Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / PrePostDescendentsWalker.cs / 1 / PrePostDescendentsWalker.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A simple subclass of DescendentsWalker which introduces a second callback // which is called after a node's children have been visited. // // History: // 04/13/2004: rruiz: Introduces class. // 10/20/2004: rruiz: Moved class to MS.Internal. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using MS.Utility; namespace MS.Internal { ////// A simple subclass of DescendentsWalker which introduces a second callback /// which is called after a node's children have been visited. /// internal class PrePostDescendentsWalker: DescendentsWalker { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates an instances of PrePostDescendentsWalker. /// /// specifies which tree should be visited first /// the callback to be called before a node's children are visited /// the callback to be called after a node's children are visited /// the data passed to each callback public PrePostDescendentsWalker(TreeWalkPriority priority, VisitedCallbackpreCallback, VisitedCallback postCallback, T data) : base(priority, preCallback, data) { _postCallback = postCallback; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Starts the walking process for the given node. /// /// the node to start the walk on /// whether or not the first node should have the callbacks called on it public override void StartWalk(DependencyObject startNode, bool skipStartNode) { try { base.StartWalk(startNode, skipStartNode); } finally { if (!skipStartNode) { if (_postCallback != null) { // This type checking is done in DescendentsWalker. Doing it here // keeps us consistent. if (FrameworkElement.DType.IsInstanceOfType(startNode) || FrameworkContentElement.DType.IsInstanceOfType(startNode)) { _postCallback(startNode, this.Data); } } } } } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// This method is called for every node touched during a walking of /// the tree. Some nodes may not have this called if the preCallback /// returns false - thereby preventing its subtree from being visited. /// /// the node to visit protected override void _VisitNode(DependencyObject d) { try { base._VisitNode(d); } finally { if (_postCallback != null) { _postCallback(d, this.Data); } } } #endregion Protected Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties private VisitedCallback_postCallback; #endregion Private Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A simple subclass of DescendentsWalker which introduces a second callback // which is called after a node's children have been visited. // // History: // 04/13/2004: rruiz: Introduces class. // 10/20/2004: rruiz: Moved class to MS.Internal. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using MS.Utility; namespace MS.Internal { ////// A simple subclass of DescendentsWalker which introduces a second callback /// which is called after a node's children have been visited. /// internal class PrePostDescendentsWalker: DescendentsWalker { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates an instances of PrePostDescendentsWalker. /// /// specifies which tree should be visited first /// the callback to be called before a node's children are visited /// the callback to be called after a node's children are visited /// the data passed to each callback public PrePostDescendentsWalker(TreeWalkPriority priority, VisitedCallbackpreCallback, VisitedCallback postCallback, T data) : base(priority, preCallback, data) { _postCallback = postCallback; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Starts the walking process for the given node. /// /// the node to