Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Generated / GeometryDrawing.cs / 2 / GeometryDrawing.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class GeometryDrawing : Drawing { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GeometryDrawing Clone() { return (GeometryDrawing)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GeometryDrawing CloneCurrentValue() { return (GeometryDrawing)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void BrushPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (e.IsASubPropertyChange) { return; } GeometryDrawing target = ((GeometryDrawing) d); Brush oldV = (Brush) e.OldValue; Brush newV = (Brush) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(BrushProperty); } private static void PenPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (e.IsASubPropertyChange) { return; } GeometryDrawing target = ((GeometryDrawing) d); Pen oldV = (Pen) e.OldValue; Pen newV = (Pen) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(PenProperty); } private static void GeometryPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (e.IsASubPropertyChange) { return; } GeometryDrawing target = ((GeometryDrawing) d); Geometry oldV = (Geometry) e.OldValue; Geometry newV = (Geometry) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(GeometryProperty); } #region Public Properties ////// Brush - Brush. Default value is null. /// public Brush Brush { get { return (Brush) GetValue(BrushProperty); } set { SetValueInternal(BrushProperty, value); } } ////// Pen - Pen. Default value is null. /// public Pen Pen { get { return (Pen) GetValue(PenProperty); } set { SetValueInternal(PenProperty, value); } } ////// Geometry - Geometry. Default value is null. /// public Geometry Geometry { get { return (Geometry) GetValue(GeometryProperty); } set { SetValueInternal(GeometryProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeometryDrawing(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Brush vBrush = Brush; Pen vPen = Pen; Geometry vGeometry = Geometry; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hBrush = vBrush != null ? ((DUCE.IResource)vBrush).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hPen = vPen != null ? ((DUCE.IResource)vPen).GetHandle(channel) : DUCE.ResourceHandle.Null; DUCE.ResourceHandle hGeometry = vGeometry != null ? ((DUCE.IResource)vGeometry).GetHandle(channel) : DUCE.ResourceHandle.Null; // Pack & send command packet DUCE.MILCMD_GEOMETRYDRAWING data; unsafe { data.Type = MILCMD.MilCmdGeometryDrawing; data.Handle = _duceResource.GetHandle(channel); data.hBrush = hBrush; data.hPen = hPen; data.hGeometry = hGeometry; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_GEOMETRYDRAWING)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_GEOMETRYDRAWING)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).AddRefOnChannel(channel); Pen vPen = Pen; if (vPen != null) ((DUCE.IResource)vPen).AddRefOnChannel(channel); Geometry vGeometry = Geometry; if (vGeometry != null) ((DUCE.IResource)vGeometry).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).ReleaseOnChannel(channel); Pen vPen = Pen; if (vPen != null) ((DUCE.IResource)vPen).ReleaseOnChannel(channel); Geometry vGeometry = Geometry; if (vGeometry != null) ((DUCE.IResource)vGeometry).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GeometryDrawing.Brush property. /// public static readonly DependencyProperty BrushProperty = RegisterProperty("Brush", typeof(Brush), typeof(GeometryDrawing), null, new PropertyChangedCallback(BrushPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GeometryDrawing.Pen property. /// public static readonly DependencyProperty PenProperty = RegisterProperty("Pen", typeof(Pen), typeof(GeometryDrawing), null, new PropertyChangedCallback(PenPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GeometryDrawing.Geometry property. /// public static readonly DependencyProperty GeometryProperty = RegisterProperty("Geometry", typeof(Geometry), typeof(GeometryDrawing), null, new PropertyChangedCallback(GeometryPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
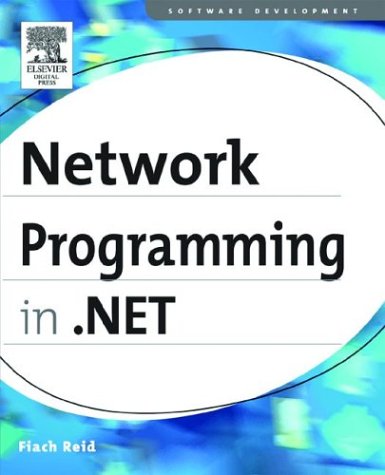
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MobileUserControl.cs
- XomlCompiler.cs
- SizeIndependentAnimationStorage.cs
- Mouse.cs
- NamedObject.cs
- ConfigurationPropertyAttribute.cs
- HttpException.cs
- DetailsViewInsertedEventArgs.cs
- XmlConverter.cs
- SettingsBase.cs
- ProfileGroupSettingsCollection.cs
- CapabilitiesRule.cs
- TextBlockAutomationPeer.cs
- Array.cs
- BaseCodeDomTreeGenerator.cs
- HelpProvider.cs
- ToolStripProgressBar.cs
- CommandValueSerializer.cs
- AccessDataSourceView.cs
- CodeLinePragma.cs
- DataGridViewComboBoxCell.cs
- OdbcConnectionHandle.cs
- OLEDB_Enum.cs
- ViewBox.cs
- StatusBarPanel.cs
- StringConverter.cs
- Rotation3DAnimation.cs
- CompositionDesigner.cs
- SortedList.cs
- XmlDataImplementation.cs
- PathFigureCollection.cs
- Trigger.cs
- SiblingIterators.cs
- InheritanceContextHelper.cs
- MultiByteCodec.cs
- SchemaImporterExtension.cs
- DefaultSerializationProviderAttribute.cs
- PermissionListSet.cs
- NonSerializedAttribute.cs
- TabPanel.cs
- MessageBox.cs
- MonitorWrapper.cs
- FlowDocumentReaderAutomationPeer.cs
- Table.cs
- ObjectDesignerDataSourceView.cs
- HtmlLinkAdapter.cs
- Application.cs
- PeerReferralPolicy.cs
- DbMetaDataColumnNames.cs
- WebPartEditorCancelVerb.cs
- XmlBoundElement.cs
- CodeDelegateCreateExpression.cs
- SiteMapNodeCollection.cs
- Column.cs
- ThreadNeutralSemaphore.cs
- CfgParser.cs
- GatewayIPAddressInformationCollection.cs
- ApplyImportsAction.cs
- WebPartConnectVerb.cs
- HtmlContainerControl.cs
- StringStorage.cs
- SecurityTokenAttachmentMode.cs
- BaseDataBoundControl.cs
- SqlUDTStorage.cs
- SolidBrush.cs
- ComponentCollection.cs
- StatusBarItem.cs
- WebPartVerbsEventArgs.cs
- ActivityStatusChangeEventArgs.cs
- BindingMemberInfo.cs
- WorkflowRuntimeService.cs
- XamlDebuggerXmlReader.cs
- CookielessHelper.cs
- DecimalAnimationUsingKeyFrames.cs
- Profiler.cs
- CompareValidator.cs
- OrderedHashRepartitionEnumerator.cs
- ObjectDataSourceSelectingEventArgs.cs
- ExeConfigurationFileMap.cs
- EditCommandColumn.cs
- Binding.cs
- MembershipSection.cs
- SubqueryRules.cs
- WorkflowViewService.cs
- TreeNode.cs
- LinqDataSourceInsertEventArgs.cs
- x509utils.cs
- VSWCFServiceContractGenerator.cs
- _SafeNetHandles.cs
- WinEventWrap.cs
- Types.cs
- CqlLexerHelpers.cs
- DefaultPropertyAttribute.cs
- RuntimeUtils.cs
- RequestQueryParser.cs
- InputMethodStateChangeEventArgs.cs
- TextSchema.cs
- ActivityBindForm.Designer.cs
- ThrowHelper.cs
- PackWebRequestFactory.cs