Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Resources / __FastResourceComparer.cs / 1 / __FastResourceComparer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FastResourceComparer ** ** ** Purpose: A collection of quick methods for comparing ** resource keys (strings) ** ** ===========================================================*/ namespace System.Resources { using System; using System.Collections; using System.Collections.Generic; internal sealed class FastResourceComparer : IComparer, IEqualityComparer, IComparer, IEqualityComparer { internal static readonly FastResourceComparer Default = new FastResourceComparer(); // Implements IHashCodeProvider too, due to Hashtable requirements. public int GetHashCode(Object key) { String s = (String) key; return FastResourceComparer.HashFunction(s); } public int GetHashCode(String key) { return FastResourceComparer.HashFunction(key); } // This hash function MUST be publically documented with the resource // file format, AND we may NEVER change this hash function's return // value (without changing the file format). internal static int HashFunction(String key) { // Never change this hash function. We must standardize it so that // others can read & write our .resources files. Additionally, we // have a copy of it in InternalResGen as well. uint hash = 5381; for(int i=0; i bCharLength) numChars = bCharLength; if (bCharLength == 0) // Can't use fixed on a 0-element array. return (a.Length == 0) ? 0 : -1; fixed(byte* pb = bytes) { byte *pChar = pb; while (i < numChars && r == 0) { // little endian format int b = pChar[0] | pChar[1] << 8; r = a[i++] - b; pChar += sizeof(char); } } if (r != 0) return r; return a.Length - bCharLength; } public static int CompareOrdinal(byte[] bytes, int aCharLength, String b) { return -CompareOrdinal(b, bytes, aCharLength); } // This method is to handle potentially misaligned data accesses. // The byte* must point to little endian Unicode characters. internal unsafe static int CompareOrdinal(byte* a, int byteLen, String b) { BCLDebug.Assert((byteLen & 1) == 0, "CompareOrdinal is expecting a UTF-16 string length, which must be even!"); BCLDebug.Assert(a != null && b != null, "Null args not allowed."); BCLDebug.Assert(byteLen >= 0, "byteLen must be non-negative."); int r = 0; int i = 0; // Compare the min length # of characters, then return length diffs. int numChars = byteLen >> 1; if (numChars > b.Length) numChars = b.Length; while(i < numChars && r == 0) { // Must compare character by character, not byte by byte. char aCh = (char) (*a++ | (*a++ << 8)); r = aCh - b[i++]; } if (r != 0) return r; return byteLen - b.Length * 2; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FastResourceComparer ** ** ** Purpose: A collection of quick methods for comparing ** resource keys (strings) ** ** ===========================================================*/ namespace System.Resources { using System; using System.Collections; using System.Collections.Generic; internal sealed class FastResourceComparer : IComparer, IEqualityComparer, IComparer , IEqualityComparer { internal static readonly FastResourceComparer Default = new FastResourceComparer(); // Implements IHashCodeProvider too, due to Hashtable requirements. public int GetHashCode(Object key) { String s = (String) key; return FastResourceComparer.HashFunction(s); } public int GetHashCode(String key) { return FastResourceComparer.HashFunction(key); } // This hash function MUST be publically documented with the resource // file format, AND we may NEVER change this hash function's return // value (without changing the file format). internal static int HashFunction(String key) { // Never change this hash function. We must standardize it so that // others can read & write our .resources files. Additionally, we // have a copy of it in InternalResGen as well. uint hash = 5381; for(int i=0; i bCharLength) numChars = bCharLength; if (bCharLength == 0) // Can't use fixed on a 0-element array. return (a.Length == 0) ? 