Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / Int64AnimationBase.cs / 1305600 / Int64AnimationBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class Int64AnimationBase : AnimationTimeline { #region Constructors ////// Creates a new Int64AnimationBase. /// protected Int64AnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this Int64AnimationBase /// ///The copy public new Int64AnimationBase Clone() { return (Int64AnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Int64)defaultOriginValue, (Int64)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Int64); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Int64 GetCurrentValue(Int64 defaultOriginValue, Int64 defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueInt64(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Int64 GetCurrentValueCore(Int64 defaultOriginValue, Int64 defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class Int64AnimationBase : AnimationTimeline { #region Constructors ////// Creates a new Int64AnimationBase. /// protected Int64AnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this Int64AnimationBase /// ///The copy public new Int64AnimationBase Clone() { return (Int64AnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Int64)defaultOriginValue, (Int64)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Int64); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Int64 GetCurrentValue(Int64 defaultOriginValue, Int64 defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueInt64(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Int64 GetCurrentValueCore(Int64 defaultOriginValue, Int64 defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
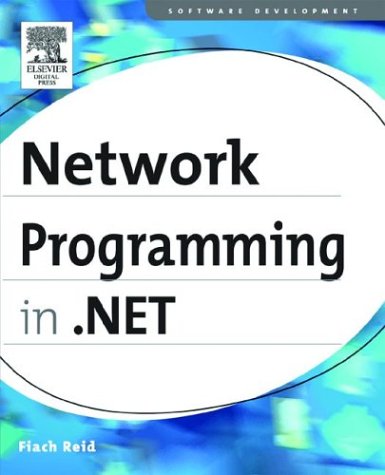
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlyphElement.cs
- WaitHandleCannotBeOpenedException.cs
- VirtualDirectoryMapping.cs
- MimeWriter.cs
- UidPropertyAttribute.cs
- ItemDragEvent.cs
- FrameworkElementFactory.cs
- HostingPreferredMapPath.cs
- CalendarDataBindingHandler.cs
- BamlLocalizationDictionary.cs
- SystemBrushes.cs
- CallSiteBinder.cs
- MessageSecurityOverHttpElement.cs
- ProjectedSlot.cs
- DESCryptoServiceProvider.cs
- WebSysDescriptionAttribute.cs
- SqlError.cs
- PeerName.cs
- InvokeWebService.cs
- QueryContinueDragEventArgs.cs
- SqlConnection.cs
- WebHttpBindingElement.cs
- ParseHttpDate.cs
- DesignerForm.cs
- ApplicationHost.cs
- CustomWebEventKey.cs
- ButtonField.cs
- ProgressBarHighlightConverter.cs
- BitmapEffect.cs
- ParameterCollection.cs
- ApplicationFileParser.cs
- RtfFormatStack.cs
- ToolStripSplitStackLayout.cs
- ScriptingProfileServiceSection.cs
- CallbackHandler.cs
- InvalidWMPVersionException.cs
- ToolStripSplitStackLayout.cs
- DES.cs
- RemoteWebConfigurationHost.cs
- InternalCache.cs
- BoundField.cs
- validation.cs
- BaseAsyncResult.cs
- MasterPageCodeDomTreeGenerator.cs
- PageMediaType.cs
- IncrementalReadDecoders.cs
- TreeNodeStyleCollection.cs
- KeyValueSerializer.cs
- DynamicQueryableWrapper.cs
- BaseDataList.cs
- CodeGeneratorOptions.cs
- XmlSchemaNotation.cs
- MailFileEditor.cs
- BitmapEffectGeneralTransform.cs
- WebServiceData.cs
- AnimatedTypeHelpers.cs
- TimeoutTimer.cs
- MimeObjectFactory.cs
- Timer.cs
- XMLSyntaxException.cs
- GridView.cs
- LoginName.cs
- ApplicationException.cs
- ConstantExpression.cs
- AttributeCollection.cs
- OdbcPermission.cs
- OrderingExpression.cs
- ThrowHelper.cs
- QueueProcessor.cs
- RunInstallerAttribute.cs
- ControlValuePropertyAttribute.cs
- KeyboardEventArgs.cs
- RawUIStateInputReport.cs
- EndPoint.cs
- Configuration.cs
- Slider.cs
- MouseGestureConverter.cs
- ChildDocumentBlock.cs
- MulticastIPAddressInformationCollection.cs
- BooleanFunctions.cs
- LambdaCompiler.Address.cs
- MessagePropertyVariants.cs
- ToolboxSnapDragDropEventArgs.cs
- HeaderFilter.cs
- activationcontext.cs
- _NativeSSPI.cs
- util.cs
- XPathSelfQuery.cs
- Misc.cs
- HideDisabledControlAdapter.cs
- HtmlImage.cs
- BinaryFormatterWriter.cs
- FileSystemEventArgs.cs
- TextOptionsInternal.cs
- DataBoundLiteralControl.cs
- PersonalizationStateInfo.cs
- GeometryGroup.cs
- WebPartZone.cs
- WorkflowTerminatedException.cs
- ButtonPopupAdapter.cs