Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / TagMapInfo.cs / 1 / TagMapInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.UI; using System.Web.Compilation; using System.Threading; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TagMapInfo : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTagTypeName = new ConfigurationProperty("tagType", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propMappedTagTypeName = new ConfigurationProperty("mappedTagType", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); static TagMapInfo() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTagTypeName); _properties.Add(_propMappedTagTypeName); } internal TagMapInfo() { } public TagMapInfo(String tagTypeName, String mappedTagTypeName) : this() { TagType = tagTypeName; MappedTagType = mappedTagTypeName; } public override bool Equals(object o) { TagMapInfo tm = o as TagMapInfo; return StringUtil.Equals(TagType, tm.TagType) && StringUtil.Equals(MappedTagType, tm.MappedTagType); } public override int GetHashCode() { return TagType.GetHashCode() ^ MappedTagType.GetHashCode(); } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("mappedTagType")] [StringValidator(MinLength = 1)] public string MappedTagType { get { return (string)base[_propMappedTagTypeName]; } set { base[_propMappedTagTypeName] = value; } } [ConfigurationProperty("tagType", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string TagType { get { return (string)base[_propTagTypeName]; } set { base[_propTagTypeName] = value; } } void Verify() { if (String.IsNullOrEmpty(TagType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Config_base_required_attribute_missing, "tagType")); } if (String.IsNullOrEmpty(MappedTagType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Config_base_required_attribute_missing, "mappedTagType")); } } protected override bool SerializeElement(XmlWriter writer, bool serializeCollectionKey) { Verify(); return base.SerializeElement(writer, serializeCollectionKey); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.UI; using System.Web.Compilation; using System.Threading; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TagMapInfo : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTagTypeName = new ConfigurationProperty("tagType", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propMappedTagTypeName = new ConfigurationProperty("mappedTagType", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); static TagMapInfo() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTagTypeName); _properties.Add(_propMappedTagTypeName); } internal TagMapInfo() { } public TagMapInfo(String tagTypeName, String mappedTagTypeName) : this() { TagType = tagTypeName; MappedTagType = mappedTagTypeName; } public override bool Equals(object o) { TagMapInfo tm = o as TagMapInfo; return StringUtil.Equals(TagType, tm.TagType) && StringUtil.Equals(MappedTagType, tm.MappedTagType); } public override int GetHashCode() { return TagType.GetHashCode() ^ MappedTagType.GetHashCode(); } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("mappedTagType")] [StringValidator(MinLength = 1)] public string MappedTagType { get { return (string)base[_propMappedTagTypeName]; } set { base[_propMappedTagTypeName] = value; } } [ConfigurationProperty("tagType", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string TagType { get { return (string)base[_propTagTypeName]; } set { base[_propTagTypeName] = value; } } void Verify() { if (String.IsNullOrEmpty(TagType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Config_base_required_attribute_missing, "tagType")); } if (String.IsNullOrEmpty(MappedTagType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Config_base_required_attribute_missing, "mappedTagType")); } } protected override bool SerializeElement(XmlWriter writer, bool serializeCollectionKey) { Verify(); return base.SerializeElement(writer, serializeCollectionKey); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
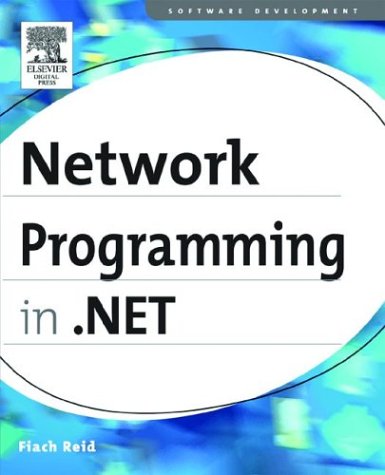
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConditionCollection.cs
- XmlSchemaSimpleTypeList.cs
- OletxVolatileEnlistment.cs
- PermissionSetTriple.cs
- IteratorDescriptor.cs
- OracleColumn.cs
- CodeParameterDeclarationExpression.cs
- SingleAnimation.cs
- DataViewListener.cs
- DrawingContextWalker.cs
- Graph.cs
- CompareValidator.cs
- PromptBuilder.cs
- MediaContextNotificationWindow.cs
- StringCollection.cs
- FtpWebResponse.cs
- DescendentsWalker.cs
- ThreadExceptionDialog.cs
- DrawListViewItemEventArgs.cs
- Profiler.cs
- ModuleBuilderData.cs
- HttpListenerException.cs
- wgx_commands.cs
- ProfileSection.cs
- ListViewSortEventArgs.cs
- InternalResources.cs
- FileUtil.cs
- ListViewItem.cs
- SchemaNotation.cs
- ColumnMapCopier.cs
- TextServicesContext.cs
- BitmapCacheBrush.cs
- SQLSingleStorage.cs
- StringBuilder.cs
- ProtocolsConfigurationHandler.cs
- FixedElement.cs
- SynchronizedInputHelper.cs
- FirstQueryOperator.cs
- MouseEvent.cs
- OptimisticConcurrencyException.cs
- DiscoveryInnerClientAdhoc11.cs
- Oid.cs
- ScanQueryOperator.cs
- WSSecureConversationFeb2005.cs
- ValueSerializerAttribute.cs
- ListItem.cs
- TextEditorThreadLocalStore.cs
- _PooledStream.cs
- SafeEventLogWriteHandle.cs
- DataServiceContext.cs
- AspNetPartialTrustHelpers.cs
- CanonicalizationDriver.cs
- BinHexDecoder.cs
- DataFormats.cs
- WebPartManagerInternals.cs
- DataGridTableCollection.cs
- StylusPointDescription.cs
- CharacterMetrics.cs
- ExpressionBuilder.cs
- PeerInputChannelListener.cs
- OleDbParameterCollection.cs
- GPPOINTF.cs
- AnonymousIdentificationModule.cs
- CodeTypeOfExpression.cs
- ImageList.cs
- EdmValidator.cs
- ZipArchive.cs
- ImageDrawing.cs
- TextMetrics.cs
- DiagnosticsConfiguration.cs
- ResourcesBuildProvider.cs
- WebPartCloseVerb.cs
- GatewayIPAddressInformationCollection.cs
- IntranetCredentialPolicy.cs
- PrimitiveCodeDomSerializer.cs
- FontFaceLayoutInfo.cs
- WebPageTraceListener.cs
- InputProcessorProfilesLoader.cs
- Vector3DCollectionConverter.cs
- HtmlInputFile.cs
- X509ChainPolicy.cs
- SessionStateUtil.cs
- QueryLifecycle.cs
- ActivityTypeResolver.xaml.cs
- CompositeFontParser.cs
- XmlComment.cs
- ReadOnlyDictionary.cs
- GenericTypeParameterBuilder.cs
- WeakEventManager.cs
- ProviderSettings.cs
- WebPartHeaderCloseVerb.cs
- ValueExpressions.cs
- ZipIOCentralDirectoryFileHeader.cs
- DataMemberFieldConverter.cs
- ApplicationSettingsBase.cs
- SqlInternalConnectionSmi.cs
- SHA512Managed.cs
- CellIdBoolean.cs
- DataGridViewColumnHeaderCell.cs
- CollectionView.cs