0 : -1; fixed(byte* pb = bytes) { byte *pChar = pb; while (i < numChars && r == 0) { // little endian format int b = pChar[0] | pChar[1] << 8; r = a[i++] - b; pChar += sizeof(char); } } if (r != 0) return r; return a.Length - bCharLength; } public static int CompareOrdinal(byte[] bytes, int aCharLength, String b) { return -CompareOrdinal(b, bytes, aCharLength); } // This method is to handle potentially misaligned data accesses. // The byte* must point to little endian Unicode characters. internal unsafe static int CompareOrdinal(byte* a, int byteLen, String b) { BCLDebug.Assert((byteLen & 1) == 0, "CompareOrdinal is expecting a UTF-16 string length, which must be even!"); BCLDebug.Assert(a != null && b != null, "Null args not allowed."); BCLDebug.Assert(byteLen >= 0, "byteLen must be non-negative."); int r = 0; int i = 0; // Compare the min length # of characters, then return length diffs. int numChars = byteLen >> 1; if (numChars > b.Length) numChars = b.Length; while(i < numChars && r == 0) { // Must compare character by character, not byte by byte. char aCh = (char) (*a++ | (*a++ << 8)); r = aCh - b[i++]; } if (r != 0) return r; return byteLen - b.Length * 2; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
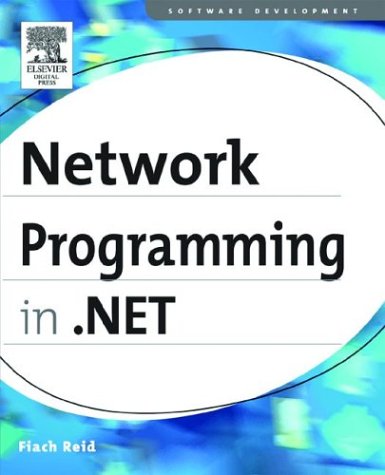
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OpenFileDialog.cs
- ConnectionInterfaceCollection.cs
- UIHelper.cs
- QuaternionIndependentAnimationStorage.cs
- ProviderBase.cs
- TraceSection.cs
- codemethodreferenceexpression.cs
- IdentityReference.cs
- DecimalAnimation.cs
- UseAttributeSetsAction.cs
- Selector.cs
- ReadWriteSpinLock.cs
- StringUtil.cs
- SqlCrossApplyToCrossJoin.cs
- Int16KeyFrameCollection.cs
- WebCodeGenerator.cs
- __Filters.cs
- Solver.cs
- DetailsViewDeleteEventArgs.cs
- InputBinder.cs
- ElementNotAvailableException.cs
- InlinedLocationReference.cs
- CacheDependency.cs
- AttributedMetaModel.cs
- ToolStripDropDownButton.cs
- XmlLanguage.cs
- COAUTHIDENTITY.cs
- ProgressBarAutomationPeer.cs
- DefaultMergeHelper.cs
- BrushConverter.cs
- XmlSerializerFactory.cs
- LicFileLicenseProvider.cs
- AsyncStreamReader.cs
- KeyboardNavigation.cs
- EdmConstants.cs
- StandardCommandToolStripMenuItem.cs
- SessionStateItemCollection.cs
- OciLobLocator.cs
- ComboBoxItem.cs
- IIS7WorkerRequest.cs
- GetPageCompletedEventArgs.cs
- PerfCounters.cs
- WebScriptEndpoint.cs
- EntityDataSourceColumn.cs
- LifetimeServices.cs
- OletxDependentTransaction.cs
- RedirectionProxy.cs
- DesignerAttribute.cs
- SoundPlayerAction.cs
- WorkflowWebService.cs
- AddInAttribute.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ClusterRegistryConfigurationProvider.cs
- TdsValueSetter.cs
- DocumentApplicationDocumentViewer.cs
- GetReadStreamResult.cs
- CommandLibraryHelper.cs
- ParseChildrenAsPropertiesAttribute.cs
- BaseCodeDomTreeGenerator.cs
- DrawingServices.cs
- WebPartHeaderCloseVerb.cs
- WSSecurityXXX2005.cs
- TransformPatternIdentifiers.cs
- Util.cs
- SqlBuilder.cs
- Point3DConverter.cs
- TextEditorCopyPaste.cs
- cookiecontainer.cs
- FileLogRecordHeader.cs
- EventListenerClientSide.cs
- PlatformCulture.cs
- HWStack.cs
- RowsCopiedEventArgs.cs
- OrderedEnumerableRowCollection.cs
- WindowsTitleBar.cs
- CreateUserWizard.cs
- UserCancellationException.cs
- EditorPartCollection.cs
- LocalFileSettingsProvider.cs
- CodeIdentifier.cs
- QueryTask.cs
- BooleanConverter.cs
- GridViewColumnCollection.cs
- EncoderReplacementFallback.cs
- DataServices.cs
- ErasingStroke.cs
- RootBrowserWindowAutomationPeer.cs
- UpdateExpressionVisitor.cs
- FileEnumerator.cs
- DesignerDataTable.cs
- TrackingAnnotationCollection.cs
- COM2TypeInfoProcessor.cs
- WinFormsSecurity.cs
- MethodRental.cs
- Selector.cs
- ProcessingInstructionAction.cs
- XmlNodeList.cs
- ClientRoleProvider.cs
- SmiRecordBuffer.cs
- InputReportEventArgs.cs