start the walk on /// whether or not the first node should have the callbacks called on it public override void StartWalk(DependencyObject startNode, bool skipStartNode) { try { base.StartWalk(startNode, skipStartNode); } finally { if (!skipStartNode) { if (_postCallback != null) { // This type checking is done in DescendentsWalker. Doing it here // keeps us consistent. if (FrameworkElement.DType.IsInstanceOfType(startNode) || FrameworkContentElement.DType.IsInstanceOfType(startNode)) { _postCallback(startNode, this.Data); } } } } } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// This method is called for every node touched during a walking of /// the tree. Some nodes may not have this called if the preCallback /// returns false - thereby preventing its subtree from being visited. /// /// the node to visit protected override void _VisitNode(DependencyObject d) { try { base._VisitNode(d); } finally { if (_postCallback != null) { _postCallback(d, this.Data); } } } #endregion Protected Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties private VisitedCallback_postCallback; #endregion Private Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
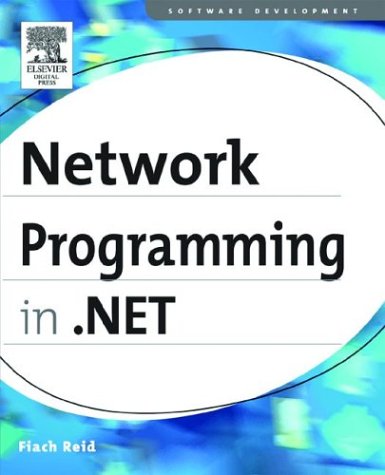
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RadioButtonPopupAdapter.cs
- WsatAdminException.cs
- EventLogInformation.cs
- PenThreadWorker.cs
- AuthorizationSection.cs
- MachineKeyValidationConverter.cs
- _ServiceNameStore.cs
- ConsoleKeyInfo.cs
- XmlNodeList.cs
- DetailsView.cs
- FileDialog.cs
- Cell.cs
- SingleAnimation.cs
- RTTrackingProfile.cs
- RefExpr.cs
- ToolboxComponentsCreatedEventArgs.cs
- BadImageFormatException.cs
- OleDragDropHandler.cs
- OracleConnectionString.cs
- FixedDSBuilder.cs
- PolicyLevel.cs
- TrackingProfileManager.cs
- PartManifestEntry.cs
- PersonalizationStateInfoCollection.cs
- SerializationSectionGroup.cs
- WebPartConnectionsCloseVerb.cs
- ColumnCollection.cs
- CheckableControlBaseAdapter.cs
- SpnegoTokenAuthenticator.cs
- EditingCoordinator.cs
- DictionaryItemsCollection.cs
- ConfigurationConverterBase.cs
- ArraySegment.cs
- EastAsianLunisolarCalendar.cs
- DetailsViewInsertEventArgs.cs
- DeploymentExceptionMapper.cs
- WebInvokeAttribute.cs
- PerformanceCounter.cs
- Scripts.cs
- JpegBitmapEncoder.cs
- MultiBinding.cs
- PageCodeDomTreeGenerator.cs
- X509Certificate.cs
- CodeAttributeArgument.cs
- Material.cs
- Misc.cs
- RegionData.cs
- SessionPageStateSection.cs
- RegexGroup.cs
- BindingMAnagerBase.cs
- ProfileGroupSettingsCollection.cs
- KeyInfo.cs
- COM2ColorConverter.cs
- BaseCollection.cs
- WindowHideOrCloseTracker.cs
- SqlErrorCollection.cs
- Update.cs
- UnaryQueryOperator.cs
- OracleDataAdapter.cs
- DependencyObjectProvider.cs
- ToolboxDataAttribute.cs
- ListViewEditEventArgs.cs
- ChannelProtectionRequirements.cs
- TableTextElementCollectionInternal.cs
- InvalidEnumArgumentException.cs
- DataViewManagerListItemTypeDescriptor.cs
- TypeUtil.cs
- RegexCaptureCollection.cs
- GiveFeedbackEvent.cs
- PingReply.cs
- WpfXamlMember.cs
- FastEncoder.cs
- DataServiceConfiguration.cs
- ModuleElement.cs
- DbProviderManifest.cs
- BaseTreeIterator.cs
- ColorDialog.cs
- PostBackOptions.cs
- WebServiceMethodData.cs
- NotSupportedException.cs
- MyContact.cs
- Point3DCollection.cs
- BooleanFacetDescriptionElement.cs
- OpenFileDialog.cs
- SingleObjectCollection.cs
- ListViewTableRow.cs
- PrefixQName.cs
- SqlCacheDependencyDatabaseCollection.cs
- DataGridColumnDropSeparator.cs
- PropertyValue.cs
- LambdaValue.cs
- Rfc2898DeriveBytes.cs
- IndexedDataBuffer.cs
- HtmlTitle.cs
- Dynamic.cs
- FormViewInsertedEventArgs.cs
- SqlException.cs
- MappingItemCollection.cs
- StickyNoteContentControl.cs
- RandomDelaySendsAsyncResult.cs