
- StringInfo.cs
- DbProviderFactoriesConfigurationHandler.cs
- WebPartMovingEventArgs.cs
- EventEntry.cs
- RegistryPermission.cs
- GridLengthConverter.cs
- PkcsUtils.cs
- Grant.cs
- BitmapEncoder.cs
- WebPartEditorOkVerb.cs
- AppDomainFactory.cs
- Helper.cs
- DesignerLoader.cs
- SmtpNtlmAuthenticationModule.cs
- DataKey.cs
- Visual.cs
- ObjectDataProvider.cs
- PropertyKey.cs
- ClientSettingsSection.cs
- CompensatableTransactionScopeActivity.cs
- HttpDebugHandler.cs
- SoapSchemaExporter.cs
- TextSelectionHelper.cs
- XmlAttributeProperties.cs
- UnsignedPublishLicense.cs
- Adorner.cs
- OleDbConnectionInternal.cs
- AppSettingsExpressionBuilder.cs
- ErrorWrapper.cs